Microsoft SQL Server v0.0.8 published on Wednesday, Nov 1, 2023 by pulumiverse
mssql.getDatabaseRoles
Explore with Pulumi AI
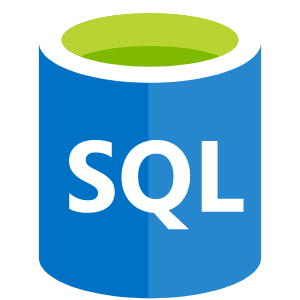
Microsoft SQL Server v0.0.8 published on Wednesday, Nov 1, 2023 by pulumiverse
Obtains information about all roles defined in a database.
Example Usage
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Mssql = Pulumi.Mssql;
return await Deployment.RunAsync(() =>
{
var master = Mssql.GetDatabase.Invoke(new()
{
Name = "master",
});
var example = Mssql.GetDatabaseRoles.Invoke(new()
{
DatabaseId = master.Apply(getDatabaseResult => getDatabaseResult.Id),
});
return new Dictionary<string, object?>
{
["roles"] = example.Apply(getDatabaseRolesResult => getDatabaseRolesResult.Roles),
};
});
package main
import (
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
"github.com/pulumiverse/pulumi-mssql/sdk/go/mssql"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
master, err := mssql.LookupDatabase(ctx, &mssql.LookupDatabaseArgs{
Name: "master",
}, nil)
if err != nil {
return err
}
example, err := mssql.GetDatabaseRoles(ctx, &mssql.GetDatabaseRolesArgs{
DatabaseId: pulumi.StringRef(master.Id),
}, nil)
if err != nil {
return err
}
ctx.Export("roles", example.Roles)
return nil
})
}
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.mssql.MssqlFunctions;
import com.pulumi.mssql.inputs.GetDatabaseArgs;
import com.pulumi.mssql.inputs.GetDatabaseRolesArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var master = MssqlFunctions.getDatabase(GetDatabaseArgs.builder()
.name("master")
.build());
final var example = MssqlFunctions.getDatabaseRoles(GetDatabaseRolesArgs.builder()
.databaseId(master.applyValue(getDatabaseResult -> getDatabaseResult.id()))
.build());
ctx.export("roles", example.applyValue(getDatabaseRolesResult -> getDatabaseRolesResult.roles()));
}
}
import pulumi
import pulumi_mssql as mssql
master = mssql.get_database(name="master")
example = mssql.get_database_roles(database_id=master.id)
pulumi.export("roles", example.roles)
import * as pulumi from "@pulumi/pulumi";
import * as mssql from "@pulumi/mssql";
const master = mssql.getDatabase({
name: "master",
});
const example = master.then(master => mssql.getDatabaseRoles({
databaseId: master.id,
}));
export const roles = example.then(example => example.roles);
variables:
master:
fn::invoke:
Function: mssql:getDatabase
Arguments:
name: master
example:
fn::invoke:
Function: mssql:getDatabaseRoles
Arguments:
databaseId: ${master.id}
outputs:
roles: ${example.roles}
Using getDatabaseRoles
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getDatabaseRoles(args: GetDatabaseRolesArgs, opts?: InvokeOptions): Promise<GetDatabaseRolesResult>
function getDatabaseRolesOutput(args: GetDatabaseRolesOutputArgs, opts?: InvokeOptions): Output<GetDatabaseRolesResult>
def get_database_roles(database_id: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetDatabaseRolesResult
def get_database_roles_output(database_id: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetDatabaseRolesResult]
func GetDatabaseRoles(ctx *Context, args *GetDatabaseRolesArgs, opts ...InvokeOption) (*GetDatabaseRolesResult, error)
func GetDatabaseRolesOutput(ctx *Context, args *GetDatabaseRolesOutputArgs, opts ...InvokeOption) GetDatabaseRolesResultOutput
> Note: This function is named GetDatabaseRoles
in the Go SDK.
public static class GetDatabaseRoles
{
public static Task<GetDatabaseRolesResult> InvokeAsync(GetDatabaseRolesArgs args, InvokeOptions? opts = null)
public static Output<GetDatabaseRolesResult> Invoke(GetDatabaseRolesInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetDatabaseRolesResult> getDatabaseRoles(GetDatabaseRolesArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: mssql:index/getDatabaseRoles:getDatabaseRoles
arguments:
# arguments dictionary
The following arguments are supported:
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
.
