proxmoxve.Storage.File
Explore with Pulumi AI
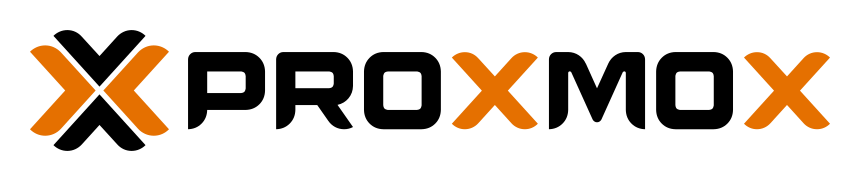
Manages a file.
Example Usage
Backups (dump
)
The resource with this content type uses SSH access to the node. You might need to configure the
ssh
option in theprovider
section.
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const backup = new proxmoxve.storage.File("backup", {
contentType: "dump",
datastoreId: "local",
nodeName: "pve",
sourceFile: {
path: "vzdump-lxc-100-2023_11_08-23_10_05.tar",
},
});
import pulumi
import pulumi_proxmoxve as proxmoxve
backup = proxmoxve.storage.File("backup",
content_type="dump",
datastore_id="local",
node_name="pve",
source_file={
"path": "vzdump-lxc-100-2023_11_08-23_10_05.tar",
})
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Storage"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := Storage.NewFile(ctx, "backup", &Storage.FileArgs{
ContentType: pulumi.String("dump"),
DatastoreId: pulumi.String("local"),
NodeName: pulumi.String("pve"),
SourceFile: &storage.FileSourceFileArgs{
Path: pulumi.String("vzdump-lxc-100-2023_11_08-23_10_05.tar"),
},
})
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var backup = new ProxmoxVE.Storage.File("backup", new()
{
ContentType = "dump",
DatastoreId = "local",
NodeName = "pve",
SourceFile = new ProxmoxVE.Storage.Inputs.FileSourceFileArgs
{
Path = "vzdump-lxc-100-2023_11_08-23_10_05.tar",
},
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Storage.File;
import com.pulumi.proxmoxve.Storage.FileArgs;
import com.pulumi.proxmoxve.Storage.inputs.FileSourceFileArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var backup = new File("backup", FileArgs.builder()
.contentType("dump")
.datastoreId("local")
.nodeName("pve")
.sourceFile(FileSourceFileArgs.builder()
.path("vzdump-lxc-100-2023_11_08-23_10_05.tar")
.build())
.build());
}
}
resources:
backup:
type: proxmoxve:Storage:File
properties:
contentType: dump
datastoreId: local
nodeName: pve
sourceFile:
path: vzdump-lxc-100-2023_11_08-23_10_05.tar
Images
Consider using
proxmoxve.Download.File
resource instead. Using this resource for images is less efficient (requires to transfer uploaded image to node) though still supported.
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const ubuntuContainerTemplate = new proxmoxve.storage.File("ubuntuContainerTemplate", {
contentType: "iso",
datastoreId: "local",
nodeName: "pve",
sourceFile: {
path: "https://cloud-images.ubuntu.com/jammy/20230929/jammy-server-cloudimg-amd64-disk-kvm.img",
},
});
import pulumi
import pulumi_proxmoxve as proxmoxve
ubuntu_container_template = proxmoxve.storage.File("ubuntuContainerTemplate",
content_type="iso",
datastore_id="local",
node_name="pve",
source_file={
"path": "https://cloud-images.ubuntu.com/jammy/20230929/jammy-server-cloudimg-amd64-disk-kvm.img",
})
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Storage"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := Storage.NewFile(ctx, "ubuntuContainerTemplate", &Storage.FileArgs{
ContentType: pulumi.String("iso"),
DatastoreId: pulumi.String("local"),
NodeName: pulumi.String("pve"),
SourceFile: &storage.FileSourceFileArgs{
Path: pulumi.String("https://cloud-images.ubuntu.com/jammy/20230929/jammy-server-cloudimg-amd64-disk-kvm.img"),
},
})
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var ubuntuContainerTemplate = new ProxmoxVE.Storage.File("ubuntuContainerTemplate", new()
{
ContentType = "iso",
DatastoreId = "local",
NodeName = "pve",
SourceFile = new ProxmoxVE.Storage.Inputs.FileSourceFileArgs
{
Path = "https://cloud-images.ubuntu.com/jammy/20230929/jammy-server-cloudimg-amd64-disk-kvm.img",
},
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Storage.File;
