proxmoxve.Network.NetworkBridge
Explore with Pulumi AI
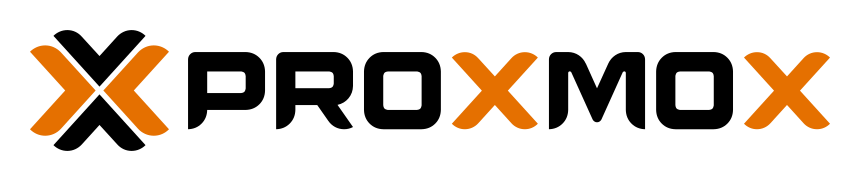
Manages a Linux Bridge network interface in a Proxmox VE node.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const vmbr99 = new proxmoxve.network.NetworkBridge("vmbr99", {
nodeName: "pve",
address: "99.99.99.99/16",
comment: "vmbr99 comment",
ports: ["ens18.99"],
}, {
dependsOn: [proxmox_virtual_environment_network_linux_vlan.vlan99],
});
import pulumi
import pulumi_proxmoxve as proxmoxve
vmbr99 = proxmoxve.network.NetworkBridge("vmbr99",
node_name="pve",
address="99.99.99.99/16",
comment="vmbr99 comment",
ports=["ens18.99"],
opts = pulumi.ResourceOptions(depends_on=[proxmox_virtual_environment_network_linux_vlan["vlan99"]]))
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Network"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := Network.NewNetworkBridge(ctx, "vmbr99", &Network.NetworkBridgeArgs{
NodeName: pulumi.String("pve"),
Address: pulumi.String("99.99.99.99/16"),
Comment: pulumi.String("vmbr99 comment"),
Ports: pulumi.StringArray{
pulumi.String("ens18.99"),
},
}, pulumi.DependsOn([]pulumi.Resource{
proxmox_virtual_environment_network_linux_vlan.Vlan99,
}))
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var vmbr99 = new ProxmoxVE.Network.NetworkBridge("vmbr99", new()
{
NodeName = "pve",
Address = "99.99.99.99/16",
Comment = "vmbr99 comment",
Ports = new[]
{
"ens18.99",
},
}, new CustomResourceOptions
{
DependsOn =
{
proxmox_virtual_environment_network_linux_vlan.Vlan99,
},
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Network.NetworkBridge;
import com.pulumi.proxmoxve.Network.NetworkBridgeArgs;
import com.pulumi.resources.CustomResourceOptions;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var vmbr99 = new NetworkBridge("vmbr99", NetworkBridgeArgs.builder()
.nodeName("pve")
.address("99.99.99.99/16")
.comment("vmbr99 comment")
.ports("ens18.99")
.build(), CustomResourceOptions.builder()
.dependsOn(proxmox_virtual_environment_network_linux_vlan.vlan99())
.build());
}
}
resources:
vmbr99:
type: proxmoxve:Network:NetworkBridge
properties:
nodeName: pve
address: 99.99.99.99/16
comment: vmbr99 comment
ports:
- ens18.99
options:
dependson:
- ${proxmox_virtual_environment_network_linux_vlan.vlan99}
Create NetworkBridge Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new NetworkBridge(name: string, args: NetworkBridgeArgs, opts?: CustomResourceOptions);
@overload
def NetworkBridge(resource_name: str,
args: NetworkBridgeArgs,
opts: Optional[ResourceOptions] = None)
@overload
def NetworkBridge(resource_name: str,
opts: Optional[ResourceOptions] = None,
node_name: Optional[str] = None,
address: Optional[str] = None,
address6: Optional[str] = None,
autostart: Optional[bool] = None,
comment: Optional[str] = None,
gateway: Optional[str] = None,
gateway6: Optional[str] = None,
mtu: Optional[int] = None,
name: Optional[str] = None,
ports: Optional[Sequence[str]] = None,
vlan_aware: Optional[bool] = None)
func NewNetworkBridge(ctx *Context, name string, args NetworkBridgeArgs, opts ...ResourceOption) (*NetworkBridge, error)
public NetworkBridge(string name, NetworkBridgeArgs args, CustomResourceOptions? opts = null)
public NetworkBridge(String name, NetworkBridgeArgs args)
public NetworkBridge(String name, NetworkBridgeArgs args, CustomResourceOptions options)
type: proxmoxve:Network:NetworkBridge
