DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.
datarobot.PredictionEnvironment
Explore with Pulumi AI
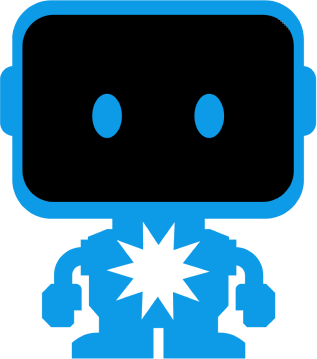
DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.
prediction environment
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as datarobot from "@datarobot/pulumi-datarobot";
const example = new datarobot.PredictionEnvironment("example", {
batchJobsMaxConcurrent: 20,
batchJobsPriority: "high",
credentialId: "<credential_id>",
datastoreId: "<datastore_id>",
description: "Description for the example prediction environment",
managedBy: "selfManaged",
platform: "datarobotServerless",
supportedModelFormats: [
"datarobot",
"customModel",
],
});
import pulumi
import pulumi_datarobot as datarobot
example = datarobot.PredictionEnvironment("example",
batch_jobs_max_concurrent=20,
batch_jobs_priority="high",
credential_id="<credential_id>",
datastore_id="<datastore_id>",
description="Description for the example prediction environment",
managed_by="selfManaged",
platform="datarobotServerless",
supported_model_formats=[
"datarobot",
"customModel",
])
package main
import (
"github.com/datarobot-community/pulumi-datarobot/sdk/go/datarobot"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := datarobot.NewPredictionEnvironment(ctx, "example", &datarobot.PredictionEnvironmentArgs{
BatchJobsMaxConcurrent: pulumi.Int(20),
BatchJobsPriority: pulumi.String("high"),
CredentialId: pulumi.String("<credential_id>"),
DatastoreId: pulumi.String("<datastore_id>"),
Description: pulumi.String("Description for the example prediction environment"),
ManagedBy: pulumi.String("selfManaged"),
Platform: pulumi.String("datarobotServerless"),
SupportedModelFormats: pulumi.StringArray{
pulumi.String("datarobot"),
pulumi.String("customModel"),
},
})
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Datarobot = DataRobotPulumi.Datarobot;
return await Deployment.RunAsync(() =>
{
var example = new Datarobot.PredictionEnvironment("example", new()
{
BatchJobsMaxConcurrent = 20,
BatchJobsPriority = "high",
CredentialId = "<credential_id>",
DatastoreId = "<datastore_id>",
Description = "Description for the example prediction environment",
ManagedBy = "selfManaged",
Platform = "datarobotServerless",
SupportedModelFormats = new[]
{
"datarobot",
"customModel",
},
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.datarobot.PredictionEnvironment;
import com.pulumi.datarobot.PredictionEnvironmentArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var example = new PredictionEnvironment("example", PredictionEnvironmentArgs.builder()
.batchJobsMaxConcurrent(20)
.batchJobsPriority("high")
.credentialId("<credential_id>")
.datastoreId("<datastore_id>")
.description("Description for the example prediction environment")
.managedBy("selfManaged")
.platform("datarobotServerless")
.supportedModelFormats(
"datarobot",
"customModel")
.build());
}
}
resources:
example:
type: datarobot:PredictionEnvironment
properties:
# Optional
batchJobsMaxConcurrent: 20
batchJobsPriority: high
credentialId: <credential_id>
datastoreId: <datastore_id>
description: Description for the example prediction environment
managedBy: selfManaged
platform: datarobotServerless
supportedModelFormats:
- datarobot
- customModel
Create PredictionEnvironment Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new PredictionEnvironment(name: string, args: PredictionEnvironmentArgs, opts?: CustomResourceOptions);
@overload
def PredictionEnvironment(resource_name: str,
args: PredictionEnvironmentArgs,
opts: Optional[ResourceOptions] = None)
@overload
def PredictionEnvironment(resource_name: str,
opts: Optional[ResourceOptions] = None,
platform: Optional[str] = None,
batch_jobs_max_concurrent: Optional[int] = None,
batch_jobs_priority: Optional[str] = None,
credential_id: Optional[str] = None,
datastore_id: Optional[str] = None,
description: Optional[str] = None,
managed_by: Optional[str] = None,
name: Optional[str] = None,
supported_model_formats: Optional[Sequence[str]] = None)
func NewPredictionEnvironment(ctx *Context, name string, args PredictionEnvironmentArgs, opts ...ResourceOption) (*PredictionEnvironment, error)
public PredictionEnvironment(string name, PredictionEnvironmentArgs args, CustomResourceOptions? opts = null)
public PredictionEnvironment(String name, PredictionEnvironmentArgs args)
public PredictionEnvironment(String name, PredictionEnvironmentArgs args, CustomResourceOptions options)
