DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.
datarobot.QaApplication
Explore with Pulumi AI
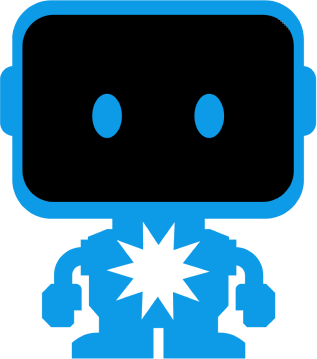
DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.
Q&A Application
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as datarobot from "@datarobot/pulumi-datarobot";
const exampleCustomModel = new datarobot.CustomModel("exampleCustomModel", {
description: "Description for the example custom model",
targetType: "Binary",
targetName: "my_label",
baseEnvironmentName: "[GenAI] Python 3.11 with Moderations",
files: ["example.py"],
});
const exampleRegisteredModel = new datarobot.RegisteredModel("exampleRegisteredModel", {
customModelVersionId: exampleCustomModel.versionId,
description: "Description for the example registered model",
});
const examplePredictionEnvironment = new datarobot.PredictionEnvironment("examplePredictionEnvironment", {
description: "Description for the example prediction environment",
platform: "datarobotServerless",
});
const exampleDeployment = new datarobot.Deployment("exampleDeployment", {
label: "An example deployment",
predictionEnvironmentId: examplePredictionEnvironment.id,
registeredModelVersionId: exampleRegisteredModel.versionId,
});
const exampleQaApplication = new datarobot.QaApplication("exampleQaApplication", {
deploymentId: exampleDeployment.id,
externalAccessEnabled: true,
externalAccessRecipients: ["recipient@example.com"],
});
export const datarobotQaApplicationId = exampleQaApplication.id;
export const datarobotQaApplicationSourceId = exampleQaApplication.sourceId;
export const datarobotQaApplicationSourceVersionId = exampleQaApplication.sourceVersionId;
export const datarobotQaApplicationUrl = exampleQaApplication.applicationUrl;
import pulumi
import pulumi_datarobot as datarobot
example_custom_model = datarobot.CustomModel("exampleCustomModel",
description="Description for the example custom model",
target_type="Binary",
target_name="my_label",
base_environment_name="[GenAI] Python 3.11 with Moderations",
files=["example.py"])
example_registered_model = datarobot.RegisteredModel("exampleRegisteredModel",
custom_model_version_id=example_custom_model.version_id,
description="Description for the example registered model")
example_prediction_environment = datarobot.PredictionEnvironment("examplePredictionEnvironment",
description="Description for the example prediction environment",
platform="datarobotServerless")
example_deployment = datarobot.Deployment("exampleDeployment",
label="An example deployment",
prediction_environment_id=example_prediction_environment.id,
registered_model_version_id=example_registered_model.version_id)
example_qa_application = datarobot.QaApplication("exampleQaApplication",
deployment_id=example_deployment.id,
external_access_enabled=True,
external_access_recipients=["recipient@example.com"])
pulumi.export("datarobotQaApplicationId", example_qa_application.id)
pulumi.export("datarobotQaApplicationSourceId", example_qa_application.source_id)
pulumi.export("datarobotQaApplicationSourceVersionId", example_qa_application.source_version_id)
pulumi.export("datarobotQaApplicationUrl", example_qa_application.application_url)
package main
import (
"github.com/datarobot-community/pulumi-datarobot/sdk/go/datarobot"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
exampleCustomModel, err := datarobot.NewCustomModel(ctx, "exampleCustomModel", &datarobot.CustomModelArgs{
Description: pulumi.String("Description for the example custom model"),
TargetType: pulumi.String("Binary"),
TargetName: pulumi.String("my_label"),
BaseEnvironmentName: pulumi.String("[GenAI] Python 3.11 with Moderations"),
Files: pulumi.Any{
"example.py",
},
})
if err != nil {
return err
}
exampleRegisteredModel, err := datarobot.NewRegisteredModel(ctx, "exampleRegisteredModel", &datarobot.RegisteredModelArgs{
CustomModelVersionId: exampleCustomModel.VersionId,
Description: pulumi.String("Description for the example registered model"),
})
if err != nil {
return err
}
examplePredictionEnvironment, err := datarobot.NewPredictionEnvironment(ctx, "examplePredictionEnvironment", &datarobot.PredictionEnvironmentArgs{
Description: pulumi.String("Description for the example prediction environment"),
Platform: pulumi.String("datarobotServerless"),
})
if err != nil {
return err
}
