DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.
datarobot.VectorDatabase
Explore with Pulumi AI
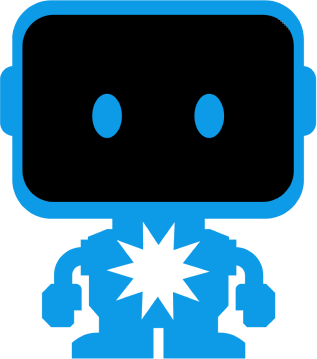
DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.
Vector database
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as datarobot from "@datarobot/pulumi-datarobot";
const exampleUseCase = new datarobot.UseCase("exampleUseCase", {description: "Description for the example use case"});
const exampleDatasetFromFile = new datarobot.DatasetFromFile("exampleDatasetFromFile", {
filePath: "[Path to file to upload]",
useCaseIds: [exampleUseCase.id],
});
const exampleVectorDatabase = new datarobot.VectorDatabase("exampleVectorDatabase", {
useCaseId: exampleUseCase.id,
datasetId: exampleDatasetFromFile.id,
});
// Optional
// chunking_parameters = {
// chunk_overlap_percentage = 0
// chunk_size = 512
// chunking_method = "recursive"
// embedding_model = "jinaai/jina-embedding-t-en-v1"
// separators = ["\n", " "]
// }
export const exampleId = exampleVectorDatabase.id;
import pulumi
import pulumi_datarobot as datarobot
example_use_case = datarobot.UseCase("exampleUseCase", description="Description for the example use case")
example_dataset_from_file = datarobot.DatasetFromFile("exampleDatasetFromFile",
file_path="[Path to file to upload]",
use_case_ids=[example_use_case.id])
example_vector_database = datarobot.VectorDatabase("exampleVectorDatabase",
use_case_id=example_use_case.id,
dataset_id=example_dataset_from_file.id)
# Optional
# chunking_parameters = {
# chunk_overlap_percentage = 0
# chunk_size = 512
# chunking_method = "recursive"
# embedding_model = "jinaai/jina-embedding-t-en-v1"
# separators = ["\n", " "]
# }
pulumi.export("exampleId", example_vector_database.id)
package main
import (
"github.com/datarobot-community/pulumi-datarobot/sdk/go/datarobot"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
exampleUseCase, err := datarobot.NewUseCase(ctx, "exampleUseCase", &datarobot.UseCaseArgs{
Description: pulumi.String("Description for the example use case"),
})
if err != nil {
return err
}
exampleDatasetFromFile, err := datarobot.NewDatasetFromFile(ctx, "exampleDatasetFromFile", &datarobot.DatasetFromFileArgs{
FilePath: pulumi.String("[Path to file to upload]"),
UseCaseIds: pulumi.StringArray{
exampleUseCase.ID(),
},
})
if err != nil {
return err
}
exampleVectorDatabase, err := datarobot.NewVectorDatabase(ctx, "exampleVectorDatabase", &datarobot.VectorDatabaseArgs{
UseCaseId: exampleUseCase.ID(),
DatasetId: exampleDatasetFromFile.ID(),
})
if err != nil {
return err
}
ctx.Export("exampleId", exampleVectorDatabase.ID())
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Datarobot = DataRobotPulumi.Datarobot;
return await Deployment.RunAsync(() =>
{
var exampleUseCase = new Datarobot.UseCase("exampleUseCase", new()
{
Description = "Description for the example use case",
});
var exampleDatasetFromFile = new Datarobot.DatasetFromFile("exampleDatasetFromFile", new()
{
FilePath = "[Path to file to upload]",
UseCaseIds = new[]
{
exampleUseCase.Id,
},
});
var exampleVectorDatabase = new Datarobot.VectorDatabase("exampleVectorDatabase", new()
{
UseCaseId = exampleUseCase.Id,
DatasetId = exampleDatasetFromFile.Id,
});
// Optional
// chunking_parameters = {
// chunk_overlap_percentage = 0
// chunk_size = 512
// chunking_method = "recursive"
// embedding_model = "jinaai/jina-embedding-t-en-v1"
// separators = ["\n", " "]
// }
return new Dictionary<string, object?>
{
["exampleId"] = exampleVectorDatabase.Id,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.datarobot.UseCase;
import com.pulumi.datarobot.UseCaseArgs;
import com.pulumi.datarobot.DatasetFromFile;
import com.pulumi.datarobot.DatasetFromFileArgs;
import com.pulumi.datarobot.VectorDatabase;