type: datarobot:PredictionEnvironment
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args PredictionEnvironmentArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args PredictionEnvironmentArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args PredictionEnvironmentArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args PredictionEnvironmentArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args PredictionEnvironmentArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var predictionEnvironmentResource = new Datarobot.PredictionEnvironment("predictionEnvironmentResource", new()
{
Platform = "string",
BatchJobsMaxConcurrent = 0,
BatchJobsPriority = "string",
CredentialId = "string",
DatastoreId = "string",
Description = "string",
ManagedBy = "string",
Name = "string",
SupportedModelFormats = new[]
{
"string",
},
});
example, err := datarobot.NewPredictionEnvironment(ctx, "predictionEnvironmentResource", &datarobot.PredictionEnvironmentArgs{
Platform: pulumi.String("string"),
BatchJobsMaxConcurrent: pulumi.Int(0),
BatchJobsPriority: pulumi.String("string"),
CredentialId: pulumi.String("string"),
DatastoreId: pulumi.String("string"),
Description: pulumi.String("string"),
ManagedBy: pulumi.String("string"),
Name: pulumi.String("string"),
SupportedModelFormats: pulumi.StringArray{
pulumi.String("string"),
},
})
var predictionEnvironmentResource = new PredictionEnvironment("predictionEnvironmentResource", PredictionEnvironmentArgs.builder()
.platform("string")
.batchJobsMaxConcurrent(0)
.batchJobsPriority("string")
.credentialId("string")
.datastoreId("string")
.description("string")
.managedBy("string")
.name("string")
.supportedModelFormats("string")
.build());
prediction_environment_resource = datarobot.PredictionEnvironment("predictionEnvironmentResource",
platform="string",
batch_jobs_max_concurrent=0,
batch_jobs_priority="string",
credential_id="string",
datastore_id="string",
description="string",
managed_by="string",
name="string",
supported_model_formats=["string"])
const predictionEnvironmentResource = new datarobot.PredictionEnvironment("predictionEnvironmentResource", {
platform: "string",
batchJobsMaxConcurrent: 0,
batchJobsPriority: "string",
credentialId: "string",
datastoreId: "string",
description: "string",
managedBy: "string",
name: "string",
supportedModelFormats: ["string"],
});
type: datarobot:PredictionEnvironment
properties:
batchJobsMaxConcurrent: 0
batchJobsPriority: string
credentialId: string
datastoreId: string
description: string
managedBy: string
name: string
platform: string
supportedModelFormats:
- string
PredictionEnvironment Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The PredictionEnvironment resource accepts the following input properties:
- Platform string
- The platform for the Prediction Environment.
- Batch
Jobs intMax Concurrent - The maximum number of concurrent batch prediction jobs.
- Batch
Jobs stringPriority - The importance of batch jobs.
- Credential
Id string - The ID of the credential associated with the data connection. Only applicable for external prediction environments managed by DataRobot.
- Datastore
Id string - The ID of the data store connection configuration. Only applicable for external prediction environments managed by DataRobot.
- Description string
- The description of the Prediction Environment.
- Managed
By string - Determines if the prediction environment should be managed by the management agent, datarobot, or self-managed. Self-managed by default.
- Name string
- The name of the Prediction Environment.
- Supported
Model List<string>Formats - The list of supported model formats.
- Platform string
- The platform for the Prediction Environment.
- Batch
Jobs intMax Concurrent - The maximum number of concurrent batch prediction jobs.
- Batch
Jobs stringPriority - The importance of batch jobs.
- Credential
Id string - The ID of the credential associated with the data connection. Only applicable for external prediction environments managed by DataRobot.
- Datastore
Id string - The ID of the data store connection configuration. Only applicable for external prediction environments managed by DataRobot.
- Description string
- The description of the Prediction Environment.
- Managed
By string - Determines if the prediction environment should be managed by the management agent, datarobot, or self-managed. Self-managed by default.
- Name string
- The name of the Prediction Environment.
