DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.
datarobot.CustomModel
Explore with Pulumi AI
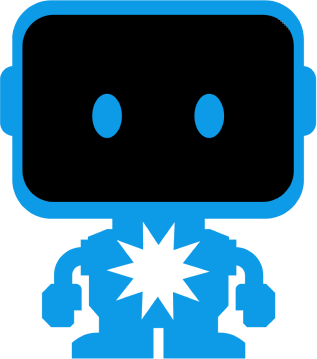
DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.
Data set from file
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as datarobot from "@datarobot/pulumi-datarobot";
const exampleRemoteRepository = new datarobot.RemoteRepository("exampleRemoteRepository", {
description: "GitHub repository with Datarobot user models",
location: "https://github.com/datarobot/datarobot-user-models",
sourceType: "github",
});
// set the credential id for private repositories
// credential_id = datarobot_api_token_credential.example.id
const exampleCustomModel = new datarobot.CustomModel("exampleCustomModel", {
description: "An example custom model from GitHub repository",
files: [
"file1.py",
"file2.py",
],
targetType: "Binary",
targetName: "my_label",
baseEnvironmentName: "[GenAI] Python 3.11 with Moderations",
});
// Optional
// source_remote_repositories = [
// {
// id = datarobot_remote_repository.example.id
// ref = "master"
// source_paths = [
// "model_templates/python3_dummy_binary",
// ]
// }
// ]
// guard_configurations = [
// {
// template_name = "Rouge 1"
// name = "Rouge 1 response"
// stages = ["response"]
// intervention = {
// action = "block"
// message = "response has been blocked by Rogue 1 guard"
// condition = {
// comparand = 0.8
// comparator = "lessThan"
// }
// }
// },
// ]
// overall_moderation_configuration = {
// timeout_sec = 120
// timeout_action = "score"
// }
// resource_settings = {
// memory_mb = 512
// replicas = 2
// network_access = "NONE"
// }
export const exampleId = exampleCustomModel.id;
import pulumi
import pulumi_datarobot as datarobot
example_remote_repository = datarobot.RemoteRepository("exampleRemoteRepository",
description="GitHub repository with Datarobot user models",
location="https://github.com/datarobot/datarobot-user-models",
source_type="github")
# set the credential id for private repositories
# credential_id = datarobot_api_token_credential.example.id
example_custom_model = datarobot.CustomModel("exampleCustomModel",
description="An example custom model from GitHub repository",
files=[
"file1.py",
"file2.py",
],
target_type="Binary",
target_name="my_label",
base_environment_name="[GenAI] Python 3.11 with Moderations")
# Optional
# source_remote_repositories = [
# {
# id = datarobot_remote_repository.example.id
# ref = "master"
# source_paths = [
# "model_templates/python3_dummy_binary",
# ]
# }
# ]
# guard_configurations = [
# {
# template_name = "Rouge 1"
# name = "Rouge 1 response"
# stages = ["response"]
# intervention = {
# action = "block"
# message = "response has been blocked by Rogue 1 guard"
# condition = {
# comparand = 0.8
# comparator = "lessThan"
# }
# }
# },
# ]
# overall_moderation_configuration = {
# timeout_sec = 120
# timeout_action = "score"
# }
# resource_settings = {
# memory_mb = 512
# replicas = 2
# network_access = "NONE"
# }
pulumi.export("exampleId", example_custom_model.id)
package main
import (
"github.com/datarobot-community/pulumi-datarobot/sdk/go/datarobot"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := datarobot.NewRemoteRepository(ctx, "exampleRemoteRepository", &datarobot.RemoteRepositoryArgs{
Description: pulumi.String("GitHub repository with Datarobot user models"),
Location: pulumi.String("https://github.com/datarobot/datarobot-user-models"),
SourceType: pulumi.String("github"),
})
if err != nil {
return err
}
exampleCustomModel, err := datarobot.NewCustomModel(ctx, "exampleCustomModel", &datarobot.CustomModelArgs{
Description: pulumi.String("An example custom model from GitHub repository"),
Files: pulumi.Any{
"file1.py",
"file2.py",
},
TargetType: pulumi.String("Binary"),
TargetName: pulumi.String("my_label"),
BaseEnvironmentName: pulumi.String("[GenAI] Python 3.11 with Moderations"),
})
if err != nil {
return err
}
ctx.Export("exampleId", exampleCustomModel.ID())
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Datarobot = DataRobotPulumi.Datarobot;
return await Deployment.RunAsync(() =>
{
var exampleRemoteRepository = new Datarobot.RemoteRepository("exampleRemoteRepository", new()
{
Description = "GitHub repository with Datarobot user models",
Location = "https://github.com/datarobot/datarobot-user-models",
SourceType = "github",
});
// set the credential id for private repositories
// credential_id = datarobot_api_token_credential.example.id
var exampleCustomModel = new Datarobot.CustomModel("exampleCustomModel", new()
{
Description = "An example custom model from GitHub repository",
Files = new[]
{
"file1.py",
"file2.py",
},
TargetType = "Binary",
TargetName = "my_label",
BaseEnvironmentName = "[GenAI] Python 3.11 with Moderations",
});
// Optional
// source_remote_repositories = [
// {
// id = datarobot_remote_repository.example.id
// ref = "master"
// source_paths = [
// "model_templates/python3_dummy_binary",
// ]
// }
// ]
// guard_configurations = [
// {
// template_name = "Rouge 1"
// name = "Rouge 1 response"
// stages = ["response"]
// intervention = {
// action = "block"
// message = "response has been blocked by Rogue 1 guard"
// condition = {
// comparand = 0.8
// comparator = "lessThan"
// }
// }
// },
// ]
// overall_moderation_configuration = {
// timeout_sec = 120
// timeout_action = "score"
// }
// resource_settings = {
// memory_mb = 512
// replicas = 2
// network_access = "NONE"
// }
return new Dictionary<string, object?>
{
["exampleId"] = exampleCustomModel.Id,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.datarobot.RemoteRepository;
import com.pulumi.datarobot.RemoteRepositoryArgs;
import com.pulumi.datarobot.CustomModel;
import com.pulumi.datarobot.CustomModelArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var exampleRemoteRepository = new RemoteRepository("exampleRemoteRepository", RemoteRepositoryArgs.builder()
.description("GitHub repository with Datarobot user models")
.location("https://github.com/datarobot/datarobot-user-models")
.sourceType("github")
.build());
// set the credential id for private repositories
// credential_id = datarobot_api_token_credential.example.id
var exampleCustomModel = new CustomModel("exampleCustomModel", CustomModelArgs.builder()
.description("An example custom model from GitHub repository")
.files(
"file1.py",
"file2.py")
.targetType("Binary")
.targetName("my_label")
.baseEnvironmentName("[GenAI] Python 3.11 with Moderations")
.build());
// Optional
// source_remote_repositories = [
// {
// id = datarobot_remote_repository.example.id
// ref = "master"
// source_paths = [
// "model_templates/python3_dummy_binary",
// ]
// }
// ]
// guard_configurations = [
// {
// template_name = "Rouge 1"