import com.pulumi.proxmoxve.Storage.FileArgs;
import com.pulumi.proxmoxve.Storage.inputs.FileSourceFileArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var ubuntuContainerTemplate = new File("ubuntuContainerTemplate", FileArgs.builder()
.contentType("iso")
.datastoreId("local")
.nodeName("pve")
.sourceFile(FileSourceFileArgs.builder()
.path("https://cloud-images.ubuntu.com/jammy/20230929/jammy-server-cloudimg-amd64-disk-kvm.img")
.build())
.build());
}
}
resources:
ubuntuContainerTemplate:
type: proxmoxve:Storage:File
properties:
contentType: iso
datastoreId: local
nodeName: pve
sourceFile:
path: https://cloud-images.ubuntu.com/jammy/20230929/jammy-server-cloudimg-amd64-disk-kvm.img
Container Template (vztmpl
)
Consider using
proxmoxve.Download.File
resource instead. Using this resource for container images is less efficient (requires to transfer uploaded image to node) though still supported.
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@muhlba91/pulumi-proxmoxve";
const ubuntuContainerTemplate = new proxmoxve.storage.File("ubuntuContainerTemplate", {
contentType: "vztmpl",
datastoreId: "local",
nodeName: "first-node",
sourceFile: {
path: "https://download.proxmox.com/images/system/ubuntu-20.04-standard_20.04-1_amd64.tar.gz",
},
});
import pulumi
import pulumi_proxmoxve as proxmoxve
ubuntu_container_template = proxmoxve.storage.File("ubuntuContainerTemplate",
content_type="vztmpl",
datastore_id="local",
node_name="first-node",
source_file={
"path": "https://download.proxmox.com/images/system/ubuntu-20.04-standard_20.04-1_amd64.tar.gz",
})
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Storage"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := Storage.NewFile(ctx, "ubuntuContainerTemplate", &Storage.FileArgs{
ContentType: pulumi.String("vztmpl"),
DatastoreId: pulumi.String("local"),
NodeName: pulumi.String("first-node"),
SourceFile: &storage.FileSourceFileArgs{
Path: pulumi.String("https://download.proxmox.com/images/system/ubuntu-20.04-standard_20.04-1_amd64.tar.gz"),
},
})
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var ubuntuContainerTemplate = new ProxmoxVE.Storage.File("ubuntuContainerTemplate", new()
{
ContentType = "vztmpl",
DatastoreId = "local",
NodeName = "first-node",
SourceFile = new ProxmoxVE.Storage.Inputs.FileSourceFileArgs
{
Path = "https://download.proxmox.com/images/system/ubuntu-20.04-standard_20.04-1_amd64.tar.gz",
},
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Storage.File;
import com.pulumi.proxmoxve.Storage.FileArgs;
import com.pulumi.proxmoxve.Storage.inputs.FileSourceFileArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var ubuntuContainerTemplate = new File("ubuntuContainerTemplate", FileArgs.builder()
.contentType("vztmpl")
.datastoreId("local")
.nodeName("first-node")
.sourceFile(FileSourceFileArgs.builder()
.path("https://download.proxmox.com/images/system/ubuntu-20.04-standard_20.04-1_amd64.tar.gz")
.build())
.build());
}
}
resources:
ubuntuContainerTemplate:
type: proxmoxve:Storage:File
properties:
contentType: vztmpl
datastoreId: local
nodeName: first-node
sourceFile:
path: https://download.proxmox.com/images/system/ubuntu-20.04-standard_20.04-1_amd64.tar.gz
Create File Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new File(name: string, args: FileArgs, opts?: CustomResourceOptions);
@overload
def File(resource_name: str,
args: FileArgs,
opts: Optional[ResourceOptions] = None)
@overload
def File(resource_name: str,
opts: Optional[ResourceOptions] = None,
datastore_id: Optional[str] = None,
node_name: Optional[str] = None,
content_type: Optional[str] = None,
file_mode: Optional[str] = None,
overwrite: Optional[bool] = None,
