Microsoft SQL Server v0.0.8 published on Wednesday, Nov 1, 2023 by pulumiverse
mssql.getSqlLogin
Explore with Pulumi AI
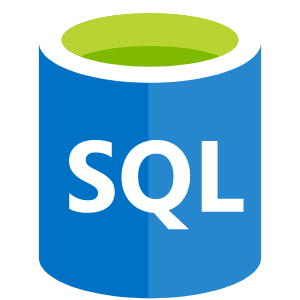
Microsoft SQL Server v0.0.8 published on Wednesday, Nov 1, 2023 by pulumiverse
Obtains information about single SQL login.
Example Usage
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Mssql = Pulumi.Mssql;
return await Deployment.RunAsync(() =>
{
var sa = Mssql.GetSqlLogin.Invoke(new()
{
Name = "sa",
});
return new Dictionary<string, object?>
{
["id"] = sa.Apply(getSqlLoginResult => getSqlLoginResult.Id),
["dbId"] = sa.Apply(getSqlLoginResult => getSqlLoginResult.DefaultDatabaseId),
};
});
package main
import (
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
"github.com/pulumiverse/pulumi-mssql/sdk/go/mssql"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
sa, err := mssql.LookupSqlLogin(ctx, &mssql.LookupSqlLoginArgs{
Name: "sa",
}, nil)
if err != nil {
return err
}
ctx.Export("id", sa.Id)
ctx.Export("dbId", sa.DefaultDatabaseId)
return nil
})
}
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.mssql.MssqlFunctions;
import com.pulumi.mssql.inputs.GetSqlLoginArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var sa = MssqlFunctions.getSqlLogin(GetSqlLoginArgs.builder()
.name("sa")
.build());
ctx.export("id", sa.applyValue(getSqlLoginResult -> getSqlLoginResult.id()));
ctx.export("dbId", sa.applyValue(getSqlLoginResult -> getSqlLoginResult.defaultDatabaseId()));
}
}
import pulumi
import pulumi_mssql as mssql
sa = mssql.get_sql_login(name="sa")
pulumi.export("id", sa.id)
pulumi.export("dbId", sa.default_database_id)
import * as pulumi from "@pulumi/pulumi";
import * as mssql from "@pulumi/mssql";
const sa = mssql.getSqlLogin({
name: "sa",
});
export const id = sa.then(sa => sa.id);
export const dbId = sa.then(sa => sa.defaultDatabaseId);
variables:
sa:
fn::invoke:
Function: mssql:getSqlLogin
Arguments:
name: sa
outputs:
id: ${sa.id}
dbId: ${sa.defaultDatabaseId}
Using getSqlLogin
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getSqlLogin(args: GetSqlLoginArgs, opts?: InvokeOptions): Promise<GetSqlLoginResult>
function getSqlLoginOutput(args: GetSqlLoginOutputArgs, opts?: InvokeOptions): Output<GetSqlLoginResult>
def get_sql_login(name: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetSqlLoginResult
def get_sql_login_output(name: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetSqlLoginResult]
func LookupSqlLogin(ctx *Context, args *LookupSqlLoginArgs, opts ...InvokeOption) (*LookupSqlLoginResult, error)
func LookupSqlLoginOutput(ctx *Context, args *LookupSqlLoginOutputArgs, opts ...InvokeOption) LookupSqlLoginResultOutput
> Note: This function is named LookupSqlLogin
in the Go SDK.
public static class GetSqlLogin
{
public static Task<GetSqlLoginResult> InvokeAsync(GetSqlLoginArgs args, InvokeOptions? opts = null)
public static Output<GetSqlLoginResult> Invoke(GetSqlLoginInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetSqlLoginResult> getSqlLogin(GetSqlLoginArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: mssql:index/getSqlLogin:getSqlLogin
arguments:
# arguments dictionary
The following arguments are supported:
- Name string
- Login name. Must follow Regular Identifiers rules and cannot contain
\
- Name string
- Login name. Must follow Regular Identifiers rules and cannot contain
\
- name String
- Login name. Must follow Regular Identifiers rules and cannot contain
\
- name string
- Login name. Must follow Regular Identifiers rules and cannot contain
\
- name str
- Login name. Must follow Regular Identifiers rules and cannot contain
\
- name String
- Login name. Must follow Regular Identifiers rules and cannot contain
\
getSqlLogin Result
The following output properties are available:
- Check
Password boolExpiration - When
true
, password expiration policy is enforced for this login. - Check
Password boolPolicy - When
true
, the Windows password policies of the computer on which SQL Server is running are enforced on this login. - Default
Database stringId - ID of login's default DB. The ID can be retrieved using
mssql.Database
data resource. - Default
Language string - Default language assigned to login.
