proxmoxve.CT.Container
Explore with Pulumi AI
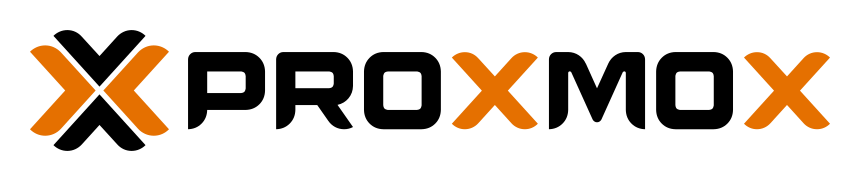
Manages a container.
Create Container Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new Container(name: string, args: ContainerArgs, opts?: CustomResourceOptions);
@overload
def Container(resource_name: str,
args: ContainerArgs,
opts: Optional[ResourceOptions] = None)
@overload
def Container(resource_name: str,
opts: Optional[ResourceOptions] = None,
node_name: Optional[str] = None,
operating_system: Optional[_ct.ContainerOperatingSystemArgs] = None,
description: Optional[str] = None,
protection: Optional[bool] = None,
start_on_boot: Optional[bool] = None,
features: Optional[_ct.ContainerFeaturesArgs] = None,
hook_script_file_id: Optional[str] = None,
initialization: Optional[_ct.ContainerInitializationArgs] = None,
memory: Optional[_ct.ContainerMemoryArgs] = None,
mount_points: Optional[Sequence[_ct.ContainerMountPointArgs]] = None,
network_interfaces: Optional[Sequence[_ct.ContainerNetworkInterfaceArgs]] = None,
console: Optional[_ct.ContainerConsoleArgs] = None,
clone: Optional[_ct.ContainerCloneArgs] = None,
vm_id: Optional[int] = None,
cpu: Optional[_ct.ContainerCpuArgs] = None,
disk: Optional[_ct.ContainerDiskArgs] = None,
started: Optional[bool] = None,
startup: Optional[_ct.ContainerStartupArgs] = None,
tags: Optional[Sequence[str]] = None,
template: Optional[bool] = None,
timeout_clone: Optional[int] = None,
timeout_create: Optional[int] = None,
timeout_delete: Optional[int] = None,
timeout_start: Optional[int] = None,
timeout_update: Optional[int] = None,
unprivileged: Optional[bool] = None,
pool_id: Optional[str] = None)
func NewContainer(ctx *Context, name string, args ContainerArgs, opts ...ResourceOption) (*Container, error)
public Container(string name, ContainerArgs args, CustomResourceOptions? opts = null)
public Container(String name, ContainerArgs args)
public Container(String name, ContainerArgs args, CustomResourceOptions options)
type: proxmoxve:CT:Container
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args ContainerArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args ContainerArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args ContainerArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args ContainerArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args ContainerArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var containerResource = new ProxmoxVE.CT.Container("containerResource", new()
{
NodeName = "string",
OperatingSystem = new ProxmoxVE.CT.Inputs.ContainerOperatingSystemArgs
{
TemplateFileId = "string",
Type = "string",
},
Description = "string",
Protection = false,
StartOnBoot = false,
Features = new ProxmoxVE.CT.Inputs.ContainerFeaturesArgs
{
Fuse = false,
Keyctl = false,
Mounts = new[]
{
"string",
},
Nesting = false,
},
HookScriptFileId = "string",
Initialization = new ProxmoxVE.CT.Inputs.ContainerInitializationArgs
{
Dns = new ProxmoxVE.CT.Inputs.ContainerInitializationDnsArgs
{
Domain = "string",
Servers = new[]
{
"string",
},
},
Hostname = "string",
IpConfigs = new[]
{
new ProxmoxVE.CT.Inputs.ContainerInitializationIpConfigArgs
{
Ipv4 = new ProxmoxVE.CT.Inputs.ContainerInitializationIpConfigIpv4Args
{
Address = "string",
Gateway = "string",
},
Ipv6 = new ProxmoxVE.CT.Inputs.ContainerInitializationIpConfigIpv6Args
{
Address = "string",
Gateway = "string",
},
},
},
UserAccount = new ProxmoxVE.CT.Inputs.ContainerInitializationUserAccountArgs
{
Keys = new[]
{
"string",
},
Password = "string",
},
},
Memory = new ProxmoxVE.CT.Inputs.ContainerMemoryArgs
{
Dedicated = 0,
Swap = 0,
},
MountPoints = new[]
{
new ProxmoxVE.CT.Inputs.ContainerMountPointArgs
{
Path = "string",
Volume = "string",
Acl = false,
Backup = false,
MountOptions = new[]
{
"string",
},
Quota = false,
ReadOnly = false,
Replicate = false,
Shared = false,
Size = "string",
},
},
NetworkInterfaces = new[]
{
new ProxmoxVE.CT.Inputs.ContainerNetworkInterfaceArgs
{
Name = "string",
Bridge = "string",
Enabled = false,
Firewall = false,
MacAddress = "string",
Mtu = 0,
RateLimit = 0,
VlanId = 0,
},
},
Console = new ProxmoxVE.CT.Inputs.ContainerConsoleArgs
{
Enabled = false,
TtyCount = 0,
Type = "string",
},
Clone = new ProxmoxVE.CT.Inputs.ContainerCloneArgs
{
VmId = 0,
DatastoreId = "string",
NodeName = "string",
},
VmId = 0,
Cpu = new ProxmoxVE.CT.Inputs.ContainerCpuArgs
{
Architecture = "string",
Cores = 0,
Units = 0,
},
Disk = new ProxmoxVE.CT.Inputs.ContainerDiskArgs
{
DatastoreId = "string",
Size = 0,
},
Started = false,
Startup = new ProxmoxVE.CT.Inputs.ContainerStartupArgs
{
DownDelay = 0,
Order = 0,
UpDelay = 0,
},
Tags = new[]
{
"string",
},
Template = false,
TimeoutClone = 0,
TimeoutCreate = 0,
TimeoutDelete = 0,
TimeoutUpdate = 0,
Unprivileged = false,
PoolId = "string",
});
example, err := CT.NewContainer(ctx, "containerResource", &CT.ContainerArgs{
NodeName: pulumi.String("string"),
OperatingSystem: &ct.ContainerOperatingSystemArgs{
TemplateFileId: pulumi.String("string"),
Type: pulumi.String("string"),
},
Description: pulumi.String("string"),
Protection: pulumi.Bool(false),
StartOnBoot: pulumi.Bool(false),
Features: &ct.ContainerFeaturesArgs{
Fuse: pulumi.Bool(false),
Keyctl: pulumi.Bool(false),
Mounts: pulumi.StringArray{
pulumi.String("string"),
},
Nesting: pulumi.Bool(false),
},
HookScriptFileId: pulumi.String("string"),
Initialization: &ct.ContainerInitializationArgs{
Dns: &ct.ContainerInitializationDnsArgs{
Domain: pulumi.String("string"),
Servers: pulumi.StringArray{
pulumi.String("string"),
},
},
Hostname: pulumi.String("string"),
IpConfigs: ct.ContainerInitializationIpConfigArray{
&ct.ContainerInitializationIpConfigArgs{
Ipv4: &ct.ContainerInitializationIpConfigIpv4Args{
Address: pulumi.String("string"),
Gateway: pulumi.String("string"),
},
Ipv6: &ct.ContainerInitializationIpConfigIpv6Args{
Address: pulumi.String("string"),
Gateway: pulumi.String("string"),
},
},
},
UserAccount: &ct.ContainerInitializationUserAccountArgs{
Keys: pulumi.StringArray{
pulumi.String("string"),
},
Password: pulumi.String("string"),
},
},
Memory: &ct.ContainerMemoryArgs{
Dedicated: pulumi.Int(0),
Swap: pulumi.Int(0),
},
MountPoints: ct.ContainerMountPointArray{
&ct.ContainerMountPointArgs{
Path: pulumi.String("string"),
Volume: pulumi.String("string"),
Acl: pulumi.Bool(false),
Backup: pulumi.Bool(false),
MountOptions: pulumi.StringArray{
pulumi.String("string"),
},
Quota: pulumi.Bool(false),
ReadOnly: pulumi.Bool(false),
Replicate: pulumi.Bool(false),
Shared: pulumi.Bool(false),
Size: pulumi.String("string"),
