DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.
datarobot.ApplicationSource
Explore with Pulumi AI
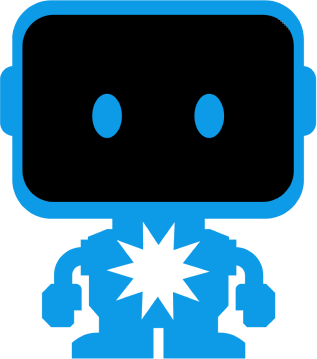
DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.
Application Source
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as datarobot from "@datarobot/pulumi-datarobot";
const example = new datarobot.ApplicationSource("example", {files: [
["start-app.sh"],
["streamlit-app.py"],
]});
export const datarobotApplicationSourceId = example.id;
export const datarobotApplicationSourceVersionId = example.versionId;
import pulumi
import pulumi_datarobot as datarobot
example = datarobot.ApplicationSource("example", files=[
["start-app.sh"],
["streamlit-app.py"],
])
pulumi.export("datarobotApplicationSourceId", example.id)
pulumi.export("datarobotApplicationSourceVersionId", example.version_id)
package main
import (
"github.com/datarobot-community/pulumi-datarobot/sdk/go/datarobot"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
example, err := datarobot.NewApplicationSource(ctx, "example", &datarobot.ApplicationSourceArgs{
Files: pulumi.Any{
[]string{
"start-app.sh",
},
[]string{
"streamlit-app.py",
},
},
})
if err != nil {
return err
}
ctx.Export("datarobotApplicationSourceId", example.ID())
ctx.Export("datarobotApplicationSourceVersionId", example.VersionId)
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Datarobot = DataRobotPulumi.Datarobot;
return await Deployment.RunAsync(() =>
{
var example = new Datarobot.ApplicationSource("example", new()
{
Files = new[]
{
new[]
{
"start-app.sh",
},
new[]
{
"streamlit-app.py",
},
},
});
return new Dictionary<string, object?>
{
["datarobotApplicationSourceId"] = example.Id,
["datarobotApplicationSourceVersionId"] = example.VersionId,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.datarobot.ApplicationSource;
import com.pulumi.datarobot.ApplicationSourceArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var example = new ApplicationSource("example", ApplicationSourceArgs.builder()
.files(
"start-app.sh",
"streamlit-app.py")
.build());
ctx.export("datarobotApplicationSourceId", example.id());
ctx.export("datarobotApplicationSourceVersionId", example.versionId());
}
}
resources:
example:
type: datarobot:ApplicationSource
properties:
files:
- - start-app.sh
- - streamlit-app.py
outputs:
datarobotApplicationSourceId: ${example.id}
datarobotApplicationSourceVersionId: ${example.versionId}
Create ApplicationSource Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new ApplicationSource(name: string, args?: ApplicationSourceArgs, opts?: CustomResourceOptions);
@overload
def ApplicationSource(resource_name: str,
args: Optional[ApplicationSourceArgs] = None,
opts: Optional[ResourceOptions] = None)
@overload
def ApplicationSource(resource_name: str,
opts: Optional[ResourceOptions] = None,
files: Optional[Any] = None,
folder_path: Optional[str] = None,
name: Optional[str] = None,
resource_settings: Optional[ApplicationSourceResourceSettingsArgs] = None,
runtime_parameter_values: Optional[Sequence[ApplicationSourceRuntimeParameterValueArgs]] = None)
func NewApplicationSource(ctx *Context, name string, args *ApplicationSourceArgs, opts ...ResourceOption) (*ApplicationSource, error)
public ApplicationSource(string name, ApplicationSourceArgs? args = null, CustomResourceOptions? opts = null)
public ApplicationSource(String name, ApplicationSourceArgs args)
public ApplicationSource(String name, ApplicationSourceArgs args, CustomResourceOptions options)
type: datarobot:ApplicationSource
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args ApplicationSourceArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args ApplicationSourceArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args ApplicationSourceArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args ApplicationSourceArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args ApplicationSourceArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var applicationSourceResource = new Datarobot.ApplicationSource("applicationSourceResource", new()
{
Files = "any",
FolderPath = "string",
Name = "string",
ResourceSettings = new Datarobot.Inputs.ApplicationSourceResourceSettingsArgs
{
Replicas = 0,
},
RuntimeParameterValues = new[]
{
new Datarobot.Inputs.ApplicationSourceRuntimeParameterValueArgs
{
Key = "string",
Type = "string",
Value = "string",
},
},
});
example, err := datarobot.NewApplicationSource(ctx, "applicationSourceResource", &datarobot.ApplicationSourceArgs{
Files: pulumi.Any("any"),
FolderPath: pulumi.String("string"),
Name: pulumi.String("string"),
ResourceSettings: &datarobot.ApplicationSourceResourceSettingsArgs{
Replicas: pulumi.Int(0),
},
RuntimeParameterValues: datarobot.ApplicationSourceRuntimeParameterValueArray{
&datarobot.ApplicationSourceRuntimeParameterValueArgs{
Key: pulumi.String("string"),
Type: pulumi.String("string"),
Value: pulumi.String("string"),
},
},
})
var applicationSourceResource = new ApplicationSource("applicationSourceResource", ApplicationSourceArgs.builder()
.files("any")
.folderPath("string")
.name("string")