// name = "Rouge 1 response"
// stages = ["response"]
// intervention = {
// action = "block"
// message = "response has been blocked by Rogue 1 guard"
// condition = {
// comparand = 0.8
// comparator = "lessThan"
// }
// }
// },
// ]
// overall_moderation_configuration = {
// timeout_sec = 120
// timeout_action = "score"
// }
// resource_settings = {
// memory_mb = 512
// replicas = 2
// network_access = "NONE"
// }
ctx.export("exampleId", exampleCustomModel.id());
}
}
resources:
exampleRemoteRepository:
type: datarobot:RemoteRepository
properties:
description: GitHub repository with Datarobot user models
location: https://github.com/datarobot/datarobot-user-models
sourceType: github
exampleCustomModel:
type: datarobot:CustomModel
properties:
description: An example custom model from GitHub repository
files:
- file1.py
- file2.py
targetType: Binary
targetName: my_label
baseEnvironmentName: '[GenAI] Python 3.11 with Moderations'
outputs:
exampleId: ${exampleCustomModel.id}
Create CustomModel Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new CustomModel(name: string, args?: CustomModelArgs, opts?: CustomResourceOptions);
@overload
def CustomModel(resource_name: str,
args: Optional[CustomModelArgs] = None,
opts: Optional[ResourceOptions] = None)
@overload
def CustomModel(resource_name: str,
opts: Optional[ResourceOptions] = None,
base_environment_id: Optional[str] = None,
base_environment_name: Optional[str] = None,
base_environment_version_id: Optional[str] = None,
class_labels: Optional[Sequence[str]] = None,
class_labels_file: Optional[str] = None,
description: Optional[str] = None,
files: Optional[Any] = None,
folder_path: Optional[str] = None,
guard_configurations: Optional[Sequence[CustomModelGuardConfigurationArgs]] = None,
is_proxy: Optional[bool] = None,
language: Optional[str] = None,
name: Optional[str] = None,
negative_class_label: Optional[str] = None,
overall_moderation_configuration: Optional[CustomModelOverallModerationConfigurationArgs] = None,
positive_class_label: Optional[str] = None,
prediction_threshold: Optional[float] = None,
resource_settings: Optional[CustomModelResourceSettingsArgs] = None,
runtime_parameter_values: Optional[Sequence[CustomModelRuntimeParameterValueArgs]] = None,
source_llm_blueprint_id: Optional[str] = None,
source_remote_repositories: Optional[Sequence[CustomModelSourceRemoteRepositoryArgs]] = None,
target_name: Optional[str] = None,
target_type: Optional[str] = None,
training_data_partition_column: Optional[str] = None,
training_dataset_id: Optional[str] = None)
func NewCustomModel(ctx *Context, name string, args *CustomModelArgs, opts ...ResourceOption) (*CustomModel, error)
public CustomModel(string name, CustomModelArgs? args = null, CustomResourceOptions? opts = null)
public CustomModel(String name, CustomModelArgs args)
public CustomModel(String name, CustomModelArgs args, CustomResourceOptions options)
type: datarobot:CustomModel
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args CustomModelArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args CustomModelArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args CustomModelArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args CustomModelArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args CustomModelArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var customModelResource = new Datarobot.CustomModel("customModelResource", new()
{
BaseEnvironmentId = "string",
BaseEnvironmentName = "string",
BaseEnvironmentVersionId = "string",
ClassLabels = new[]
{
"string",
},
ClassLabelsFile = "string",
Description = "string",
Files = "any",
FolderPath = "string",
GuardConfigurations = new[]
{
new Datarobot.Inputs.CustomModelGuardConfigurationArgs
{
Intervention = new Datarobot.Inputs.CustomModelGuardConfigurationInterventionArgs
{
Action = "string",
Condition = new Datarobot.Inputs.CustomModelGuardConfigurationInterventionConditionArgs
{
Comparand = 0,
Comparator = "string",
},
Message = "string",
},
Name = "string",
Stages = new[]
{
"string",
},
TemplateName = "string",
DeploymentId = "string",
InputColumnName = "string",
LlmType = "string",
OpenaiApiBase = "string",
OpenaiCredential = "string",
OpenaiDeploymentId = "string",
OutputColumnName = "string",
},
},
IsProxy = false,
Language = "string",
Name = "string",
NegativeClassLabel = "string",
OverallModerationConfiguration = new Datarobot.Inputs.CustomModelOverallModerationConfigurationArgs
{
TimeoutAction = "string",
TimeoutSec = 0,
},
PositiveClassLabel = "string",
PredictionThreshold = 0,
ResourceSettings = new Datarobot.Inputs.CustomModelResourceSettingsArgs
{
MemoryMb = 0,
NetworkAccess = "string",
Replicas = 0,
},
RuntimeParameterValues = new[]
{
new Datarobot.Inputs.CustomModelRuntimeParameterValueArgs
{
Key = "string",
Type = "string",
Value = "string",
},
},
SourceLlmBlueprintId = "string",
SourceRemoteRepositories = new[]
{
new Datarobot.Inputs.CustomModelSourceRemoteRepositoryArgs
{
Id = "string",
Ref = "string",
SourcePaths = new[]
{
"string",
},
},
},
TargetName = "string",
TargetType = "string",
TrainingDataPartitionColumn = "string",
TrainingDatasetId = "string",
});
example, err := datarobot.NewCustomModel(ctx, "customModelResource", &datarobot.CustomModelArgs{
BaseEnvironmentId: pulumi.String("string"),
BaseEnvironmentName: pulumi.String("string"),
BaseEnvironmentVersionId: pulumi.String("string"),
ClassLabels: pulumi.StringArray{
pulumi.String("string"),
},
ClassLabelsFile: pulumi.String("string"),
Description: pulumi.String("string"),
Files: pulumi.Any("any"),
FolderPath: pulumi.String("string"),
GuardConfigurations: datarobot.CustomModelGuardConfigurationArray{
&datarobot.CustomModelGuardConfigurationArgs{
Intervention: &datarobot.CustomModelGuardConfigurationInterventionArgs{
Action: pulumi.String("string"),
Condition: &datarobot.CustomModelGuardConfigurationInterventionConditionArgs{
Comparand: pulumi.Float64(0),
Comparator: pulumi.String("string"),
},
Message: pulumi.String("string"),
},
Name: pulumi.String("string"),
Stages: pulumi.StringArray{
pulumi.String("string"),
},
TemplateName: pulumi.String("string"),
DeploymentId: pulumi.String("string"),
InputColumnName: pulumi.String("string"),
LlmType: pulumi.String("string"),
OpenaiApiBase: pulumi.String("string"),
OpenaiCredential: pulumi.String("string"),
OpenaiDeploymentId: pulumi.String("string"),
OutputColumnName: pulumi.String("string"),
},
},
IsProxy: pulumi.Bool(false),
Language: pulumi.String("string"),
Name: pulumi.String("string"),
NegativeClassLabel: pulumi.String("string"),
OverallModerationConfiguration: &datarobot.CustomModelOverallModerationConfigurationArgs{
TimeoutAction: pulumi.String("string"),
TimeoutSec: pulumi.Int(0),
},