},
},
NetworkInterfaces: ct.ContainerNetworkInterfaceArray{
&ct.ContainerNetworkInterfaceArgs{
Name: pulumi.String("string"),
Bridge: pulumi.String("string"),
Enabled: pulumi.Bool(false),
Firewall: pulumi.Bool(false),
MacAddress: pulumi.String("string"),
Mtu: pulumi.Int(0),
RateLimit: pulumi.Float64(0),
VlanId: pulumi.Int(0),
},
},
Console: &ct.ContainerConsoleArgs{
Enabled: pulumi.Bool(false),
TtyCount: pulumi.Int(0),
Type: pulumi.String("string"),
},
Clone: &ct.ContainerCloneArgs{
VmId: pulumi.Int(0),
DatastoreId: pulumi.String("string"),
NodeName: pulumi.String("string"),
},
VmId: pulumi.Int(0),
Cpu: &ct.ContainerCpuArgs{
Architecture: pulumi.String("string"),
Cores: pulumi.Int(0),
Units: pulumi.Int(0),
},
Disk: &ct.ContainerDiskArgs{
DatastoreId: pulumi.String("string"),
Size: pulumi.Int(0),
},
Started: pulumi.Bool(false),
Startup: &ct.ContainerStartupArgs{
DownDelay: pulumi.Int(0),
Order: pulumi.Int(0),
UpDelay: pulumi.Int(0),
},
Tags: pulumi.StringArray{
pulumi.String("string"),
},
Template: pulumi.Bool(false),
TimeoutClone: pulumi.Int(0),
TimeoutCreate: pulumi.Int(0),
TimeoutDelete: pulumi.Int(0),
TimeoutUpdate: pulumi.Int(0),
Unprivileged: pulumi.Bool(false),
PoolId: pulumi.String("string"),
})
var containerResource = new Container("containerResource", ContainerArgs.builder()
.nodeName("string")
.operatingSystem(ContainerOperatingSystemArgs.builder()
.templateFileId("string")
.type("string")
.build())
.description("string")
.protection(false)
.startOnBoot(false)
.features(ContainerFeaturesArgs.builder()
.fuse(false)
.keyctl(false)
.mounts("string")
.nesting(false)
.build())
.hookScriptFileId("string")
.initialization(ContainerInitializationArgs.builder()
.dns(ContainerInitializationDnsArgs.builder()
.domain("string")
.servers("string")
.build())
.hostname("string")
.ipConfigs(ContainerInitializationIpConfigArgs.builder()
.ipv4(ContainerInitializationIpConfigIpv4Args.builder()
.address("string")
.gateway("string")
.build())
.ipv6(ContainerInitializationIpConfigIpv6Args.builder()
.address("string")
.gateway("string")
.build())
.build())
.userAccount(ContainerInitializationUserAccountArgs.builder()
.keys("string")
.password("string")
.build())
.build())
.memory(ContainerMemoryArgs.builder()
.dedicated(0)
.swap(0)
.build())
.mountPoints(ContainerMountPointArgs.builder()
.path("string")
.volume("string")
.acl(false)
.backup(false)
.mountOptions("string")
.quota(false)
.readOnly(false)
.replicate(false)
.shared(false)
.size("string")
.build())
.networkInterfaces(ContainerNetworkInterfaceArgs.builder()
.name("string")
.bridge("string")
.enabled(false)
.firewall(false)
.macAddress("string")
.mtu(0)
.rateLimit(0)
.vlanId(0)
.build())
.console(ContainerConsoleArgs.builder()
.enabled(false)
.ttyCount(0)
.type("string")
.build())
.clone(ContainerCloneArgs.builder()
.vmId(0)
.datastoreId("string")
.nodeName("string")
.build())
.vmId(0)
.cpu(ContainerCpuArgs.builder()
.architecture("string")
.cores(0)
.units(0)
.build())
.disk(ContainerDiskArgs.builder()
.datastoreId("string")
.size(0)
.build())
.started(false)
.startup(ContainerStartupArgs.builder()
.downDelay(0)
.order(0)
.upDelay(0)
.build())
.tags("string")
.template(false)
.timeoutClone(0)
.timeoutCreate(0)
.timeoutDelete(0)
.timeoutUpdate(0)
.unprivileged(false)
.poolId("string")
.build());
container_resource = proxmoxve.ct.Container("containerResource",
node_name="string",
operating_system=proxmoxve.ct.ContainerOperatingSystemArgs(
template_file_id="string",
type="string",
),
description="string",
protection=False,
start_on_boot=False,
features=proxmoxve.ct.ContainerFeaturesArgs(
fuse=False,
keyctl=False,
mounts=["string"],
nesting=False,
),
hook_script_file_id="string",
initialization=proxmoxve.ct.ContainerInitializationArgs(
dns=proxmoxve.ct.ContainerInitializationDnsArgs(
domain="string",
servers=["string"],
),
hostname="string",
ip_configs=[proxmoxve.ct.ContainerInitializationIpConfigArgs(
ipv4=proxmoxve.ct.ContainerInitializationIpConfigIpv4Args(
address="string",
gateway="string",
),
ipv6=proxmoxve.ct.ContainerInitializationIpConfigIpv6Args(
address="string",
gateway="string",
),
)],
user_account=proxmoxve.ct.ContainerInitializationUserAccountArgs(
keys=["string"],
password="string",
),
),
memory=proxmoxve.ct.ContainerMemoryArgs(
dedicated=0,
swap=0,
),
mount_points=[proxmoxve.ct.ContainerMountPointArgs(
path="string",
volume="string",
acl=False,
backup=False,
mount_options=["string"],
quota=False,
read_only=False,
replicate=False,
shared=False,
size="string",
)],
network_interfaces=[proxmoxve.ct.ContainerNetworkInterfaceArgs(
name="string",
bridge="string",
enabled=False,
firewall=False,
mac_address="string",
mtu=0,
rate_limit=0,
vlan_id=0,
)],
console=proxmoxve.ct.ContainerConsoleArgs(
enabled=False,
tty_count=0,
type="string",
),
clone=proxmoxve.ct.ContainerCloneArgs(
vm_id=0,
datastore_id="string",
node_name="string",
),
vm_id=0,
cpu=proxmoxve.ct.ContainerCpuArgs(
architecture="string",
cores=0,
units=0,
),
disk=proxmoxve.ct.ContainerDiskArgs(
datastore_id="string",
size=0,
),
started=False,
startup=proxmoxve.ct.ContainerStartupArgs(
down_delay=0,
order=0,
up_delay=0,
),
tags=["string"],
template=False,
timeout_clone=0,
timeout_create=0,
timeout_delete=0,
timeout_update=0,
unprivileged=False,
pool_id="string")
const containerResource = new proxmoxve.ct.Container("containerResource", {
nodeName: "string",
operatingSystem: {
templateFileId: "string",
type: "string",
},
description: "string",
protection: false,
startOnBoot: false,
features: {
fuse: false,
keyctl: false,
mounts: ["string"],
nesting: false,
},
hookScriptFileId: "string",
initialization: {
dns: {
domain: "string",
servers: ["string"],
},
hostname: "string",
ipConfigs: [{
ipv4: {
address: "string",
gateway: "string",
},
ipv6: {
address: "string",
gateway: "string",
},
}],
userAccount: {
keys: ["string"],
password: "string",
},
},
memory: {
dedicated: 0,
swap: 0,
},
mountPoints: [{
path: "string",
volume: "string",
acl: false,
backup: false,
mountOptions: ["string"],
quota: false,
readOnly: false,
replicate: false,
shared: false,
size: "string",
}],
networkInterfaces: [{
name: "string",
bridge: "string",
enabled: false,
firewall: false,
macAddress: "string",
mtu: 0,
rateLimit: 0,
vlanId: 0,
}],
console: {
enabled: false,
ttyCount: 0,
type: "string",
},
clone: {
vmId: 0,
datastoreId: "string",
nodeName: "string",
},
vmId: 0,
cpu: {
architecture: "string",
cores: 0,
units: 0,
},
disk: {
datastoreId: "string",
size: 0,
},
started: false,
startup: {
downDelay: 0,
order: 0,
upDelay: 0,
},
tags: ["string"],