- Supported
Model []stringFormats - The list of supported model formats.
- platform String
- The platform for the Prediction Environment.
- batch
Jobs IntegerMax Concurrent - The maximum number of concurrent batch prediction jobs.
- batch
Jobs StringPriority - The importance of batch jobs.
- credential
Id String - The ID of the credential associated with the data connection. Only applicable for external prediction environments managed by DataRobot.
- datastore
Id String - The ID of the data store connection configuration. Only applicable for external prediction environments managed by DataRobot.
- description String
- The description of the Prediction Environment.
- managed
By String - Determines if the prediction environment should be managed by the management agent, datarobot, or self-managed. Self-managed by default.
- name String
- The name of the Prediction Environment.
- supported
Model List<String>Formats - The list of supported model formats.
- platform string
- The platform for the Prediction Environment.
- batch
Jobs numberMax Concurrent - The maximum number of concurrent batch prediction jobs.
- batch
Jobs stringPriority - The importance of batch jobs.
- credential
Id string - The ID of the credential associated with the data connection. Only applicable for external prediction environments managed by DataRobot.
- datastore
Id string - The ID of the data store connection configuration. Only applicable for external prediction environments managed by DataRobot.
- description string
- The description of the Prediction Environment.
- managed
By string - Determines if the prediction environment should be managed by the management agent, datarobot, or self-managed. Self-managed by default.
- name string
- The name of the Prediction Environment.
- supported
Model string[]Formats - The list of supported model formats.
- platform str
- The platform for the Prediction Environment.
- batch_
jobs_ intmax_ concurrent - The maximum number of concurrent batch prediction jobs.
- batch_
jobs_ strpriority - The importance of batch jobs.
- credential_
id str - The ID of the credential associated with the data connection. Only applicable for external prediction environments managed by DataRobot.
- datastore_
id str - The ID of the data store connection configuration. Only applicable for external prediction environments managed by DataRobot.
- description str
- The description of the Prediction Environment.
- managed_
by str - Determines if the prediction environment should be managed by the management agent, datarobot, or self-managed. Self-managed by default.
- name str
- The name of the Prediction Environment.
- supported_
model_ Sequence[str]formats - The list of supported model formats.
- platform String
- The platform for the Prediction Environment.
- batch
Jobs NumberMax Concurrent - The maximum number of concurrent batch prediction jobs.
- batch
Jobs StringPriority - The importance of batch jobs.
- credential
Id String - The ID of the credential associated with the data connection. Only applicable for external prediction environments managed by DataRobot.
- datastore
Id String - The ID of the data store connection configuration. Only applicable for external prediction environments managed by DataRobot.
- description String
- The description of the Prediction Environment.
- managed
By String - Determines if the prediction environment should be managed by the management agent, datarobot, or self-managed. Self-managed by default.
- name String
- The name of the Prediction Environment.
- supported
Model List<String>Formats - The list of supported model formats.
Outputs
All input properties are implicitly available as output properties. Additionally, the PredictionEnvironment resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing PredictionEnvironment Resource
Get an existing PredictionEnvironment resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: PredictionEnvironmentState, opts?: CustomResourceOptions): PredictionEnvironment
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
batch_jobs_max_concurrent: Optional[int] = None,
batch_jobs_priority: Optional[str] = None,
credential_id: Optional[str] = None,
datastore_id: Optional[str] = None,
description: Optional[str] = None,
managed_by: Optional[str] = None,
name: Optional[str] = None,
platform: Optional[str] = None,
supported_model_formats: Optional[Sequence[str]] = None) -> PredictionEnvironment
func GetPredictionEnvironment(ctx *Context, name string, id IDInput, state *PredictionEnvironmentState, opts ...ResourceOption) (*PredictionEnvironment, error)
public static PredictionEnvironment Get(string name, Input<string> id, PredictionEnvironmentState? state, CustomResourceOptions? opts = null)
public static PredictionEnvironment get(String name, Output<String> id, PredictionEnvironmentState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Batch
Jobs intMax Concurrent - The maximum number of concurrent batch prediction jobs.
- Batch
Jobs stringPriority - The importance of batch jobs.
- Credential
Id string - The ID of the credential associated with the data connection. Only applicable for external prediction environments managed by DataRobot.
- Datastore
Id string - The ID of the data store connection configuration. Only applicable for external prediction environments managed by DataRobot.
- Description string
- The description of the Prediction Environment.
