DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.
datarobot.Deployment
Explore with Pulumi AI
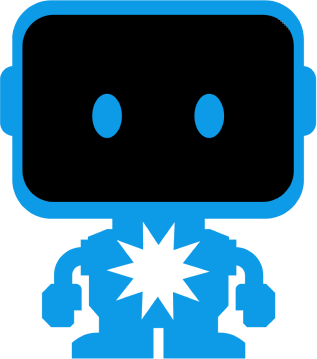
DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.
Deployment
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as datarobot from "@datarobot/pulumi-datarobot";
const exampleCustomModel = new datarobot.CustomModel("exampleCustomModel", {
description: "Description for the example custom model",
targetType: "Binary",
targetName: "my_label",
baseEnvironmentName: "[GenAI] Python 3.11 with Moderations",
files: ["example.py"],
});
const exampleRegisteredModel = new datarobot.RegisteredModel("exampleRegisteredModel", {
customModelVersionId: exampleCustomModel.versionId,
description: "Description for the example registered model",
});
const examplePredictionEnvironment = new datarobot.PredictionEnvironment("examplePredictionEnvironment", {
description: "Description for the example prediction environment",
platform: "datarobotServerless",
});
const exampleDeployment = new datarobot.Deployment("exampleDeployment", {
label: "An example deployment",
predictionEnvironmentId: examplePredictionEnvironment.id,
registeredModelVersionId: exampleRegisteredModel.versionId,
});
// Optional settings
// challenger_models_settings = {}
// challenger_replay_settings = {}
// segment_analysis_settings = {}
// bias_and_fairness_settings = {}
// predictions_by_forecast_date_settings = {}
// drift_tracking_settings = {}
// association_id_settings = {}
// predictions_data_collection_settings = {}
// prediction_warning_settings = {}
// prediction_interval_settings = {}
// predictions_settings = {}
// health_settings = {}
export const datarobotDeploymentId = exampleDeployment.id;
import pulumi
import pulumi_datarobot as datarobot
example_custom_model = datarobot.CustomModel("exampleCustomModel",
description="Description for the example custom model",
target_type="Binary",
target_name="my_label",
base_environment_name="[GenAI] Python 3.11 with Moderations",
files=["example.py"])
example_registered_model = datarobot.RegisteredModel("exampleRegisteredModel",
custom_model_version_id=example_custom_model.version_id,
description="Description for the example registered model")
example_prediction_environment = datarobot.PredictionEnvironment("examplePredictionEnvironment",
description="Description for the example prediction environment",
platform="datarobotServerless")
example_deployment = datarobot.Deployment("exampleDeployment",
label="An example deployment",
prediction_environment_id=example_prediction_environment.id,
registered_model_version_id=example_registered_model.version_id)
# Optional settings
# challenger_models_settings = {}
# challenger_replay_settings = {}
# segment_analysis_settings = {}
# bias_and_fairness_settings = {}
# predictions_by_forecast_date_settings = {}
# drift_tracking_settings = {}
# association_id_settings = {}
# predictions_data_collection_settings = {}
# prediction_warning_settings = {}
# prediction_interval_settings = {}
# predictions_settings = {}
# health_settings = {}
pulumi.export("datarobotDeploymentId", example_deployment.id)
package main
import (
"github.com/datarobot-community/pulumi-datarobot/sdk/go/datarobot"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
exampleCustomModel, err := datarobot.NewCustomModel(ctx, "exampleCustomModel", &datarobot.CustomModelArgs{
Description: pulumi.String("Description for the example custom model"),
TargetType: pulumi.String("Binary"),
TargetName: pulumi.String("my_label"),
BaseEnvironmentName: pulumi.String("[GenAI] Python 3.11 with Moderations"),
Files: pulumi.Any{
"example.py",
},
})
if err != nil {
return err
}
exampleRegisteredModel, err := datarobot.NewRegisteredModel(ctx, "exampleRegisteredModel", &datarobot.RegisteredModelArgs{
CustomModelVersionId: exampleCustomModel.VersionId,
Description: pulumi.String("Description for the example registered model"),
})
if err != nil {
return err
}
examplePredictionEnvironment, err := datarobot.NewPredictionEnvironment(ctx, "examplePredictionEnvironment", &datarobot.PredictionEnvironmentArgs{
Description: pulumi.String("Description for the example prediction environment"),
Platform: pulumi.String("datarobotServerless"),
})
if err != nil {
return err
}
exampleDeployment, err := datarobot.NewDeployment(ctx, "exampleDeployment", &datarobot.DeploymentArgs{
Label: pulumi.String("An example deployment"),
PredictionEnvironmentId: examplePredictionEnvironment.ID(),
RegisteredModelVersionId: exampleRegisteredModel.VersionId,
})
if err != nil {
return err
}
ctx.Export("datarobotDeploymentId", exampleDeployment.ID())
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Datarobot = DataRobotPulumi.Datarobot;
return await Deployment.RunAsync(() =>
{
var exampleCustomModel = new Datarobot.CustomModel("exampleCustomModel", new()
{
Description = "Description for the example custom model",
TargetType = "Binary",
TargetName = "my_label",
BaseEnvironmentName = "[GenAI] Python 3.11 with Moderations",
Files = new[]
{
"example.py",
},
});
var exampleRegisteredModel = new Datarobot.RegisteredModel("exampleRegisteredModel", new()
{
CustomModelVersionId = exampleCustomModel.VersionId,
Description = "Description for the example registered model",
});
var examplePredictionEnvironment = new Datarobot.PredictionEnvironment("examplePredictionEnvironment", new()
{
Description = "Description for the example prediction environment",
Platform = "datarobotServerless",
});
var exampleDeployment = new Datarobot.Deployment("exampleDeployment", new()
{
Label = "An example deployment",
PredictionEnvironmentId = examplePredictionEnvironment.Id,
RegisteredModelVersionId = exampleRegisteredModel.VersionId,
});
// Optional settings
// challenger_models_settings = {}
// challenger_replay_settings = {}
// segment_analysis_settings = {}
// bias_and_fairness_settings = {}
// predictions_by_forecast_date_settings = {}
// drift_tracking_settings = {}
// association_id_settings = {}
// predictions_data_collection_settings = {}
// prediction_warning_settings = {}
// prediction_interval_settings = {}
// predictions_settings = {}
// health_settings = {}
return new Dictionary<string, object?>
{
["datarobotDeploymentId"] = exampleDeployment.Id,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.datarobot.CustomModel;
import com.pulumi.datarobot.CustomModelArgs;
import com.pulumi.datarobot.RegisteredModel;
import com.pulumi.datarobot.RegisteredModelArgs;
import com.pulumi.datarobot.PredictionEnvironment;
import com.pulumi.datarobot.PredictionEnvironmentArgs;
import com.pulumi.datarobot.Deployment;
import com.pulumi.datarobot.DeploymentArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var exampleCustomModel = new CustomModel("exampleCustomModel", CustomModelArgs.builder()
.description("Description for the example custom model")
.targetType("Binary")
.targetName("my_label")
.baseEnvironmentName("[GenAI] Python 3.11 with Moderations")
.files("example.py")
.build());
var exampleRegisteredModel = new RegisteredModel("exampleRegisteredModel", RegisteredModelArgs.builder()
.customModelVersionId(exampleCustomModel.versionId())
.description("Description for the example registered model")
.build());
var examplePredictionEnvironment = new PredictionEnvironment("examplePredictionEnvironment", PredictionEnvironmentArgs.builder()
.description("Description for the example prediction environment")
.platform("datarobotServerless")
.build());
var exampleDeployment = new Deployment("exampleDeployment", DeploymentArgs.builder()
.label("An example deployment")
.predictionEnvironmentId(examplePredictionEnvironment.id())
.registeredModelVersionId(exampleRegisteredModel.versionId())
.build());
// Optional settings
// challenger_models_settings = {}
// challenger_replay_settings = {}
// segment_analysis_settings = {}
// bias_and_fairness_settings = {}
// predictions_by_forecast_date_settings = {}
// drift_tracking_settings = {}
// association_id_settings = {}
// predictions_data_collection_settings = {}
// prediction_warning_settings = {}
// prediction_interval_settings = {}
// predictions_settings = {}
// health_settings = {}
ctx.export("datarobotDeploymentId", exampleDeployment.id());
}
}
resources:
exampleCustomModel:
type: datarobot:CustomModel
properties:
description: Description for the example custom model
targetType: Binary
targetName: my_label
baseEnvironmentName: '[GenAI] Python 3.11 with Moderations'
files:
- example.py
exampleRegisteredModel:
type: datarobot:RegisteredModel
properties:
customModelVersionId: ${exampleCustomModel.versionId}
description: Description for the example registered model
examplePredictionEnvironment:
type: datarobot:PredictionEnvironment
properties:
description: Description for the example prediction environment
platform: datarobotServerless
exampleDeployment:
type: datarobot:Deployment
properties:
label: An example deployment
predictionEnvironmentId: ${examplePredictionEnvironment.id}
registeredModelVersionId: ${exampleRegisteredModel.versionId}
outputs:
datarobotDeploymentId: ${exampleDeployment.id}
Create Deployment Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new Deployment(name: string, args: DeploymentArgs, opts?: CustomResourceOptions);
@overload
def Deployment(resource_name: str,
args: DeploymentArgs,
opts: Optional[ResourceOptions] = None)
@overload
def Deployment(resource_name: str,
opts: Optional[ResourceOptions] = None,
label: Optional[str] = None,
registered_model_version_id: Optional[str] = None,
prediction_environment_id: Optional[str] = None,
drift_tracking_settings: Optional[DeploymentDriftTrackingSettingsArgs] = None,
association_id_settings: Optional[DeploymentAssociationIdSettingsArgs] = None,