template: false,
timeoutClone: 0,
timeoutCreate: 0,
timeoutDelete: 0,
timeoutUpdate: 0,
unprivileged: false,
poolId: "string",
});
type: proxmoxve:CT:Container
properties:
clone:
datastoreId: string
nodeName: string
vmId: 0
console:
enabled: false
ttyCount: 0
type: string
cpu:
architecture: string
cores: 0
units: 0
description: string
disk:
datastoreId: string
size: 0
features:
fuse: false
keyctl: false
mounts:
- string
nesting: false
hookScriptFileId: string
initialization:
dns:
domain: string
servers:
- string
hostname: string
ipConfigs:
- ipv4:
address: string
gateway: string
ipv6:
address: string
gateway: string
userAccount:
keys:
- string
password: string
memory:
dedicated: 0
swap: 0
mountPoints:
- acl: false
backup: false
mountOptions:
- string
path: string
quota: false
readOnly: false
replicate: false
shared: false
size: string
volume: string
networkInterfaces:
- bridge: string
enabled: false
firewall: false
macAddress: string
mtu: 0
name: string
rateLimit: 0
vlanId: 0
nodeName: string
operatingSystem:
templateFileId: string
type: string
poolId: string
protection: false
startOnBoot: false
started: false
startup:
downDelay: 0
order: 0
upDelay: 0
tags:
- string
template: false
timeoutClone: 0
timeoutCreate: 0
timeoutDelete: 0
timeoutUpdate: 0
unprivileged: false
vmId: 0
Container Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The Container resource accepts the following input properties:
- Node
Name string - The name of the node to assign the container to.
- Clone
Pulumi.
Proxmox VE. CT. Inputs. Container Clone - The cloning configuration.
- Console
Pulumi.
Proxmox VE. CT. Inputs. Container Console - The console configuration.
- Cpu
Pulumi.
Proxmox VE. CT. Inputs. Container Cpu - The CPU configuration.
- Description string
- The description.
- Disk
Pulumi.
Proxmox VE. CT. Inputs. Container Disk - The disk configuration.
- Features
Pulumi.
Proxmox VE. CT. Inputs. Container Features - The container feature flags. Changing flags (except nesting) is only allowed for
root@pam
authenticated user. - Hook
Script stringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - Initialization
Pulumi.
Proxmox VE. CT. Inputs. Container Initialization - The initialization configuration.
- Memory
Pulumi.
Proxmox VE. CT. Inputs. Container Memory - The memory configuration.
- Mount
Points List<Pulumi.Proxmox VE. CT. Inputs. Container Mount Point> - A mount point
- Network
Interfaces List<Pulumi.Proxmox VE. CT. Inputs. Container Network Interface> - A network interface (multiple blocks supported).
- Operating
System Pulumi.Proxmox VE. CT. Inputs. Container Operating System - The Operating System configuration.
- Pool
Id string - The identifier for a pool to assign the container to.
- Protection bool
- Whether to set the protection flag of the container (defaults to
false
). This will prevent the container itself and its disk for remove/update operations. - Start
On boolBoot - Automatically start container when the host
system boots (defaults to
true
). - Started bool
- Whether to start the container (defaults to
true
). - Startup
Pulumi.
Proxmox VE. CT. Inputs. Container Startup - Defines startup and shutdown behavior of the container.
- List<string>
- A list of tags the container tags. This is only meta
information (defaults to
[]
). Note: Proxmox always sorts the container tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - Template bool
- Whether to create a template (defaults to
false
). - Timeout
Clone int - Timeout for cloning a container in seconds (defaults to 1800).
- Timeout
Create int - Timeout for creating a container in seconds (defaults to 1800).
- Timeout
Delete int - Timeout for deleting a container in seconds (defaults to 60).
- Timeout
Start int - Start container timeout
- Timeout
Update int - Timeout for updating a container in seconds (defaults to 1800).
- Unprivileged bool
- Whether the container runs as unprivileged on
the host (defaults to
false
). - Vm
Id int - The container identifier
- Node
Name string - The name of the node to assign the container to.
- Clone
Container
Clone Args - The cloning configuration.
- Console
Container
Console Args - The console configuration.
- Cpu
Container
Cpu Args - The CPU configuration.
- Description string
- The description.
- Disk
Container
Disk Args - The disk configuration.
- Features
Container
Features Args - The container feature flags. Changing flags (except nesting) is only allowed for
root@pam
authenticated user. - Hook
Script stringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - Initialization
Container
Initialization Args - The initialization configuration.
- Memory
Container
Memory Args - The memory configuration.
- Mount
Points []ContainerMount Point Args - A mount point
- Network
Interfaces []ContainerNetwork Interface Args - A network interface (multiple blocks supported).
- Operating
System ContainerOperating System Args - The Operating System configuration.
- Pool
Id string - The identifier for a pool to assign the container to.
- Protection bool
- Whether to set the protection flag of the container (defaults to
false
). This will prevent the container itself and its disk for remove/update operations. - Start
On boolBoot - Automatically start container when the host
system boots (defaults to
true
). - Started bool
- Whether to start the container (defaults to
true
). - Startup
Container
Startup Args - Defines startup and shutdown behavior of the container.
- []string
- A list of tags the container tags. This is only meta
information (defaults to
[]
). Note: Proxmox always sorts the container tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - Template bool
- Whether to create a template (defaults to
false
). - Timeout
Clone int - Timeout for cloning a container in seconds (defaults to 1800).
- Timeout
Create int - Timeout for creating a container in seconds (defaults to 1800).
- Timeout
Delete int - Timeout for deleting a container in seconds (defaults to 60).
- Timeout
Start int - Start container timeout
- Timeout
Update int - Timeout for updating a container in seconds (defaults to 1800).
- Unprivileged bool
- Whether the container runs as unprivileged on
the host (defaults to
false
). - Vm
Id int - The container identifier
- node
Name String - The name of the node to assign the container to.
- clone_
Container
Clone - The cloning configuration.
- console
Container
Console - The console configuration.
- cpu
Container
Cpu - The CPU configuration.
- description String
- The description.
- disk
Container
Disk - The disk configuration.