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
.
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
.
- database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
.
- database_
id str - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
.
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
.
getDatabaseRoles Result
The following output properties are available:
- Id string
- ID of the resource, equals to database ID
- Roles
List<Pulumiverse.
Mssql. Outputs. Get Database Roles Role> - Set of database role objects
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. Defaults to ID ofmaster
.
- Id string
- ID of the resource, equals to database ID
- Roles
[]Get
Database Roles Role - Set of database role objects
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. Defaults to ID ofmaster
.
- id String
- ID of the resource, equals to database ID
- roles
List<Get
Database Roles Role> - Set of database role objects
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. Defaults to ID ofmaster
.
- id string
- ID of the resource, equals to database ID
- roles
Get
Database Roles Role[] - Set of database role objects
- database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. Defaults to ID ofmaster
.
- id str
- ID of the resource, equals to database ID
- roles
Sequence[Get
Database Roles Role] - Set of database role objects
- database_
id str - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. Defaults to ID ofmaster
.
- id String
- ID of the resource, equals to database ID
- roles List<Property Map>
- Set of database role objects
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. Defaults to ID ofmaster
.
Supporting Types
GetDatabaseRolesRole
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - Id string
<database_id>/<role_id>
. Role ID can be retrieved usingSELECT DATABASE_PRINCIPAL_ID('<role_name>')
- Name string
- Role name. Must follow Regular Identifiers rules and cannot be longer than 128 chars.
- Owner
Id string - ID of another database role or user owning this role. Can be retrieved using
mssql.DatabaseRole
ormssql.SqlUser
.
- Database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - Id string
<database_id>/<role_id>
. Role ID can be retrieved usingSELECT DATABASE_PRINCIPAL_ID('<role_name>')
- Name string
- Role name. Must follow Regular Identifiers rules and cannot be longer than 128 chars.
- Owner
Id string - ID of another database role or user owning this role. Can be retrieved using
mssql.DatabaseRole
ormssql.SqlUser
.
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id String
<database_id>/<role_id>
. Role ID can be retrieved usingSELECT DATABASE_PRINCIPAL_ID('<role_name>')
- name String
- Role name. Must follow Regular Identifiers rules and cannot be longer than 128 chars.
- owner
Id String - ID of another database role or user owning this role. Can be retrieved using
mssql.DatabaseRole
ormssql.SqlUser
.
- database
Id string - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id string
<database_id>/<role_id>
. Role ID can be retrieved usingSELECT DATABASE_PRINCIPAL_ID('<role_name>')
- name string
- Role name. Must follow Regular Identifiers rules and cannot be longer than 128 chars.
- owner
Id string - ID of another database role or user owning this role. Can be retrieved using
mssql.DatabaseRole
ormssql.SqlUser
.
- database_
id str - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id str
<database_id>/<role_id>
. Role ID can be retrieved usingSELECT DATABASE_PRINCIPAL_ID('<role_name>')
- name str
- Role name. Must follow Regular Identifiers rules and cannot be longer than 128 chars.
- owner_
id str - ID of another database role or user owning this role. Can be retrieved using
mssql.DatabaseRole
ormssql.SqlUser
.
- database
Id String - ID of database. Can be retrieved using
mssql.Database
orSELECT DB_ID('<db_name>')
. - id String
<database_id>/<role_id>
. Role ID can be retrieved usingSELECT DATABASE_PRINCIPAL_ID('<role_name>')
- name String
- Role name. Must follow Regular Identifiers rules and cannot be longer than 128 chars.
- owner
Id String - ID of another database role or user owning this role. Can be retrieved using
mssql.DatabaseRole
ormssql.SqlUser
.
Package Details
- Repository
- mssql pulumiverse/pulumi-mssql
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
mssql
Terraform Provider.
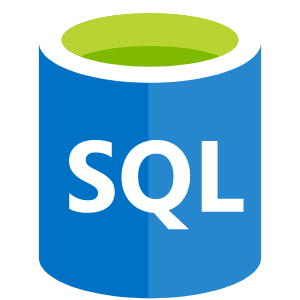
Microsoft SQL Server v0.0.8 published on Wednesday, Nov 1, 2023 by pulumiverse