health_settings: Optional[DeploymentHealthSettingsArgs] = None,
importance: Optional[str] = None,
challenger_replay_settings: Optional[DeploymentChallengerReplaySettingsArgs] = None,
challenger_models_settings: Optional[DeploymentChallengerModelsSettingsArgs] = None,
prediction_intervals_settings: Optional[DeploymentPredictionIntervalsSettingsArgs] = None,
prediction_warning_settings: Optional[DeploymentPredictionWarningSettingsArgs] = None,
predictions_by_forecast_date_settings: Optional[DeploymentPredictionsByForecastDateSettingsArgs] = None,
predictions_data_collection_settings: Optional[DeploymentPredictionsDataCollectionSettingsArgs] = None,
predictions_settings: Optional[DeploymentPredictionsSettingsArgs] = None,
bias_and_fairness_settings: Optional[DeploymentBiasAndFairnessSettingsArgs] = None,
segment_analysis_settings: Optional[DeploymentSegmentAnalysisSettingsArgs] = None)
func NewDeployment(ctx *Context, name string, args DeploymentArgs, opts ...ResourceOption) (*Deployment, error)
public Deployment(string name, DeploymentArgs args, CustomResourceOptions? opts = null)
public Deployment(String name, DeploymentArgs args)
public Deployment(String name, DeploymentArgs args, CustomResourceOptions options)
type: datarobot:Deployment
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args DeploymentArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args DeploymentArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args DeploymentArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args DeploymentArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args DeploymentArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var deploymentResource = new Datarobot.Deployment("deploymentResource", new()
{
Label = "string",
RegisteredModelVersionId = "string",
PredictionEnvironmentId = "string",
DriftTrackingSettings = new Datarobot.Inputs.DeploymentDriftTrackingSettingsArgs
{
FeatureDriftEnabled = false,
TargetDriftEnabled = false,
},
AssociationIdSettings = new Datarobot.Inputs.DeploymentAssociationIdSettingsArgs
{
AutoGenerateId = false,
ColumnNames = new[]
{
"string",
},
RequiredInPredictionRequests = false,
},
HealthSettings = new Datarobot.Inputs.DeploymentHealthSettingsArgs
{
Accuracy = new Datarobot.Inputs.DeploymentHealthSettingsAccuracyArgs
{
BatchCount = 0,
FailingThreshold = 0,
Measurement = "string",
Metric = "string",
WarningThreshold = 0,
},
ActualsTimeliness = new Datarobot.Inputs.DeploymentHealthSettingsActualsTimelinessArgs
{
Enabled = false,
ExpectedFrequency = "string",
},
CustomMetrics = new Datarobot.Inputs.DeploymentHealthSettingsCustomMetricsArgs
{
FailingConditions = new[]
{
new Datarobot.Inputs.DeploymentHealthSettingsCustomMetricsFailingConditionArgs
{
CompareOperator = "string",
MetricId = "string",
Threshold = 0,
},
},
WarningConditions = new[]
{
new Datarobot.Inputs.DeploymentHealthSettingsCustomMetricsWarningConditionArgs
{
CompareOperator = "string",
MetricId = "string",
Threshold = 0,
},
},
},
DataDrift = new Datarobot.Inputs.DeploymentHealthSettingsDataDriftArgs
{
BatchCount = 0,
DriftThreshold = 0,
ExcludeFeatures = new[]
{
"string",
},
HighImportanceFailingCount = 0,
HighImportanceWarningCount = 0,
ImportanceThreshold = 0,
LowImportanceFailingCount = 0,
LowImportanceWarningCount = 0,
StarredFeatures = new[]
{
"string",
},
TimeInterval = "string",
},
Fairness = new Datarobot.Inputs.DeploymentHealthSettingsFairnessArgs
{
ProtectedClassFailingCount = 0,
ProtectedClassWarningCount = 0,
},
PredictionsTimeliness = new Datarobot.Inputs.DeploymentHealthSettingsPredictionsTimelinessArgs
{
Enabled = false,
ExpectedFrequency = "string",
},
Service = new Datarobot.Inputs.DeploymentHealthSettingsServiceArgs
{
BatchCount = 0,
},
},
Importance = "string",
ChallengerReplaySettings = new Datarobot.Inputs.DeploymentChallengerReplaySettingsArgs
{
Enabled = false,
},
ChallengerModelsSettings = new Datarobot.Inputs.DeploymentChallengerModelsSettingsArgs
{
Enabled = false,
},
PredictionIntervalsSettings = new Datarobot.Inputs.DeploymentPredictionIntervalsSettingsArgs
{
Enabled = false,
Percentiles = new[]
{
0,
},
},
PredictionWarningSettings = new Datarobot.Inputs.DeploymentPredictionWarningSettingsArgs
{
Enabled = false,
CustomBoundaries = new Datarobot.Inputs.DeploymentPredictionWarningSettingsCustomBoundariesArgs
{
LowerBoundary = 0,
UpperBoundary = 0,
},
},
PredictionsByForecastDateSettings = new Datarobot.Inputs.DeploymentPredictionsByForecastDateSettingsArgs
{
Enabled = false,
ColumnName = "string",
DatetimeFormat = "string",
},
PredictionsDataCollectionSettings = new Datarobot.Inputs.DeploymentPredictionsDataCollectionSettingsArgs
{
Enabled = false,
},
PredictionsSettings = new Datarobot.Inputs.DeploymentPredictionsSettingsArgs
{
MaxComputes = 0,
MinComputes = 0,
RealTime = false,
},
BiasAndFairnessSettings = new Datarobot.Inputs.DeploymentBiasAndFairnessSettingsArgs
{
FairnessMetricSet = "string",
FairnessThreshold = 0,
PreferableTargetValue = false,
ProtectedFeatures = new[]
{
"string",
},
},
SegmentAnalysisSettings = new Datarobot.Inputs.DeploymentSegmentAnalysisSettingsArgs
{
Enabled = false,
Attributes = new[]
{
"string",
},
},
});
example, err := datarobot.NewDeployment(ctx, "deploymentResource", &datarobot.DeploymentArgs{
Label: pulumi.String("string"),
RegisteredModelVersionId: pulumi.String("string"),
PredictionEnvironmentId: pulumi.String("string"),
DriftTrackingSettings: &datarobot.DeploymentDriftTrackingSettingsArgs{
FeatureDriftEnabled: pulumi.Bool(false),
TargetDriftEnabled: pulumi.Bool(false),
},
AssociationIdSettings: &datarobot.DeploymentAssociationIdSettingsArgs{
AutoGenerateId: pulumi.Bool(false),
ColumnNames: pulumi.StringArray{
pulumi.String("string"),
},
RequiredInPredictionRequests: pulumi.Bool(false),
},
HealthSettings: &datarobot.DeploymentHealthSettingsArgs{
Accuracy: &datarobot.DeploymentHealthSettingsAccuracyArgs{
BatchCount: pulumi.Int(0),
FailingThreshold: pulumi.Float64(0),
Measurement: pulumi.String("string"),
Metric: pulumi.String("string"),
WarningThreshold: pulumi.Float64(0),
},
ActualsTimeliness: &datarobot.DeploymentHealthSettingsActualsTimelinessArgs{
Enabled: pulumi.Bool(false),
ExpectedFrequency: pulumi.String("string"),
},
CustomMetrics: &datarobot.DeploymentHealthSettingsCustomMetricsArgs{
FailingConditions: datarobot.DeploymentHealthSettingsCustomMetricsFailingConditionArray{
&datarobot.DeploymentHealthSettingsCustomMetricsFailingConditionArgs{
CompareOperator: pulumi.String("string"),
MetricId: pulumi.String("string"),
Threshold: pulumi.Float64(0),
},
},
WarningConditions: datarobot.DeploymentHealthSettingsCustomMetricsWarningConditionArray{
&datarobot.DeploymentHealthSettingsCustomMetricsWarningConditionArgs{
CompareOperator: pulumi.String("string"),
MetricId: pulumi.String("string"),
Threshold: pulumi.Float64(0),
},
},
},
DataDrift: &datarobot.DeploymentHealthSettingsDataDriftArgs{
BatchCount: pulumi.Int(0),
DriftThreshold: pulumi.Float64(0),
ExcludeFeatures: pulumi.StringArray{
pulumi.String("string"),
},
HighImportanceFailingCount: pulumi.Int(0),
HighImportanceWarningCount: pulumi.Int(0),
ImportanceThreshold: pulumi.Float64(0),
LowImportanceFailingCount: pulumi.Int(0),
LowImportanceWarningCount: pulumi.Int(0),
StarredFeatures: pulumi.StringArray{
pulumi.String("string"),
},
TimeInterval: pulumi.String("string"),
},
Fairness: &datarobot.DeploymentHealthSettingsFairnessArgs{
ProtectedClassFailingCount: pulumi.Int(0),
ProtectedClassWarningCount: pulumi.Int(0),
},
PredictionsTimeliness: &datarobot.DeploymentHealthSettingsPredictionsTimelinessArgs{
Enabled: pulumi.Bool(false),
ExpectedFrequency: pulumi.String("string"),
},
Service: &datarobot.DeploymentHealthSettingsServiceArgs{
BatchCount: pulumi.Int(0),
},
},
Importance: pulumi.String("string"),
ChallengerReplaySettings: &datarobot.DeploymentChallengerReplaySettingsArgs{
Enabled: pulumi.Bool(false),
},
ChallengerModelsSettings: &datarobot.DeploymentChallengerModelsSettingsArgs{
Enabled: pulumi.Bool(false),
},
PredictionIntervalsSettings: &datarobot.DeploymentPredictionIntervalsSettingsArgs{
Enabled: pulumi.Bool(false),
Percentiles: pulumi.IntArray{
pulumi.Int(0),
},
},
PredictionWarningSettings: &datarobot.DeploymentPredictionWarningSettingsArgs{
Enabled: pulumi.Bool(false),
CustomBoundaries: &datarobot.DeploymentPredictionWarningSettingsCustomBoundariesArgs{
LowerBoundary: pulumi.Float64(0),
UpperBoundary: pulumi.Float64(0),
},
},
PredictionsByForecastDateSettings: &datarobot.DeploymentPredictionsByForecastDateSettingsArgs{
Enabled: pulumi.Bool(false),
ColumnName: pulumi.String("string"),
DatetimeFormat: pulumi.String("string"),
},
PredictionsDataCollectionSettings: &datarobot.DeploymentPredictionsDataCollectionSettingsArgs{
Enabled: pulumi.Bool(false),
},
PredictionsSettings: &datarobot.DeploymentPredictionsSettingsArgs{
MaxComputes: pulumi.Int(0),
MinComputes: pulumi.Int(0),
RealTime: pulumi.Bool(false),
},
BiasAndFairnessSettings: &datarobot.DeploymentBiasAndFairnessSettingsArgs{
FairnessMetricSet: pulumi.String("string"),
FairnessThreshold: pulumi.Float64(0),
PreferableTargetValue: pulumi.Bool(false),
ProtectedFeatures: pulumi.StringArray{
pulumi.String("string"),
},
},
SegmentAnalysisSettings: &datarobot.DeploymentSegmentAnalysisSettingsArgs{
Enabled: pulumi.Bool(false),
Attributes: pulumi.StringArray{
pulumi.String("string"),
},
},
})
var deploymentResource = new Deployment("deploymentResource", DeploymentArgs.builder()
.label("string")
.registeredModelVersionId("string")
.predictionEnvironmentId("string")
.driftTrackingSettings(DeploymentDriftTrackingSettingsArgs.builder()
.featureDriftEnabled(false)
.targetDriftEnabled(false)
.build())
.associationIdSettings(DeploymentAssociationIdSettingsArgs.builder()
.autoGenerateId(false)