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args NetworkBridgeArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args NetworkBridgeArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args NetworkBridgeArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args NetworkBridgeArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args NetworkBridgeArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var networkBridgeResource = new ProxmoxVE.Network.NetworkBridge("networkBridgeResource", new()
{
NodeName = "string",
Address = "string",
Address6 = "string",
Autostart = false,
Comment = "string",
Gateway = "string",
Gateway6 = "string",
Mtu = 0,
Name = "string",
Ports = new[]
{
"string",
},
VlanAware = false,
});
example, err := Network.NewNetworkBridge(ctx, "networkBridgeResource", &Network.NetworkBridgeArgs{
NodeName: pulumi.String("string"),
Address: pulumi.String("string"),
Address6: pulumi.String("string"),
Autostart: pulumi.Bool(false),
Comment: pulumi.String("string"),
Gateway: pulumi.String("string"),
Gateway6: pulumi.String("string"),
Mtu: pulumi.Int(0),
Name: pulumi.String("string"),
Ports: pulumi.StringArray{
pulumi.String("string"),
},
VlanAware: pulumi.Bool(false),
})
var networkBridgeResource = new NetworkBridge("networkBridgeResource", NetworkBridgeArgs.builder()
.nodeName("string")
.address("string")
.address6("string")
.autostart(false)
.comment("string")
.gateway("string")
.gateway6("string")
.mtu(0)
.name("string")
.ports("string")
.vlanAware(false)
.build());
network_bridge_resource = proxmoxve.network.NetworkBridge("networkBridgeResource",
node_name="string",
address="string",
address6="string",
autostart=False,
comment="string",
gateway="string",
gateway6="string",
mtu=0,
name="string",
ports=["string"],
vlan_aware=False)
const networkBridgeResource = new proxmoxve.network.NetworkBridge("networkBridgeResource", {
nodeName: "string",
address: "string",
address6: "string",
autostart: false,
comment: "string",
gateway: "string",
gateway6: "string",
mtu: 0,
name: "string",
ports: ["string"],
vlanAware: false,
});
type: proxmoxve:Network:NetworkBridge
properties:
address: string
address6: string
autostart: false
comment: string
gateway: string
gateway6: string
mtu: 0
name: string
nodeName: string
ports:
- string
vlanAware: false
NetworkBridge Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The NetworkBridge resource accepts the following input properties:
- Node
Name string - The name of the node.
- Address string
- The interface IPv4/CIDR address.
- Address6 string
- The interface IPv6/CIDR address.
- Autostart bool
- Automatically start interface on boot (defaults to
true
). - Comment string
- Comment for the interface.
- Gateway string
- Default gateway address.
- Gateway6 string
- Default IPv6 gateway address.
- Mtu int
- The interface MTU.
- Name string
- The interface name. Must be
vmbrN
, where N is a number between 0 and 9999. - Ports List<string>
- The interface bridge ports.
- Vlan
Aware bool - Whether the interface bridge is VLAN aware (defaults to
false
).
- Node
Name string - The name of the node.
- Address string
- The interface IPv4/CIDR address.
- Address6 string
- The interface IPv6/CIDR address.
- Autostart bool
- Automatically start interface on boot (defaults to
true
). - Comment string
- Comment for the interface.
- Gateway string
- Default gateway address.
- Gateway6 string
- Default IPv6 gateway address.
- Mtu int
- The interface MTU.
- Name string
- The interface name. Must be
vmbrN
, where N is a number between 0 and 9999. - Ports []string
- The interface bridge ports.
- Vlan
Aware bool - Whether the interface bridge is VLAN aware (defaults to
false
).
