proxmoxve.AcmeAccount
Explore with Pulumi AI
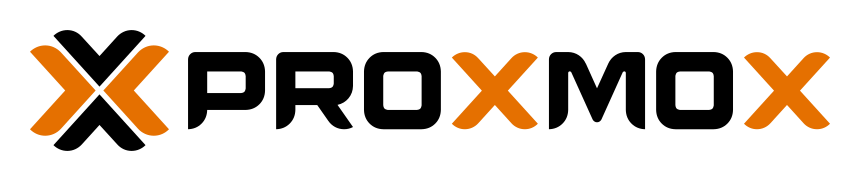
Manages an ACME account in a Proxmox VE cluster.
This resource requires
root@pam
authentication.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const example = new proxmoxve.AcmeAccount("example", {
contact: "example@email.com",
directory: "https://acme-staging-v02.api.letsencrypt.org/directory",
tos: "https://letsencrypt.org/documents/LE-SA-v1.3-September-21-2022.pdf",
});
import pulumi
import pulumi_proxmoxve as proxmoxve
example = proxmoxve.AcmeAccount("example",
contact="example@email.com",
directory="https://acme-staging-v02.api.letsencrypt.org/directory",
tos="https://letsencrypt.org/documents/LE-SA-v1.3-September-21-2022.pdf")
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := proxmoxve.NewAcmeAccount(ctx, "example", &proxmoxve.AcmeAccountArgs{
Contact: pulumi.String("example@email.com"),
Directory: pulumi.String("https://acme-staging-v02.api.letsencrypt.org/directory"),
Tos: pulumi.String("https://letsencrypt.org/documents/LE-SA-v1.3-September-21-2022.pdf"),
})
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var example = new ProxmoxVE.AcmeAccount("example", new()
{
Contact = "example@email.com",
Directory = "https://acme-staging-v02.api.letsencrypt.org/directory",
Tos = "https://letsencrypt.org/documents/LE-SA-v1.3-September-21-2022.pdf",
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.AcmeAccount;
import com.pulumi.proxmoxve.AcmeAccountArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var example = new AcmeAccount("example", AcmeAccountArgs.builder()
.contact("example@email.com")
.directory("https://acme-staging-v02.api.letsencrypt.org/directory")
.tos("https://letsencrypt.org/documents/LE-SA-v1.3-September-21-2022.pdf")
.build());
}
}
resources:
example:
type: proxmoxve:AcmeAccount
properties:
contact: example@email.com
directory: https://acme-staging-v02.api.letsencrypt.org/directory
tos: https://letsencrypt.org/documents/LE-SA-v1.3-September-21-2022.pdf
Create AcmeAccount Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new AcmeAccount(name: string, args: AcmeAccountArgs, opts?: CustomResourceOptions);
@overload
def AcmeAccount(resource_name: str,
args: AcmeAccountArgs,
opts: Optional[ResourceOptions] = None)
@overload
def AcmeAccount(resource_name: str,
opts: Optional[ResourceOptions] = None,
contact: Optional[str] = None,
directory: Optional[str] = None,
eab_hmac_key: Optional[str] = None,
eab_kid: Optional[str] = None,
name: Optional[str] = None,
tos: Optional[str] = None)
func NewAcmeAccount(ctx *Context, name string, args AcmeAccountArgs, opts ...ResourceOption) (*AcmeAccount, error)
public AcmeAccount(string name, AcmeAccountArgs args, CustomResourceOptions? opts = null)
public AcmeAccount(String name, AcmeAccountArgs args)
public AcmeAccount(String name, AcmeAccountArgs args, CustomResourceOptions options)
type: proxmoxve:AcmeAccount
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args AcmeAccountArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args AcmeAccountArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args AcmeAccountArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args AcmeAccountArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args AcmeAccountArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var acmeAccountResource = new ProxmoxVE.AcmeAccount("acmeAccountResource", new()
{
Contact = "string",
Directory = "string",
EabHmacKey = "string",
EabKid = "string",
Name = "string",
Tos = "string",
});
example, err := proxmoxve.NewAcmeAccount(ctx, "acmeAccountResource", &proxmoxve.AcmeAccountArgs{
