Proxmox Virtual Environment (Proxmox VE) v6.14.0 published on Monday, Sep 9, 2024 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.Apt.getRepository
Explore with Pulumi AI
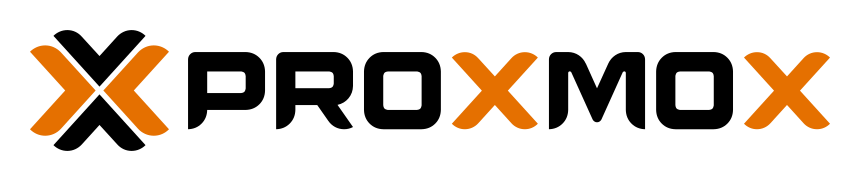
Proxmox Virtual Environment (Proxmox VE) v6.14.0 published on Monday, Sep 9, 2024 by Daniel Muehlbachler-Pietrzykowski
Retrieves an APT repository from a Proxmox VE cluster.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@pulumi/proxmoxve";
const example = proxmoxve.Apt.getRepository({
filePath: "/etc/apt/sources.list",
index: 0,
node: "pve",
});
export const proxmoxVirtualEnvironmentAptRepository = example;
import pulumi
import pulumi_proxmoxve as proxmoxve
example = proxmoxve.Apt.get_repository(file_path="/etc/apt/sources.list",
index=0,
node="pve")
pulumi.export("proxmoxVirtualEnvironmentAptRepository", example)
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Apt"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
example, err := Apt.GetRepository(ctx, &apt.GetRepositoryArgs{
FilePath: "/etc/apt/sources.list",
Index: 0,
Node: "pve",
}, nil)
if err != nil {
return err
}
ctx.Export("proxmoxVirtualEnvironmentAptRepository", example)
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var example = ProxmoxVE.Apt.GetRepository.Invoke(new()
{
FilePath = "/etc/apt/sources.list",
Index = 0,
Node = "pve",
});
return new Dictionary<string, object?>
{
["proxmoxVirtualEnvironmentAptRepository"] = example,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Apt.AptFunctions;
import com.pulumi.proxmoxve.Apt.inputs.GetRepositoryArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var example = AptFunctions.getRepository(GetRepositoryArgs.builder()
.filePath("/etc/apt/sources.list")
.index(0)
.node("pve")
.build());
ctx.export("proxmoxVirtualEnvironmentAptRepository", example.applyValue(getRepositoryResult -> getRepositoryResult));
}
}
variables:
example:
fn::invoke:
Function: proxmoxve:Apt:getRepository
Arguments:
filePath: /etc/apt/sources.list
index: 0
node: pve
outputs:
proxmoxVirtualEnvironmentAptRepository: ${example}
Using getRepository
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getRepository(args: GetRepositoryArgs, opts?: InvokeOptions): Promise<GetRepositoryResult>
function getRepositoryOutput(args: GetRepositoryOutputArgs, opts?: InvokeOptions): Output<GetRepositoryResult>
def get_repository(file_path: Optional[str] = None,
index: Optional[int] = None,
node: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetRepositoryResult
def get_repository_output(file_path: Optional[pulumi.Input[str]] = None,
index: Optional[pulumi.Input[int]] = None,
node: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetRepositoryResult]
func GetRepository(ctx *Context, args *GetRepositoryArgs, opts ...InvokeOption) (*GetRepositoryResult, error)
func GetRepositoryOutput(ctx *Context, args *GetRepositoryOutputArgs, opts ...InvokeOption) GetRepositoryResultOutput
> Note: This function is named GetRepository
in the Go SDK.
public static class GetRepository
{
public static Task<GetRepositoryResult> InvokeAsync(GetRepositoryArgs args, InvokeOptions? opts = null)
public static Output<GetRepositoryResult> Invoke(GetRepositoryInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetRepositoryResult> getRepository(GetRepositoryArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: proxmoxve:Apt/getRepository:getRepository
arguments:
# arguments dictionary
The following arguments are supported:
getRepository Result
The following output properties are available:
- Comment string
- The associated comment.