.columnNames("string")
.requiredInPredictionRequests(false)
.build())
.healthSettings(DeploymentHealthSettingsArgs.builder()
.accuracy(DeploymentHealthSettingsAccuracyArgs.builder()
.batchCount(0)
.failingThreshold(0)
.measurement("string")
.metric("string")
.warningThreshold(0)
.build())
.actualsTimeliness(DeploymentHealthSettingsActualsTimelinessArgs.builder()
.enabled(false)
.expectedFrequency("string")
.build())
.customMetrics(DeploymentHealthSettingsCustomMetricsArgs.builder()
.failingConditions(DeploymentHealthSettingsCustomMetricsFailingConditionArgs.builder()
.compareOperator("string")
.metricId("string")
.threshold(0)
.build())
.warningConditions(DeploymentHealthSettingsCustomMetricsWarningConditionArgs.builder()
.compareOperator("string")
.metricId("string")
.threshold(0)
.build())
.build())
.dataDrift(DeploymentHealthSettingsDataDriftArgs.builder()
.batchCount(0)
.driftThreshold(0)
.excludeFeatures("string")
.highImportanceFailingCount(0)
.highImportanceWarningCount(0)
.importanceThreshold(0)
.lowImportanceFailingCount(0)
.lowImportanceWarningCount(0)
.starredFeatures("string")
.timeInterval("string")
.build())
.fairness(DeploymentHealthSettingsFairnessArgs.builder()
.protectedClassFailingCount(0)
.protectedClassWarningCount(0)
.build())
.predictionsTimeliness(DeploymentHealthSettingsPredictionsTimelinessArgs.builder()
.enabled(false)
.expectedFrequency("string")
.build())
.service(DeploymentHealthSettingsServiceArgs.builder()
.batchCount(0)
.build())
.build())
.importance("string")
.challengerReplaySettings(DeploymentChallengerReplaySettingsArgs.builder()
.enabled(false)
.build())
.challengerModelsSettings(DeploymentChallengerModelsSettingsArgs.builder()
.enabled(false)
.build())
.predictionIntervalsSettings(DeploymentPredictionIntervalsSettingsArgs.builder()
.enabled(false)
.percentiles(0)
.build())
.predictionWarningSettings(DeploymentPredictionWarningSettingsArgs.builder()
.enabled(false)
.customBoundaries(DeploymentPredictionWarningSettingsCustomBoundariesArgs.builder()
.lowerBoundary(0)
.upperBoundary(0)
.build())
.build())
.predictionsByForecastDateSettings(DeploymentPredictionsByForecastDateSettingsArgs.builder()
.enabled(false)
.columnName("string")
.datetimeFormat("string")
.build())
.predictionsDataCollectionSettings(DeploymentPredictionsDataCollectionSettingsArgs.builder()
.enabled(false)
.build())
.predictionsSettings(DeploymentPredictionsSettingsArgs.builder()
.maxComputes(0)
.minComputes(0)
.realTime(false)
.build())
.biasAndFairnessSettings(DeploymentBiasAndFairnessSettingsArgs.builder()
.fairnessMetricSet("string")
.fairnessThreshold(0)
.preferableTargetValue(false)
.protectedFeatures("string")
.build())
.segmentAnalysisSettings(DeploymentSegmentAnalysisSettingsArgs.builder()
.enabled(false)
.attributes("string")
.build())
.build());
deployment_resource = datarobot.Deployment("deploymentResource",
label="string",
registered_model_version_id="string",
prediction_environment_id="string",
drift_tracking_settings=datarobot.DeploymentDriftTrackingSettingsArgs(
feature_drift_enabled=False,
target_drift_enabled=False,
),
association_id_settings=datarobot.DeploymentAssociationIdSettingsArgs(
auto_generate_id=False,
column_names=["string"],
required_in_prediction_requests=False,
),
health_settings=datarobot.DeploymentHealthSettingsArgs(
accuracy=datarobot.DeploymentHealthSettingsAccuracyArgs(
batch_count=0,
failing_threshold=0,
measurement="string",
metric="string",
warning_threshold=0,
),
actuals_timeliness=datarobot.DeploymentHealthSettingsActualsTimelinessArgs(
enabled=False,
expected_frequency="string",
),
custom_metrics=datarobot.DeploymentHealthSettingsCustomMetricsArgs(
failing_conditions=[datarobot.DeploymentHealthSettingsCustomMetricsFailingConditionArgs(
compare_operator="string",
metric_id="string",
threshold=0,
)],
warning_conditions=[datarobot.DeploymentHealthSettingsCustomMetricsWarningConditionArgs(
compare_operator="string",
metric_id="string",
threshold=0,
)],
),
data_drift=datarobot.DeploymentHealthSettingsDataDriftArgs(
batch_count=0,
drift_threshold=0,
exclude_features=["string"],
high_importance_failing_count=0,
high_importance_warning_count=0,
importance_threshold=0,
low_importance_failing_count=0,
low_importance_warning_count=0,
starred_features=["string"],
time_interval="string",
),
fairness=datarobot.DeploymentHealthSettingsFairnessArgs(
protected_class_failing_count=0,
protected_class_warning_count=0,
),
predictions_timeliness=datarobot.DeploymentHealthSettingsPredictionsTimelinessArgs(
enabled=False,
expected_frequency="string",
),
service=datarobot.DeploymentHealthSettingsServiceArgs(
batch_count=0,
),
),
importance="string",
challenger_replay_settings=datarobot.DeploymentChallengerReplaySettingsArgs(
enabled=False,
),
challenger_models_settings=datarobot.DeploymentChallengerModelsSettingsArgs(
enabled=False,
),
prediction_intervals_settings=datarobot.DeploymentPredictionIntervalsSettingsArgs(
enabled=False,
percentiles=[0],
),
prediction_warning_settings=datarobot.DeploymentPredictionWarningSettingsArgs(
enabled=False,
custom_boundaries=datarobot.DeploymentPredictionWarningSettingsCustomBoundariesArgs(
lower_boundary=0,
upper_boundary=0,
),
),
predictions_by_forecast_date_settings=datarobot.DeploymentPredictionsByForecastDateSettingsArgs(
enabled=False,
column_name="string",
datetime_format="string",
),
predictions_data_collection_settings=datarobot.DeploymentPredictionsDataCollectionSettingsArgs(
enabled=False,
),
predictions_settings=datarobot.DeploymentPredictionsSettingsArgs(
max_computes=0,
min_computes=0,
real_time=False,
),
bias_and_fairness_settings=datarobot.DeploymentBiasAndFairnessSettingsArgs(
fairness_metric_set="string",
fairness_threshold=0,
preferable_target_value=False,
protected_features=["string"],
),
segment_analysis_settings=datarobot.DeploymentSegmentAnalysisSettingsArgs(
enabled=False,
attributes=["string"],
))
const deploymentResource = new datarobot.Deployment("deploymentResource", {
label: "string",
registeredModelVersionId: "string",
predictionEnvironmentId: "string",
driftTrackingSettings: {
featureDriftEnabled: false,
targetDriftEnabled: false,
},
associationIdSettings: {
autoGenerateId: false,
columnNames: ["string"],
requiredInPredictionRequests: false,
},
healthSettings: {
accuracy: {
batchCount: 0,
failingThreshold: 0,
measurement: "string",
metric: "string",
warningThreshold: 0,
},
actualsTimeliness: {
enabled: false,
expectedFrequency: "string",
},
customMetrics: {
failingConditions: [{
compareOperator: "string",
metricId: "string",
threshold: 0,
}],
warningConditions: [{
compareOperator: "string",
metricId: "string",
threshold: 0,
}],
},
dataDrift: {
batchCount: 0,
driftThreshold: 0,
excludeFeatures: ["string"],
highImportanceFailingCount: 0,
highImportanceWarningCount: 0,
importanceThreshold: 0,
lowImportanceFailingCount: 0,
lowImportanceWarningCount: 0,
starredFeatures: ["string"],
timeInterval: "string",
},
fairness: {
protectedClassFailingCount: 0,
protectedClassWarningCount: 0,
},
predictionsTimeliness: {
enabled: false,
expectedFrequency: "string",
},
service: {
batchCount: 0,
},
},
importance: "string",
challengerReplaySettings: {
enabled: false,
},
challengerModelsSettings: {
enabled: false,
},
predictionIntervalsSettings: {
enabled: false,
percentiles: [0],
},
predictionWarningSettings: {
enabled: false,
customBoundaries: {
lowerBoundary: 0,
upperBoundary: 0,
},
},
predictionsByForecastDateSettings: {
enabled: false,
columnName: "string",
datetimeFormat: "string",
},
predictionsDataCollectionSettings: {
enabled: false,
},
predictionsSettings: {
maxComputes: 0,
minComputes: 0,
realTime: false,
},
biasAndFairnessSettings: {
fairnessMetricSet: "string",
fairnessThreshold: 0,
preferableTargetValue: false,
protectedFeatures: ["string"],
},
segmentAnalysisSettings: {
enabled: false,
attributes: ["string"],
},
});
type: datarobot:Deployment
properties:
associationIdSettings:
autoGenerateId: false
columnNames:
- string
requiredInPredictionRequests: false
biasAndFairnessSettings:
fairnessMetricSet: string
fairnessThreshold: 0
preferableTargetValue: false
protectedFeatures:
- string
challengerModelsSettings:
enabled: false
challengerReplaySettings:
enabled: false
driftTrackingSettings:
featureDriftEnabled: false
targetDriftEnabled: false
healthSettings:
accuracy:
batchCount: 0
failingThreshold: 0
measurement: string
metric: string
warningThreshold: 0
actualsTimeliness:
enabled: false
expectedFrequency: string
customMetrics:
failingConditions:
- compareOperator: string
metricId: string
threshold: 0
warningConditions:
- compareOperator: string
metricId: string
threshold: 0
dataDrift:
batchCount: 0
driftThreshold: 0
excludeFeatures:
- string
highImportanceFailingCount: 0
highImportanceWarningCount: 0
importanceThreshold: 0
lowImportanceFailingCount: 0
lowImportanceWarningCount: 0
starredFeatures:
- string
timeInterval: string
fairness:
protectedClassFailingCount: 0
protectedClassWarningCount: 0
predictionsTimeliness:
enabled: false
expectedFrequency: string
service:
batchCount: 0
importance: string
label: string
predictionEnvironmentId: string
predictionIntervalsSettings:
enabled: false
percentiles:
- 0
predictionWarningSettings:
customBoundaries:
lowerBoundary: 0
upperBoundary: 0
enabled: false
predictionsByForecastDateSettings:
columnName: string
datetimeFormat: string
enabled: false
predictionsDataCollectionSettings:
enabled: false
predictionsSettings:
maxComputes: 0
minComputes: 0
realTime: false
registeredModelVersionId: string
segmentAnalysisSettings:
attributes:
- string
enabled: false
Deployment Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The Deployment resource accepts the following input properties:
- Label string
- The label of the Deployment.
