Proxmox Virtual Environment (Proxmox VE) v6.14.0 published on Monday, Sep 9, 2024 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.Acme.getPlugin
Explore with Pulumi AI
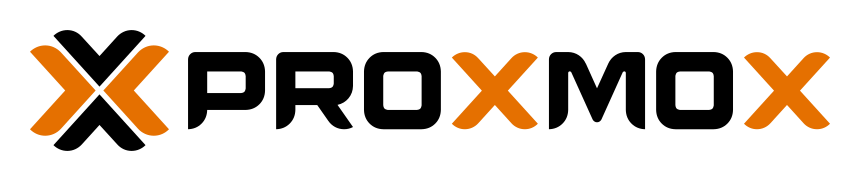
Proxmox Virtual Environment (Proxmox VE) v6.14.0 published on Monday, Sep 9, 2024 by Daniel Muehlbachler-Pietrzykowski
Retrieves a single ACME plugin by plugin ID name.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@pulumi/proxmoxve";
const example = proxmoxve.Acme.getPlugin({
plugin: "standalone",
});
export const dataProxmoxVirtualEnvironmentAcmePlugin = example;
import pulumi
import pulumi_proxmoxve as proxmoxve
example = proxmoxve.Acme.get_plugin(plugin="standalone")
pulumi.export("dataProxmoxVirtualEnvironmentAcmePlugin", example)
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Acme"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
example, err := Acme.GetPlugin(ctx, &acme.GetPluginArgs{
Plugin: "standalone",
}, nil)
if err != nil {
return err
}
ctx.Export("dataProxmoxVirtualEnvironmentAcmePlugin", example)
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var example = ProxmoxVE.Acme.GetPlugin.Invoke(new()
{
Plugin = "standalone",
});
return new Dictionary<string, object?>
{
["dataProxmoxVirtualEnvironmentAcmePlugin"] = example,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Acme.AcmeFunctions;
import com.pulumi.proxmoxve.Acme.inputs.GetPluginArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var example = AcmeFunctions.getPlugin(GetPluginArgs.builder()
.plugin("standalone")
.build());
ctx.export("dataProxmoxVirtualEnvironmentAcmePlugin", example.applyValue(getPluginResult -> getPluginResult));
}
}
variables:
example:
fn::invoke:
Function: proxmoxve:Acme:getPlugin
Arguments:
plugin: standalone
outputs:
dataProxmoxVirtualEnvironmentAcmePlugin: ${example}
Using getPlugin
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getPlugin(args: GetPluginArgs, opts?: InvokeOptions): Promise<GetPluginResult>
function getPluginOutput(args: GetPluginOutputArgs, opts?: InvokeOptions): Output<GetPluginResult>
def get_plugin(plugin: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetPluginResult
def get_plugin_output(plugin: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetPluginResult]
func GetPlugin(ctx *Context, args *GetPluginArgs, opts ...InvokeOption) (*GetPluginResult, error)
func GetPluginOutput(ctx *Context, args *GetPluginOutputArgs, opts ...InvokeOption) GetPluginResultOutput
> Note: This function is named GetPlugin
in the Go SDK.
public static class GetPlugin
{
public static Task<GetPluginResult> InvokeAsync(GetPluginArgs args, InvokeOptions? opts = null)
public static Output<GetPluginResult> Invoke(GetPluginInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetPluginResult> getPlugin(GetPluginArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: proxmoxve:Acme/getPlugin:getPlugin
arguments:
# arguments dictionary
The following arguments are supported:
- Plugin string
- ACME Plugin ID name.
- Plugin string
- ACME Plugin ID name.
- plugin String
- ACME Plugin ID name.
- plugin string
- ACME Plugin ID name.
- plugin str
- ACME Plugin ID name.
- plugin String
- ACME Plugin ID name.
getPlugin Result
The following output properties are available:
- Api string
- API plugin name.
- Data Dictionary<string, string>
- DNS plugin data.
- Digest string
- Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- Id string
- The provider-assigned unique ID for this managed resource.
- Plugin string
- ACME Plugin ID name.
- Type string
- ACME challenge type (dns, standalone).
- Validation
Delay int - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- Api string
- API plugin name.
- Data map[string]string
- DNS plugin data.
- Digest string
- Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- Id string
- The provider-assigned unique ID for this managed resource.
- Plugin string
- ACME Plugin ID name.
- Type string
- ACME challenge type (dns, standalone).
- Validation
Delay int - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- api String
- API plugin name.
- data Map<String,String>
- DNS plugin data.
- digest String
- Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- id String
- The provider-assigned unique ID for this managed resource.
- plugin String
- ACME Plugin ID name.
- type String
- ACME challenge type (dns, standalone).
- validation
Delay Integer - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- api string
- API plugin name.
- data {[key: string]: string}
- DNS plugin data.
- digest string
- Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- id string
- The provider-assigned unique ID for this managed resource.
- plugin string
- ACME Plugin ID name.
- type string
- ACME challenge type (dns, standalone).
- validation
Delay number - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- api str
- API plugin name.
- data Mapping[str, str]
- DNS plugin data.
- digest str
- Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- id str
- The provider-assigned unique ID for this managed resource.
- plugin str
- ACME Plugin ID name.
- type str
- ACME challenge type (dns, standalone).
- validation_
delay int - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- api String
- API plugin name.
- data Map<String>
- DNS plugin data.
- digest String
- Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- id String
- The provider-assigned unique ID for this managed resource.
- plugin String
- ACME Plugin ID name.
- type String
- ACME challenge type (dns, standalone).
- validation
Delay Number - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
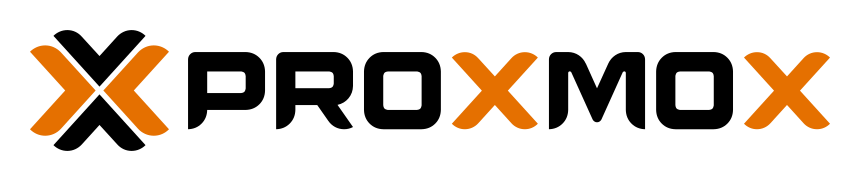
Proxmox Virtual Environment (Proxmox VE) v6.14.0 published on Monday, Sep 9, 2024 by Daniel Muehlbachler-Pietrzykowski