- Components List<string>
- The list of components.
- Enabled bool
- Indicates the activation status.
- File
Path string - The absolute path of the source list file that contains this repository.
- File
Type string - The format of the defining source list file.
- Id string
- The unique identifier of this APT repository data source.
- Index int
- The index within the defining source list file.
- Node string
- The name of the target Proxmox VE node.
- Package
Types List<string> - The list of package types.
- Suites List<string>
- The list of package distributions.
- Uris List<string>
- The list of repository URIs.
- Comment string
- The associated comment.
- Components []string
- The list of components.
- Enabled bool
- Indicates the activation status.
- File
Path string - The absolute path of the source list file that contains this repository.
- File
Type string - The format of the defining source list file.
- Id string
- The unique identifier of this APT repository data source.
- Index int
- The index within the defining source list file.
- Node string
- The name of the target Proxmox VE node.
- Package
Types []string - The list of package types.
- Suites []string
- The list of package distributions.
- Uris []string
- The list of repository URIs.
- comment String
- The associated comment.
- components List<String>
- The list of components.
- enabled Boolean
- Indicates the activation status.
- file
Path String - The absolute path of the source list file that contains this repository.
- file
Type String - The format of the defining source list file.
- id String
- The unique identifier of this APT repository data source.
- index Integer
- The index within the defining source list file.
- node String
- The name of the target Proxmox VE node.
- package
Types List<String> - The list of package types.
- suites List<String>
- The list of package distributions.
- uris List<String>
- The list of repository URIs.
- comment string
- The associated comment.
- components string[]
- The list of components.
- enabled boolean
- Indicates the activation status.
- file
Path string - The absolute path of the source list file that contains this repository.
- file
Type string - The format of the defining source list file.
- id string
- The unique identifier of this APT repository data source.
- index number
- The index within the defining source list file.
- node string
- The name of the target Proxmox VE node.
- package
Types string[] - The list of package types.
- suites string[]
- The list of package distributions.
- uris string[]
- The list of repository URIs.
- comment str
- The associated comment.
- components Sequence[str]
- The list of components.
- enabled bool
- Indicates the activation status.
- file_
path str - The absolute path of the source list file that contains this repository.
- file_
type str - The format of the defining source list file.
- id str
- The unique identifier of this APT repository data source.
- index int
- The index within the defining source list file.
- node str
- The name of the target Proxmox VE node.
- package_
types Sequence[str] - The list of package types.
- suites Sequence[str]
- The list of package distributions.
- uris Sequence[str]
- The list of repository URIs.
- comment String
- The associated comment.
- components List<String>
- The list of components.
- enabled Boolean
- Indicates the activation status.
- file
Path String - The absolute path of the source list file that contains this repository.
- file
Type String - The format of the defining source list file.
- id String
- The unique identifier of this APT repository data source.
- index Number
- The index within the defining source list file.
- node String
- The name of the target Proxmox VE node.
- package
Types List<String> - The list of package types.
- suites List<String>
- The list of package distributions.
- uris List<String>
- The list of repository URIs.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
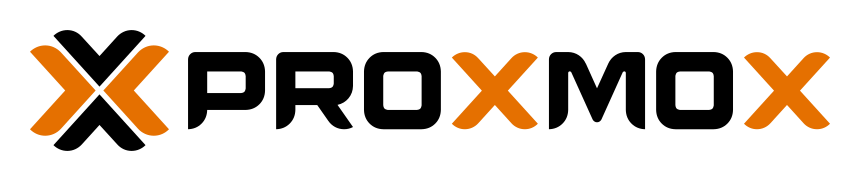
Proxmox Virtual Environment (Proxmox VE) v6.14.0 published on Monday, Sep 9, 2024 by Daniel Muehlbachler-Pietrzykowski