- node
Name String - The name of the node.
- address String
- The interface IPv4/CIDR address.
- address6 String
- The interface IPv6/CIDR address.
- autostart Boolean
- Automatically start interface on boot (defaults to
true
). - comment String
- Comment for the interface.
- gateway String
- Default gateway address.
- gateway6 String
- Default IPv6 gateway address.
- mtu Integer
- The interface MTU.
- name String
- The interface name. Must be
vmbrN
, where N is a number between 0 and 9999. - ports List<String>
- The interface bridge ports.
- vlan
Aware Boolean - Whether the interface bridge is VLAN aware (defaults to
false
).
- node
Name string - The name of the node.
- address string
- The interface IPv4/CIDR address.
- address6 string
- The interface IPv6/CIDR address.
- autostart boolean
- Automatically start interface on boot (defaults to
true
). - comment string
- Comment for the interface.
- gateway string
- Default gateway address.
- gateway6 string
- Default IPv6 gateway address.
- mtu number
- The interface MTU.
- name string
- The interface name. Must be
vmbrN
, where N is a number between 0 and 9999. - ports string[]
- The interface bridge ports.
- vlan
Aware boolean - Whether the interface bridge is VLAN aware (defaults to
false
).
- node_
name str - The name of the node.
- address str
- The interface IPv4/CIDR address.
- address6 str
- The interface IPv6/CIDR address.
- autostart bool
- Automatically start interface on boot (defaults to
true
). - comment str
- Comment for the interface.
- gateway str
- Default gateway address.
- gateway6 str
- Default IPv6 gateway address.
- mtu int
- The interface MTU.
- name str
- The interface name. Must be
vmbrN
, where N is a number between 0 and 9999. - ports Sequence[str]
- The interface bridge ports.
- vlan_
aware bool - Whether the interface bridge is VLAN aware (defaults to
false
).
- node
Name String - The name of the node.
- address String
- The interface IPv4/CIDR address.
- address6 String
- The interface IPv6/CIDR address.
- autostart Boolean
- Automatically start interface on boot (defaults to
true
). - comment String
- Comment for the interface.
- gateway String
- Default gateway address.
- gateway6 String
- Default IPv6 gateway address.
- mtu Number
- The interface MTU.
- name String
- The interface name. Must be
vmbrN
, where N is a number between 0 and 9999. - ports List<String>
- The interface bridge ports.
- vlan
Aware Boolean - Whether the interface bridge is VLAN aware (defaults to
false
).
Outputs
All input properties are implicitly available as output properties. Additionally, the NetworkBridge resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing NetworkBridge Resource
Get an existing NetworkBridge resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: NetworkBridgeState, opts?: CustomResourceOptions): NetworkBridge
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
address: Optional[str] = None,
address6: Optional[str] = None,
autostart: Optional[bool] = None,
comment: Optional[str] = None,
gateway: Optional[str] = None,
gateway6: Optional[str] = None,
mtu: Optional[int] = None,
name: Optional[str] = None,
node_name: Optional[str] = None,
ports: Optional[Sequence[str]] = None,
vlan_aware: Optional[bool] = None) -> NetworkBridge
func GetNetworkBridge(ctx *Context, name string, id IDInput, state *NetworkBridgeState, opts ...ResourceOption) (*NetworkBridge, error)
public static NetworkBridge Get(string name, Input<string> id, NetworkBridgeState? state, CustomResourceOptions? opts = null)
public static NetworkBridge get(String name, Output<String> id, NetworkBridgeState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Address string
- The interface IPv4/CIDR address.
- Address6 string
- The interface IPv6/CIDR address.
- Autostart bool
- Automatically start interface on boot (defaults to
true
). - Comment string
- Comment for the interface.
- Gateway string
- Default gateway address.
- Gateway6 string
- Default IPv6 gateway address.
- Mtu int
- The interface MTU.
- Name string
- The interface name. Must be
vmbrN
, where N is a number between 0 and 9999. - Node
Name string - The name of the node.
- Ports List<string>
- The interface bridge ports.