.resourceSettings(ApplicationSourceResourceSettingsArgs.builder()
.replicas(0)
.build())
.runtimeParameterValues(ApplicationSourceRuntimeParameterValueArgs.builder()
.key("string")
.type("string")
.value("string")
.build())
.build());
application_source_resource = datarobot.ApplicationSource("applicationSourceResource",
files="any",
folder_path="string",
name="string",
resource_settings=datarobot.ApplicationSourceResourceSettingsArgs(
replicas=0,
),
runtime_parameter_values=[datarobot.ApplicationSourceRuntimeParameterValueArgs(
key="string",
type="string",
value="string",
)])
const applicationSourceResource = new datarobot.ApplicationSource("applicationSourceResource", {
files: "any",
folderPath: "string",
name: "string",
resourceSettings: {
replicas: 0,
},
runtimeParameterValues: [{
key: "string",
type: "string",
value: "string",
}],
});
type: datarobot:ApplicationSource
properties:
files: any
folderPath: string
name: string
resourceSettings:
replicas: 0
runtimeParameterValues:
- key: string
type: string
value: string
ApplicationSource Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The ApplicationSource resource accepts the following input properties:
- Files object
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Application Source. If list is of strings, then basenames will be used for tuples.
- Folder
Path string - The path to a folder containing files to build the Application Source. Each file in the folder is uploaded under path relative to a folder path.
- Name string
- The name of the Application Source.
- Resource
Settings DataRobot Application Source Resource Settings - The resource settings for the Application Source.
- Runtime
Parameter List<DataValues Robot Application Source Runtime Parameter Value> - The runtime parameter values for the Application Source.
- Files interface{}
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Application Source. If list is of strings, then basenames will be used for tuples.
- Folder
Path string - The path to a folder containing files to build the Application Source. Each file in the folder is uploaded under path relative to a folder path.
- Name string
- The name of the Application Source.
- Resource
Settings ApplicationSource Resource Settings Args - The resource settings for the Application Source.
- Runtime
Parameter []ApplicationValues Source Runtime Parameter Value Args - The runtime parameter values for the Application Source.
- files Object
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Application Source. If list is of strings, then basenames will be used for tuples.
- folder
Path String - The path to a folder containing files to build the Application Source. Each file in the folder is uploaded under path relative to a folder path.
- name String
- The name of the Application Source.
- resource
Settings ApplicationSource Resource Settings - The resource settings for the Application Source.
- runtime
Parameter List<ApplicationValues Source Runtime Parameter Value> - The runtime parameter values for the Application Source.
- files any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Application Source. If list is of strings, then basenames will be used for tuples.
- folder
Path string - The path to a folder containing files to build the Application Source. Each file in the folder is uploaded under path relative to a folder path.
- name string
- The name of the Application Source.
- resource
Settings ApplicationSource Resource Settings - The resource settings for the Application Source.
- runtime
Parameter ApplicationValues Source Runtime Parameter Value[] - The runtime parameter values for the Application Source.
- files Any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Application Source. If list is of strings, then basenames will be used for tuples.
- folder_
path str - The path to a folder containing files to build the Application Source. Each file in the folder is uploaded under path relative to a folder path.
- name str
- The name of the Application Source.
- resource_
settings ApplicationSource Resource Settings Args - The resource settings for the Application Source.
- runtime_
parameter_ Sequence[Applicationvalues Source Runtime Parameter Value Args] - The runtime parameter values for the Application Source.
- files Any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Application Source. If list is of strings, then basenames will be used for tuples.
- folder
Path String - The path to a folder containing files to build the Application Source. Each file in the folder is uploaded under path relative to a folder path.
- name String
- The name of the Application Source.
- resource
Settings Property Map - The resource settings for the Application Source.
- runtime
Parameter List<Property Map>Values - The runtime parameter values for the Application Source.
Outputs
All input properties are implicitly available as output properties. Additionally, the ApplicationSource resource produces the following output properties:
- id str
- The provider-assigned unique ID for this managed resource.
- version_
id str - The version ID of the Application Source.