exampleDeployment, err := datarobot.NewDeployment(ctx, "exampleDeployment", &datarobot.DeploymentArgs{
Label: pulumi.String("An example deployment"),
PredictionEnvironmentId: examplePredictionEnvironment.ID(),
RegisteredModelVersionId: exampleRegisteredModel.VersionId,
})
if err != nil {
return err
}
exampleQaApplication, err := datarobot.NewQaApplication(ctx, "exampleQaApplication", &datarobot.QaApplicationArgs{
DeploymentId: exampleDeployment.ID(),
ExternalAccessEnabled: pulumi.Bool(true),
ExternalAccessRecipients: pulumi.StringArray{
pulumi.String("recipient@example.com"),
},
})
if err != nil {
return err
}
ctx.Export("datarobotQaApplicationId", exampleQaApplication.ID())
ctx.Export("datarobotQaApplicationSourceId", exampleQaApplication.SourceId)
ctx.Export("datarobotQaApplicationSourceVersionId", exampleQaApplication.SourceVersionId)
ctx.Export("datarobotQaApplicationUrl", exampleQaApplication.ApplicationUrl)
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Datarobot = DataRobotPulumi.Datarobot;
return await Deployment.RunAsync(() =>
{
var exampleCustomModel = new Datarobot.CustomModel("exampleCustomModel", new()
{
Description = "Description for the example custom model",
TargetType = "Binary",
TargetName = "my_label",
BaseEnvironmentName = "[GenAI] Python 3.11 with Moderations",
Files = new[]
{
"example.py",
},
});
var exampleRegisteredModel = new Datarobot.RegisteredModel("exampleRegisteredModel", new()
{
CustomModelVersionId = exampleCustomModel.VersionId,
Description = "Description for the example registered model",
});
var examplePredictionEnvironment = new Datarobot.PredictionEnvironment("examplePredictionEnvironment", new()
{
Description = "Description for the example prediction environment",
Platform = "datarobotServerless",
});
var exampleDeployment = new Datarobot.Deployment("exampleDeployment", new()
{
Label = "An example deployment",
PredictionEnvironmentId = examplePredictionEnvironment.Id,
RegisteredModelVersionId = exampleRegisteredModel.VersionId,
});
var exampleQaApplication = new Datarobot.QaApplication("exampleQaApplication", new()
{
DeploymentId = exampleDeployment.Id,
ExternalAccessEnabled = true,
ExternalAccessRecipients = new[]
{
"recipient@example.com",
},
});
return new Dictionary<string, object?>
{
["datarobotQaApplicationId"] = exampleQaApplication.Id,
["datarobotQaApplicationSourceId"] = exampleQaApplication.SourceId,
["datarobotQaApplicationSourceVersionId"] = exampleQaApplication.SourceVersionId,
["datarobotQaApplicationUrl"] = exampleQaApplication.ApplicationUrl,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.datarobot.CustomModel;
import com.pulumi.datarobot.CustomModelArgs;
import com.pulumi.datarobot.RegisteredModel;
import com.pulumi.datarobot.RegisteredModelArgs;
import com.pulumi.datarobot.PredictionEnvironment;
import com.pulumi.datarobot.PredictionEnvironmentArgs;
import com.pulumi.datarobot.Deployment;
import com.pulumi.datarobot.DeploymentArgs;
import com.pulumi.datarobot.QaApplication;
import com.pulumi.datarobot.QaApplicationArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var exampleCustomModel = new CustomModel("exampleCustomModel", CustomModelArgs.builder()
.description("Description for the example custom model")
.targetType("Binary")
.targetName("my_label")
.baseEnvironmentName("[GenAI] Python 3.11 with Moderations")
.files("example.py")
.build());
var exampleRegisteredModel = new RegisteredModel("exampleRegisteredModel", RegisteredModelArgs.builder()
.customModelVersionId(exampleCustomModel.versionId())
.description("Description for the example registered model")
.build());
var examplePredictionEnvironment = new PredictionEnvironment("examplePredictionEnvironment", PredictionEnvironmentArgs.builder()
.description("Description for the example prediction environment")
.platform("datarobotServerless")
.build());
var exampleDeployment = new Deployment("exampleDeployment", DeploymentArgs.builder()
.label("An example deployment")
.predictionEnvironmentId(examplePredictionEnvironment.id())
.registeredModelVersionId(exampleRegisteredModel.versionId())
.build());
var exampleQaApplication = new QaApplication("exampleQaApplication", QaApplicationArgs.builder()
.deploymentId(exampleDeployment.id())
.externalAccessEnabled(true)
.externalAccessRecipients("recipient@example.com")
.build());
ctx.export("datarobotQaApplicationId", exampleQaApplication.id());