source_file: Optional[_storage.FileSourceFileArgs] = None,
source_raw: Optional[_storage.FileSourceRawArgs] = None,
timeout_upload: Optional[int] = None)
func NewFile(ctx *Context, name string, args FileArgs, opts ...ResourceOption) (*File, error)
public File(string name, FileArgs args, CustomResourceOptions? opts = null)
type: proxmoxve:Storage:File
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args FileArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args FileArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args FileArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args FileArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args FileArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var proxmoxveFileResource = new ProxmoxVE.Storage.File("proxmoxveFileResource", new()
{
DatastoreId = "string",
NodeName = "string",
ContentType = "string",
FileMode = "string",
Overwrite = false,
SourceFile = new ProxmoxVE.Storage.Inputs.FileSourceFileArgs
{
Path = "string",
Changed = false,
Checksum = "string",
FileName = "string",
Insecure = false,
MinTls = "string",
},
SourceRaw = new ProxmoxVE.Storage.Inputs.FileSourceRawArgs
{
Data = "string",
FileName = "string",
Resize = 0,
},
TimeoutUpload = 0,
});
example, err := Storage.NewFile(ctx, "proxmoxveFileResource", &Storage.FileArgs{
DatastoreId: pulumi.String("string"),
NodeName: pulumi.String("string"),
ContentType: pulumi.String("string"),
FileMode: pulumi.String("string"),
Overwrite: pulumi.Bool(false),
SourceFile: &storage.FileSourceFileArgs{
Path: pulumi.String("string"),
Changed: pulumi.Bool(false),
Checksum: pulumi.String("string"),
FileName: pulumi.String("string"),
Insecure: pulumi.Bool(false),
MinTls: pulumi.String("string"),
},
SourceRaw: &storage.FileSourceRawArgs{
Data: pulumi.String("string"),
FileName: pulumi.String("string"),
Resize: pulumi.Int(0),
},
TimeoutUpload: pulumi.Int(0),
})
var proxmoxveFileResource = new File("proxmoxveFileResource", FileArgs.builder()
.datastoreId("string")
.nodeName("string")
.contentType("string")
.fileMode("string")
.overwrite(false)
.sourceFile(FileSourceFileArgs.builder()
.path("string")
.changed(false)
.checksum("string")
.fileName("string")
.insecure(false)
.minTls("string")
.build())
.sourceRaw(FileSourceRawArgs.builder()
.data("string")
.fileName("string")
.resize(0)
.build())
.timeoutUpload(0)
.build());
proxmoxve_file_resource = proxmoxve.storage.File("proxmoxveFileResource",
datastore_id="string",
node_name="string",
content_type="string",
file_mode="string",
overwrite=False,
source_file=proxmoxve.storage.FileSourceFileArgs(
path="string",
changed=False,
checksum="string",
file_name="string",
insecure=False,
min_tls="string",
),
source_raw=proxmoxve.storage.FileSourceRawArgs(
data="string",
file_name="string",
resize=0,
),
timeout_upload=0)
const proxmoxveFileResource = new proxmoxve.storage.File("proxmoxveFileResource", {
datastoreId: "string",
nodeName: "string",
contentType: "string",
fileMode: "string",
overwrite: false,
sourceFile: {
path: "string",
changed: false,
checksum: "string",
fileName: "string",
insecure: false,
minTls: "string",
},
sourceRaw: {
data: "string",
fileName: "string",
resize: 0,
},
timeoutUpload: 0,
});
type: proxmoxve:Storage:File
properties:
contentType: string
datastoreId: string
fileMode: string
nodeName: string
overwrite: false
sourceFile:
changed: false
checksum: string
fileName: string
insecure: false
minTls: string
path: string
sourceRaw:
data: string
fileName: string
resize: 0
timeoutUpload: 0
File Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The File resource accepts the following input properties:
- Datastore
Id string - The datastore id.