- features
Container
Features - The container feature flags. Changing flags (except nesting) is only allowed for
root@pam
authenticated user. - hook
Script StringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - initialization
Container
Initialization - The initialization configuration.
- memory
Container
Memory - The memory configuration.
- mount
Points List<ContainerMount Point> - A mount point
- network
Interfaces List<ContainerNetwork Interface> - A network interface (multiple blocks supported).
- operating
System ContainerOperating System - The Operating System configuration.
- pool
Id String - The identifier for a pool to assign the container to.
- protection Boolean
- Whether to set the protection flag of the container (defaults to
false
). This will prevent the container itself and its disk for remove/update operations. - start
On BooleanBoot - Automatically start container when the host
system boots (defaults to
true
). - started Boolean
- Whether to start the container (defaults to
true
). - startup
Container
Startup - Defines startup and shutdown behavior of the container.
- List<String>
- A list of tags the container tags. This is only meta
information (defaults to
[]
). Note: Proxmox always sorts the container tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template Boolean
- Whether to create a template (defaults to
false
). - timeout
Clone Integer - Timeout for cloning a container in seconds (defaults to 1800).
- timeout
Create Integer - Timeout for creating a container in seconds (defaults to 1800).
- timeout
Delete Integer - Timeout for deleting a container in seconds (defaults to 60).
- timeout
Start Integer - Start container timeout
- timeout
Update Integer - Timeout for updating a container in seconds (defaults to 1800).
- unprivileged Boolean
- Whether the container runs as unprivileged on
the host (defaults to
false
). - vm
Id Integer - The container identifier
- node
Name string - The name of the node to assign the container to.
- clone
Container
Clone - The cloning configuration.
- console
Container
Console - The console configuration.
- cpu
Container
Cpu - The CPU configuration.
- description string
- The description.
- disk
Container
Disk - The disk configuration.
- features
Container
Features - The container feature flags. Changing flags (except nesting) is only allowed for
root@pam
authenticated user. - hook
Script stringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - initialization
Container
Initialization - The initialization configuration.
- memory
Container
Memory - The memory configuration.
- mount
Points ContainerMount Point[] - A mount point
- network
Interfaces ContainerNetwork Interface[] - A network interface (multiple blocks supported).
- operating
System ContainerOperating System - The Operating System configuration.
- pool
Id string - The identifier for a pool to assign the container to.
- protection boolean
- Whether to set the protection flag of the container (defaults to
false
). This will prevent the container itself and its disk for remove/update operations. - start
On booleanBoot - Automatically start container when the host
system boots (defaults to
true
). - started boolean
- Whether to start the container (defaults to
true
). - startup
Container
Startup - Defines startup and shutdown behavior of the container.
- string[]
- A list of tags the container tags. This is only meta
information (defaults to
[]
). Note: Proxmox always sorts the container tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template boolean
- Whether to create a template (defaults to
false
). - timeout
Clone number - Timeout for cloning a container in seconds (defaults to 1800).
- timeout
Create number - Timeout for creating a container in seconds (defaults to 1800).
- timeout
Delete number - Timeout for deleting a container in seconds (defaults to 60).
- timeout
Start number - Start container timeout
- timeout
Update number - Timeout for updating a container in seconds (defaults to 1800).
- unprivileged boolean
- Whether the container runs as unprivileged on
the host (defaults to
false
). - vm
Id number - The container identifier
- node_
name str - The name of the node to assign the container to.
- clone
ct.
Container Clone Args - The cloning configuration.
- console
ct.
Container Console Args - The console configuration.
- cpu
ct.
Container Cpu Args - The CPU configuration.
- description str
- The description.
- disk
ct.
Container Disk Args - The disk configuration.
- features
ct.
Container Features Args - The container feature flags. Changing flags (except nesting) is only allowed for
root@pam
authenticated user. - hook_
script_ strfile_ id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - initialization
ct.
Container Initialization Args - The initialization configuration.
- memory
ct.
Container Memory Args - The memory configuration.
- mount_
points Sequence[ct.Container Mount Point Args] - A mount point
- network_
interfaces Sequence[ct.Container Network Interface Args] - A network interface (multiple blocks supported).
- operating_
system ct.Container Operating System Args - The Operating System configuration.
- pool_
id str - The identifier for a pool to assign the container to.
- protection bool
- Whether to set the protection flag of the container (defaults to
false
). This will prevent the container itself and its disk for remove/update operations. - start_
on_ boolboot - Automatically start container when the host
system boots (defaults to
true
). - started bool
- Whether to start the container (defaults to
true
). - startup
ct.
Container Startup Args - Defines startup and shutdown behavior of the container.
- Sequence[str]
- A list of tags the container tags. This is only meta
information (defaults to
[]
). Note: Proxmox always sorts the container tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template bool
- Whether to create a template (defaults to
false
). - timeout_
clone int - Timeout for cloning a container in seconds (defaults to 1800).
- timeout_
create int - Timeout for creating a container in seconds (defaults to 1800).
- timeout_
delete int - Timeout for deleting a container in seconds (defaults to 60).
- timeout_
start int - Start container timeout
- timeout_
update int - Timeout for updating a container in seconds (defaults to 1800).
- unprivileged bool
- Whether the container runs as unprivileged on
the host (defaults to
false
). - vm_
id int - The container identifier
- node
Name String - The name of the node to assign the container to.
- clone Property Map
- The cloning configuration.
- console Property Map
- The console configuration.
- cpu Property Map
- The CPU configuration.
- description String
- The description.
- disk Property Map
- The disk configuration.
- features Property Map
- The container feature flags. Changing flags (except nesting) is only allowed for
root@pam
authenticated user. - hook
Script StringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - initialization Property Map
- The initialization configuration.
- memory Property Map
- The memory configuration.
- mount
Points List<Property Map> - A mount point
- network
Interfaces List<Property Map> - A network interface (multiple blocks supported).
- operating
System Property Map - The Operating System configuration.
- pool
Id String - The identifier for a pool to assign the container to.
- protection Boolean
- Whether to set the protection flag of the container (defaults to
false
). This will prevent the container itself and its disk for remove/update operations. - start
On BooleanBoot - Automatically start container when the host
system boots (defaults to
true
). - started Boolean
- Whether to start the container (defaults to
true
). - startup Property Map
- Defines startup and shutdown behavior of the container.
- List<String>
- A list of tags the container tags. This is only meta
information (defaults to
[]
). Note: Proxmox always sorts the container tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template Boolean
- Whether to create a template (defaults to
false
). - timeout
Clone Number - Timeout for cloning a container in seconds (defaults to 1800).
- timeout
Create Number - Timeout for creating a container in seconds (defaults to 1800).
- timeout
Delete Number - Timeout for deleting a container in seconds (defaults to 60).
- timeout
Start Number - Start container timeout
- timeout
Update Number - Timeout for updating a container in seconds (defaults to 1800).