- Prediction
Environment stringId - The ID of the predication environment for this Deployment.
- Registered
Model stringVersion Id - The ID of the registered model version for this Deployment.
- Association
Id DataSettings Robot Deployment Association Id Settings - Association ID settings for this Deployment.
- Bias
And DataFairness Settings Robot Deployment Bias And Fairness Settings - Bias and fairness settings for the Deployment.
- Challenger
Models DataSettings Robot Deployment Challenger Models Settings - The challenger models settings for the Deployment.
- Challenger
Replay DataSettings Robot Deployment Challenger Replay Settings - The challenger replay settings for the Deployment.
- Drift
Tracking DataSettings Robot Deployment Drift Tracking Settings - The drift tracking settings for the Deployment.
- Health
Settings DataRobot Deployment Health Settings - The health settings for this Deployment.
- Importance string
- The importance of the Deployment.
- Prediction
Intervals DataSettings Robot Deployment Prediction Intervals Settings - The prediction intervals settings for this Deployment.
- Prediction
Warning DataSettings Robot Deployment Prediction Warning Settings - The prediction warning settings for the Deployment.
- Predictions
By DataForecast Date Settings Robot Deployment Predictions By Forecast Date Settings - The predictions by forecase date settings for the Deployment.
- Predictions
Data DataCollection Settings Robot Deployment Predictions Data Collection Settings - The predictions data collection settings for the Deployment.
- Predictions
Settings DataRobot Deployment Predictions Settings - Settings for the predictions.
- Segment
Analysis DataSettings Robot Deployment Segment Analysis Settings - The segment analysis settings for the Deployment.
- Label string
- The label of the Deployment.
- Prediction
Environment stringId - The ID of the predication environment for this Deployment.
- Registered
Model stringVersion Id - The ID of the registered model version for this Deployment.
- Association
Id DeploymentSettings Association Id Settings Args - Association ID settings for this Deployment.
- Bias
And DeploymentFairness Settings Bias And Fairness Settings Args - Bias and fairness settings for the Deployment.
- Challenger
Models DeploymentSettings Challenger Models Settings Args - The challenger models settings for the Deployment.
- Challenger
Replay DeploymentSettings Challenger Replay Settings Args - The challenger replay settings for the Deployment.
- Drift
Tracking DeploymentSettings Drift Tracking Settings Args - The drift tracking settings for the Deployment.
- Health
Settings DeploymentHealth Settings Args - The health settings for this Deployment.
- Importance string
- The importance of the Deployment.
- Prediction
Intervals DeploymentSettings Prediction Intervals Settings Args - The prediction intervals settings for this Deployment.
- Prediction
Warning DeploymentSettings Prediction Warning Settings Args - The prediction warning settings for the Deployment.
- Predictions
By DeploymentForecast Date Settings Predictions By Forecast Date Settings Args - The predictions by forecase date settings for the Deployment.
- Predictions
Data DeploymentCollection Settings Predictions Data Collection Settings Args - The predictions data collection settings for the Deployment.
- Predictions
Settings DeploymentPredictions Settings Args - Settings for the predictions.
- Segment
Analysis DeploymentSettings Segment Analysis Settings Args - The segment analysis settings for the Deployment.
- label String
- The label of the Deployment.
- prediction
Environment StringId - The ID of the predication environment for this Deployment.
- registered
Model StringVersion Id - The ID of the registered model version for this Deployment.
- association
Id DeploymentSettings Association Id Settings - Association ID settings for this Deployment.
- bias
And DeploymentFairness Settings Bias And Fairness Settings - Bias and fairness settings for the Deployment.
- challenger
Models DeploymentSettings Challenger Models Settings - The challenger models settings for the Deployment.
- challenger
Replay DeploymentSettings Challenger Replay Settings - The challenger replay settings for the Deployment.
- drift
Tracking DeploymentSettings Drift Tracking Settings - The drift tracking settings for the Deployment.
- health
Settings DeploymentHealth Settings - The health settings for this Deployment.
- importance String
- The importance of the Deployment.
- prediction
Intervals DeploymentSettings Prediction Intervals Settings - The prediction intervals settings for this Deployment.
- prediction
Warning DeploymentSettings Prediction Warning Settings - The prediction warning settings for the Deployment.
- predictions
By DeploymentForecast Date Settings Predictions By Forecast Date Settings - The predictions by forecase date settings for the Deployment.
- predictions
Data DeploymentCollection Settings Predictions Data Collection Settings - The predictions data collection settings for the Deployment.
- predictions
Settings DeploymentPredictions Settings - Settings for the predictions.
- segment
Analysis DeploymentSettings Segment Analysis Settings - The segment analysis settings for the Deployment.
- label string
- The label of the Deployment.
- prediction
Environment stringId - The ID of the predication environment for this Deployment.
- registered
Model stringVersion Id - The ID of the registered model version for this Deployment.
- association
Id DeploymentSettings Association Id Settings - Association ID settings for this Deployment.
- bias
And DeploymentFairness Settings Bias And Fairness Settings - Bias and fairness settings for the Deployment.
- challenger
Models DeploymentSettings Challenger Models Settings - The challenger models settings for the Deployment.
- challenger
Replay DeploymentSettings Challenger Replay Settings - The challenger replay settings for the Deployment.
- drift
Tracking DeploymentSettings Drift Tracking Settings - The drift tracking settings for the Deployment.
- health
Settings DeploymentHealth Settings - The health settings for this Deployment.
- importance string
- The importance of the Deployment.
- prediction
Intervals DeploymentSettings Prediction Intervals Settings - The prediction intervals settings for this Deployment.
- prediction
Warning DeploymentSettings Prediction Warning Settings - The prediction warning settings for the Deployment.
- predictions
By DeploymentForecast Date Settings Predictions By Forecast Date Settings - The predictions by forecase date settings for the Deployment.
- predictions
Data DeploymentCollection Settings Predictions Data Collection Settings - The predictions data collection settings for the Deployment.
- predictions
Settings DeploymentPredictions Settings - Settings for the predictions.
- segment
Analysis DeploymentSettings Segment Analysis Settings - The segment analysis settings for the Deployment.
- label str
- The label of the Deployment.
- prediction_
environment_ strid - The ID of the predication environment for this Deployment.
- registered_
model_ strversion_ id - The ID of the registered model version for this Deployment.
- association_
id_ Deploymentsettings Association Id Settings Args - Association ID settings for this Deployment.
- bias_
and_ Deploymentfairness_ settings Bias And Fairness Settings Args - Bias and fairness settings for the Deployment.
- challenger_
models_ Deploymentsettings Challenger Models Settings Args - The challenger models settings for the Deployment.
- challenger_
replay_ Deploymentsettings Challenger Replay Settings Args - The challenger replay settings for the Deployment.
- drift_
tracking_ Deploymentsettings Drift Tracking Settings Args - The drift tracking settings for the Deployment.
- health_
settings DeploymentHealth Settings Args - The health settings for this Deployment.
- importance str
- The importance of the Deployment.
- prediction_
intervals_ Deploymentsettings Prediction Intervals Settings Args - The prediction intervals settings for this Deployment.
- prediction_
warning_ Deploymentsettings Prediction Warning Settings Args - The prediction warning settings for the Deployment.
- predictions_
by_ Deploymentforecast_ date_ settings Predictions By Forecast Date Settings Args - The predictions by forecase date settings for the Deployment.
- predictions_
data_ Deploymentcollection_ settings Predictions Data Collection Settings Args - The predictions data collection settings for the Deployment.
- predictions_
settings DeploymentPredictions Settings Args - Settings for the predictions.
- segment_
analysis_ Deploymentsettings Segment Analysis Settings Args - The segment analysis settings for the Deployment.
- label String
- The label of the Deployment.
- prediction
Environment StringId - The ID of the predication environment for this Deployment.
- registered
Model StringVersion Id - The ID of the registered model version for this Deployment.
- association
Id Property MapSettings - Association ID settings for this Deployment.
- bias
And Property MapFairness Settings - Bias and fairness settings for the Deployment.
- challenger
Models Property MapSettings - The challenger models settings for the Deployment.
- challenger
Replay Property MapSettings - The challenger replay settings for the Deployment.
- drift
Tracking Property MapSettings - The drift tracking settings for the Deployment.
- health
Settings Property Map - The health settings for this Deployment.
- importance String
- The importance of the Deployment.
- prediction
Intervals Property MapSettings - The prediction intervals settings for this Deployment.
- prediction
Warning Property MapSettings - The prediction warning settings for the Deployment.
- predictions
By Property MapForecast Date Settings - The predictions by forecase date settings for the Deployment.
- predictions
Data Property MapCollection Settings - The predictions data collection settings for the Deployment.
- predictions
Settings Property Map - Settings for the predictions.
- segment
Analysis Property MapSettings - The segment analysis settings for the Deployment.