- Node
Name string - The node name.
- Content
Type string - The content type. If not specified, the content type will be inferred from the file extension. Valid values are:
- File
Mode string - The file mode in octal format, e.g.
0700
or600
. Note that the prefixes0o
and0x
is not supported! Setting this attribute is also only allowed forroot@pam
authenticated user. - Overwrite bool
- Whether to overwrite an existing file (defaults to
true
). - Source
File Pulumi.Proxmox VE. Storage. Inputs. File Source File - The source file (conflicts with
source_raw
), could be a local file or a URL. If the source file is a URL, the file will be downloaded and stored locally before uploading it to Proxmox VE. - Source
Raw Pulumi.Proxmox VE. Storage. Inputs. File Source Raw - The raw source (conflicts with
source_file
). - Timeout
Upload int - Timeout for uploading ISO/VSTMPL files in seconds (defaults to 1800).
- Datastore
Id string - The datastore id.
- Node
Name string - The node name.
- Content
Type string - The content type. If not specified, the content type will be inferred from the file extension. Valid values are:
- File
Mode string - The file mode in octal format, e.g.
0700
or600
. Note that the prefixes0o
and0x
is not supported! Setting this attribute is also only allowed forroot@pam
authenticated user. - Overwrite bool
- Whether to overwrite an existing file (defaults to
true
). - Source
File FileSource File Args - The source file (conflicts with
source_raw
), could be a local file or a URL. If the source file is a URL, the file will be downloaded and stored locally before uploading it to Proxmox VE. - Source
Raw FileSource Raw Args - The raw source (conflicts with
source_file
). - Timeout
Upload int - Timeout for uploading ISO/VSTMPL files in seconds (defaults to 1800).
- datastore
Id String - The datastore id.
- node
Name String - The node name.
- content
Type String - The content type. If not specified, the content type will be inferred from the file extension. Valid values are:
- file
Mode String - The file mode in octal format, e.g.
0700
or600
. Note that the prefixes0o
and0x
is not supported! Setting this attribute is also only allowed forroot@pam
authenticated user. - overwrite Boolean
- Whether to overwrite an existing file (defaults to
true
). - source
File FileSource File - The source file (conflicts with
source_raw
), could be a local file or a URL. If the source file is a URL, the file will be downloaded and stored locally before uploading it to Proxmox VE. - source
Raw FileSource Raw - The raw source (conflicts with
source_file
). - timeout
Upload Integer - Timeout for uploading ISO/VSTMPL files in seconds (defaults to 1800).
- datastore
Id string - The datastore id.
- node
Name string - The node name.
- content
Type string - The content type. If not specified, the content type will be inferred from the file extension. Valid values are:
- file
Mode string - The file mode in octal format, e.g.
0700
or600
. Note that the prefixes0o
and0x
is not supported! Setting this attribute is also only allowed forroot@pam
authenticated user. - overwrite boolean
- Whether to overwrite an existing file (defaults to
true
). - source
File FileSource File - The source file (conflicts with
source_raw
), could be a local file or a URL. If the source file is a URL, the file will be downloaded and stored locally before uploading it to Proxmox VE. - source
Raw FileSource Raw - The raw source (conflicts with
source_file
). - timeout
Upload number - Timeout for uploading ISO/VSTMPL files in seconds (defaults to 1800).
- datastore_
id str - The datastore id.
- node_
name str - The node name.
- content_
type str - The content type. If not specified, the content type will be inferred from the file extension. Valid values are:
- file_
mode str - The file mode in octal format, e.g.
0700
or600
. Note that the prefixes0o
and0x
is not supported! Setting this attribute is also only allowed forroot@pam
authenticated user. - overwrite bool
- Whether to overwrite an existing file (defaults to
true
). - source_
file storage.File Source File Args - The source file (conflicts with
source_raw
), could be a local file or a URL. If the source file is a URL, the file will be downloaded and stored locally before uploading it to Proxmox VE. - source_
raw storage.File Source Raw Args - The raw source (conflicts with
source_file
). - timeout_
upload int - Timeout for uploading ISO/VSTMPL files in seconds (defaults to 1800).