- Managed
By string - Determines if the prediction environment should be managed by the management agent, datarobot, or self-managed. Self-managed by default.
- Name string
- The name of the Prediction Environment.
- Platform string
- The platform for the Prediction Environment.
- Supported
Model List<string>Formats - The list of supported model formats.
- Batch
Jobs intMax Concurrent - The maximum number of concurrent batch prediction jobs.
- Batch
Jobs stringPriority - The importance of batch jobs.
- Credential
Id string - The ID of the credential associated with the data connection. Only applicable for external prediction environments managed by DataRobot.
- Datastore
Id string - The ID of the data store connection configuration. Only applicable for external prediction environments managed by DataRobot.
- Description string
- The description of the Prediction Environment.
- Managed
By string - Determines if the prediction environment should be managed by the management agent, datarobot, or self-managed. Self-managed by default.
- Name string
- The name of the Prediction Environment.
- Platform string
- The platform for the Prediction Environment.
- Supported
Model []stringFormats - The list of supported model formats.
- batch
Jobs IntegerMax Concurrent - The maximum number of concurrent batch prediction jobs.
- batch
Jobs StringPriority - The importance of batch jobs.
- credential
Id String - The ID of the credential associated with the data connection. Only applicable for external prediction environments managed by DataRobot.
- datastore
Id String - The ID of the data store connection configuration. Only applicable for external prediction environments managed by DataRobot.
- description String
- The description of the Prediction Environment.
- managed
By String - Determines if the prediction environment should be managed by the management agent, datarobot, or self-managed. Self-managed by default.
- name String
- The name of the Prediction Environment.
- platform String
- The platform for the Prediction Environment.
- supported
Model List<String>Formats - The list of supported model formats.
- batch
Jobs numberMax Concurrent - The maximum number of concurrent batch prediction jobs.
- batch
Jobs stringPriority - The importance of batch jobs.
- credential
Id string - The ID of the credential associated with the data connection. Only applicable for external prediction environments managed by DataRobot.
- datastore
Id string - The ID of the data store connection configuration. Only applicable for external prediction environments managed by DataRobot.
- description string
- The description of the Prediction Environment.
- managed
By string - Determines if the prediction environment should be managed by the management agent, datarobot, or self-managed. Self-managed by default.
- name string
- The name of the Prediction Environment.
- platform string
- The platform for the Prediction Environment.
- supported
Model string[]Formats - The list of supported model formats.
- batch_
jobs_ intmax_ concurrent - The maximum number of concurrent batch prediction jobs.
- batch_
jobs_ strpriority - The importance of batch jobs.
- credential_
id str - The ID of the credential associated with the data connection. Only applicable for external prediction environments managed by DataRobot.
- datastore_
id str - The ID of the data store connection configuration. Only applicable for external prediction environments managed by DataRobot.
- description str
- The description of the Prediction Environment.
- managed_
by str - Determines if the prediction environment should be managed by the management agent, datarobot, or self-managed. Self-managed by default.
- name str
- The name of the Prediction Environment.
- platform str
- The platform for the Prediction Environment.
- supported_
model_ Sequence[str]formats - The list of supported model formats.
- batch
Jobs NumberMax Concurrent - The maximum number of concurrent batch prediction jobs.
- batch
Jobs StringPriority - The importance of batch jobs.
- credential
Id String - The ID of the credential associated with the data connection. Only applicable for external prediction environments managed by DataRobot.
- datastore
Id String - The ID of the data store connection configuration. Only applicable for external prediction environments managed by DataRobot.
- description String
- The description of the Prediction Environment.
- managed
By String - Determines if the prediction environment should be managed by the management agent, datarobot, or self-managed. Self-managed by default.
- name String
- The name of the Prediction Environment.
- platform String
- The platform for the Prediction Environment.
- supported
Model List<String>Formats - The list of supported model formats.
Package Details
- Repository
- datarobot datarobot-community/pulumi-datarobot
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
datarobot
Terraform Provider.
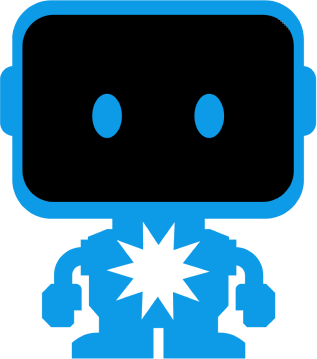
DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.