Outputs
All input properties are implicitly available as output properties. Additionally, the Deployment resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing Deployment Resource
Get an existing Deployment resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: DeploymentState, opts?: CustomResourceOptions): Deployment
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
association_id_settings: Optional[DeploymentAssociationIdSettingsArgs] = None,
bias_and_fairness_settings: Optional[DeploymentBiasAndFairnessSettingsArgs] = None,
challenger_models_settings: Optional[DeploymentChallengerModelsSettingsArgs] = None,
challenger_replay_settings: Optional[DeploymentChallengerReplaySettingsArgs] = None,
drift_tracking_settings: Optional[DeploymentDriftTrackingSettingsArgs] = None,
health_settings: Optional[DeploymentHealthSettingsArgs] = None,
importance: Optional[str] = None,
label: Optional[str] = None,
prediction_environment_id: Optional[str] = None,
prediction_intervals_settings: Optional[DeploymentPredictionIntervalsSettingsArgs] = None,
prediction_warning_settings: Optional[DeploymentPredictionWarningSettingsArgs] = None,
predictions_by_forecast_date_settings: Optional[DeploymentPredictionsByForecastDateSettingsArgs] = None,
predictions_data_collection_settings: Optional[DeploymentPredictionsDataCollectionSettingsArgs] = None,
predictions_settings: Optional[DeploymentPredictionsSettingsArgs] = None,
registered_model_version_id: Optional[str] = None,
segment_analysis_settings: Optional[DeploymentSegmentAnalysisSettingsArgs] = None) -> Deployment
func GetDeployment(ctx *Context, name string, id IDInput, state *DeploymentState, opts ...ResourceOption) (*Deployment, error)
public static Deployment Get(string name, Input<string> id, DeploymentState? state, CustomResourceOptions? opts = null)
public static Deployment get(String name, Output<String> id, DeploymentState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Association
Id DataSettings Robot Deployment Association Id Settings - Association ID settings for this Deployment.
- Bias
And DataFairness Settings Robot Deployment Bias And Fairness Settings - Bias and fairness settings for the Deployment.
- Challenger
Models DataSettings Robot Deployment Challenger Models Settings - The challenger models settings for the Deployment.
- Challenger
Replay DataSettings Robot Deployment Challenger Replay Settings - The challenger replay settings for the Deployment.
- Drift
Tracking DataSettings Robot Deployment Drift Tracking Settings - The drift tracking settings for the Deployment.
- Health
Settings DataRobot Deployment Health Settings - The health settings for this Deployment.
- Importance string
- The importance of the Deployment.
- Label string
- The label of the Deployment.
- Prediction
Environment stringId - The ID of the predication environment for this Deployment.
- Prediction
Intervals DataSettings Robot Deployment Prediction Intervals Settings - The prediction intervals settings for this Deployment.
- Prediction
Warning DataSettings Robot Deployment Prediction Warning Settings - The prediction warning settings for the Deployment.
- Predictions
By DataForecast Date Settings Robot Deployment Predictions By Forecast Date Settings - The predictions by forecase date settings for the Deployment.
- Predictions
Data DataCollection Settings Robot Deployment Predictions Data Collection Settings - The predictions data collection settings for the Deployment.
- Predictions
Settings DataRobot Deployment Predictions Settings - Settings for the predictions.
- Registered
Model stringVersion Id - The ID of the registered model version for this Deployment.
- Segment
Analysis DataSettings Robot Deployment Segment Analysis Settings - The segment analysis settings for the Deployment.
- Association
Id DeploymentSettings Association Id Settings Args - Association ID settings for this Deployment.
- Bias
And DeploymentFairness Settings Bias And Fairness Settings Args - Bias and fairness settings for the Deployment.
- Challenger
Models DeploymentSettings Challenger Models Settings Args - The challenger models settings for the Deployment.
- Challenger
Replay DeploymentSettings Challenger Replay Settings Args - The challenger replay settings for the Deployment.
- Drift
Tracking DeploymentSettings Drift Tracking Settings Args - The drift tracking settings for the Deployment.
- Health
Settings DeploymentHealth Settings Args - The health settings for this Deployment.
- Importance string
- The importance of the Deployment.
- Label string
- The label of the Deployment.
- Prediction
Environment stringId - The ID of the predication environment for this Deployment.
- Prediction
Intervals DeploymentSettings Prediction Intervals Settings Args - The prediction intervals settings for this Deployment.
- Prediction
Warning DeploymentSettings Prediction Warning Settings Args - The prediction warning settings for the Deployment.
- Predictions
By DeploymentForecast Date Settings Predictions By Forecast Date Settings Args - The predictions by forecase date settings for the Deployment.
- Predictions
Data DeploymentCollection Settings Predictions Data Collection Settings Args - The predictions data collection settings for the Deployment.
- Predictions
Settings DeploymentPredictions Settings Args - Settings for the predictions.
- Registered
Model stringVersion Id - The ID of the registered model version for this Deployment.
- Segment
Analysis DeploymentSettings Segment Analysis Settings Args - The segment analysis settings for the Deployment.
- association
Id DeploymentSettings Association Id Settings - Association ID settings for this Deployment.
- bias
And DeploymentFairness Settings Bias And Fairness Settings - Bias and fairness settings for the Deployment.
- challenger
Models DeploymentSettings Challenger Models Settings - The challenger models settings for the Deployment.
- challenger
Replay DeploymentSettings Challenger Replay Settings - The challenger replay settings for the Deployment.
- drift
Tracking DeploymentSettings Drift Tracking Settings - The drift tracking settings for the Deployment.
- health
Settings DeploymentHealth Settings - The health settings for this Deployment.
- importance String
- The importance of the Deployment.
- label String
- The label of the Deployment.
- prediction
Environment StringId - The ID of the predication environment for this Deployment.
- prediction
Intervals DeploymentSettings Prediction Intervals Settings - The prediction intervals settings for this Deployment.
- prediction
Warning DeploymentSettings Prediction Warning Settings - The prediction warning settings for the Deployment.
- predictions
By DeploymentForecast Date Settings Predictions By Forecast Date Settings - The predictions by forecase date settings for the Deployment.
- predictions
Data DeploymentCollection Settings Predictions Data Collection Settings - The predictions data collection settings for the Deployment.
- predictions
Settings DeploymentPredictions Settings - Settings for the predictions.
- registered
Model StringVersion Id - The ID of the registered model version for this Deployment.
- segment
Analysis DeploymentSettings Segment Analysis Settings - The segment analysis settings for the Deployment.
- association
Id DeploymentSettings Association Id Settings - Association ID settings for this Deployment.
- bias
And DeploymentFairness Settings Bias And Fairness Settings - Bias and fairness settings for the Deployment.
- challenger
Models DeploymentSettings Challenger Models Settings - The challenger models settings for the Deployment.
- challenger
Replay DeploymentSettings Challenger Replay Settings - The challenger replay settings for the Deployment.
- drift
Tracking DeploymentSettings Drift Tracking Settings - The drift tracking settings for the Deployment.
- health
Settings DeploymentHealth Settings - The health settings for this Deployment.
- importance string
- The importance of the Deployment.
- label string
- The label of the Deployment.
- prediction
Environment stringId - The ID of the predication environment for this Deployment.
- prediction
Intervals DeploymentSettings Prediction Intervals Settings - The prediction intervals settings for this Deployment.
- prediction
Warning DeploymentSettings Prediction Warning Settings - The prediction warning settings for the Deployment.
- predictions
By DeploymentForecast Date Settings Predictions By Forecast Date Settings - The predictions by forecase date settings for the Deployment.
- predictions
Data DeploymentCollection Settings Predictions Data Collection Settings - The predictions data collection settings for the Deployment.
- predictions
Settings DeploymentPredictions Settings - Settings for the predictions.
- registered
Model stringVersion Id - The ID of the registered model version for this Deployment.
- segment
Analysis DeploymentSettings Segment Analysis Settings - The segment analysis settings for the Deployment.
- association_
id_ Deploymentsettings Association Id Settings Args - Association ID settings for this Deployment.
- bias_
and_ Deploymentfairness_ settings Bias And Fairness Settings Args - Bias and fairness settings for the Deployment.
- challenger_
models_ Deploymentsettings Challenger Models Settings Args - The challenger models settings for the Deployment.
- challenger_
replay_ Deploymentsettings Challenger Replay Settings Args - The challenger replay settings for the Deployment.
- drift_
tracking_ Deploymentsettings Drift Tracking Settings Args - The drift tracking settings for the Deployment.
- health_
settings DeploymentHealth Settings Args - The health settings for this Deployment.
- importance str
- The importance of the Deployment.
- label str
- The label of the Deployment.
- prediction_
environment_ strid - The ID of the predication environment for this Deployment.
- prediction_
intervals_ Deploymentsettings Prediction Intervals Settings Args - The prediction intervals settings for this Deployment.
- prediction_
warning_ Deploymentsettings Prediction Warning Settings Args - The prediction warning settings for the Deployment.
- predictions_
by_ Deploymentforecast_ date_ settings Predictions By Forecast Date Settings Args - The predictions by forecase date settings for the Deployment.
- predictions_
data_ Deploymentcollection_ settings Predictions Data Collection Settings Args - The predictions data collection settings for the Deployment.
- predictions_
settings DeploymentPredictions Settings Args - Settings for the predictions.
- registered_
model_ strversion_ id - The ID of the registered model version for this Deployment.
- segment_
analysis_ Deploymentsettings Segment Analysis Settings Args - The segment analysis settings for the Deployment.
- association
Id Property MapSettings - Association ID settings for this Deployment.
- bias
And Property MapFairness Settings - Bias and fairness settings for the Deployment.
- challenger
Models Property MapSettings - The challenger models settings for the Deployment.
- challenger
Replay Property MapSettings - The challenger replay settings for the Deployment.
- drift
Tracking Property MapSettings - The drift tracking settings for the Deployment.
- health
Settings Property Map - The health settings for this Deployment.
- importance String
- The importance of the Deployment.
- label String
- The label of the Deployment.
- prediction
Environment StringId - The ID of the predication environment for this Deployment.
- prediction
Intervals Property MapSettings - The prediction intervals settings for this Deployment.
- prediction
Warning Property MapSettings - The prediction warning settings for the Deployment.
- predictions
By Property MapForecast Date Settings - The predictions by forecase date settings for the Deployment.
- predictions
Data Property MapCollection Settings - The predictions data collection settings for the Deployment.
- predictions
Settings Property Map - Settings for the predictions.
- registered
Model StringVersion Id - The ID of the registered model version for this Deployment.
- segment
Analysis Property MapSettings - The segment analysis settings for the Deployment.
Supporting Types
DeploymentAssociationIdSettings, DeploymentAssociationIdSettingsArgs
- Auto
Generate boolId - Whether to auto generate ID.
- Column
Names List<string> - Name of the columns to be used as association ID, currently only support a list of one string.
- Required
In boolPrediction Requests - Whether the association ID column is required in prediction requests.
- Auto
Generate boolId - Whether to auto generate ID.
- Column
Names []string - Name of the columns to be used as association ID, currently only support a list of one string.