- datastore
Id String - The datastore id.
- node
Name String - The node name.
- content
Type String - The content type. If not specified, the content type will be inferred from the file extension. Valid values are:
- file
Mode String - The file mode in octal format, e.g.
0700
or600
. Note that the prefixes0o
and0x
is not supported! Setting this attribute is also only allowed forroot@pam
authenticated user. - overwrite Boolean
- Whether to overwrite an existing file (defaults to
true
). - source
File Property Map - The source file (conflicts with
source_raw
), could be a local file or a URL. If the source file is a URL, the file will be downloaded and stored locally before uploading it to Proxmox VE. - source
Raw Property Map - The raw source (conflicts with
source_file
). - timeout
Upload Number - Timeout for uploading ISO/VSTMPL files in seconds (defaults to 1800).
Outputs
All input properties are implicitly available as output properties. Additionally, the File resource produces the following output properties:
- File
Modification stringDate - The file modification date (RFC 3339).
- File
Name string - The file name.
- File
Size int - The file size in bytes.
- File
Tag string - The file tag.
- Id string
- The provider-assigned unique ID for this managed resource.
- File
Modification stringDate - The file modification date (RFC 3339).
- File
Name string - The file name.
- File
Size int - The file size in bytes.
- File
Tag string - The file tag.
- Id string
- The provider-assigned unique ID for this managed resource.
- file
Modification StringDate - The file modification date (RFC 3339).
- file
Name String - The file name.
- file
Size Integer - The file size in bytes.
- file
Tag String - The file tag.
- id String
- The provider-assigned unique ID for this managed resource.
- file
Modification stringDate - The file modification date (RFC 3339).
- file
Name string - The file name.
- file
Size number - The file size in bytes.
- file
Tag string - The file tag.
- id string
- The provider-assigned unique ID for this managed resource.
- file_
modification_ strdate - The file modification date (RFC 3339).
- file_
name str - The file name.
- file_
size int - The file size in bytes.
- file_
tag str - The file tag.
- id str
- The provider-assigned unique ID for this managed resource.
- file
Modification StringDate - The file modification date (RFC 3339).
- file
Name String - The file name.
- file
Size Number - The file size in bytes.
- file
Tag String - The file tag.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing File Resource
Get an existing File resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: FileState, opts?: CustomResourceOptions): File
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
content_type: Optional[str] = None,
datastore_id: Optional[str] = None,
file_mode: Optional[str] = None,
file_modification_date: Optional[str] = None,
file_name: Optional[str] = None,
file_size: Optional[int] = None,
file_tag: Optional[str] = None,
node_name: Optional[str] = None,
overwrite: Optional[bool] = None,
source_file: Optional[_storage.FileSourceFileArgs] = None,
source_raw: Optional[_storage.FileSourceRawArgs] = None,
timeout_upload: Optional[int] = None) -> File
func GetFile(ctx *Context, name string, id IDInput, state *FileState, opts ...ResourceOption) (*File, error)
public static File Get(string name, Input<string> id, FileState? state, CustomResourceOptions? opts = null)
public static File get(String name, Output<String> id, FileState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Content
Type string - The content type. If not specified, the content type will be inferred from the file extension. Valid values are:
- Datastore
Id string - The datastore id.
- File
Mode string - The file mode in octal format, e.g.
0700
or600
. Note that the prefixes0o
and0x
is not supported! Setting this attribute is also only allowed forroot@pam
authenticated user. - File
Modification stringDate - The file modification date (RFC 3339).
- File
Name string - The file name.
- File
Size int - The file size in bytes.
- File
Tag string - The file tag.
- Node
Name string - The node name.