PositiveClassLabel: pulumi.String("string"),
PredictionThreshold: pulumi.Float64(0),
ResourceSettings: &datarobot.CustomModelResourceSettingsArgs{
MemoryMb: pulumi.Int(0),
NetworkAccess: pulumi.String("string"),
Replicas: pulumi.Int(0),
},
RuntimeParameterValues: datarobot.CustomModelRuntimeParameterValueArray{
&datarobot.CustomModelRuntimeParameterValueArgs{
Key: pulumi.String("string"),
Type: pulumi.String("string"),
Value: pulumi.String("string"),
},
},
SourceLlmBlueprintId: pulumi.String("string"),
SourceRemoteRepositories: datarobot.CustomModelSourceRemoteRepositoryArray{
&datarobot.CustomModelSourceRemoteRepositoryArgs{
Id: pulumi.String("string"),
Ref: pulumi.String("string"),
SourcePaths: pulumi.StringArray{
pulumi.String("string"),
},
},
},
TargetName: pulumi.String("string"),
TargetType: pulumi.String("string"),
TrainingDataPartitionColumn: pulumi.String("string"),
TrainingDatasetId: pulumi.String("string"),
})
var customModelResource = new CustomModel("customModelResource", CustomModelArgs.builder()
.baseEnvironmentId("string")
.baseEnvironmentName("string")
.baseEnvironmentVersionId("string")
.classLabels("string")
.classLabelsFile("string")
.description("string")
.files("any")
.folderPath("string")
.guardConfigurations(CustomModelGuardConfigurationArgs.builder()
.intervention(CustomModelGuardConfigurationInterventionArgs.builder()
.action("string")
.condition(CustomModelGuardConfigurationInterventionConditionArgs.builder()
.comparand(0)
.comparator("string")
.build())
.message("string")
.build())
.name("string")
.stages("string")
.templateName("string")
.deploymentId("string")
.inputColumnName("string")
.llmType("string")
.openaiApiBase("string")
.openaiCredential("string")
.openaiDeploymentId("string")
.outputColumnName("string")
.build())
.isProxy(false)
.language("string")
.name("string")
.negativeClassLabel("string")
.overallModerationConfiguration(CustomModelOverallModerationConfigurationArgs.builder()
.timeoutAction("string")
.timeoutSec(0)
.build())
.positiveClassLabel("string")
.predictionThreshold(0)
.resourceSettings(CustomModelResourceSettingsArgs.builder()
.memoryMb(0)
.networkAccess("string")
.replicas(0)
.build())
.runtimeParameterValues(CustomModelRuntimeParameterValueArgs.builder()
.key("string")
.type("string")
.value("string")
.build())
.sourceLlmBlueprintId("string")
.sourceRemoteRepositories(CustomModelSourceRemoteRepositoryArgs.builder()
.id("string")
.ref("string")
.sourcePaths("string")
.build())
.targetName("string")
.targetType("string")
.trainingDataPartitionColumn("string")
.trainingDatasetId("string")
.build());
custom_model_resource = datarobot.CustomModel("customModelResource",
base_environment_id="string",
base_environment_name="string",
base_environment_version_id="string",
class_labels=["string"],
class_labels_file="string",
description="string",
files="any",
folder_path="string",
guard_configurations=[datarobot.CustomModelGuardConfigurationArgs(
intervention=datarobot.CustomModelGuardConfigurationInterventionArgs(
action="string",
condition=datarobot.CustomModelGuardConfigurationInterventionConditionArgs(
comparand=0,
comparator="string",
),
message="string",
),
name="string",
stages=["string"],
template_name="string",
deployment_id="string",
input_column_name="string",
llm_type="string",
openai_api_base="string",
openai_credential="string",
openai_deployment_id="string",
output_column_name="string",
)],
is_proxy=False,
language="string",
name="string",
negative_class_label="string",
overall_moderation_configuration=datarobot.CustomModelOverallModerationConfigurationArgs(
timeout_action="string",
timeout_sec=0,
),
positive_class_label="string",
prediction_threshold=0,
resource_settings=datarobot.CustomModelResourceSettingsArgs(
memory_mb=0,
network_access="string",
replicas=0,
),
runtime_parameter_values=[datarobot.CustomModelRuntimeParameterValueArgs(
key="string",
type="string",
value="string",
)],
source_llm_blueprint_id="string",
source_remote_repositories=[datarobot.CustomModelSourceRemoteRepositoryArgs(
id="string",
ref="string",
source_paths=["string"],
)],
target_name="string",
target_type="string",
training_data_partition_column="string",
training_dataset_id="string")
const customModelResource = new datarobot.CustomModel("customModelResource", {
baseEnvironmentId: "string",
baseEnvironmentName: "string",
baseEnvironmentVersionId: "string",
classLabels: ["string"],
classLabelsFile: "string",
description: "string",
files: "any",
folderPath: "string",
guardConfigurations: [{
intervention: {
action: "string",
condition: {
comparand: 0,
comparator: "string",
},
message: "string",
},
name: "string",
stages: ["string"],
templateName: "string",
deploymentId: "string",
inputColumnName: "string",
llmType: "string",
openaiApiBase: "string",
openaiCredential: "string",
openaiDeploymentId: "string",
outputColumnName: "string",
}],
isProxy: false,
language: "string",
name: "string",
negativeClassLabel: "string",
overallModerationConfiguration: {
timeoutAction: "string",
timeoutSec: 0,
},
positiveClassLabel: "string",
predictionThreshold: 0,
resourceSettings: {
memoryMb: 0,
networkAccess: "string",
replicas: 0,
},
runtimeParameterValues: [{
key: "string",
type: "string",
value: "string",
}],
sourceLlmBlueprintId: "string",
sourceRemoteRepositories: [{
id: "string",
ref: "string",
sourcePaths: ["string"],
}],
targetName: "string",
targetType: "string",
trainingDataPartitionColumn: "string",
trainingDatasetId: "string",
});
type: datarobot:CustomModel
properties:
baseEnvironmentId: string
baseEnvironmentName: string
baseEnvironmentVersionId: string
classLabels:
- string
classLabelsFile: string
description: string
files: any
folderPath: string
guardConfigurations:
- deploymentId: string
inputColumnName: string
intervention:
action: string
condition:
comparand: 0
comparator: string
message: string
llmType: string
name: string
openaiApiBase: string
openaiCredential: string
openaiDeploymentId: string
outputColumnName: string
stages:
- string
templateName: string
isProxy: false
language: string
name: string
negativeClassLabel: string
overallModerationConfiguration:
timeoutAction: string
timeoutSec: 0
positiveClassLabel: string
predictionThreshold: 0
resourceSettings:
memoryMb: 0
networkAccess: string
replicas: 0
runtimeParameterValues:
- key: string
type: string
value: string
sourceLlmBlueprintId: string
sourceRemoteRepositories:
- id: string
ref: string
sourcePaths:
- string
targetName: string
targetType: string
trainingDataPartitionColumn: string
trainingDatasetId: string
CustomModel Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The CustomModel resource accepts the following input properties:
- Base
Environment stringId - The ID of the base environment for the Custom Model.