- Required
In boolPrediction Requests - Whether the association ID column is required in prediction requests.
- auto
Generate BooleanId - Whether to auto generate ID.
- column
Names List<String> - Name of the columns to be used as association ID, currently only support a list of one string.
- required
In BooleanPrediction Requests - Whether the association ID column is required in prediction requests.
- auto
Generate booleanId - Whether to auto generate ID.
- column
Names string[] - Name of the columns to be used as association ID, currently only support a list of one string.
- required
In booleanPrediction Requests - Whether the association ID column is required in prediction requests.
- auto_
generate_ boolid - Whether to auto generate ID.
- column_
names Sequence[str] - Name of the columns to be used as association ID, currently only support a list of one string.
- required_
in_ boolprediction_ requests - Whether the association ID column is required in prediction requests.
- auto
Generate BooleanId - Whether to auto generate ID.
- column
Names List<String> - Name of the columns to be used as association ID, currently only support a list of one string.
- required
In BooleanPrediction Requests - Whether the association ID column is required in prediction requests.
DeploymentBiasAndFairnessSettings, DeploymentBiasAndFairnessSettingsArgs
- Fairness
Metric stringSet - A set of fairness metrics to use for calculating fairness.
- Fairness
Threshold double - Threshold value of the fairness metric. Cannot be less than 0 or greater than 1.
- Preferable
Target boolValue - A target value that should be treated as a positive outcome for the prediction.
- Protected
Features List<string> - A list of features to mark as protected.
- Fairness
Metric stringSet - A set of fairness metrics to use for calculating fairness.
- Fairness
Threshold float64 - Threshold value of the fairness metric. Cannot be less than 0 or greater than 1.
- Preferable
Target boolValue - A target value that should be treated as a positive outcome for the prediction.
- Protected
Features []string - A list of features to mark as protected.
- fairness
Metric StringSet - A set of fairness metrics to use for calculating fairness.
- fairness
Threshold Double - Threshold value of the fairness metric. Cannot be less than 0 or greater than 1.
- preferable
Target BooleanValue - A target value that should be treated as a positive outcome for the prediction.
- protected
Features List<String> - A list of features to mark as protected.
- fairness
Metric stringSet - A set of fairness metrics to use for calculating fairness.
- fairness
Threshold number - Threshold value of the fairness metric. Cannot be less than 0 or greater than 1.
- preferable
Target booleanValue - A target value that should be treated as a positive outcome for the prediction.
- protected
Features string[] - A list of features to mark as protected.
- fairness_
metric_ strset - A set of fairness metrics to use for calculating fairness.
- fairness_
threshold float - Threshold value of the fairness metric. Cannot be less than 0 or greater than 1.
- preferable_
target_ boolvalue - A target value that should be treated as a positive outcome for the prediction.
- protected_
features Sequence[str] - A list of features to mark as protected.
- fairness
Metric StringSet - A set of fairness metrics to use for calculating fairness.
- fairness
Threshold Number - Threshold value of the fairness metric. Cannot be less than 0 or greater than 1.
- preferable
Target BooleanValue - A target value that should be treated as a positive outcome for the prediction.
- protected
Features List<String> - A list of features to mark as protected.
DeploymentChallengerModelsSettings, DeploymentChallengerModelsSettingsArgs
- Enabled bool
- Is 'True' if challenger models is enabled for this deployment.
- Enabled bool
- Is 'True' if challenger models is enabled for this deployment.
- enabled Boolean
- Is 'True' if challenger models is enabled for this deployment.
- enabled boolean
- Is 'True' if challenger models is enabled for this deployment.
- enabled bool
- Is 'True' if challenger models is enabled for this deployment.
- enabled Boolean
- Is 'True' if challenger models is enabled for this deployment.
DeploymentChallengerReplaySettings, DeploymentChallengerReplaySettingsArgs
- Enabled bool
- If challenger replay is enabled.
- Enabled bool
- If challenger replay is enabled.
- enabled Boolean
- If challenger replay is enabled.
- enabled boolean
- If challenger replay is enabled.
- enabled bool
- If challenger replay is enabled.
- enabled Boolean
- If challenger replay is enabled.
DeploymentDriftTrackingSettings, DeploymentDriftTrackingSettingsArgs
- Feature
Drift boolEnabled - If feature drift tracking is to be turned on.
- Target
Drift boolEnabled - If target drift tracking is to be turned on.
- Feature
Drift boolEnabled - If feature drift tracking is to be turned on.
- Target
Drift boolEnabled - If target drift tracking is to be turned on.
- feature
Drift BooleanEnabled - If feature drift tracking is to be turned on.
- target
Drift BooleanEnabled - If target drift tracking is to be turned on.
- feature
Drift booleanEnabled - If feature drift tracking is to be turned on.
- target
Drift booleanEnabled - If target drift tracking is to be turned on.
- feature_
drift_ boolenabled - If feature drift tracking is to be turned on.
- target_
drift_ boolenabled - If target drift tracking is to be turned on.
- feature
Drift BooleanEnabled - If feature drift tracking is to be turned on.
- target
Drift BooleanEnabled - If target drift tracking is to be turned on.
DeploymentHealthSettings, DeploymentHealthSettingsArgs
- Accuracy
Data
Robot Deployment Health Settings Accuracy - The accuracy health settings for this Deployment.
- Actuals
Timeliness DataRobot Deployment Health Settings Actuals Timeliness - The actuals timeliness health settings for this Deployment.
- Custom
Metrics DataRobot Deployment Health Settings Custom Metrics - The custom metrics health settings for this Deployment.
- Data
Drift DataRobot Deployment Health Settings Data Drift - The data drift health settings for this Deployment.
- Fairness
Data
Robot Deployment Health Settings Fairness - The fairness health settings for this Deployment.
- Predictions
Timeliness DataRobot Deployment Health Settings Predictions Timeliness - The predictions timeliness health settings for this Deployment.
- Service
Data
Robot Deployment Health Settings Service - The service health settings for this Deployment.
- Accuracy
Deployment
Health Settings Accuracy - The accuracy health settings for this Deployment.
- Actuals
Timeliness DeploymentHealth Settings Actuals Timeliness - The actuals timeliness health settings for this Deployment.
- Custom
Metrics DeploymentHealth Settings Custom Metrics - The custom metrics health settings for this Deployment.
- Data
Drift DeploymentHealth Settings Data Drift - The data drift health settings for this Deployment.
- Fairness
Deployment
Health Settings Fairness - The fairness health settings for this Deployment.
- Predictions
Timeliness DeploymentHealth Settings Predictions Timeliness - The predictions timeliness health settings for this Deployment.
- Service
Deployment
Health Settings Service - The service health settings for this Deployment.
- accuracy
Deployment
Health Settings Accuracy - The accuracy health settings for this Deployment.
- actuals
Timeliness DeploymentHealth Settings Actuals Timeliness - The actuals timeliness health settings for this Deployment.
- custom
Metrics DeploymentHealth Settings Custom Metrics - The custom metrics health settings for this Deployment.
- data
Drift DeploymentHealth Settings Data Drift - The data drift health settings for this Deployment.
- fairness
Deployment
Health Settings Fairness - The fairness health settings for this Deployment.
- predictions
Timeliness DeploymentHealth Settings Predictions Timeliness - The predictions timeliness health settings for this Deployment.
- service
Deployment
Health Settings Service - The service health settings for this Deployment.
- accuracy
Deployment
Health Settings Accuracy - The accuracy health settings for this Deployment.
- actuals
Timeliness DeploymentHealth Settings Actuals Timeliness - The actuals timeliness health settings for this Deployment.
- custom
Metrics DeploymentHealth Settings Custom Metrics - The custom metrics health settings for this Deployment.
- data
Drift DeploymentHealth Settings Data Drift - The data drift health settings for this Deployment.
- fairness
Deployment
Health Settings Fairness - The fairness health settings for this Deployment.
- predictions
Timeliness DeploymentHealth Settings Predictions Timeliness - The predictions timeliness health settings for this Deployment.
- service
Deployment
Health Settings Service - The service health settings for this Deployment.
- accuracy
Deployment
Health Settings Accuracy - The accuracy health settings for this Deployment.
- actuals_
timeliness DeploymentHealth Settings Actuals Timeliness - The actuals timeliness health settings for this Deployment.
- custom_
metrics DeploymentHealth Settings Custom Metrics - The custom metrics health settings for this Deployment.
- data_
drift DeploymentHealth Settings Data Drift - The data drift health settings for this Deployment.
- fairness
Deployment
Health Settings Fairness - The fairness health settings for this Deployment.
- predictions_
timeliness DeploymentHealth Settings Predictions Timeliness - The predictions timeliness health settings for this Deployment.
- service
Deployment
Health Settings Service - The service health settings for this Deployment.
- accuracy Property Map
- The accuracy health settings for this Deployment.
- actuals
Timeliness Property Map - The actuals timeliness health settings for this Deployment.
- custom
Metrics Property Map - The custom metrics health settings for this Deployment.
- data
Drift Property Map - The data drift health settings for this Deployment.
- fairness Property Map
- The fairness health settings for this Deployment.
- predictions
Timeliness Property Map - The predictions timeliness health settings for this Deployment.
- service Property Map
- The service health settings for this Deployment.
DeploymentHealthSettingsAccuracy, DeploymentHealthSettingsAccuracyArgs
- Batch
Count int - The batch count for the accuracy health settings.
- Failing
Threshold double - The failing threshold for the accuracy health settings.
- Measurement string
- The measurement for the accuracy health settings.
- Metric string
- The metric for the accuracy health settings.
- Warning
Threshold double - The warning threshold for the accuracy health settings.
- Batch
Count int - The batch count for the accuracy health settings.
- Failing
Threshold float64 - The failing threshold for the accuracy health settings.
- Measurement string
- The measurement for the accuracy health settings.
- Metric string
- The metric for the accuracy health settings.
- Warning
Threshold float64 - The warning threshold for the accuracy health settings.
- batch
Count Integer - The batch count for the accuracy health settings.
- failing
Threshold Double - The failing threshold for the accuracy health settings.
- measurement String
- The measurement for the accuracy health settings.
- metric String
- The metric for the accuracy health settings.
- warning
Threshold Double - The warning threshold for the accuracy health settings.
- batch
Count number - The batch count for the accuracy health settings.
- failing
Threshold number - The failing threshold for the accuracy health settings.
- measurement string
- The measurement for the accuracy health settings.
- metric string
- The metric for the accuracy health settings.
- warning
Threshold number - The warning threshold for the accuracy health settings.