- Overwrite bool
- Whether to overwrite an existing file (defaults to
true
). - Source
File Pulumi.Proxmox VE. Storage. Inputs. File Source File - The source file (conflicts with
source_raw
), could be a local file or a URL. If the source file is a URL, the file will be downloaded and stored locally before uploading it to Proxmox VE. - Source
Raw Pulumi.Proxmox VE. Storage. Inputs. File Source Raw - The raw source (conflicts with
source_file
). - Timeout
Upload int - Timeout for uploading ISO/VSTMPL files in seconds (defaults to 1800).
- Content
Type string - The content type. If not specified, the content type will be inferred from the file extension. Valid values are:
- Datastore
Id string - The datastore id.
- File
Mode string - The file mode in octal format, e.g.
0700
or600
. Note that the prefixes0o
and0x
is not supported! Setting this attribute is also only allowed forroot@pam
authenticated user. - File
Modification stringDate - The file modification date (RFC 3339).
- File
Name string - The file name.
- File
Size int - The file size in bytes.
- File
Tag string - The file tag.
- Node
Name string - The node name.
- Overwrite bool
- Whether to overwrite an existing file (defaults to
true
). - Source
File FileSource File Args - The source file (conflicts with
source_raw
), could be a local file or a URL. If the source file is a URL, the file will be downloaded and stored locally before uploading it to Proxmox VE. - Source
Raw FileSource Raw Args - The raw source (conflicts with
source_file
). - Timeout
Upload int - Timeout for uploading ISO/VSTMPL files in seconds (defaults to 1800).
- content
Type String - The content type. If not specified, the content type will be inferred from the file extension. Valid values are:
- datastore
Id String - The datastore id.
- file
Mode String - The file mode in octal format, e.g.
0700
or600
. Note that the prefixes0o
and0x
is not supported! Setting this attribute is also only allowed forroot@pam
authenticated user. - file
Modification StringDate - The file modification date (RFC 3339).
- file
Name String - The file name.
- file
Size Integer - The file size in bytes.
- file
Tag String - The file tag.
- node
Name String - The node name.
- overwrite Boolean
- Whether to overwrite an existing file (defaults to
true
). - source
File FileSource File - The source file (conflicts with
source_raw
), could be a local file or a URL. If the source file is a URL, the file will be downloaded and stored locally before uploading it to Proxmox VE. - source
Raw FileSource Raw - The raw source (conflicts with
source_file
). - timeout
Upload Integer - Timeout for uploading ISO/VSTMPL files in seconds (defaults to 1800).
- content
Type string - The content type. If not specified, the content type will be inferred from the file extension. Valid values are:
- datastore
Id string - The datastore id.
- file
Mode string - The file mode in octal format, e.g.
0700
or600
. Note that the prefixes0o
and0x
is not supported! Setting this attribute is also only allowed forroot@pam
authenticated user. - file
Modification stringDate - The file modification date (RFC 3339).
- file
Name string - The file name.
- file
Size number - The file size in bytes.
- file
Tag string - The file tag.
- node
Name string - The node name.
- overwrite boolean
- Whether to overwrite an existing file (defaults to
true
). - source
File FileSource File - The source file (conflicts with
source_raw
), could be a local file or a URL. If the source file is a URL, the file will be downloaded and stored locally before uploading it to Proxmox VE. - source
Raw FileSource Raw - The raw source (conflicts with
source_file
). - timeout
Upload number - Timeout for uploading ISO/VSTMPL files in seconds (defaults to 1800).
- content_
type str - The content type. If not specified, the content type will be inferred from the file extension. Valid values are:
- datastore_
id str - The datastore id.
- file_
mode str - The file mode in octal format, e.g.
0700
or600
. Note that the prefixes0o
and0x
is not supported! Setting this attribute is also only allowed forroot@pam
authenticated user. - file_
modification_ strdate - The file modification date (RFC 3339).
- file_
name str - The file name.
- file_
size int - The file size in bytes.
- file_
tag str - The file tag.
- node_
name str - The node name.