- Base
Environment stringName - The name of the base environment for the Custom Model.
- Base
Environment stringVersion Id - The ID of the base environment version for the Custom Model.
- Class
Labels List<string> - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- Class
Labels stringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- Description string
- The description of the Custom Model.
- Files object
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- Folder
Path string - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- Guard
Configurations List<DataRobot Custom Model Guard Configuration> - The guard configurations for the Custom Model.
- Is
Proxy bool - Flag indicating if the Custom Model is a proxy model.
- Language string
- The language used to build the Custom Model.
- Name string
- The name of the Custom Model.
- Negative
Class stringLabel - The negative class label of the Custom Model.
- Overall
Moderation DataConfiguration Robot Custom Model Overall Moderation Configuration - The overall moderation configuration for the Custom Model.
- Positive
Class stringLabel - The positive class label of the Custom Model.
- Prediction
Threshold double - The prediction threshold of the Custom Model.
- Resource
Settings DataRobot Custom Model Resource Settings - The resource settings for the Custom Model.
- Runtime
Parameter List<DataValues Robot Custom Model Runtime Parameter Value> - The runtime parameter values for the Custom Model.
- Source
Llm stringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- Source
Remote List<DataRepositories Robot Custom Model Source Remote Repository> - The source remote repositories for the Custom Model.
- Target
Name string - The target name of the Custom Model.
- Target
Type string - The target type of the Custom Model.
- Training
Data stringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- Training
Dataset stringId - The ID of the training dataset assigned to the Custom Model.
- Base
Environment stringId - The ID of the base environment for the Custom Model.
- Base
Environment stringName - The name of the base environment for the Custom Model.
- Base
Environment stringVersion Id - The ID of the base environment version for the Custom Model.
- Class
Labels []string - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- Class
Labels stringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- Description string
- The description of the Custom Model.
- Files interface{}
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- Folder
Path string - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- Guard
Configurations []CustomModel Guard Configuration Args - The guard configurations for the Custom Model.
- Is
Proxy bool - Flag indicating if the Custom Model is a proxy model.
- Language string
- The language used to build the Custom Model.
- Name string
- The name of the Custom Model.
- Negative
Class stringLabel - The negative class label of the Custom Model.
- Overall
Moderation CustomConfiguration Model Overall Moderation Configuration Args - The overall moderation configuration for the Custom Model.
- Positive
Class stringLabel - The positive class label of the Custom Model.
- Prediction
Threshold float64 - The prediction threshold of the Custom Model.
- Resource
Settings CustomModel Resource Settings Args - The resource settings for the Custom Model.
- Runtime
Parameter []CustomValues Model Runtime Parameter Value Args - The runtime parameter values for the Custom Model.
- Source
Llm stringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- Source
Remote []CustomRepositories Model Source Remote Repository Args - The source remote repositories for the Custom Model.
- Target
Name string - The target name of the Custom Model.
- Target
Type string - The target type of the Custom Model.
- Training
Data stringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- Training
Dataset stringId - The ID of the training dataset assigned to the Custom Model.
- base
Environment StringId - The ID of the base environment for the Custom Model.
- base
Environment StringName - The name of the base environment for the Custom Model.
- base
Environment StringVersion Id - The ID of the base environment version for the Custom Model.
- class
Labels List<String> - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class
Labels StringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- description String
- The description of the Custom Model.
- files Object
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- folder
Path String - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- guard
Configurations List<CustomModel Guard Configuration> - The guard configurations for the Custom Model.
- is
Proxy Boolean - Flag indicating if the Custom Model is a proxy model.
- language String
- The language used to build the Custom Model.
- name String
- The name of the Custom Model.
- negative
Class StringLabel - The negative class label of the Custom Model.
- overall
Moderation CustomConfiguration Model Overall Moderation Configuration - The overall moderation configuration for the Custom Model.
- positive
Class StringLabel - The positive class label of the Custom Model.
- prediction
Threshold Double - The prediction threshold of the Custom Model.
- resource
Settings CustomModel Resource Settings - The resource settings for the Custom Model.
- runtime
Parameter List<CustomValues Model Runtime Parameter Value> - The runtime parameter values for the Custom Model.
- source
Llm StringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- source
Remote List<CustomRepositories Model Source Remote Repository> - The source remote repositories for the Custom Model.
- target
Name String - The target name of the Custom Model.
- target
Type String - The target type of the Custom Model.
- training
Data StringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- training
Dataset StringId - The ID of the training dataset assigned to the Custom Model.
- base
Environment stringId - The ID of the base environment for the Custom Model.
- base
Environment stringName - The name of the base environment for the Custom Model.
- base
Environment stringVersion Id - The ID of the base environment version for the Custom Model.
- class
Labels string[] - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class
Labels stringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- description string
- The description of the Custom Model.
- files any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- folder
Path string - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- guard
Configurations CustomModel Guard Configuration[] - The guard configurations for the Custom Model.
- is
Proxy boolean - Flag indicating if the Custom Model is a proxy model.
- language string
- The language used to build the Custom Model.
- name string
- The name of the Custom Model.
- negative
Class stringLabel - The negative class label of the Custom Model.
- overall
Moderation CustomConfiguration Model Overall Moderation Configuration - The overall moderation configuration for the Custom Model.
- positive
Class stringLabel - The positive class label of the Custom Model.
- prediction
Threshold number - The prediction threshold of the Custom Model.
- resource
Settings CustomModel Resource Settings - The resource settings for the Custom Model.
- runtime
Parameter CustomValues Model Runtime Parameter Value[] - The runtime parameter values for the Custom Model.
- source
Llm stringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- source
Remote CustomRepositories Model Source Remote Repository[] - The source remote repositories for the Custom Model.
- target
Name string - The target name of the Custom Model.
- target
Type string - The target type of the Custom Model.
- training
Data stringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- training
Dataset stringId - The ID of the training dataset assigned to the Custom Model.