- Vlan
Aware bool - Whether the interface bridge is VLAN aware (defaults to
false
).
- Address string
- The interface IPv4/CIDR address.
- Address6 string
- The interface IPv6/CIDR address.
- Autostart bool
- Automatically start interface on boot (defaults to
true
). - Comment string
- Comment for the interface.
- Gateway string
- Default gateway address.
- Gateway6 string
- Default IPv6 gateway address.
- Mtu int
- The interface MTU.
- Name string
- The interface name. Must be
vmbrN
, where N is a number between 0 and 9999. - Node
Name string - The name of the node.
- Ports []string
- The interface bridge ports.
- Vlan
Aware bool - Whether the interface bridge is VLAN aware (defaults to
false
).
- address String
- The interface IPv4/CIDR address.
- address6 String
- The interface IPv6/CIDR address.
- autostart Boolean
- Automatically start interface on boot (defaults to
true
). - comment String
- Comment for the interface.
- gateway String
- Default gateway address.
- gateway6 String
- Default IPv6 gateway address.
- mtu Integer
- The interface MTU.
- name String
- The interface name. Must be
vmbrN
, where N is a number between 0 and 9999. - node
Name String - The name of the node.
- ports List<String>
- The interface bridge ports.
- vlan
Aware Boolean - Whether the interface bridge is VLAN aware (defaults to
false
).
- address string
- The interface IPv4/CIDR address.
- address6 string
- The interface IPv6/CIDR address.
- autostart boolean
- Automatically start interface on boot (defaults to
true
). - comment string
- Comment for the interface.
- gateway string
- Default gateway address.
- gateway6 string
- Default IPv6 gateway address.
- mtu number
- The interface MTU.
- name string
- The interface name. Must be
vmbrN
, where N is a number between 0 and 9999. - node
Name string - The name of the node.
- ports string[]
- The interface bridge ports.
- vlan
Aware boolean - Whether the interface bridge is VLAN aware (defaults to
false
).
- address str
- The interface IPv4/CIDR address.
- address6 str
- The interface IPv6/CIDR address.
- autostart bool
- Automatically start interface on boot (defaults to
true
). - comment str
- Comment for the interface.
- gateway str
- Default gateway address.
- gateway6 str
- Default IPv6 gateway address.
- mtu int
- The interface MTU.
- name str
- The interface name. Must be
vmbrN
, where N is a number between 0 and 9999. - node_
name str - The name of the node.
- ports Sequence[str]
- The interface bridge ports.
- vlan_
aware bool - Whether the interface bridge is VLAN aware (defaults to
false
).
- address String
- The interface IPv4/CIDR address.
- address6 String
- The interface IPv6/CIDR address.
- autostart Boolean
- Automatically start interface on boot (defaults to
true
). - comment String
- Comment for the interface.
- gateway String
- Default gateway address.
- gateway6 String
- Default IPv6 gateway address.
- mtu Number
- The interface MTU.
- name String
- The interface name. Must be
vmbrN
, where N is a number between 0 and 9999. - node
Name String - The name of the node.
- ports List<String>
- The interface bridge ports.
- vlan
Aware Boolean - Whether the interface bridge is VLAN aware (defaults to
false
).
Import
#!/usr/bin/env sh
#Interfaces can be imported using the node_name:iface
format, e.g.
$ pulumi import proxmoxve:Network/networkBridge:NetworkBridge vmbr99 pve:vmbr99
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
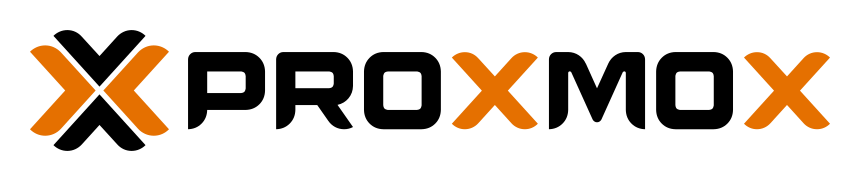