Contact: pulumi.String("string"),
Directory: pulumi.String("string"),
EabHmacKey: pulumi.String("string"),
EabKid: pulumi.String("string"),
Name: pulumi.String("string"),
Tos: pulumi.String("string"),
})
var acmeAccountResource = new AcmeAccount("acmeAccountResource", AcmeAccountArgs.builder()
.contact("string")
.directory("string")
.eabHmacKey("string")
.eabKid("string")
.name("string")
.tos("string")
.build());
acme_account_resource = proxmoxve.AcmeAccount("acmeAccountResource",
contact="string",
directory="string",
eab_hmac_key="string",
eab_kid="string",
name="string",
tos="string")
const acmeAccountResource = new proxmoxve.AcmeAccount("acmeAccountResource", {
contact: "string",
directory: "string",
eabHmacKey: "string",
eabKid: "string",
name: "string",
tos: "string",
});
type: proxmoxve:AcmeAccount
properties:
contact: string
directory: string
eabHmacKey: string
eabKid: string
name: string
tos: string
AcmeAccount Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The AcmeAccount resource accepts the following input properties:
- Contact string
- The contact email addresses.
- Directory string
- The URL of the ACME CA directory endpoint.
- Eab
Hmac stringKey - The HMAC key for External Account Binding.
- Eab
Kid string - The Key Identifier for External Account Binding.
- Name string
- The ACME account config file name.
- Tos string
- The URL of CA TermsOfService - setting this indicates agreement.
- Contact string
- The contact email addresses.
- Directory string
- The URL of the ACME CA directory endpoint.
- Eab
Hmac stringKey - The HMAC key for External Account Binding.
- Eab
Kid string - The Key Identifier for External Account Binding.
- Name string
- The ACME account config file name.
- Tos string
- The URL of CA TermsOfService - setting this indicates agreement.
- contact String
- The contact email addresses.
- directory String
- The URL of the ACME CA directory endpoint.
- eab
Hmac StringKey - The HMAC key for External Account Binding.
- eab
Kid String - The Key Identifier for External Account Binding.
- name String
- The ACME account config file name.
- tos String
- The URL of CA TermsOfService - setting this indicates agreement.
- contact string
- The contact email addresses.
- directory string
- The URL of the ACME CA directory endpoint.
- eab
Hmac stringKey - The HMAC key for External Account Binding.
- eab
Kid string - The Key Identifier for External Account Binding.
- name string
- The ACME account config file name.
- tos string
- The URL of CA TermsOfService - setting this indicates agreement.
- contact str
- The contact email addresses.
- directory str
- The URL of the ACME CA directory endpoint.
- eab_
hmac_ strkey - The HMAC key for External Account Binding.
- eab_
kid str - The Key Identifier for External Account Binding.
- name str
- The ACME account config file name.
- tos str
- The URL of CA TermsOfService - setting this indicates agreement.
- contact String
- The contact email addresses.
- directory String
- The URL of the ACME CA directory endpoint.
- eab
Hmac StringKey - The HMAC key for External Account Binding.
- eab
Kid String - The Key Identifier for External Account Binding.
- name String
- The ACME account config file name.
- tos String
- The URL of CA TermsOfService - setting this indicates agreement.
Outputs
All input properties are implicitly available as output properties. Additionally, the AcmeAccount resource produces the following output properties:
- created_
at str - The timestamp of the ACME account creation.
- id str
- The provider-assigned unique ID for this managed resource.
- location str
- The location of the ACME account.
