proxmoxve.AcmeDnsPlugin
Explore with Pulumi AI
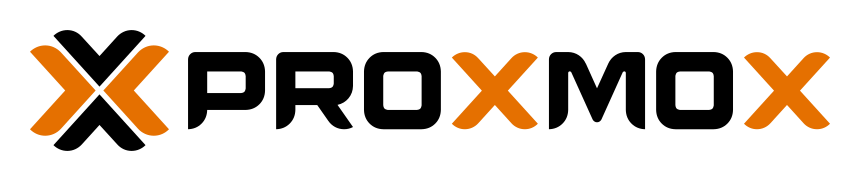
Manages an ACME plugin in a Proxmox VE cluster.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const example = new proxmoxve.AcmeDnsPlugin("example", {
api: "aws",
data: {
AWS_ACCESS_KEY_ID: "EXAMPLE",
AWS_SECRET_ACCESS_KEY: "EXAMPLE",
},
plugin: "test",
});
import pulumi
import pulumi_proxmoxve as proxmoxve
example = proxmoxve.AcmeDnsPlugin("example",
api="aws",
data={
"AWS_ACCESS_KEY_ID": "EXAMPLE",
"AWS_SECRET_ACCESS_KEY": "EXAMPLE",
},
plugin="test")
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := proxmoxve.NewAcmeDnsPlugin(ctx, "example", &proxmoxve.AcmeDnsPluginArgs{
Api: pulumi.String("aws"),
Data: pulumi.StringMap{
"AWS_ACCESS_KEY_ID": pulumi.String("EXAMPLE"),
"AWS_SECRET_ACCESS_KEY": pulumi.String("EXAMPLE"),
},
Plugin: pulumi.String("test"),
})
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var example = new ProxmoxVE.AcmeDnsPlugin("example", new()
{
Api = "aws",
Data =
{
{ "AWS_ACCESS_KEY_ID", "EXAMPLE" },
{ "AWS_SECRET_ACCESS_KEY", "EXAMPLE" },
},
Plugin = "test",
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.AcmeDnsPlugin;
import com.pulumi.proxmoxve.AcmeDnsPluginArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var example = new AcmeDnsPlugin("example", AcmeDnsPluginArgs.builder()
.api("aws")
.data(Map.ofEntries(
Map.entry("AWS_ACCESS_KEY_ID", "EXAMPLE"),
Map.entry("AWS_SECRET_ACCESS_KEY", "EXAMPLE")
))
.plugin("test")
.build());
}
}
resources:
example:
type: proxmoxve:AcmeDnsPlugin
properties:
api: aws
data:
AWS_ACCESS_KEY_ID: EXAMPLE
AWS_SECRET_ACCESS_KEY: EXAMPLE
plugin: test
Create AcmeDnsPlugin Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new AcmeDnsPlugin(name: string, args: AcmeDnsPluginArgs, opts?: CustomResourceOptions);
@overload
def AcmeDnsPlugin(resource_name: str,
args: AcmeDnsPluginArgs,
opts: Optional[ResourceOptions] = None)
@overload
def AcmeDnsPlugin(resource_name: str,
opts: Optional[ResourceOptions] = None,
api: Optional[str] = None,
plugin: Optional[str] = None,
data: Optional[Mapping[str, str]] = None,
digest: Optional[str] = None,
disable: Optional[bool] = None,
validation_delay: Optional[int] = None)
func NewAcmeDnsPlugin(ctx *Context, name string, args AcmeDnsPluginArgs, opts ...ResourceOption) (*AcmeDnsPlugin, error)
public AcmeDnsPlugin(string name, AcmeDnsPluginArgs args, CustomResourceOptions? opts = null)
public AcmeDnsPlugin(String name, AcmeDnsPluginArgs args)
public AcmeDnsPlugin(String name, AcmeDnsPluginArgs args, CustomResourceOptions options)
type: proxmoxve:AcmeDnsPlugin
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args AcmeDnsPluginArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args AcmeDnsPluginArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args AcmeDnsPluginArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args AcmeDnsPluginArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args AcmeDnsPluginArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var acmeDnsPluginResource = new ProxmoxVE.AcmeDnsPlugin("acmeDnsPluginResource", new()
{
Api = "string",
Plugin = "string",
Data =
{
{ "string", "string" },
},
Digest = "string",
Disable = false,
ValidationDelay = 0,
});
example, err := proxmoxve.NewAcmeDnsPlugin(ctx, "acmeDnsPluginResource", &proxmoxve.AcmeDnsPluginArgs{
Api: pulumi.String("string"),
Plugin: pulumi.String("string"),
Data: pulumi.StringMap{
"string": pulumi.String("string"),
},
Digest: pulumi.String("string"),
Disable: pulumi.Bool(false),
ValidationDelay: pulumi.Int(0),
})
var acmeDnsPluginResource = new AcmeDnsPlugin("acmeDnsPluginResource", AcmeDnsPluginArgs.builder()
.api("string")
.plugin("string")
.data(Map.of("string", "string"))
.digest("string")
.disable(false)
.validationDelay(0)
.build());
acme_dns_plugin_resource = proxmoxve.AcmeDnsPlugin("acmeDnsPluginResource",
api="string",
plugin="string",
data={
"string": "string",
},
digest="string",
disable=False,
validation_delay=0)
const acmeDnsPluginResource = new proxmoxve.AcmeDnsPlugin("acmeDnsPluginResource", {
api: "string",
plugin: "string",
data: {
string: "string",
},
digest: "string",
disable: false,
validationDelay: 0,
});
type: proxmoxve:AcmeDnsPlugin
properties:
api: string
data:
string: string
digest: string
disable: false
plugin: string
validationDelay: 0
AcmeDnsPlugin Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The AcmeDnsPlugin resource accepts the following input properties:
- Api string
- API plugin name.