import com.pulumi.datarobot.VectorDatabaseArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var exampleUseCase = new UseCase("exampleUseCase", UseCaseArgs.builder()
.description("Description for the example use case")
.build());
var exampleDatasetFromFile = new DatasetFromFile("exampleDatasetFromFile", DatasetFromFileArgs.builder()
.filePath("[Path to file to upload]")
.useCaseIds(exampleUseCase.id())
.build());
var exampleVectorDatabase = new VectorDatabase("exampleVectorDatabase", VectorDatabaseArgs.builder()
.useCaseId(exampleUseCase.id())
.datasetId(exampleDatasetFromFile.id())
.build());
// Optional
// chunking_parameters = {
// chunk_overlap_percentage = 0
// chunk_size = 512
// chunking_method = "recursive"
// embedding_model = "jinaai/jina-embedding-t-en-v1"
// separators = ["\n", " "]
// }
ctx.export("exampleId", exampleVectorDatabase.id());
}
}
resources:
exampleUseCase:
type: datarobot:UseCase
properties:
description: Description for the example use case
exampleDatasetFromFile:
type: datarobot:DatasetFromFile
properties:
filePath: '[Path to file to upload]'
useCaseIds:
- ${exampleUseCase.id}
exampleVectorDatabase:
type: datarobot:VectorDatabase
properties:
useCaseId: ${exampleUseCase.id}
datasetId: ${exampleDatasetFromFile.id}
outputs:
exampleId: ${exampleVectorDatabase.id}
Create VectorDatabase Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new VectorDatabase(name: string, args: VectorDatabaseArgs, opts?: CustomResourceOptions);
@overload
def VectorDatabase(resource_name: str,
args: VectorDatabaseArgs,
opts: Optional[ResourceOptions] = None)
@overload
def VectorDatabase(resource_name: str,
opts: Optional[ResourceOptions] = None,
dataset_id: Optional[str] = None,
use_case_id: Optional[str] = None,
chunking_parameters: Optional[VectorDatabaseChunkingParametersArgs] = None,
name: Optional[str] = None)
func NewVectorDatabase(ctx *Context, name string, args VectorDatabaseArgs, opts ...ResourceOption) (*VectorDatabase, error)
public VectorDatabase(string name, VectorDatabaseArgs args, CustomResourceOptions? opts = null)
public VectorDatabase(String name, VectorDatabaseArgs args)
public VectorDatabase(String name, VectorDatabaseArgs args, CustomResourceOptions options)
type: datarobot:VectorDatabase
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args VectorDatabaseArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args VectorDatabaseArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args VectorDatabaseArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args VectorDatabaseArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args VectorDatabaseArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var vectorDatabaseResource = new Datarobot.VectorDatabase("vectorDatabaseResource", new()
{
DatasetId = "string",
UseCaseId = "string",
ChunkingParameters = new Datarobot.Inputs.VectorDatabaseChunkingParametersArgs
{
ChunkOverlapPercentage = 0,
ChunkSize = 0,
ChunkingMethod = "string",
EmbeddingModel = "string",
IsSeparatorRegex = false,
Separators = new[]
{
"string",
},
},
Name = "string",
});
example, err := datarobot.NewVectorDatabase(ctx, "vectorDatabaseResource", &datarobot.VectorDatabaseArgs{
DatasetId: pulumi.String("string"),
UseCaseId: pulumi.String("string"),
ChunkingParameters: &datarobot.VectorDatabaseChunkingParametersArgs{
ChunkOverlapPercentage: pulumi.Int(0),
ChunkSize: pulumi.Int(0),
ChunkingMethod: pulumi.String("string"),
EmbeddingModel: pulumi.String("string"),
IsSeparatorRegex: pulumi.Bool(false),
Separators: pulumi.StringArray{
pulumi.String("string"),
},
},
Name: pulumi.String("string"),
})
var vectorDatabaseResource = new VectorDatabase("vectorDatabaseResource", VectorDatabaseArgs.builder()
.datasetId("string")
.useCaseId("string")
.chunkingParameters(VectorDatabaseChunkingParametersArgs.builder()