- Id string
- Login SID. Can be retrieved using
SELECT SUSER_SID('<login_name>')
. - Must
Change boolPassword - When true, password change will be forced on first logon.
- Name string
- Login name. Must follow Regular Identifiers rules and cannot contain
\
- Principal
Id string - ID used to reference SQL Login in other resources, e.g.
server_role
. Can be retrieved fromsys.sql_logins
.
- Check
Password boolExpiration - When
true
, password expiration policy is enforced for this login. - Check
Password boolPolicy - When
true
, the Windows password policies of the computer on which SQL Server is running are enforced on this login. - Default
Database stringId - ID of login's default DB. The ID can be retrieved using
mssql.Database
data resource. - Default
Language string - Default language assigned to login.
- Id string
- Login SID. Can be retrieved using
SELECT SUSER_SID('<login_name>')
. - Must
Change boolPassword - When true, password change will be forced on first logon.
- Name string
- Login name. Must follow Regular Identifiers rules and cannot contain
\
- Principal
Id string - ID used to reference SQL Login in other resources, e.g.
server_role
. Can be retrieved fromsys.sql_logins
.
- check
Password BooleanExpiration - When
true
, password expiration policy is enforced for this login. - check
Password BooleanPolicy - When
true
, the Windows password policies of the computer on which SQL Server is running are enforced on this login. - default
Database StringId - ID of login's default DB. The ID can be retrieved using
mssql.Database
data resource. - default
Language String - Default language assigned to login.
- id String
- Login SID. Can be retrieved using
SELECT SUSER_SID('<login_name>')
. - must
Change BooleanPassword - When true, password change will be forced on first logon.
- name String
- Login name. Must follow Regular Identifiers rules and cannot contain
\
- principal
Id String - ID used to reference SQL Login in other resources, e.g.
server_role
. Can be retrieved fromsys.sql_logins
.
- check
Password booleanExpiration - When
true
, password expiration policy is enforced for this login. - check
Password booleanPolicy - When
true
, the Windows password policies of the computer on which SQL Server is running are enforced on this login. - default
Database stringId - ID of login's default DB. The ID can be retrieved using
mssql.Database
data resource. - default
Language string - Default language assigned to login.
- id string
- Login SID. Can be retrieved using
SELECT SUSER_SID('<login_name>')
. - must
Change booleanPassword - When true, password change will be forced on first logon.
- name string
- Login name. Must follow Regular Identifiers rules and cannot contain
\
- principal
Id string - ID used to reference SQL Login in other resources, e.g.
server_role
. Can be retrieved fromsys.sql_logins
.
- check_
password_ boolexpiration - When
true
, password expiration policy is enforced for this login. - check_
password_ boolpolicy - When
true
, the Windows password policies of the computer on which SQL Server is running are enforced on this login. - default_
database_ strid - ID of login's default DB. The ID can be retrieved using
mssql.Database
data resource. - default_
language str - Default language assigned to login.
- id str
- Login SID. Can be retrieved using
SELECT SUSER_SID('<login_name>')
. - must_
change_ boolpassword - When true, password change will be forced on first logon.
- name str
- Login name. Must follow Regular Identifiers rules and cannot contain
\
- principal_
id str - ID used to reference SQL Login in other resources, e.g.
server_role
. Can be retrieved fromsys.sql_logins
.
- check
Password BooleanExpiration - When
true
, password expiration policy is enforced for this login. - check
Password BooleanPolicy - When
true
, the Windows password policies of the computer on which SQL Server is running are enforced on this login. - default
Database StringId - ID of login's default DB. The ID can be retrieved using
mssql.Database
data resource. - default
Language String - Default language assigned to login.
- id String
- Login SID. Can be retrieved using
SELECT SUSER_SID('<login_name>')
. - must
Change BooleanPassword - When true, password change will be forced on first logon.
- name String
- Login name. Must follow Regular Identifiers rules and cannot contain
\
- principal
Id String - ID used to reference SQL Login in other resources, e.g.
server_role
. Can be retrieved fromsys.sql_logins
.
Package Details
- Repository
- mssql pulumiverse/pulumi-mssql
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
mssql
Terraform Provider.
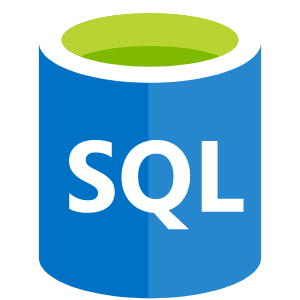
Microsoft SQL Server v0.0.8 published on Wednesday, Nov 1, 2023 by pulumiverse