- base_
environment_ strid - The ID of the base environment for the Custom Model.
- base_
environment_ strname - The name of the base environment for the Custom Model.
- base_
environment_ strversion_ id - The ID of the base environment version for the Custom Model.
- class_
labels Sequence[str] - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class_
labels_ strfile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- description str
- The description of the Custom Model.
- files Any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- folder_
path str - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- guard_
configurations Sequence[CustomModel Guard Configuration Args] - The guard configurations for the Custom Model.
- is_
proxy bool - Flag indicating if the Custom Model is a proxy model.
- language str
- The language used to build the Custom Model.
- name str
- The name of the Custom Model.
- negative_
class_ strlabel - The negative class label of the Custom Model.
- overall_
moderation_ Customconfiguration Model Overall Moderation Configuration Args - The overall moderation configuration for the Custom Model.
- positive_
class_ strlabel - The positive class label of the Custom Model.
- prediction_
threshold float - The prediction threshold of the Custom Model.
- resource_
settings CustomModel Resource Settings Args - The resource settings for the Custom Model.
- runtime_
parameter_ Sequence[Customvalues Model Runtime Parameter Value Args] - The runtime parameter values for the Custom Model.
- source_
llm_ strblueprint_ id - The ID of the source LLM Blueprint for the Custom Model.
- source_
remote_ Sequence[Customrepositories Model Source Remote Repository Args] - The source remote repositories for the Custom Model.
- target_
name str - The target name of the Custom Model.
- target_
type str - The target type of the Custom Model.
- training_
data_ strpartition_ column - The name of the partition column in the training dataset assigned to the Custom Model.
- training_
dataset_ strid - The ID of the training dataset assigned to the Custom Model.
- base
Environment StringId - The ID of the base environment for the Custom Model.
- base
Environment StringName - The name of the base environment for the Custom Model.
- base
Environment StringVersion Id - The ID of the base environment version for the Custom Model.
- class
Labels List<String> - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class
Labels StringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- description String
- The description of the Custom Model.
- files Any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- folder
Path String - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- guard
Configurations List<Property Map> - The guard configurations for the Custom Model.
- is
Proxy Boolean - Flag indicating if the Custom Model is a proxy model.
- language String
- The language used to build the Custom Model.
- name String
- The name of the Custom Model.
- negative
Class StringLabel - The negative class label of the Custom Model.
- overall
Moderation Property MapConfiguration - The overall moderation configuration for the Custom Model.
- positive
Class StringLabel - The positive class label of the Custom Model.
- prediction
Threshold Number - The prediction threshold of the Custom Model.
- resource
Settings Property Map - The resource settings for the Custom Model.
- runtime
Parameter List<Property Map>Values - The runtime parameter values for the Custom Model.
- source
Llm StringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- source
Remote List<Property Map>Repositories - The source remote repositories for the Custom Model.
- target
Name String - The target name of the Custom Model.
- target
Type String - The target type of the Custom Model.
- training
Data StringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- training
Dataset StringId - The ID of the training dataset assigned to the Custom Model.
Outputs
All input properties are implicitly available as output properties. Additionally, the CustomModel resource produces the following output properties:
- Deployments
Count int - The number of deployments for the Custom Model.
- Id string
- The provider-assigned unique ID for this managed resource.
- Training
Dataset stringName - The name of the training dataset assigned to the Custom Model.
- Training
Dataset stringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- Version
Id string - The ID of the latest Custom Model version.
- Deployments
Count int - The number of deployments for the Custom Model.
- Id string
- The provider-assigned unique ID for this managed resource.
- Training
Dataset stringName - The name of the training dataset assigned to the Custom Model.
- Training
Dataset stringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- Version
Id string - The ID of the latest Custom Model version.
- deployments
Count Integer - The number of deployments for the Custom Model.
- id String
- The provider-assigned unique ID for this managed resource.
- training
Dataset StringName - The name of the training dataset assigned to the Custom Model.
- training
Dataset StringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- version
Id String - The ID of the latest Custom Model version.
- deployments
Count number - The number of deployments for the Custom Model.
- id string
- The provider-assigned unique ID for this managed resource.
- training
Dataset stringName - The name of the training dataset assigned to the Custom Model.
- training
Dataset stringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- version
Id string - The ID of the latest Custom Model version.
- deployments_
count int - The number of deployments for the Custom Model.
- id str
- The provider-assigned unique ID for this managed resource.
- training_
dataset_ strname - The name of the training dataset assigned to the Custom Model.
- training_
dataset_ strversion_ id - The version ID of the training dataset assigned to the Custom Model.
- version_
id str - The ID of the latest Custom Model version.
- deployments
Count Number - The number of deployments for the Custom Model.
- id String
- The provider-assigned unique ID for this managed resource.
- training
Dataset StringName - The name of the training dataset assigned to the Custom Model.
- training
Dataset StringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- version
Id String - The ID of the latest Custom Model version.
Look up Existing CustomModel Resource
Get an existing CustomModel resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: CustomModelState, opts?: CustomResourceOptions): CustomModel
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
base_environment_id: Optional[str] = None,
base_environment_name: Optional[str] = None,
base_environment_version_id: Optional[str] = None,
class_labels: Optional[Sequence[str]] = None,
class_labels_file: Optional[str] = None,
deployments_count: Optional[int] = None,
description: Optional[str] = None,
files: Optional[Any] = None,
folder_path: Optional[str] = None,
guard_configurations: Optional[Sequence[CustomModelGuardConfigurationArgs]] = None,
is_proxy: Optional[bool] = None,
language: Optional[str] = None,
name: Optional[str] = None,
negative_class_label: Optional[str] = None,
overall_moderation_configuration: Optional[CustomModelOverallModerationConfigurationArgs] = None,
positive_class_label: Optional[str] = None,
prediction_threshold: Optional[float] = None,
resource_settings: Optional[CustomModelResourceSettingsArgs] = None,
runtime_parameter_values: Optional[Sequence[CustomModelRuntimeParameterValueArgs]] = None,
source_llm_blueprint_id: Optional[str] = None,
source_remote_repositories: Optional[Sequence[CustomModelSourceRemoteRepositoryArgs]] = None,
target_name: Optional[str] = None,
target_type: Optional[str] = None,
training_data_partition_column: Optional[str] = None,
training_dataset_id: Optional[str] = None,
training_dataset_name: Optional[str] = None,
training_dataset_version_id: Optional[str] = None,
version_id: Optional[str] = None) -> CustomModel
func GetCustomModel(ctx *Context, name string, id IDInput, state *CustomModelState, opts ...ResourceOption) (*CustomModel, error)
public static CustomModel Get(string name, Input<string> id, CustomModelState? state, CustomResourceOptions? opts = null)
public static CustomModel get(String name, Output<String> id, CustomModelState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Base
Environment stringId - The ID of the base environment for the Custom Model.