.chunkOverlapPercentage(0)
.chunkSize(0)
.chunkingMethod("string")
.embeddingModel("string")
.isSeparatorRegex(false)
.separators("string")
.build())
.name("string")
.build());
vector_database_resource = datarobot.VectorDatabase("vectorDatabaseResource",
dataset_id="string",
use_case_id="string",
chunking_parameters=datarobot.VectorDatabaseChunkingParametersArgs(
chunk_overlap_percentage=0,
chunk_size=0,
chunking_method="string",
embedding_model="string",
is_separator_regex=False,
separators=["string"],
),
name="string")
const vectorDatabaseResource = new datarobot.VectorDatabase("vectorDatabaseResource", {
datasetId: "string",
useCaseId: "string",
chunkingParameters: {
chunkOverlapPercentage: 0,
chunkSize: 0,
chunkingMethod: "string",
embeddingModel: "string",
isSeparatorRegex: false,
separators: ["string"],
},
name: "string",
});
type: datarobot:VectorDatabase
properties:
chunkingParameters:
chunkOverlapPercentage: 0
chunkSize: 0
chunkingMethod: string
embeddingModel: string
isSeparatorRegex: false
separators:
- string
datasetId: string
name: string
useCaseId: string
VectorDatabase Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The VectorDatabase resource accepts the following input properties:
- Dataset
Id string - The id of the Vector Database.
- Use
Case stringId - The id of the Use Case.
- Chunking
Parameters DataRobot Vector Database Chunking Parameters - The chunking parameters for the Model.
- Name string
- The name of the VectorDatabase.
- Dataset
Id string - The id of the Vector Database.
- Use
Case stringId - The id of the Use Case.
- Chunking
Parameters VectorDatabase Chunking Parameters Args - The chunking parameters for the Model.
- Name string
- The name of the VectorDatabase.
- dataset
Id String - The id of the Vector Database.
- use
Case StringId - The id of the Use Case.
- chunking
Parameters VectorDatabase Chunking Parameters - The chunking parameters for the Model.
- name String
- The name of the VectorDatabase.
- dataset
Id string - The id of the Vector Database.
- use
Case stringId - The id of the Use Case.
- chunking
Parameters VectorDatabase Chunking Parameters - The chunking parameters for the Model.
- name string
- The name of the VectorDatabase.
- dataset_
id str - The id of the Vector Database.
- use_
case_ strid - The id of the Use Case.
- chunking_
parameters VectorDatabase Chunking Parameters Args - The chunking parameters for the Model.
- name str
- The name of the VectorDatabase.
- dataset
Id String - The id of the Vector Database.
- use
Case StringId - The id of the Use Case.
- chunking
Parameters Property Map - The chunking parameters for the Model.
- name String
- The name of the VectorDatabase.
Outputs
All input properties are implicitly available as output properties. Additionally, the VectorDatabase resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing VectorDatabase Resource
Get an existing VectorDatabase resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: VectorDatabaseState, opts?: CustomResourceOptions): VectorDatabase
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
chunking_parameters: Optional[VectorDatabaseChunkingParametersArgs] = None,
dataset_id: Optional[str] = None,
name: Optional[str] = None,
use_case_id: Optional[str] = None) -> VectorDatabase
func GetVectorDatabase(ctx *Context, name string, id IDInput, state *VectorDatabaseState, opts ...ResourceOption) (*VectorDatabase, error)
public static VectorDatabase Get(string name, Input<string> id, VectorDatabaseState? state, CustomResourceOptions? opts = null)
public static VectorDatabase get(String name, Output<String> id, VectorDatabaseState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Chunking
Parameters DataRobot Vector Database Chunking Parameters - The chunking parameters for the Model.