- unprivileged Boolean
- Whether the container runs as unprivileged on
the host (defaults to
false
). - vm
Id Number - The container identifier
Outputs
All input properties are implicitly available as output properties. Additionally, the Container resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing Container Resource
Get an existing Container resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: ContainerState, opts?: CustomResourceOptions): Container
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
clone: Optional[_ct.ContainerCloneArgs] = None,
console: Optional[_ct.ContainerConsoleArgs] = None,
cpu: Optional[_ct.ContainerCpuArgs] = None,
description: Optional[str] = None,
disk: Optional[_ct.ContainerDiskArgs] = None,
features: Optional[_ct.ContainerFeaturesArgs] = None,
hook_script_file_id: Optional[str] = None,
initialization: Optional[_ct.ContainerInitializationArgs] = None,
memory: Optional[_ct.ContainerMemoryArgs] = None,
mount_points: Optional[Sequence[_ct.ContainerMountPointArgs]] = None,
network_interfaces: Optional[Sequence[_ct.ContainerNetworkInterfaceArgs]] = None,
node_name: Optional[str] = None,
operating_system: Optional[_ct.ContainerOperatingSystemArgs] = None,
pool_id: Optional[str] = None,
protection: Optional[bool] = None,
start_on_boot: Optional[bool] = None,
started: Optional[bool] = None,
startup: Optional[_ct.ContainerStartupArgs] = None,
tags: Optional[Sequence[str]] = None,
template: Optional[bool] = None,
timeout_clone: Optional[int] = None,
timeout_create: Optional[int] = None,
timeout_delete: Optional[int] = None,
timeout_start: Optional[int] = None,
timeout_update: Optional[int] = None,
unprivileged: Optional[bool] = None,
vm_id: Optional[int] = None) -> Container
func GetContainer(ctx *Context, name string, id IDInput, state *ContainerState, opts ...ResourceOption) (*Container, error)
public static Container Get(string name, Input<string> id, ContainerState? state, CustomResourceOptions? opts = null)
public static Container get(String name, Output<String> id, ContainerState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Clone
Pulumi.
Proxmox VE. CT. Inputs. Container Clone - The cloning configuration.
- Console
Pulumi.
Proxmox VE. CT. Inputs. Container Console - The console configuration.
- Cpu
Pulumi.
Proxmox VE. CT. Inputs. Container Cpu - The CPU configuration.
- Description string
- The description.
- Disk
Pulumi.
Proxmox VE. CT. Inputs. Container Disk - The disk configuration.
- Features
Pulumi.
Proxmox VE. CT. Inputs. Container Features - The container feature flags. Changing flags (except nesting) is only allowed for
root@pam
authenticated user. - Hook
Script stringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - Initialization
Pulumi.
Proxmox VE. CT. Inputs. Container Initialization - The initialization configuration.
- Memory
Pulumi.
Proxmox VE. CT. Inputs. Container Memory - The memory configuration.
- Mount
Points List<Pulumi.Proxmox VE. CT. Inputs. Container Mount Point> - A mount point
- Network
Interfaces List<Pulumi.Proxmox VE. CT. Inputs. Container Network Interface> - A network interface (multiple blocks supported).
- Node
Name string - The name of the node to assign the container to.
- Operating
System Pulumi.Proxmox VE. CT. Inputs. Container Operating System - The Operating System configuration.
- Pool
Id string - The identifier for a pool to assign the container to.
- Protection bool
- Whether to set the protection flag of the container (defaults to
false
). This will prevent the container itself and its disk for remove/update operations. - Start
On boolBoot - Automatically start container when the host
system boots (defaults to
true
). - Started bool
- Whether to start the container (defaults to
true
). - Startup
Pulumi.
Proxmox VE. CT. Inputs. Container Startup - Defines startup and shutdown behavior of the container.
- List<string>
- A list of tags the container tags. This is only meta
information (defaults to
[]
). Note: Proxmox always sorts the container tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - Template bool
- Whether to create a template (defaults to
false
). - Timeout
Clone int - Timeout for cloning a container in seconds (defaults to 1800).
- Timeout
Create int - Timeout for creating a container in seconds (defaults to 1800).
- Timeout
Delete int - Timeout for deleting a container in seconds (defaults to 60).
- Timeout
Start int - Start container timeout
- Timeout
Update int - Timeout for updating a container in seconds (defaults to 1800).
- Unprivileged bool
- Whether the container runs as unprivileged on
the host (defaults to
false
). - Vm
Id int - The container identifier
- Clone
Container
Clone Args - The cloning configuration.
- Console
Container
Console Args - The console configuration.
- Cpu
Container
Cpu Args - The CPU configuration.
- Description string
- The description.
- Disk
Container
Disk Args - The disk configuration.
- Features
Container
Features Args - The container feature flags. Changing flags (except nesting) is only allowed for
root@pam
authenticated user. - Hook
Script stringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - Initialization
Container
Initialization Args - The initialization configuration.
- Memory
Container
Memory Args - The memory configuration.
- Mount
Points []ContainerMount Point Args - A mount point
- Network
Interfaces []ContainerNetwork Interface Args - A network interface (multiple blocks supported).
- Node
Name string - The name of the node to assign the container to.
- Operating
System ContainerOperating System Args - The Operating System configuration.
- Pool
Id string - The identifier for a pool to assign the container to.
- Protection bool
- Whether to set the protection flag of the container (defaults to
false
). This will prevent the container itself and its disk for remove/update operations. - Start
On boolBoot - Automatically start container when the host
system boots (defaults to
true
). - Started bool
- Whether to start the container (defaults to
true
). - Startup
Container
Startup Args - Defines startup and shutdown behavior of the container.
- []string
- A list of tags the container tags. This is only meta
information (defaults to
[]
). Note: Proxmox always sorts the container tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - Template bool
- Whether to create a template (defaults to
false
). - Timeout
Clone int - Timeout for cloning a container in seconds (defaults to 1800).
- Timeout
Create int - Timeout for creating a container in seconds (defaults to 1800).
- Timeout
Delete int - Timeout for deleting a container in seconds (defaults to 60).
- Timeout
Start int - Start container timeout
- Timeout
Update int - Timeout for updating a container in seconds (defaults to 1800).
- Unprivileged bool
- Whether the container runs as unprivileged on
the host (defaults to
false
). - Vm
Id int - The container identifier
- clone_
Container
Clone - The cloning configuration.
- console
Container
Console - The console configuration.
- cpu
Container
Cpu - The CPU configuration.
- description String
- The description.
- disk
Container
Disk - The disk configuration.
- features
Container
Features - The container feature flags. Changing flags (except nesting) is only allowed for
root@pam
authenticated user. - hook
Script StringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - initialization
Container
Initialization - The initialization configuration.
- memory
Container
Memory - The memory configuration.
- mount
Points List<ContainerMount Point> - A mount point
- network
Interfaces List<ContainerNetwork Interface> - A network interface (multiple blocks supported).
- node
Name String - The name of the node to assign the container to.
- operating
System ContainerOperating System - The Operating System configuration.
- pool
Id String - The identifier for a pool to assign the container to.
- protection Boolean
- Whether to set the protection flag of the container (defaults to
false
). This will prevent the container itself and its disk for remove/update operations. - start
On BooleanBoot - Automatically start container when the host
system boots (defaults to
true
). - started Boolean
- Whether to start the container (defaults to
true
). - startup
Container
Startup - Defines startup and shutdown behavior of the container.
- List<String>
- A list of tags the container tags. This is only meta
information (defaults to
[]
). Note: Proxmox always sorts the container tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template Boolean
- Whether to create a template (defaults to
false
). - timeout
Clone Integer - Timeout for cloning a container in seconds (defaults to 1800).
- timeout
Create Integer - Timeout for creating a container in seconds (defaults to 1800).
- timeout
Delete Integer - Timeout for deleting a container in seconds (defaults to 60).
- timeout
Start Integer - Start container timeout
- timeout
Update Integer - Timeout for updating a container in seconds (defaults to 1800).
- unprivileged Boolean
- Whether the container runs as unprivileged on
the host (defaults to
false
). - vm
Id Integer - The container identifier
- clone
Container
Clone - The cloning configuration.
- console
Container
Console - The console configuration.