- Base
Environment stringName - The name of the base environment for the Custom Model.
- Base
Environment stringVersion Id - The ID of the base environment version for the Custom Model.
- Class
Labels List<string> - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- Class
Labels stringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- Deployments
Count int - The number of deployments for the Custom Model.
- Description string
- The description of the Custom Model.
- Files object
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- Folder
Path string - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- Guard
Configurations List<DataRobot Custom Model Guard Configuration> - The guard configurations for the Custom Model.
- Is
Proxy bool - Flag indicating if the Custom Model is a proxy model.
- Language string
- The language used to build the Custom Model.
- Name string
- The name of the Custom Model.
- Negative
Class stringLabel - The negative class label of the Custom Model.
- Overall
Moderation DataConfiguration Robot Custom Model Overall Moderation Configuration - The overall moderation configuration for the Custom Model.
- Positive
Class stringLabel - The positive class label of the Custom Model.
- Prediction
Threshold double - The prediction threshold of the Custom Model.
- Resource
Settings DataRobot Custom Model Resource Settings - The resource settings for the Custom Model.
- Runtime
Parameter List<DataValues Robot Custom Model Runtime Parameter Value> - The runtime parameter values for the Custom Model.
- Source
Llm stringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- Source
Remote List<DataRepositories Robot Custom Model Source Remote Repository> - The source remote repositories for the Custom Model.
- Target
Name string - The target name of the Custom Model.
- Target
Type string - The target type of the Custom Model.
- Training
Data stringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- Training
Dataset stringId - The ID of the training dataset assigned to the Custom Model.
- Training
Dataset stringName - The name of the training dataset assigned to the Custom Model.
- Training
Dataset stringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- Version
Id string - The ID of the latest Custom Model version.
- Base
Environment stringId - The ID of the base environment for the Custom Model.
- Base
Environment stringName - The name of the base environment for the Custom Model.
- Base
Environment stringVersion Id - The ID of the base environment version for the Custom Model.
- Class
Labels []string - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- Class
Labels stringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- Deployments
Count int - The number of deployments for the Custom Model.
- Description string
- The description of the Custom Model.
- Files interface{}
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- Folder
Path string - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- Guard
Configurations []CustomModel Guard Configuration Args - The guard configurations for the Custom Model.
- Is
Proxy bool - Flag indicating if the Custom Model is a proxy model.
- Language string
- The language used to build the Custom Model.
- Name string
- The name of the Custom Model.
- Negative
Class stringLabel - The negative class label of the Custom Model.
- Overall
Moderation CustomConfiguration Model Overall Moderation Configuration Args - The overall moderation configuration for the Custom Model.
- Positive
Class stringLabel - The positive class label of the Custom Model.
- Prediction
Threshold float64 - The prediction threshold of the Custom Model.
- Resource
Settings CustomModel Resource Settings Args - The resource settings for the Custom Model.
- Runtime
Parameter []CustomValues Model Runtime Parameter Value Args - The runtime parameter values for the Custom Model.
- Source
Llm stringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- Source
Remote []CustomRepositories Model Source Remote Repository Args - The source remote repositories for the Custom Model.
- Target
Name string - The target name of the Custom Model.
- Target
Type string - The target type of the Custom Model.
- Training
Data stringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- Training
Dataset stringId - The ID of the training dataset assigned to the Custom Model.
- Training
Dataset stringName - The name of the training dataset assigned to the Custom Model.
- Training
Dataset stringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- Version
Id string - The ID of the latest Custom Model version.
- base
Environment StringId - The ID of the base environment for the Custom Model.
- base
Environment StringName - The name of the base environment for the Custom Model.
- base
Environment StringVersion Id - The ID of the base environment version for the Custom Model.
- class
Labels List<String> - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class
Labels StringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- deployments
Count Integer - The number of deployments for the Custom Model.
- description String
- The description of the Custom Model.
- files Object
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- folder
Path String - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- guard
Configurations List<CustomModel Guard Configuration> - The guard configurations for the Custom Model.
- is
Proxy Boolean - Flag indicating if the Custom Model is a proxy model.
- language String
- The language used to build the Custom Model.
- name String
- The name of the Custom Model.
- negative
Class StringLabel - The negative class label of the Custom Model.
- overall
Moderation CustomConfiguration Model Overall Moderation Configuration - The overall moderation configuration for the Custom Model.
- positive
Class StringLabel - The positive class label of the Custom Model.
- prediction
Threshold Double - The prediction threshold of the Custom Model.
- resource
Settings CustomModel Resource Settings - The resource settings for the Custom Model.
- runtime
Parameter List<CustomValues Model Runtime Parameter Value> - The runtime parameter values for the Custom Model.
- source
Llm StringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- source
Remote List<CustomRepositories Model Source Remote Repository> - The source remote repositories for the Custom Model.
- target
Name String - The target name of the Custom Model.
- target
Type String - The target type of the Custom Model.
- training
Data StringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- training
Dataset StringId - The ID of the training dataset assigned to the Custom Model.
- training
Dataset StringName - The name of the training dataset assigned to the Custom Model.
- training
Dataset StringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- version
Id String - The ID of the latest Custom Model version.
- base
Environment stringId - The ID of the base environment for the Custom Model.
- base
Environment stringName - The name of the base environment for the Custom Model.
- base
Environment stringVersion Id - The ID of the base environment version for the Custom Model.
- class
Labels string[] - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class
Labels stringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- deployments
Count number - The number of deployments for the Custom Model.
- description string
- The description of the Custom Model.
- files any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- folder
Path string - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- guard
Configurations CustomModel Guard Configuration[] - The guard configurations for the Custom Model.
- is
Proxy boolean - Flag indicating if the Custom Model is a proxy model.
- language string
- The language used to build the Custom Model.
- name string
- The name of the Custom Model.
- negative
Class stringLabel - The negative class label of the Custom Model.
- overall
Moderation CustomConfiguration Model Overall Moderation Configuration - The overall moderation configuration for the Custom Model.
- positive
Class stringLabel - The positive class label of the Custom Model.
- prediction
Threshold number - The prediction threshold of the Custom Model.
- resource
Settings CustomModel Resource Settings - The resource settings for the Custom Model.
- runtime
Parameter CustomValues Model Runtime Parameter Value[] - The runtime parameter values for the Custom Model.
- source
Llm stringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- source
Remote CustomRepositories Model Source Remote Repository[] - The source remote repositories for the Custom Model.
- target
Name string - The target name of the Custom Model.
- target
Type string - The target type of the Custom Model.
- training
Data stringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- training
Dataset stringId - The ID of the training dataset assigned to the Custom Model.
- training
Dataset stringName - The name of the training dataset assigned to the Custom Model.
- training
Dataset stringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- version
Id string - The ID of the latest Custom Model version.