- Dataset
Id string - The id of the Vector Database.
- Name string
- The name of the VectorDatabase.
- Use
Case stringId - The id of the Use Case.
- Chunking
Parameters VectorDatabase Chunking Parameters Args - The chunking parameters for the Model.
- Dataset
Id string - The id of the Vector Database.
- Name string
- The name of the VectorDatabase.
- Use
Case stringId - The id of the Use Case.
- chunking
Parameters VectorDatabase Chunking Parameters - The chunking parameters for the Model.
- dataset
Id String - The id of the Vector Database.
- name String
- The name of the VectorDatabase.
- use
Case StringId - The id of the Use Case.
- chunking
Parameters VectorDatabase Chunking Parameters - The chunking parameters for the Model.
- dataset
Id string - The id of the Vector Database.
- name string
- The name of the VectorDatabase.
- use
Case stringId - The id of the Use Case.
- chunking_
parameters VectorDatabase Chunking Parameters Args - The chunking parameters for the Model.
- dataset_
id str - The id of the Vector Database.
- name str
- The name of the VectorDatabase.
- use_
case_ strid - The id of the Use Case.
- chunking
Parameters Property Map - The chunking parameters for the Model.
- dataset
Id String - The id of the Vector Database.
- name String
- The name of the VectorDatabase.
- use
Case StringId - The id of the Use Case.
Supporting Types
VectorDatabaseChunkingParameters, VectorDatabaseChunkingParametersArgs
- Chunk
Overlap intPercentage - The percentage of overlap between chunks.
- Chunk
Size int - The size of the chunks.
- Chunking
Method string - The method used to chunk the data.
- Embedding
Model string - The id of the Embedding Model.
- Is
Separator boolRegex - Whether the separator is a regex.
- Separators List<string>
- The separators used to split the data.
- Chunk
Overlap intPercentage - The percentage of overlap between chunks.
- Chunk
Size int - The size of the chunks.
- Chunking
Method string - The method used to chunk the data.
- Embedding
Model string - The id of the Embedding Model.
- Is
Separator boolRegex - Whether the separator is a regex.
- Separators []string
- The separators used to split the data.
- chunk
Overlap IntegerPercentage - The percentage of overlap between chunks.
- chunk
Size Integer - The size of the chunks.
- chunking
Method String - The method used to chunk the data.
- embedding
Model String - The id of the Embedding Model.
- is
Separator BooleanRegex - Whether the separator is a regex.
- separators List<String>
- The separators used to split the data.
- chunk
Overlap numberPercentage - The percentage of overlap between chunks.
- chunk
Size number - The size of the chunks.
- chunking
Method string - The method used to chunk the data.
- embedding
Model string - The id of the Embedding Model.
- is
Separator booleanRegex - Whether the separator is a regex.
- separators string[]
- The separators used to split the data.
- chunk_
overlap_ intpercentage - The percentage of overlap between chunks.
- chunk_
size int - The size of the chunks.
- chunking_
method str - The method used to chunk the data.
- embedding_
model str - The id of the Embedding Model.
- is_
separator_ boolregex - Whether the separator is a regex.
- separators Sequence[str]
- The separators used to split the data.
- chunk
Overlap NumberPercentage - The percentage of overlap between chunks.
- chunk
Size Number - The size of the chunks.
- chunking
Method String - The method used to chunk the data.
- embedding
Model String - The id of the Embedding Model.
- is
Separator BooleanRegex - Whether the separator is a regex.
- separators List<String>
- The separators used to split the data.
Package Details
- Repository
- datarobot datarobot-community/pulumi-datarobot
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
datarobot
Terraform Provider.
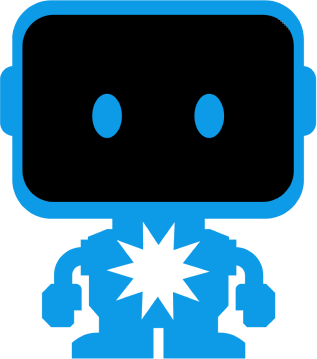
DataRobot v0.1.44 published on Monday, Sep 23, 2024 by DataRobot, Inc.