- cpu
Container
Cpu - The CPU configuration.
- description string
- The description.
- disk
Container
Disk - The disk configuration.
- features
Container
Features - The container feature flags. Changing flags (except nesting) is only allowed for
root@pam
authenticated user. - hook
Script stringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - initialization
Container
Initialization - The initialization configuration.
- memory
Container
Memory - The memory configuration.
- mount
Points ContainerMount Point[] - A mount point
- network
Interfaces ContainerNetwork Interface[] - A network interface (multiple blocks supported).
- node
Name string - The name of the node to assign the container to.
- operating
System ContainerOperating System - The Operating System configuration.
- pool
Id string - The identifier for a pool to assign the container to.
- protection boolean
- Whether to set the protection flag of the container (defaults to
false
). This will prevent the container itself and its disk for remove/update operations. - start
On booleanBoot - Automatically start container when the host
system boots (defaults to
true
). - started boolean
- Whether to start the container (defaults to
true
). - startup
Container
Startup - Defines startup and shutdown behavior of the container.
- string[]
- A list of tags the container tags. This is only meta
information (defaults to
[]
). Note: Proxmox always sorts the container tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template boolean
- Whether to create a template (defaults to
false
). - timeout
Clone number - Timeout for cloning a container in seconds (defaults to 1800).
- timeout
Create number - Timeout for creating a container in seconds (defaults to 1800).
- timeout
Delete number - Timeout for deleting a container in seconds (defaults to 60).
- timeout
Start number - Start container timeout
- timeout
Update number - Timeout for updating a container in seconds (defaults to 1800).
- unprivileged boolean
- Whether the container runs as unprivileged on
the host (defaults to
false
). - vm
Id number - The container identifier
- clone
ct.
Container Clone Args - The cloning configuration.
- console
ct.
Container Console Args - The console configuration.
- cpu
ct.
Container Cpu Args - The CPU configuration.
- description str
- The description.
- disk
ct.
Container Disk Args - The disk configuration.
- features
ct.
Container Features Args - The container feature flags. Changing flags (except nesting) is only allowed for
root@pam
authenticated user. - hook_
script_ strfile_ id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - initialization
ct.
Container Initialization Args - The initialization configuration.
- memory
ct.
Container Memory Args - The memory configuration.
- mount_
points Sequence[ct.Container Mount Point Args] - A mount point
- network_
interfaces Sequence[ct.Container Network Interface Args] - A network interface (multiple blocks supported).
- node_
name str - The name of the node to assign the container to.
- operating_
system ct.Container Operating System Args - The Operating System configuration.
- pool_
id str - The identifier for a pool to assign the container to.
- protection bool
- Whether to set the protection flag of the container (defaults to
false
). This will prevent the container itself and its disk for remove/update operations. - start_
on_ boolboot - Automatically start container when the host
system boots (defaults to
true
). - started bool
- Whether to start the container (defaults to
true
). - startup
ct.
Container Startup Args - Defines startup and shutdown behavior of the container.
- Sequence[str]
- A list of tags the container tags. This is only meta
information (defaults to
[]
). Note: Proxmox always sorts the container tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template bool
- Whether to create a template (defaults to
false
). - timeout_
clone int - Timeout for cloning a container in seconds (defaults to 1800).
- timeout_
create int - Timeout for creating a container in seconds (defaults to 1800).
- timeout_
delete int - Timeout for deleting a container in seconds (defaults to 60).
- timeout_
start int - Start container timeout
- timeout_
update int - Timeout for updating a container in seconds (defaults to 1800).
- unprivileged bool
- Whether the container runs as unprivileged on
the host (defaults to
false
). - vm_
id int - The container identifier
- clone Property Map
- The cloning configuration.
- console Property Map
- The console configuration.
- cpu Property Map
- The CPU configuration.
- description String
- The description.
- disk Property Map
- The disk configuration.
- features Property Map
- The container feature flags. Changing flags (except nesting) is only allowed for
root@pam
authenticated user. - hook
Script StringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - initialization Property Map
- The initialization configuration.
- memory Property Map
- The memory configuration.
- mount
Points List<Property Map> - A mount point
- network
Interfaces List<Property Map> - A network interface (multiple blocks supported).
- node
Name String - The name of the node to assign the container to.
- operating
System Property Map - The Operating System configuration.
- pool
Id String - The identifier for a pool to assign the container to.
- protection Boolean
- Whether to set the protection flag of the container (defaults to
false
). This will prevent the container itself and its disk for remove/update operations. - start
On BooleanBoot - Automatically start container when the host
system boots (defaults to
true
). - started Boolean
- Whether to start the container (defaults to
true
). - startup Property Map
- Defines startup and shutdown behavior of the container.
- List<String>
- A list of tags the container tags. This is only meta
information (defaults to
[]
). Note: Proxmox always sorts the container tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template Boolean
- Whether to create a template (defaults to
false
). - timeout
Clone Number - Timeout for cloning a container in seconds (defaults to 1800).
- timeout
Create Number - Timeout for creating a container in seconds (defaults to 1800).
- timeout
Delete Number - Timeout for deleting a container in seconds (defaults to 60).
- timeout
Start Number - Start container timeout
- timeout
Update Number - Timeout for updating a container in seconds (defaults to 1800).
- unprivileged Boolean
- Whether the container runs as unprivileged on
the host (defaults to
false
). - vm
Id Number - The container identifier
Supporting Types
ContainerClone, ContainerCloneArgs
- Vm
Id int - The identifier for the source container.
- Datastore
Id string - The identifier for the target datastore.
- Node
Name string - The name of the source node (leave blank, if
equal to the
node_name
argument).
- Vm
Id int - The identifier for the source container.
- Datastore
Id string - The identifier for the target datastore.
- Node
Name string - The name of the source node (leave blank, if
equal to the
node_name
argument).
- vm
Id Integer - The identifier for the source container.
- datastore
Id String - The identifier for the target datastore.
- node
Name String - The name of the source node (leave blank, if
equal to the
node_name
argument).
- vm
Id number - The identifier for the source container.
- datastore
Id string - The identifier for the target datastore.
- node
Name string - The name of the source node (leave blank, if
equal to the
node_name
argument).
- vm_
id int - The identifier for the source container.
- datastore_
id str - The identifier for the target datastore.
- node_
name str - The name of the source node (leave blank, if
equal to the
node_name
argument).
- vm
Id Number - The identifier for the source container.
- datastore
Id String - The identifier for the target datastore.
- node
Name String - The name of the source node (leave blank, if
equal to the
node_name
argument).
ContainerConsole, ContainerConsoleArgs
ContainerCpu, ContainerCpuArgs
- Architecture string
- The CPU architecture (defaults to
amd64
). - Cores int
- The number of CPU cores (defaults to
1
). - Units int
- The CPU units (defaults to
1024
).
- Architecture string
- The CPU architecture (defaults to
amd64
). - Cores int
- The number of CPU cores (defaults to
1
). - Units int
- The CPU units (defaults to
1024
).
- architecture String
- The CPU architecture (defaults to
amd64
). - cores Integer
- The number of CPU cores (defaults to
1
). - units Integer
- The CPU units (defaults to
1024
).
- architecture string
- The CPU architecture (defaults to
amd64
). - cores number
- The number of CPU cores (defaults to
1
). - units number
- The CPU units (defaults to
1024
).
- architecture str
- The CPU architecture (defaults to
amd64
). - cores int
- The number of CPU cores (defaults to
1
). - units int
- The CPU units (defaults to
1024
).