- batch_
count int - The batch count for the accuracy health settings.
- failing_
threshold float - The failing threshold for the accuracy health settings.
- measurement str
- The measurement for the accuracy health settings.
- metric str
- The metric for the accuracy health settings.
- warning_
threshold float - The warning threshold for the accuracy health settings.
- batch
Count Number - The batch count for the accuracy health settings.
- failing
Threshold Number - The failing threshold for the accuracy health settings.
- measurement String
- The measurement for the accuracy health settings.
- metric String
- The metric for the accuracy health settings.
- warning
Threshold Number - The warning threshold for the accuracy health settings.
DeploymentHealthSettingsActualsTimeliness, DeploymentHealthSettingsActualsTimelinessArgs
- Enabled bool
- If acutals timeliness is enabled for this Deployment.
- Expected
Frequency string - The expected frequency for the actuals timeliness health settings.
- Enabled bool
- If acutals timeliness is enabled for this Deployment.
- Expected
Frequency string - The expected frequency for the actuals timeliness health settings.
- enabled Boolean
- If acutals timeliness is enabled for this Deployment.
- expected
Frequency String - The expected frequency for the actuals timeliness health settings.
- enabled boolean
- If acutals timeliness is enabled for this Deployment.
- expected
Frequency string - The expected frequency for the actuals timeliness health settings.
- enabled bool
- If acutals timeliness is enabled for this Deployment.
- expected_
frequency str - The expected frequency for the actuals timeliness health settings.
- enabled Boolean
- If acutals timeliness is enabled for this Deployment.
- expected
Frequency String - The expected frequency for the actuals timeliness health settings.
DeploymentHealthSettingsCustomMetrics, DeploymentHealthSettingsCustomMetricsArgs
- Failing
Conditions List<DataRobot Deployment Health Settings Custom Metrics Failing Condition> - The failing conditions for the custom metrics health settings.
- Warning
Conditions List<DataRobot Deployment Health Settings Custom Metrics Warning Condition> - The warning conditions for the custom metrics health settings.
- Failing
Conditions []DeploymentHealth Settings Custom Metrics Failing Condition - The failing conditions for the custom metrics health settings.
- Warning
Conditions []DeploymentHealth Settings Custom Metrics Warning Condition - The warning conditions for the custom metrics health settings.
- failing
Conditions List<DeploymentHealth Settings Custom Metrics Failing Condition> - The failing conditions for the custom metrics health settings.
- warning
Conditions List<DeploymentHealth Settings Custom Metrics Warning Condition> - The warning conditions for the custom metrics health settings.
- failing
Conditions DeploymentHealth Settings Custom Metrics Failing Condition[] - The failing conditions for the custom metrics health settings.
- warning
Conditions DeploymentHealth Settings Custom Metrics Warning Condition[] - The warning conditions for the custom metrics health settings.
- failing_
conditions Sequence[DeploymentHealth Settings Custom Metrics Failing Condition] - The failing conditions for the custom metrics health settings.
- warning_
conditions Sequence[DeploymentHealth Settings Custom Metrics Warning Condition] - The warning conditions for the custom metrics health settings.
- failing
Conditions List<Property Map> - The failing conditions for the custom metrics health settings.
- warning
Conditions List<Property Map> - The warning conditions for the custom metrics health settings.
DeploymentHealthSettingsCustomMetricsFailingCondition, DeploymentHealthSettingsCustomMetricsFailingConditionArgs
- Compare
Operator string - The compare operator for the failing condition of the custom metrics health settings.
- Metric
Id string - The metric ID for the failing condition of the custom metrics health settings.
- Threshold double
- The threshold for the failing condition of the custom metrics health settings.
- Compare
Operator string - The compare operator for the failing condition of the custom metrics health settings.
- Metric
Id string - The metric ID for the failing condition of the custom metrics health settings.
- Threshold float64
- The threshold for the failing condition of the custom metrics health settings.
- compare
Operator String - The compare operator for the failing condition of the custom metrics health settings.
- metric
Id String - The metric ID for the failing condition of the custom metrics health settings.
- threshold Double
- The threshold for the failing condition of the custom metrics health settings.
- compare
Operator string - The compare operator for the failing condition of the custom metrics health settings.
- metric
Id string - The metric ID for the failing condition of the custom metrics health settings.
- threshold number
- The threshold for the failing condition of the custom metrics health settings.
- compare_
operator str - The compare operator for the failing condition of the custom metrics health settings.
- metric_
id str - The metric ID for the failing condition of the custom metrics health settings.
- threshold float
- The threshold for the failing condition of the custom metrics health settings.
- compare
Operator String - The compare operator for the failing condition of the custom metrics health settings.
- metric
Id String - The metric ID for the failing condition of the custom metrics health settings.
- threshold Number
- The threshold for the failing condition of the custom metrics health settings.
DeploymentHealthSettingsCustomMetricsWarningCondition, DeploymentHealthSettingsCustomMetricsWarningConditionArgs
- Compare
Operator string - The compare operator for the warning condition of the custom metrics health settings.
- Metric
Id string - The metric ID for the warning condition of the custom metrics health settings.
- Threshold double
- The threshold for the warning condition of the custom metrics health settings.
- Compare
Operator string - The compare operator for the warning condition of the custom metrics health settings.
- Metric
Id string - The metric ID for the warning condition of the custom metrics health settings.
- Threshold float64
- The threshold for the warning condition of the custom metrics health settings.
- compare
Operator String - The compare operator for the warning condition of the custom metrics health settings.
- metric
Id String - The metric ID for the warning condition of the custom metrics health settings.
- threshold Double
- The threshold for the warning condition of the custom metrics health settings.
- compare
Operator string - The compare operator for the warning condition of the custom metrics health settings.
- metric
Id string - The metric ID for the warning condition of the custom metrics health settings.
- threshold number
- The threshold for the warning condition of the custom metrics health settings.
- compare_
operator str - The compare operator for the warning condition of the custom metrics health settings.
- metric_
id str - The metric ID for the warning condition of the custom metrics health settings.
- threshold float
- The threshold for the warning condition of the custom metrics health settings.
- compare
Operator String - The compare operator for the warning condition of the custom metrics health settings.
- metric
Id String - The metric ID for the warning condition of the custom metrics health settings.
- threshold Number
- The threshold for the warning condition of the custom metrics health settings.
DeploymentHealthSettingsDataDrift, DeploymentHealthSettingsDataDriftArgs
- Batch
Count int - The batch count for the data drift health settings.
- Drift
Threshold double - The drift threshold for the data drift health settings.
- Exclude
Features List<string> - The exclude features for the data drift health settings.
- High
Importance intFailing Count - The high importance failing count for the data drift health settings.
- High
Importance intWarning Count - The high importance warning count for the data drift health settings.
- Importance
Threshold double - The importance threshold for the data drift health settings.
- Low
Importance intFailing Count - The low importance failing count for the data drift health settings.
- Low
Importance intWarning Count - The low importance warning count for the data drift health settings.
- Starred
Features List<string> - The starred features for the data drift health settings.
- Time
Interval string - The time interval for the data drift health settings.
- Batch
Count int - The batch count for the data drift health settings.
- Drift
Threshold float64 - The drift threshold for the data drift health settings.
- Exclude
Features []string - The exclude features for the data drift health settings.
- High
Importance intFailing Count - The high importance failing count for the data drift health settings.
- High
Importance intWarning Count - The high importance warning count for the data drift health settings.
- Importance
Threshold float64 - The importance threshold for the data drift health settings.
- Low
Importance intFailing Count - The low importance failing count for the data drift health settings.
- Low
Importance intWarning Count - The low importance warning count for the data drift health settings.
- Starred
Features []string - The starred features for the data drift health settings.
- Time
Interval string - The time interval for the data drift health settings.
- batch
Count Integer - The batch count for the data drift health settings.
- drift
Threshold Double - The drift threshold for the data drift health settings.
- exclude
Features List<String> - The exclude features for the data drift health settings.
- high
Importance IntegerFailing Count - The high importance failing count for the data drift health settings.
- high
Importance IntegerWarning Count - The high importance warning count for the data drift health settings.
- importance
Threshold Double - The importance threshold for the data drift health settings.
- low
Importance IntegerFailing Count - The low importance failing count for the data drift health settings.
- low
Importance IntegerWarning Count - The low importance warning count for the data drift health settings.
- starred
Features List<String> - The starred features for the data drift health settings.
- time
Interval String - The time interval for the data drift health settings.
- batch
Count number - The batch count for the data drift health settings.
- drift
Threshold number - The drift threshold for the data drift health settings.
- exclude
Features string[] - The exclude features for the data drift health settings.
- high
Importance numberFailing Count - The high importance failing count for the data drift health settings.
- high
Importance numberWarning Count - The high importance warning count for the data drift health settings.
- importance
Threshold number - The importance threshold for the data drift health settings.
- low
Importance numberFailing Count - The low importance failing count for the data drift health settings.
- low
Importance numberWarning Count - The low importance warning count for the data drift health settings.
- starred
Features string[] - The starred features for the data drift health settings.
- time
Interval string - The time interval for the data drift health settings.
- batch_
count int - The batch count for the data drift health settings.
- drift_
threshold float - The drift threshold for the data drift health settings.
- exclude_
features Sequence[str] - The exclude features for the data drift health settings.
- high_
importance_ intfailing_ count - The high importance failing count for the data drift health settings.
- high_
importance_ intwarning_ count - The high importance warning count for the data drift health settings.
- importance_
threshold float - The importance threshold for the data drift health settings.
- low_
importance_ intfailing_ count - The low importance failing count for the data drift health settings.
- low_
importance_ intwarning_ count - The low importance warning count for the data drift health settings.
- starred_
features Sequence[str] - The starred features for the data drift health settings.
- time_
interval str - The time interval for the data drift health settings.
- batch
Count Number - The batch count for the data drift health settings.
- drift
Threshold Number - The drift threshold for the data drift health settings.
- exclude
Features List<String> - The exclude features for the data drift health settings.
- high
Importance NumberFailing Count - The high importance failing count for the data drift health settings.
- high
Importance NumberWarning Count - The high importance warning count for the data drift health settings.
- importance
Threshold Number - The importance threshold for the data drift health settings.
- low
Importance NumberFailing Count - The low importance failing count for the data drift health settings.
- low
Importance NumberWarning Count - The low importance warning count for the data drift health settings.
- starred
Features List<String> - The starred features for the data drift health settings.
- time
Interval String - The time interval for the data drift health settings.