- Plugin string
- ACME Plugin ID name.
- Data Dictionary<string, string>
- DNS plugin data.
- Digest string
- SHA1 digest of the current configuration. Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- Disable bool
- Flag to disable the config.
- Validation
Delay int - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- Api string
- API plugin name.
- Plugin string
- ACME Plugin ID name.
- Data map[string]string
- DNS plugin data.
- Digest string
- SHA1 digest of the current configuration. Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- Disable bool
- Flag to disable the config.
- Validation
Delay int - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- api String
- API plugin name.
- plugin String
- ACME Plugin ID name.
- data Map<String,String>
- DNS plugin data.
- digest String
- SHA1 digest of the current configuration. Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- disable Boolean
- Flag to disable the config.
- validation
Delay Integer - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- api string
- API plugin name.
- plugin string
- ACME Plugin ID name.
- data {[key: string]: string}
- DNS plugin data.
- digest string
- SHA1 digest of the current configuration. Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- disable boolean
- Flag to disable the config.
- validation
Delay number - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- api str
- API plugin name.
- plugin str
- ACME Plugin ID name.
- data Mapping[str, str]
- DNS plugin data.
- digest str
- SHA1 digest of the current configuration. Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- disable bool
- Flag to disable the config.
- validation_
delay int - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- api String
- API plugin name.
- plugin String
- ACME Plugin ID name.
- data Map<String>
- DNS plugin data.
- digest String
- SHA1 digest of the current configuration. Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- disable Boolean
- Flag to disable the config.
- validation
Delay Number - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
Outputs
All input properties are implicitly available as output properties. Additionally, the AcmeDnsPlugin resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing AcmeDnsPlugin Resource
Get an existing AcmeDnsPlugin resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: AcmeDnsPluginState, opts?: CustomResourceOptions): AcmeDnsPlugin
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
api: Optional[str] = None,
data: Optional[Mapping[str, str]] = None,
digest: Optional[str] = None,
disable: Optional[bool] = None,
plugin: Optional[str] = None,
validation_delay: Optional[int] = None) -> AcmeDnsPlugin
func GetAcmeDnsPlugin(ctx *Context, name string, id IDInput, state *AcmeDnsPluginState, opts ...ResourceOption) (*AcmeDnsPlugin, error)
public static AcmeDnsPlugin Get(string name, Input<string> id, AcmeDnsPluginState? state, CustomResourceOptions? opts = null)
public static AcmeDnsPlugin get(String name, Output<String> id, AcmeDnsPluginState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Api string
- API plugin name.
- Data Dictionary<string, string>
- DNS plugin data.
- Digest string
- SHA1 digest of the current configuration. Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- Disable bool
- Flag to disable the config.
- Plugin string
- ACME Plugin ID name.
- Validation
Delay int - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- Api string
- API plugin name.
- Data map[string]string
- DNS plugin data.
- Digest string
- SHA1 digest of the current configuration. Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- Disable bool
- Flag to disable the config.
- Plugin string
- ACME Plugin ID name.
- Validation
Delay int - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- api String
- API plugin name.
- data Map<String,String>
- DNS plugin data.
- digest String
- SHA1 digest of the current configuration. Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- disable Boolean
- Flag to disable the config.
- plugin String
- ACME Plugin ID name.
- validation
Delay Integer - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- api string
- API plugin name.
- data {[key: string]: string}
- DNS plugin data.
- digest string
- SHA1 digest of the current configuration. Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- disable boolean
- Flag to disable the config.
- plugin string
- ACME Plugin ID name.
- validation
Delay number - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- api str
- API plugin name.
- data Mapping[str, str]
- DNS plugin data.
- digest str
- SHA1 digest of the current configuration. Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- disable bool
- Flag to disable the config.
- plugin str
- ACME Plugin ID name.
- validation_
delay int - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
- api String
- API plugin name.
- data Map<String>
- DNS plugin data.
- digest String
- SHA1 digest of the current configuration. Prevent changes if current configuration file has a different digest. This can be used to prevent concurrent modifications.
- disable Boolean
- Flag to disable the config.
- plugin String
- ACME Plugin ID name.
- validation
Delay Number - Extra delay in seconds to wait before requesting validation. Allows to cope with a long TTL of DNS records (0 - 172800).
Import
#!/usr/bin/env sh
ACME accounts can be imported using their name, e.g.:
$ pulumi import proxmoxve:index/acmeDnsPlugin:AcmeDnsPlugin example test
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
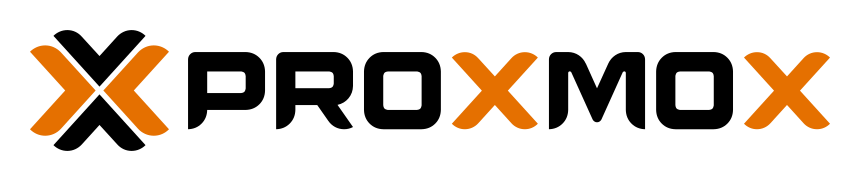