- architecture String
- The CPU architecture (defaults to
amd64
). - cores Number
- The number of CPU cores (defaults to
1
). - units Number
- The CPU units (defaults to
1024
).
ContainerDisk, ContainerDiskArgs
- Datastore
Id string - The identifier for the datastore to create the
disk in (defaults to
local
). - Size int
- The size of the root filesystem in gigabytes (defaults
to
4
). Requiresdatastore_id
to be set.
- Datastore
Id string - The identifier for the datastore to create the
disk in (defaults to
local
). - Size int
- The size of the root filesystem in gigabytes (defaults
to
4
). Requiresdatastore_id
to be set.
- datastore
Id String - The identifier for the datastore to create the
disk in (defaults to
local
). - size Integer
- The size of the root filesystem in gigabytes (defaults
to
4
). Requiresdatastore_id
to be set.
- datastore
Id string - The identifier for the datastore to create the
disk in (defaults to
local
). - size number
- The size of the root filesystem in gigabytes (defaults
to
4
). Requiresdatastore_id
to be set.
- datastore_
id str - The identifier for the datastore to create the
disk in (defaults to
local
). - size int
- The size of the root filesystem in gigabytes (defaults
to
4
). Requiresdatastore_id
to be set.
- datastore
Id String - The identifier for the datastore to create the
disk in (defaults to
local
). - size Number
- The size of the root filesystem in gigabytes (defaults
to
4
). Requiresdatastore_id
to be set.
ContainerFeatures, ContainerFeaturesArgs
ContainerInitialization, ContainerInitializationArgs
- Dns
Pulumi.
Proxmox VE. CT. Inputs. Container Initialization Dns - The DNS configuration.
- Hostname string
- The hostname.
- Ip
Configs List<Pulumi.Proxmox VE. CT. Inputs. Container Initialization Ip Config> - The IP configuration (one block per network device).
- User
Account Pulumi.Proxmox VE. CT. Inputs. Container Initialization User Account - The user account configuration.
- Dns
Container
Initialization Dns - The DNS configuration.
- Hostname string
- The hostname.
- Ip
Configs []ContainerInitialization Ip Config - The IP configuration (one block per network device).
- User
Account ContainerInitialization User Account - The user account configuration.
- dns
Container
Initialization Dns - The DNS configuration.
- hostname String
- The hostname.
- ip
Configs List<ContainerInitialization Ip Config> - The IP configuration (one block per network device).
- user
Account ContainerInitialization User Account - The user account configuration.
- dns
Container
Initialization Dns - The DNS configuration.
- hostname string
- The hostname.
- ip
Configs ContainerInitialization Ip Config[] - The IP configuration (one block per network device).
- user
Account ContainerInitialization User Account - The user account configuration.
- dns
ct.
Container Initialization Dns - The DNS configuration.
- hostname str
- The hostname.
- ip_
configs Sequence[ct.Container Initialization Ip Config] - The IP configuration (one block per network device).
- user_
account ct.Container Initialization User Account - The user account configuration.
- dns Property Map
- The DNS configuration.
- hostname String
- The hostname.
- ip
Configs List<Property Map> - The IP configuration (one block per network device).
- user
Account Property Map - The user account configuration.
ContainerInitializationDns, ContainerInitializationDnsArgs
ContainerInitializationIpConfig, ContainerInitializationIpConfigArgs
- Ipv4
Pulumi.
Proxmox VE. CT. Inputs. Container Initialization Ip Config Ipv4 - The IPv4 configuration.
- Ipv6
Pulumi.
Proxmox VE. CT. Inputs. Container Initialization Ip Config Ipv6 - The IPv4 configuration.
- Ipv4
Container
Initialization Ip Config Ipv4 - The IPv4 configuration.
- Ipv6
Container
Initialization Ip Config Ipv6 - The IPv4 configuration.
- ipv4
Container
Initialization Ip Config Ipv4 - The IPv4 configuration.
- ipv6
Container
Initialization Ip Config Ipv6 - The IPv4 configuration.
- ipv4
Container
Initialization Ip Config Ipv4 - The IPv4 configuration.
- ipv6
Container
Initialization Ip Config Ipv6 - The IPv4 configuration.
- ipv4
ct.
Container Initialization Ip Config Ipv4 - The IPv4 configuration.
- ipv6
ct.
Container Initialization Ip Config Ipv6 - The IPv4 configuration.
- ipv4 Property Map
- The IPv4 configuration.
- ipv6 Property Map
- The IPv4 configuration.
ContainerInitializationIpConfigIpv4, ContainerInitializationIpConfigIpv4Args
ContainerInitializationIpConfigIpv6, ContainerInitializationIpConfigIpv6Args
ContainerInitializationUserAccount, ContainerInitializationUserAccountArgs
ContainerMemory, ContainerMemoryArgs
ContainerMountPoint, ContainerMountPointArgs
- Path string
- Path to the mount point as seen from inside the container.
- Volume string
- Volume, device or directory to mount into the container.
- Acl bool
- Explicitly enable or disable ACL support.
- Backup bool
- Whether to include the mount point in backups (only
used for volume mount points, defaults to
false
). - Mount
Options List<string> - List of extra mount options.
- Quota bool
- Enable user quotas inside the container (not supported with ZFS subvolumes).
- Read
Only bool - Read-only mount point.
- Replicate bool
- Will include this volume to a storage replica job.
- bool
- Mark this non-volume mount point as available on all nodes.
- Size string
- Volume size (only for volume mount points).
Can be specified with a unit suffix (e.g.
10G
).
- Path string
- Path to the mount point as seen from inside the container.
- Volume string
- Volume, device or directory to mount into the container.
- Acl bool
- Explicitly enable or disable ACL support.
- Backup bool
- Whether to include the mount point in backups (only
used for volume mount points, defaults to
false
). - Mount
Options []string - List of extra mount options.
- Quota bool
- Enable user quotas inside the container (not supported with ZFS subvolumes).
- Read
Only bool - Read-only mount point.
- Replicate bool
- Will include this volume to a storage replica job.
- bool
- Mark this non-volume mount point as available on all nodes.
- Size string
- Volume size (only for volume mount points).
Can be specified with a unit suffix (e.g.
10G
).
- path String
- Path to the mount point as seen from inside the container.
- volume String
- Volume, device or directory to mount into the container.
- acl Boolean
- Explicitly enable or disable ACL support.
- backup Boolean
- Whether to include the mount point in backups (only
used for volume mount points, defaults to
false
). - mount
Options List<String> - List of extra mount options.
- quota Boolean
- Enable user quotas inside the container (not supported with ZFS subvolumes).
- read
Only Boolean - Read-only mount point.
- replicate Boolean
- Will include this volume to a storage replica job.
- Boolean
- Mark this non-volume mount point as available on all nodes.
- size String
- Volume size (only for volume mount points).
Can be specified with a unit suffix (e.g.
10G
).
- path string
- Path to the mount point as seen from inside the container.
- volume string
- Volume, device or directory to mount into the container.
- acl boolean
- Explicitly enable or disable ACL support.
- backup boolean
- Whether to include the mount point in backups (only
used for volume mount points, defaults to
false
). - mount
Options string[] - List of extra mount options.
- quota boolean
- Enable user quotas inside the container (not supported with ZFS subvolumes).
- read
Only boolean - Read-only mount point.
- replicate boolean
- Will include this volume to a storage replica job.
- boolean
- Mark this non-volume mount point as available on all nodes.
- size string
- Volume size (only for volume mount points).
Can be specified with a unit suffix (e.g.
10G
).
- path str
- Path to the mount point as seen from inside the container.