DeploymentHealthSettingsFairness, DeploymentHealthSettingsFairnessArgs
- Protected
Class intFailing Count - The protected class failing count for the fairness health settings.
- Protected
Class intWarning Count - The protected class warning count for the fairness health settings.
- Protected
Class intFailing Count - The protected class failing count for the fairness health settings.
- Protected
Class intWarning Count - The protected class warning count for the fairness health settings.
- protected
Class IntegerFailing Count - The protected class failing count for the fairness health settings.
- protected
Class IntegerWarning Count - The protected class warning count for the fairness health settings.
- protected
Class numberFailing Count - The protected class failing count for the fairness health settings.
- protected
Class numberWarning Count - The protected class warning count for the fairness health settings.
- protected_
class_ intfailing_ count - The protected class failing count for the fairness health settings.
- protected_
class_ intwarning_ count - The protected class warning count for the fairness health settings.
- protected
Class NumberFailing Count - The protected class failing count for the fairness health settings.
- protected
Class NumberWarning Count - The protected class warning count for the fairness health settings.
DeploymentHealthSettingsPredictionsTimeliness, DeploymentHealthSettingsPredictionsTimelinessArgs
- Enabled bool
- If predictions timeliness is enabled for this Deployment.
- Expected
Frequency string - The expected frequency for the predictions timeliness health settings.
- Enabled bool
- If predictions timeliness is enabled for this Deployment.
- Expected
Frequency string - The expected frequency for the predictions timeliness health settings.
- enabled Boolean
- If predictions timeliness is enabled for this Deployment.
- expected
Frequency String - The expected frequency for the predictions timeliness health settings.
- enabled boolean
- If predictions timeliness is enabled for this Deployment.
- expected
Frequency string - The expected frequency for the predictions timeliness health settings.
- enabled bool
- If predictions timeliness is enabled for this Deployment.
- expected_
frequency str - The expected frequency for the predictions timeliness health settings.
- enabled Boolean
- If predictions timeliness is enabled for this Deployment.
- expected
Frequency String - The expected frequency for the predictions timeliness health settings.
DeploymentHealthSettingsService, DeploymentHealthSettingsServiceArgs
- Batch
Count int - The batch count for the service health settings.
- Batch
Count int - The batch count for the service health settings.
- batch
Count Integer - The batch count for the service health settings.
- batch
Count number - The batch count for the service health settings.
- batch_
count int - The batch count for the service health settings.
- batch
Count Number - The batch count for the service health settings.
DeploymentPredictionIntervalsSettings, DeploymentPredictionIntervalsSettingsArgs
- Enabled bool
- Whether prediction intervals are enabled for this deployment.
- Percentiles List<int>
- List of enabled prediction intervals’ sizes for this deployment.
- Enabled bool
- Whether prediction intervals are enabled for this deployment.
- Percentiles []int
- List of enabled prediction intervals’ sizes for this deployment.
- enabled Boolean
- Whether prediction intervals are enabled for this deployment.
- percentiles List<Integer>
- List of enabled prediction intervals’ sizes for this deployment.
- enabled boolean
- Whether prediction intervals are enabled for this deployment.
- percentiles number[]
- List of enabled prediction intervals’ sizes for this deployment.
- enabled bool
- Whether prediction intervals are enabled for this deployment.
- percentiles Sequence[int]
- List of enabled prediction intervals’ sizes for this deployment.
- enabled Boolean
- Whether prediction intervals are enabled for this deployment.
- percentiles List<Number>
- List of enabled prediction intervals’ sizes for this deployment.
DeploymentPredictionWarningSettings, DeploymentPredictionWarningSettingsArgs
- Enabled bool
- If target prediction warning is enabled for this Deployment.
- Custom
Boundaries DataRobot Deployment Prediction Warning Settings Custom Boundaries - The custom boundaries for prediction warnings.
- Enabled bool
- If target prediction warning is enabled for this Deployment.
- Custom
Boundaries DeploymentPrediction Warning Settings Custom Boundaries - The custom boundaries for prediction warnings.
- enabled Boolean
- If target prediction warning is enabled for this Deployment.
- custom
Boundaries DeploymentPrediction Warning Settings Custom Boundaries - The custom boundaries for prediction warnings.
- enabled boolean
- If target prediction warning is enabled for this Deployment.
- custom
Boundaries DeploymentPrediction Warning Settings Custom Boundaries - The custom boundaries for prediction warnings.
- enabled bool
- If target prediction warning is enabled for this Deployment.
- custom_
boundaries DeploymentPrediction Warning Settings Custom Boundaries - The custom boundaries for prediction warnings.
- enabled Boolean
- If target prediction warning is enabled for this Deployment.
- custom
Boundaries Property Map - The custom boundaries for prediction warnings.
DeploymentPredictionWarningSettingsCustomBoundaries, DeploymentPredictionWarningSettingsCustomBoundariesArgs
- Lower
Boundary double - All predictions less than provided value will be considered anomalous.
- Upper
Boundary double - All predictions greater than provided value will be considered anomalous.
- Lower
Boundary float64 - All predictions less than provided value will be considered anomalous.
- Upper
Boundary float64 - All predictions greater than provided value will be considered anomalous.
- lower
Boundary Double - All predictions less than provided value will be considered anomalous.
- upper
Boundary Double - All predictions greater than provided value will be considered anomalous.
- lower
Boundary number - All predictions less than provided value will be considered anomalous.
- upper
Boundary number - All predictions greater than provided value will be considered anomalous.
- lower_
boundary float - All predictions less than provided value will be considered anomalous.
- upper_
boundary float - All predictions greater than provided value will be considered anomalous.
- lower
Boundary Number - All predictions less than provided value will be considered anomalous.
- upper
Boundary Number - All predictions greater than provided value will be considered anomalous.
DeploymentPredictionsByForecastDateSettings, DeploymentPredictionsByForecastDateSettingsArgs
- Enabled bool
- Is ’True’ if predictions by forecast date is enabled for this deployment.
- Column
Name string - The column name in prediction datasets to be used as forecast date.
- Datetime
Format string - The datetime format of the forecast date column in prediction datasets.
- Enabled bool
- Is ’True’ if predictions by forecast date is enabled for this deployment.
- Column
Name string - The column name in prediction datasets to be used as forecast date.
- Datetime
Format string - The datetime format of the forecast date column in prediction datasets.
- enabled Boolean
- Is ’True’ if predictions by forecast date is enabled for this deployment.
- column
Name String - The column name in prediction datasets to be used as forecast date.
- datetime
Format String - The datetime format of the forecast date column in prediction datasets.
- enabled boolean
- Is ’True’ if predictions by forecast date is enabled for this deployment.
- column
Name string - The column name in prediction datasets to be used as forecast date.
- datetime
Format string - The datetime format of the forecast date column in prediction datasets.
- enabled bool
- Is ’True’ if predictions by forecast date is enabled for this deployment.
- column_
name str - The column name in prediction datasets to be used as forecast date.
- datetime_
format str - The datetime format of the forecast date column in prediction datasets.
- enabled Boolean
- Is ’True’ if predictions by forecast date is enabled for this deployment.
- column
Name String - The column name in prediction datasets to be used as forecast date.
- datetime
Format String - The datetime format of the forecast date column in prediction datasets.
DeploymentPredictionsDataCollectionSettings, DeploymentPredictionsDataCollectionSettingsArgs
- Enabled bool
- If predictions data collections is enabled for this Deployment.
- Enabled bool
- If predictions data collections is enabled for this Deployment.
- enabled Boolean
- If predictions data collections is enabled for this Deployment.
- enabled boolean
- If predictions data collections is enabled for this Deployment.
- enabled bool
- If predictions data collections is enabled for this Deployment.
- enabled Boolean
- If predictions data collections is enabled for this Deployment.
DeploymentPredictionsSettings, DeploymentPredictionsSettingsArgs
- Max
Computes int - The maximum number of computes to use for predictions.
- Min
Computes int - The minimum number of computes to use for predictions.
- Real
Time bool - Whether to use real-time predictions.
- Max
Computes int - The maximum number of computes to use for predictions.
- Min
Computes int - The minimum number of computes to use for predictions.
- Real
Time bool - Whether to use real-time predictions.
- max
Computes Integer - The maximum number of computes to use for predictions.
- min
Computes Integer - The minimum number of computes to use for predictions.
- real
Time Boolean - Whether to use real-time predictions.
- max
Computes number - The maximum number of computes to use for predictions.
- min
Computes number - The minimum number of computes to use for predictions.
- real
Time boolean - Whether to use real-time predictions.
- max_
computes int - The maximum number of computes to use for predictions.
- min_
computes int - The minimum number of computes to use for predictions.
- real_
time bool - Whether to use real-time predictions.
- max
Computes Number - The maximum number of computes to use for predictions.
- min
Computes Number - The minimum number of computes to use for predictions.
- real
Time Boolean - Whether to use real-time predictions.
DeploymentSegmentAnalysisSettings, DeploymentSegmentAnalysisSettingsArgs
- Enabled bool
- Set to 'True' if segment analysis is enabled for this deployment.
- Attributes List<string>
- A list of strings that gives the segment attributes selected for tracking.
- Enabled bool
- Set to 'True' if segment analysis is enabled for this deployment.
- Attributes []string
- A list of strings that gives the segment attributes selected for tracking.
- enabled Boolean
- Set to 'True' if segment analysis is enabled for this deployment.
- attributes List<String>
- A list of strings that gives the segment attributes selected for tracking.
- enabled boolean
- Set to 'True' if segment analysis is enabled for this deployment.
- attributes string[]
- A list of strings that gives the segment attributes selected for tracking.
- enabled bool
- Set to 'True' if segment analysis is enabled for this deployment.
- attributes Sequence[str]
- A list of strings that gives the segment attributes selected for tracking.
- enabled Boolean
- Set to 'True' if segment analysis is enabled for this deployment.
- attributes List<String>
- A list of strings that gives the segment attributes selected for tracking.
Package Details
- Repository
- datarobot datarobot-community/pulumi-datarobot
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
datarobot
Terraform Provider.
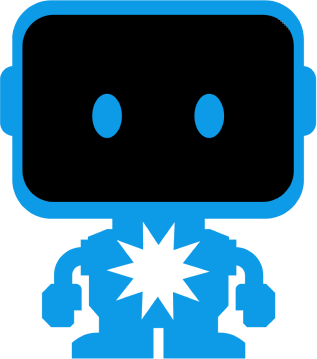
DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.