- base_
environment_ strid - The ID of the base environment for the Custom Model.
- base_
environment_ strname - The name of the base environment for the Custom Model.
- base_
environment_ strversion_ id - The ID of the base environment version for the Custom Model.
- class_
labels Sequence[str] - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class_
labels_ strfile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- deployments_
count int - The number of deployments for the Custom Model.
- description str
- The description of the Custom Model.
- files Any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- folder_
path str - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- guard_
configurations Sequence[CustomModel Guard Configuration Args] - The guard configurations for the Custom Model.
- is_
proxy bool - Flag indicating if the Custom Model is a proxy model.
- language str
- The language used to build the Custom Model.
- name str
- The name of the Custom Model.
- negative_
class_ strlabel - The negative class label of the Custom Model.
- overall_
moderation_ Customconfiguration Model Overall Moderation Configuration Args - The overall moderation configuration for the Custom Model.
- positive_
class_ strlabel - The positive class label of the Custom Model.
- prediction_
threshold float - The prediction threshold of the Custom Model.
- resource_
settings CustomModel Resource Settings Args - The resource settings for the Custom Model.
- runtime_
parameter_ Sequence[Customvalues Model Runtime Parameter Value Args] - The runtime parameter values for the Custom Model.
- source_
llm_ strblueprint_ id - The ID of the source LLM Blueprint for the Custom Model.
- source_
remote_ Sequence[Customrepositories Model Source Remote Repository Args] - The source remote repositories for the Custom Model.
- target_
name str - The target name of the Custom Model.
- target_
type str - The target type of the Custom Model.
- training_
data_ strpartition_ column - The name of the partition column in the training dataset assigned to the Custom Model.
- training_
dataset_ strid - The ID of the training dataset assigned to the Custom Model.
- training_
dataset_ strname - The name of the training dataset assigned to the Custom Model.
- training_
dataset_ strversion_ id - The version ID of the training dataset assigned to the Custom Model.
- version_
id str - The ID of the latest Custom Model version.
- base
Environment StringId - The ID of the base environment for the Custom Model.
- base
Environment StringName - The name of the base environment for the Custom Model.
- base
Environment StringVersion Id - The ID of the base environment version for the Custom Model.
- class
Labels List<String> - Class labels for multiclass classification. Cannot be used with classlabelsfile.
- class
Labels StringFile - Path to file containing newline separated class labels for multiclass classification. Cannot be used with class_labels.
- deployments
Count Number - The number of deployments for the Custom Model.
- description String
- The description of the Custom Model.
- files Any
- The list of tuples, where values in each tuple are the local filesystem path and the path the file should be placed in the Custom Model. If list is of strings, then basenames will be used for tuples.
- folder
Path String - The path to a folder containing files to build the Custom Model. Each file in the folder is uploaded under path relative to a folder path.
- guard
Configurations List<Property Map> - The guard configurations for the Custom Model.
- is
Proxy Boolean - Flag indicating if the Custom Model is a proxy model.
- language String
- The language used to build the Custom Model.
- name String
- The name of the Custom Model.
- negative
Class StringLabel - The negative class label of the Custom Model.
- overall
Moderation Property MapConfiguration - The overall moderation configuration for the Custom Model.
- positive
Class StringLabel - The positive class label of the Custom Model.
- prediction
Threshold Number - The prediction threshold of the Custom Model.
- resource
Settings Property Map - The resource settings for the Custom Model.
- runtime
Parameter List<Property Map>Values - The runtime parameter values for the Custom Model.
- source
Llm StringBlueprint Id - The ID of the source LLM Blueprint for the Custom Model.
- source
Remote List<Property Map>Repositories - The source remote repositories for the Custom Model.
- target
Name String - The target name of the Custom Model.
- target
Type String - The target type of the Custom Model.
- training
Data StringPartition Column - The name of the partition column in the training dataset assigned to the Custom Model.
- training
Dataset StringId - The ID of the training dataset assigned to the Custom Model.
- training
Dataset StringName - The name of the training dataset assigned to the Custom Model.
- training
Dataset StringVersion Id - The version ID of the training dataset assigned to the Custom Model.
- version
Id String - The ID of the latest Custom Model version.
Supporting Types
CustomModelGuardConfiguration, CustomModelGuardConfigurationArgs
- Intervention
Data
Robot Custom Model Guard Configuration Intervention - The intervention for the guard configuration.
- Name string
- The name of the guard configuration.
- Stages List<string>
- The list of stages for the guard configuration.
- Template
Name string - The template name of the guard configuration.
- Deployment
Id string - The deployment ID of this guard.
- Input
Column stringName - The input column name of this guard.
- Llm
Type string - The LLM type for this guard.
- Openai
Api stringBase - The OpenAI API base URL for this guard.
- Openai
Credential string - The ID of an OpenAI credential for this guard.
- Openai
Deployment stringId - The ID of an OpenAI deployment for this guard.
- Output
Column stringName - The output column name of this guard.
- Intervention
Custom
Model Guard Configuration Intervention - The intervention for the guard configuration.
- Name string
- The name of the guard configuration.
- Stages []string
- The list of stages for the guard configuration.
- Template
Name string - The template name of the guard configuration.
- Deployment
Id string - The deployment ID of this guard.
- Input
Column stringName - The input column name of this guard.
- Llm
Type string - The LLM type for this guard.
- Openai
Api stringBase - The OpenAI API base URL for this guard.
- Openai
Credential string - The ID of an OpenAI credential for this guard.
- Openai
Deployment stringId - The ID of an OpenAI deployment for this guard.
- Output
Column stringName - The output column name of this guard.
- intervention
Custom
Model Guard Configuration Intervention - The intervention for the guard configuration.
- name String
- The name of the guard configuration.
- stages List<String>
- The list of stages for the guard configuration.
- template
Name String - The template name of the guard configuration.
- deployment
Id String - The deployment ID of this guard.
- input
Column StringName - The input column name of this guard.
- llm
Type String - The LLM type for this guard.
- openai
Api StringBase - The OpenAI API base URL for this guard.
- openai
Credential String - The ID of an OpenAI credential for this guard.
- openai
Deployment StringId - The ID of an OpenAI deployment for this guard.
- output
Column StringName - The output column name of this guard.
- intervention
Custom
Model Guard Configuration Intervention - The intervention for the guard configuration.
- name string
- The name of the guard configuration.
- stages string[]
- The list of stages for the guard configuration.
- template
Name string - The template name of the guard configuration.
- deployment
Id string - The deployment ID of this guard.
- input
Column stringName - The input column name of this guard.
- llm
Type string - The LLM type for this guard.
- openai
Api stringBase - The OpenAI API base URL for this guard.
- openai
Credential string - The ID of an OpenAI credential for this guard.
- openai
Deployment stringId - The ID of an OpenAI deployment for this guard.