- volume str
- Volume, device or directory to mount into the container.
- acl bool
- Explicitly enable or disable ACL support.
- backup bool
- Whether to include the mount point in backups (only
used for volume mount points, defaults to
false
). - mount_
options Sequence[str] - List of extra mount options.
- quota bool
- Enable user quotas inside the container (not supported with ZFS subvolumes).
- read_
only bool - Read-only mount point.
- replicate bool
- Will include this volume to a storage replica job.
- bool
- Mark this non-volume mount point as available on all nodes.
- size str
- Volume size (only for volume mount points).
Can be specified with a unit suffix (e.g.
10G
).
- path String
- Path to the mount point as seen from inside the container.
- volume String
- Volume, device or directory to mount into the container.
- acl Boolean
- Explicitly enable or disable ACL support.
- backup Boolean
- Whether to include the mount point in backups (only
used for volume mount points, defaults to
false
). - mount
Options List<String> - List of extra mount options.
- quota Boolean
- Enable user quotas inside the container (not supported with ZFS subvolumes).
- read
Only Boolean - Read-only mount point.
- replicate Boolean
- Will include this volume to a storage replica job.
- Boolean
- Mark this non-volume mount point as available on all nodes.
- size String
- Volume size (only for volume mount points).
Can be specified with a unit suffix (e.g.
10G
).
ContainerNetworkInterface, ContainerNetworkInterfaceArgs
- Name string
- The network interface name.
- Bridge string
- The name of the network bridge (defaults
to
vmbr0
). - Enabled bool
- Whether to enable the network device (defaults
to
true
). - Firewall bool
- Whether this interface's firewall rules should be
used (defaults to
false
). - Mac
Address string - The MAC address.
- Mtu int
- Maximum transfer unit of the interface. Cannot be larger than the bridge's MTU.
- Rate
Limit double - The rate limit in megabytes per second.
- Vlan
Id int - The VLAN identifier.
- Name string
- The network interface name.
- Bridge string
- The name of the network bridge (defaults
to
vmbr0
). - Enabled bool
- Whether to enable the network device (defaults
to
true
). - Firewall bool
- Whether this interface's firewall rules should be
used (defaults to
false
). - Mac
Address string - The MAC address.
- Mtu int
- Maximum transfer unit of the interface. Cannot be larger than the bridge's MTU.
- Rate
Limit float64 - The rate limit in megabytes per second.
- Vlan
Id int - The VLAN identifier.
- name String
- The network interface name.
- bridge String
- The name of the network bridge (defaults
to
vmbr0
). - enabled Boolean
- Whether to enable the network device (defaults
to
true
). - firewall Boolean
- Whether this interface's firewall rules should be
used (defaults to
false
). - mac
Address String - The MAC address.
- mtu Integer
- Maximum transfer unit of the interface. Cannot be larger than the bridge's MTU.
- rate
Limit Double - The rate limit in megabytes per second.
- vlan
Id Integer - The VLAN identifier.
- name string
- The network interface name.
- bridge string
- The name of the network bridge (defaults
to
vmbr0
). - enabled boolean
- Whether to enable the network device (defaults
to
true
). - firewall boolean
- Whether this interface's firewall rules should be
used (defaults to
false
). - mac
Address string - The MAC address.
- mtu number
- Maximum transfer unit of the interface. Cannot be larger than the bridge's MTU.
- rate
Limit number - The rate limit in megabytes per second.
- vlan
Id number - The VLAN identifier.
- name str
- The network interface name.
- bridge str
- The name of the network bridge (defaults
to
vmbr0
). - enabled bool
- Whether to enable the network device (defaults
to
true
). - firewall bool
- Whether this interface's firewall rules should be
used (defaults to
false
). - mac_
address str - The MAC address.
- mtu int
- Maximum transfer unit of the interface. Cannot be larger than the bridge's MTU.
- rate_
limit float - The rate limit in megabytes per second.
- vlan_
id int - The VLAN identifier.
- name String
- The network interface name.
- bridge String
- The name of the network bridge (defaults
to
vmbr0
). - enabled Boolean
- Whether to enable the network device (defaults
to
true
). - firewall Boolean
- Whether this interface's firewall rules should be
used (defaults to
false
). - mac
Address String - The MAC address.
- mtu Number
- Maximum transfer unit of the interface. Cannot be larger than the bridge's MTU.
- rate
Limit Number - The rate limit in megabytes per second.
- vlan
Id Number - The VLAN identifier.
ContainerOperatingSystem, ContainerOperatingSystemArgs
- Template
File stringId - The identifier for an OS template file.
The ID format is
<datastore_id>:<content_type>/<file_name>
, for examplelocal:iso/jammy-server-cloudimg-amd64.tar.gz
. Can be also taken fromproxmoxve.Download.File
resource, or from the output ofpvesm list <storage>
. - Type string
- The type (defaults to
unmanaged
).
- Template
File stringId - The identifier for an OS template file.
The ID format is
<datastore_id>:<content_type>/<file_name>
, for examplelocal:iso/jammy-server-cloudimg-amd64.tar.gz
. Can be also taken fromproxmoxve.Download.File
resource, or from the output ofpvesm list <storage>
. - Type string
- The type (defaults to
unmanaged
).
- template
File StringId - The identifier for an OS template file.
The ID format is
<datastore_id>:<content_type>/<file_name>
, for examplelocal:iso/jammy-server-cloudimg-amd64.tar.gz
. Can be also taken fromproxmoxve.Download.File
resource, or from the output ofpvesm list <storage>
. - type String
- The type (defaults to
unmanaged
).
- template
File stringId - The identifier for an OS template file.
The ID format is
<datastore_id>:<content_type>/<file_name>
, for examplelocal:iso/jammy-server-cloudimg-amd64.tar.gz
. Can be also taken fromproxmoxve.Download.File
resource, or from the output ofpvesm list <storage>
. - type string
- The type (defaults to
unmanaged
).
- template_
file_ strid - The identifier for an OS template file.
The ID format is
<datastore_id>:<content_type>/<file_name>
, for examplelocal:iso/jammy-server-cloudimg-amd64.tar.gz
. Can be also taken fromproxmoxve.Download.File
resource, or from the output ofpvesm list <storage>
. - type str
- The type (defaults to
unmanaged
).
- template
File StringId - The identifier for an OS template file.
The ID format is
<datastore_id>:<content_type>/<file_name>
, for examplelocal:iso/jammy-server-cloudimg-amd64.tar.gz
. Can be also taken fromproxmoxve.Download.File
resource, or from the output ofpvesm list <storage>
. - type String
- The type (defaults to
unmanaged
).
ContainerStartup, ContainerStartupArgs
- down_
delay int - A non-negative number defining the delay in seconds before the next container is shut down.
- order int
- A non-negative number defining the general startup order.
- up_
delay int - A non-negative number defining the delay in seconds before the next container is started.
Import
Instances can be imported using the node_name
and the vm_id
, e.g.,
bash
$ pulumi import proxmoxve:CT/container:Container ubuntu_container first-node/1234
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
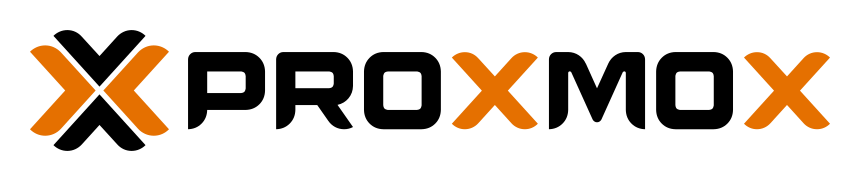