Look up Existing AcmeAccount Resource
Get an existing AcmeAccount resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: AcmeAccountState, opts?: CustomResourceOptions): AcmeAccount
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
contact: Optional[str] = None,
created_at: Optional[str] = None,
directory: Optional[str] = None,
eab_hmac_key: Optional[str] = None,
eab_kid: Optional[str] = None,
location: Optional[str] = None,
name: Optional[str] = None,
tos: Optional[str] = None) -> AcmeAccount
func GetAcmeAccount(ctx *Context, name string, id IDInput, state *AcmeAccountState, opts ...ResourceOption) (*AcmeAccount, error)
public static AcmeAccount Get(string name, Input<string> id, AcmeAccountState? state, CustomResourceOptions? opts = null)
public static AcmeAccount get(String name, Output<String> id, AcmeAccountState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Contact string
- The contact email addresses.
- Created
At string - The timestamp of the ACME account creation.
- Directory string
- The URL of the ACME CA directory endpoint.
- Eab
Hmac stringKey - The HMAC key for External Account Binding.
- Eab
Kid string - The Key Identifier for External Account Binding.
- Location string
- The location of the ACME account.
- Name string
- The ACME account config file name.
- Tos string
- The URL of CA TermsOfService - setting this indicates agreement.
- Contact string
- The contact email addresses.
- Created
At string - The timestamp of the ACME account creation.
- Directory string
- The URL of the ACME CA directory endpoint.
- Eab
Hmac stringKey - The HMAC key for External Account Binding.
- Eab
Kid string - The Key Identifier for External Account Binding.
- Location string
- The location of the ACME account.
- Name string
- The ACME account config file name.
- Tos string
- The URL of CA TermsOfService - setting this indicates agreement.
- contact String
- The contact email addresses.
- created
At String - The timestamp of the ACME account creation.
- directory String
- The URL of the ACME CA directory endpoint.
- eab
Hmac StringKey - The HMAC key for External Account Binding.
- eab
Kid String - The Key Identifier for External Account Binding.
- location String
- The location of the ACME account.
- name String
- The ACME account config file name.
- tos String
- The URL of CA TermsOfService - setting this indicates agreement.
- contact string
- The contact email addresses.
- created
At string - The timestamp of the ACME account creation.
- directory string
- The URL of the ACME CA directory endpoint.
- eab
Hmac stringKey - The HMAC key for External Account Binding.
- eab
Kid string - The Key Identifier for External Account Binding.
- location string
- The location of the ACME account.
- name string
- The ACME account config file name.
- tos string
- The URL of CA TermsOfService - setting this indicates agreement.
- contact str
- The contact email addresses.
- created_
at str - The timestamp of the ACME account creation.
- directory str
- The URL of the ACME CA directory endpoint.
- eab_
hmac_ strkey - The HMAC key for External Account Binding.
- eab_
kid str - The Key Identifier for External Account Binding.
- location str
- The location of the ACME account.
- name str
- The ACME account config file name.
- tos str
- The URL of CA TermsOfService - setting this indicates agreement.
- contact String
- The contact email addresses.
- created
At String - The timestamp of the ACME account creation.
- directory String
- The URL of the ACME CA directory endpoint.
- eab
Hmac StringKey - The HMAC key for External Account Binding.
- eab
Kid String - The Key Identifier for External Account Binding.
- location String
- The location of the ACME account.
- name String
- The ACME account config file name.
- tos String
- The URL of CA TermsOfService - setting this indicates agreement.
Import
#!/usr/bin/env sh
ACME accounts can be imported using their name, e.g.:
$ pulumi import proxmoxve:index/acmeAccount:AcmeAccount example example
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
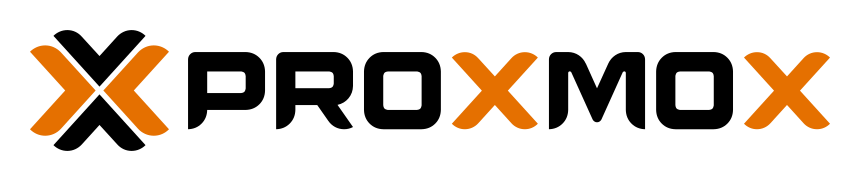