- output
Column stringName - The output column name of this guard.
- intervention
Custom
Model Guard Configuration Intervention - The intervention for the guard configuration.
- name str
- The name of the guard configuration.
- stages Sequence[str]
- The list of stages for the guard configuration.
- template_
name str - The template name of the guard configuration.
- deployment_
id str - The deployment ID of this guard.
- input_
column_ strname - The input column name of this guard.
- llm_
type str - The LLM type for this guard.
- openai_
api_ strbase - The OpenAI API base URL for this guard.
- openai_
credential str - The ID of an OpenAI credential for this guard.
- openai_
deployment_ strid - The ID of an OpenAI deployment for this guard.
- output_
column_ strname - The output column name of this guard.
- intervention Property Map
- The intervention for the guard configuration.
- name String
- The name of the guard configuration.
- stages List<String>
- The list of stages for the guard configuration.
- template
Name String - The template name of the guard configuration.
- deployment
Id String - The deployment ID of this guard.
- input
Column StringName - The input column name of this guard.
- llm
Type String - The LLM type for this guard.
- openai
Api StringBase - The OpenAI API base URL for this guard.
- openai
Credential String - The ID of an OpenAI credential for this guard.
- openai
Deployment StringId - The ID of an OpenAI deployment for this guard.
- output
Column StringName - The output column name of this guard.
CustomModelGuardConfigurationIntervention, CustomModelGuardConfigurationInterventionArgs
- Action string
- The action of the guard intervention.
- Condition
Data
Robot Custom Model Guard Configuration Intervention Condition - The list of conditions for the guard intervention.
- Message string
- The message of the guard intervention.
- Action string
- The action of the guard intervention.
- Condition
Custom
Model Guard Configuration Intervention Condition - The list of conditions for the guard intervention.
- Message string
- The message of the guard intervention.
- action String
- The action of the guard intervention.
- condition
Custom
Model Guard Configuration Intervention Condition - The list of conditions for the guard intervention.
- message String
- The message of the guard intervention.
- action string
- The action of the guard intervention.
- condition
Custom
Model Guard Configuration Intervention Condition - The list of conditions for the guard intervention.
- message string
- The message of the guard intervention.
- action str
- The action of the guard intervention.
- condition
Custom
Model Guard Configuration Intervention Condition - The list of conditions for the guard intervention.
- message str
- The message of the guard intervention.
- action String
- The action of the guard intervention.
- condition Property Map
- The list of conditions for the guard intervention.
- message String
- The message of the guard intervention.
CustomModelGuardConfigurationInterventionCondition, CustomModelGuardConfigurationInterventionConditionArgs
- Comparand double
- The comparand of the guard condition.
- Comparator string
- The comparator of the guard condition.
- Comparand float64
- The comparand of the guard condition.
- Comparator string
- The comparator of the guard condition.
- comparand Double
- The comparand of the guard condition.
- comparator String
- The comparator of the guard condition.
- comparand number
- The comparand of the guard condition.
- comparator string
- The comparator of the guard condition.
- comparand float
- The comparand of the guard condition.
- comparator str
- The comparator of the guard condition.
- comparand Number
- The comparand of the guard condition.
- comparator String
- The comparator of the guard condition.
CustomModelOverallModerationConfiguration, CustomModelOverallModerationConfigurationArgs
- Timeout
Action string - The timeout action of the overall moderation configuration.
- Timeout
Sec int - The timeout in seconds of the overall moderation configuration.
- Timeout
Action string - The timeout action of the overall moderation configuration.
- Timeout
Sec int - The timeout in seconds of the overall moderation configuration.
- timeout
Action String - The timeout action of the overall moderation configuration.
- timeout
Sec Integer - The timeout in seconds of the overall moderation configuration.
- timeout
Action string - The timeout action of the overall moderation configuration.
- timeout
Sec number - The timeout in seconds of the overall moderation configuration.
- timeout_
action str - The timeout action of the overall moderation configuration.
- timeout_
sec int - The timeout in seconds of the overall moderation configuration.
- timeout
Action String - The timeout action of the overall moderation configuration.
- timeout
Sec Number - The timeout in seconds of the overall moderation configuration.
CustomModelResourceSettings, CustomModelResourceSettingsArgs
- Memory
Mb int - The memory in MB for the Custom Model.
- Network
Access string - The network access for the Custom Model.
- Replicas int
- The replicas for the Custom Model.
- Memory
Mb int - The memory in MB for the Custom Model.
- Network
Access string - The network access for the Custom Model.
- Replicas int
- The replicas for the Custom Model.
- memory
Mb Integer - The memory in MB for the Custom Model.
- network
Access String - The network access for the Custom Model.
- replicas Integer
- The replicas for the Custom Model.
- memory
Mb number - The memory in MB for the Custom Model.
- network
Access string - The network access for the Custom Model.
- replicas number
- The replicas for the Custom Model.
- memory_
mb int - The memory in MB for the Custom Model.
- network_
access str - The network access for the Custom Model.
- replicas int
- The replicas for the Custom Model.
- memory
Mb Number - The memory in MB for the Custom Model.
- network
Access String - The network access for the Custom Model.
- replicas Number
- The replicas for the Custom Model.
CustomModelRuntimeParameterValue, CustomModelRuntimeParameterValueArgs
CustomModelSourceRemoteRepository, CustomModelSourceRemoteRepositoryArgs
- Id string
- The ID of the source remote repository.
- Ref string
- The reference of the source remote repository.
- Source
Paths List<string> - The list of source paths in the source remote repository.
- Id string
- The ID of the source remote repository.
- Ref string
- The reference of the source remote repository.
- Source
Paths []string - The list of source paths in the source remote repository.
- id String
- The ID of the source remote repository.
- ref String
- The reference of the source remote repository.
- source
Paths List<String> - The list of source paths in the source remote repository.
- id string
- The ID of the source remote repository.
- ref string
- The reference of the source remote repository.
- source
Paths string[] - The list of source paths in the source remote repository.
- id str
- The ID of the source remote repository.
- ref str
- The reference of the source remote repository.
- source_
paths Sequence[str] - The list of source paths in the source remote repository.
- id String
- The ID of the source remote repository.
- ref String
- The reference of the source remote repository.
- source
Paths List<String> - The list of source paths in the source remote repository.
Package Details
- Repository
- datarobot datarobot-community/pulumi-datarobot
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
datarobot
Terraform Provider.
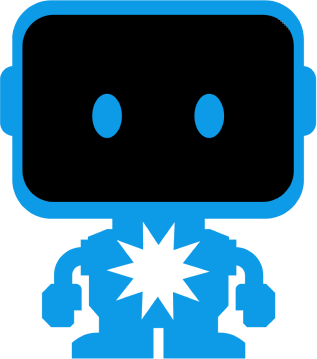
DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.