ctx.export("datarobotQaApplicationSourceId", exampleQaApplication.sourceId());
ctx.export("datarobotQaApplicationSourceVersionId", exampleQaApplication.sourceVersionId());
ctx.export("datarobotQaApplicationUrl", exampleQaApplication.applicationUrl());
}
}
resources:
exampleCustomModel:
type: datarobot:CustomModel
properties:
description: Description for the example custom model
targetType: Binary
targetName: my_label
baseEnvironmentName: '[GenAI] Python 3.11 with Moderations'
files:
- example.py
exampleRegisteredModel:
type: datarobot:RegisteredModel
properties:
customModelVersionId: ${exampleCustomModel.versionId}
description: Description for the example registered model
examplePredictionEnvironment:
type: datarobot:PredictionEnvironment
properties:
description: Description for the example prediction environment
platform: datarobotServerless
exampleDeployment:
type: datarobot:Deployment
properties:
label: An example deployment
predictionEnvironmentId: ${examplePredictionEnvironment.id}
registeredModelVersionId: ${exampleRegisteredModel.versionId}
exampleQaApplication:
type: datarobot:QaApplication
properties:
deploymentId: ${exampleDeployment.id}
externalAccessEnabled: true
externalAccessRecipients:
- recipient@example.com
outputs:
datarobotQaApplicationId: ${exampleQaApplication.id}
datarobotQaApplicationSourceId: ${exampleQaApplication.sourceId}
datarobotQaApplicationSourceVersionId: ${exampleQaApplication.sourceVersionId}
datarobotQaApplicationUrl: ${exampleQaApplication.applicationUrl}
Create QaApplication Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new QaApplication(name: string, args: QaApplicationArgs, opts?: CustomResourceOptions);
@overload
def QaApplication(resource_name: str,
args: QaApplicationArgs,
opts: Optional[ResourceOptions] = None)
@overload
def QaApplication(resource_name: str,
opts: Optional[ResourceOptions] = None,
deployment_id: Optional[str] = None,
external_access_enabled: Optional[bool] = None,
external_access_recipients: Optional[Sequence[str]] = None,
name: Optional[str] = None)
func NewQaApplication(ctx *Context, name string, args QaApplicationArgs, opts ...ResourceOption) (*QaApplication, error)
public QaApplication(string name, QaApplicationArgs args, CustomResourceOptions? opts = null)
public QaApplication(String name, QaApplicationArgs args)
public QaApplication(String name, QaApplicationArgs args, CustomResourceOptions options)
type: datarobot:QaApplication
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args QaApplicationArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args QaApplicationArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args QaApplicationArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args QaApplicationArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args QaApplicationArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var qaApplicationResource = new Datarobot.QaApplication("qaApplicationResource", new()
{
DeploymentId = "string",
ExternalAccessEnabled = false,
ExternalAccessRecipients = new[]
{
"string",
},
Name = "string",
});
example, err := datarobot.NewQaApplication(ctx, "qaApplicationResource", &datarobot.QaApplicationArgs{
DeploymentId: pulumi.String("string"),
ExternalAccessEnabled: pulumi.Bool(false),
ExternalAccessRecipients: pulumi.StringArray{
pulumi.String("string"),
},
Name: pulumi.String("string"),
})
var qaApplicationResource = new QaApplication("qaApplicationResource", QaApplicationArgs.builder()
.deploymentId("string")
.externalAccessEnabled(false)
.externalAccessRecipients("string")
.name("string")
.build());
qa_application_resource = datarobot.QaApplication("qaApplicationResource",
deployment_id="string",
external_access_enabled=False,
external_access_recipients=["string"],
name="string")
const qaApplicationResource = new datarobot.QaApplication("qaApplicationResource", {
deploymentId: "string",
externalAccessEnabled: false,
externalAccessRecipients: ["string"],
name: "string",
});
type: datarobot:QaApplication
properties:
deploymentId: string
externalAccessEnabled: false
externalAccessRecipients:
- string
name: string
QaApplication Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The QaApplication resource accepts the following input properties:
- Deployment
Id string - The deployment ID of the Q&A Application.