Look up Existing ApplicationSource Resource
Get an existing ApplicationSource resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: ApplicationSourceState, opts?: CustomResourceOptions): ApplicationSource
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
files: Optional[Any] = None,
folder_path: Optional[str] = None,
name: Optional[str] = None,
resource_settings: Optional[ApplicationSourceResourceSettingsArgs] = None,
runtime_parameter_values: Optional[Sequence[ApplicationSourceRuntimeParameterValueArgs]] = None,
version_id: Optional[str] = None) -> ApplicationSource
func GetApplicationSource(ctx *Context, name string, id IDInput, state *ApplicationSourceState, opts ...ResourceOption) (*ApplicationSource, error)
public static ApplicationSource Get(string name, Input<string> id, ApplicationSourceState? state, CustomResourceOptions? opts = null)
public static ApplicationSource get(String name, Output<String> id, ApplicationSourceState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Files object
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Application Source. If list is of strings, then basenames will be used for tuples.
- Folder
Path string - The path to a folder containing files to build the Application Source. Each file in the folder is uploaded under path relative to a folder path.
- Name string
- The name of the Application Source.
- Resource
Settings DataRobot Application Source Resource Settings - The resource settings for the Application Source.
- Runtime
Parameter List<DataValues Robot Application Source Runtime Parameter Value> - The runtime parameter values for the Application Source.
- Version
Id string - The version ID of the Application Source.
- Files interface{}
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Application Source. If list is of strings, then basenames will be used for tuples.
- Folder
Path string - The path to a folder containing files to build the Application Source. Each file in the folder is uploaded under path relative to a folder path.
- Name string
- The name of the Application Source.
- Resource
Settings ApplicationSource Resource Settings Args - The resource settings for the Application Source.
- Runtime
Parameter []ApplicationValues Source Runtime Parameter Value Args - The runtime parameter values for the Application Source.
- Version
Id string - The version ID of the Application Source.
- files Object
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Application Source. If list is of strings, then basenames will be used for tuples.
- folder
Path String - The path to a folder containing files to build the Application Source. Each file in the folder is uploaded under path relative to a folder path.
- name String
- The name of the Application Source.
- resource
Settings ApplicationSource Resource Settings - The resource settings for the Application Source.
- runtime
Parameter List<ApplicationValues Source Runtime Parameter Value> - The runtime parameter values for the Application Source.
- version
Id String - The version ID of the Application Source.
- files any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Application Source. If list is of strings, then basenames will be used for tuples.
- folder
Path string - The path to a folder containing files to build the Application Source. Each file in the folder is uploaded under path relative to a folder path.
- name string
- The name of the Application Source.
- resource
Settings ApplicationSource Resource Settings - The resource settings for the Application Source.
- runtime
Parameter ApplicationValues Source Runtime Parameter Value[] - The runtime parameter values for the Application Source.
- version
Id string - The version ID of the Application Source.
- files Any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Application Source. If list is of strings, then basenames will be used for tuples.
- folder_
path str - The path to a folder containing files to build the Application Source. Each file in the folder is uploaded under path relative to a folder path.
- name str
- The name of the Application Source.
- resource_
settings ApplicationSource Resource Settings Args - The resource settings for the Application Source.
- runtime_
parameter_ Sequence[Applicationvalues Source Runtime Parameter Value Args] - The runtime parameter values for the Application Source.
- version_
id str - The version ID of the Application Source.
- files Any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Application Source. If list is of strings, then basenames will be used for tuples.
- folder
Path String - The path to a folder containing files to build the Application Source. Each file in the folder is uploaded under path relative to a folder path.
- name String
- The name of the Application Source.
- resource
Settings Property Map - The resource settings for the Application Source.
- runtime
Parameter List<Property Map>Values - The runtime parameter values for the Application Source.
- version
Id String - The version ID of the Application Source.
Supporting Types
ApplicationSourceResourceSettings, ApplicationSourceResourceSettingsArgs
- Replicas int
- The replicas for the Application Source.
- Replicas int
- The replicas for the Application Source.
- replicas Integer
- The replicas for the Application Source.
- replicas number
- The replicas for the Application Source.
- replicas int
- The replicas for the Application Source.
- replicas Number
- The replicas for the Application Source.
ApplicationSourceRuntimeParameterValue, ApplicationSourceRuntimeParameterValueArgs
Package Details
- Repository
- datarobot datarobot-community/pulumi-datarobot
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
datarobot
Terraform Provider.
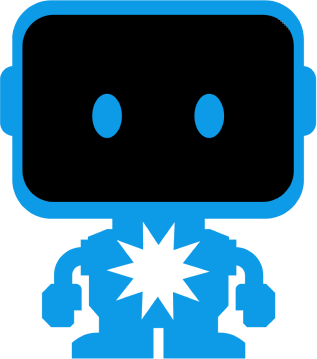
DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.