- External
Access boolEnabled - Whether external access is enabled for the Q&A Application.
- External
Access List<string>Recipients - The list of external email addresses that have access to the Q&A Application.
- Name string
- The name of the Q&A Application.
- Deployment
Id string - The deployment ID of the Q&A Application.
- External
Access boolEnabled - Whether external access is enabled for the Q&A Application.
- External
Access []stringRecipients - The list of external email addresses that have access to the Q&A Application.
- Name string
- The name of the Q&A Application.
- deployment
Id String - The deployment ID of the Q&A Application.
- external
Access BooleanEnabled - Whether external access is enabled for the Q&A Application.
- external
Access List<String>Recipients - The list of external email addresses that have access to the Q&A Application.
- name String
- The name of the Q&A Application.
- deployment
Id string - The deployment ID of the Q&A Application.
- external
Access booleanEnabled - Whether external access is enabled for the Q&A Application.
- external
Access string[]Recipients - The list of external email addresses that have access to the Q&A Application.
- name string
- The name of the Q&A Application.
- deployment_
id str - The deployment ID of the Q&A Application.
- external_
access_ boolenabled - Whether external access is enabled for the Q&A Application.
- external_
access_ Sequence[str]recipients - The list of external email addresses that have access to the Q&A Application.
- name str
- The name of the Q&A Application.
- deployment
Id String - The deployment ID of the Q&A Application.
- external
Access BooleanEnabled - Whether external access is enabled for the Q&A Application.
- external
Access List<String>Recipients - The list of external email addresses that have access to the Q&A Application.
- name String
- The name of the Q&A Application.
Outputs
All input properties are implicitly available as output properties. Additionally, the QaApplication resource produces the following output properties:
- Application
Url string - The URL of the Q&A Application.
- Id string
- The provider-assigned unique ID for this managed resource.
- Source
Id string - The ID of the Q&A Application Source.
- Source
Version stringId - The version ID of the Q&A Application Source.
- Application
Url string - The URL of the Q&A Application.
- Id string
- The provider-assigned unique ID for this managed resource.
- Source
Id string - The ID of the Q&A Application Source.
- Source
Version stringId - The version ID of the Q&A Application Source.
- application
Url String - The URL of the Q&A Application.
- id String
- The provider-assigned unique ID for this managed resource.
- source
Id String - The ID of the Q&A Application Source.
- source
Version StringId - The version ID of the Q&A Application Source.
- application
Url string - The URL of the Q&A Application.
- id string
- The provider-assigned unique ID for this managed resource.
- source
Id string - The ID of the Q&A Application Source.
- source
Version stringId - The version ID of the Q&A Application Source.
- application_
url str - The URL of the Q&A Application.
- id str
- The provider-assigned unique ID for this managed resource.
- source_
id str - The ID of the Q&A Application Source.
- source_
version_ strid - The version ID of the Q&A Application Source.
- application
Url String - The URL of the Q&A Application.
- id String
- The provider-assigned unique ID for this managed resource.
- source
Id String - The ID of the Q&A Application Source.
- source
Version StringId - The version ID of the Q&A Application Source.
Look up Existing QaApplication Resource
Get an existing QaApplication resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: QaApplicationState, opts?: CustomResourceOptions): QaApplication
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
application_url: Optional[str] = None,
deployment_id: Optional[str] = None,
external_access_enabled: Optional[bool] = None,
external_access_recipients: Optional[Sequence[str]] = None,
name: Optional[str] = None,
source_id: Optional[str] = None,
source_version_id: Optional[str] = None) -> QaApplication
func GetQaApplication(ctx *Context, name string, id IDInput, state *QaApplicationState, opts ...ResourceOption) (*QaApplication, error)
public static QaApplication Get(string name, Input<string> id, QaApplicationState? state, CustomResourceOptions? opts = null)
public static QaApplication get(String name, Output<String> id, QaApplicationState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Application
Url string - The URL of the Q&A Application.
- Deployment
Id string - The deployment ID of the Q&A Application.
- External
Access boolEnabled - Whether external access is enabled for the Q&A Application.
- External
Access List<string>Recipients - The list of external email addresses that have access to the Q&A Application.
- Name string
- The name of the Q&A Application.
- Source
Id string - The ID of the Q&A Application Source.
- Source
Version stringId - The version ID of the Q&A Application Source.
- Application
Url string - The URL of the Q&A Application.
- Deployment
Id string - The deployment ID of the Q&A Application.
- External
Access boolEnabled - Whether external access is enabled for the Q&A Application.
- External
Access []stringRecipients - The list of external email addresses that have access to the Q&A Application.
- Name string
- The name of the Q&A Application.
- Source
Id string - The ID of the Q&A Application Source.
- Source
Version stringId - The version ID of the Q&A Application Source.
- application
Url String - The URL of the Q&A Application.
- deployment
Id String - The deployment ID of the Q&A Application.
- external
Access BooleanEnabled - Whether external access is enabled for the Q&A Application.
- external
Access List<String>Recipients - The list of external email addresses that have access to the Q&A Application.
- name String
- The name of the Q&A Application.
- source
Id String - The ID of the Q&A Application Source.
- source
Version StringId - The version ID of the Q&A Application Source.
- application
Url string - The URL of the Q&A Application.
- deployment
Id string - The deployment ID of the Q&A Application.
- external
Access booleanEnabled - Whether external access is enabled for the Q&A Application.
- external
Access string[]Recipients - The list of external email addresses that have access to the Q&A Application.
- name string
- The name of the Q&A Application.
- source
Id string - The ID of the Q&A Application Source.
- source
Version stringId - The version ID of the Q&A Application Source.
- application_
url str - The URL of the Q&A Application.
- deployment_
id str - The deployment ID of the Q&A Application.
- external_
access_ boolenabled - Whether external access is enabled for the Q&A Application.
- external_
access_ Sequence[str]recipients - The list of external email addresses that have access to the Q&A Application.
- name str
- The name of the Q&A Application.
- source_
id str - The ID of the Q&A Application Source.
- source_
version_ strid - The version ID of the Q&A Application Source.
- application
Url String - The URL of the Q&A Application.
- deployment
Id String - The deployment ID of the Q&A Application.
- external
Access BooleanEnabled - Whether external access is enabled for the Q&A Application.
- external
Access List<String>Recipients - The list of external email addresses that have access to the Q&A Application.
- name String
- The name of the Q&A Application.
- source
Id String - The ID of the Q&A Application Source.
- source
Version StringId - The version ID of the Q&A Application Source.
Package Details
- Repository
- datarobot datarobot-community/pulumi-datarobot
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
datarobot
Terraform Provider.
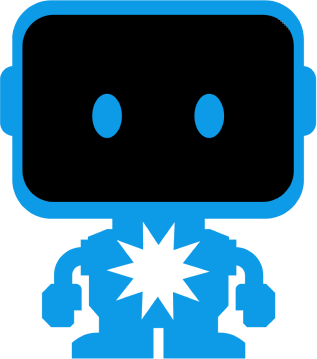
DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.