- overwrite bool
- Whether to overwrite an existing file (defaults to
true
). - source_
file storage.File Source File Args - The source file (conflicts with
source_raw
), could be a local file or a URL. If the source file is a URL, the file will be downloaded and stored locally before uploading it to Proxmox VE. - source_
raw storage.File Source Raw Args - The raw source (conflicts with
source_file
). - timeout_
upload int - Timeout for uploading ISO/VSTMPL files in seconds (defaults to 1800).
- content
Type String - The content type. If not specified, the content type will be inferred from the file extension. Valid values are:
- datastore
Id String - The datastore id.
- file
Mode String - The file mode in octal format, e.g.
0700
or600
. Note that the prefixes0o
and0x
is not supported! Setting this attribute is also only allowed forroot@pam
authenticated user. - file
Modification StringDate - The file modification date (RFC 3339).
- file
Name String - The file name.
- file
Size Number - The file size in bytes.
- file
Tag String - The file tag.
- node
Name String - The node name.
- overwrite Boolean
- Whether to overwrite an existing file (defaults to
true
). - source
File Property Map - The source file (conflicts with
source_raw
), could be a local file or a URL. If the source file is a URL, the file will be downloaded and stored locally before uploading it to Proxmox VE. - source
Raw Property Map - The raw source (conflicts with
source_file
). - timeout
Upload Number - Timeout for uploading ISO/VSTMPL files in seconds (defaults to 1800).
Supporting Types
FileSourceFile, FileSourceFileArgs
- Path string
- A path to a local file or a URL.
- Changed bool
- Whether the source file has changed since the last run
- Checksum string
- The SHA256 checksum of the source file.
- File
Name string - The file name to use instead of the source file
name. Useful when the source file does not have a valid file extension,
for example when the source file is a URL referencing a
.qcow2
image. - Insecure bool
- Whether to skip the TLS verification step for
HTTPS sources (defaults to
false
). - Min
Tls string - The minimum required TLS version for HTTPS
sources. "Supported values:
1.0|1.1|1.2|1.3
(defaults to1.3
).
- Path string
- A path to a local file or a URL.
- Changed bool
- Whether the source file has changed since the last run
- Checksum string
- The SHA256 checksum of the source file.
- File
Name string - The file name to use instead of the source file
name. Useful when the source file does not have a valid file extension,
for example when the source file is a URL referencing a
.qcow2
image. - Insecure bool
- Whether to skip the TLS verification step for
HTTPS sources (defaults to
false
). - Min
Tls string - The minimum required TLS version for HTTPS
sources. "Supported values:
1.0|1.1|1.2|1.3
(defaults to1.3
).
- path String
- A path to a local file or a URL.
- changed Boolean
- Whether the source file has changed since the last run
- checksum String
- The SHA256 checksum of the source file.
- file
Name String - The file name to use instead of the source file
name. Useful when the source file does not have a valid file extension,
for example when the source file is a URL referencing a
.qcow2
image. - insecure Boolean
- Whether to skip the TLS verification step for
HTTPS sources (defaults to
false
). - min
Tls String - The minimum required TLS version for HTTPS
sources. "Supported values:
1.0|1.1|1.2|1.3
(defaults to1.3
).
- path string
- A path to a local file or a URL.
- changed boolean
- Whether the source file has changed since the last run
- checksum string
- The SHA256 checksum of the source file.
- file
Name string - The file name to use instead of the source file
name. Useful when the source file does not have a valid file extension,
for example when the source file is a URL referencing a
.qcow2
image. - insecure boolean
- Whether to skip the TLS verification step for
HTTPS sources (defaults to
false
). - min
Tls string - The minimum required TLS version for HTTPS
sources. "Supported values:
1.0|1.1|1.2|1.3
(defaults to1.3
).
- path str
- A path to a local file or a URL.
- changed bool
- Whether the source file has changed since the last run
- checksum str
- The SHA256 checksum of the source file.
- file_
name str - The file name to use instead of the source file
name. Useful when the source file does not have a valid file extension,
for example when the source file is a URL referencing a
.qcow2
image. - insecure bool
- Whether to skip the TLS verification step for
HTTPS sources (defaults to
false
). - min_
tls str - The minimum required TLS version for HTTPS
sources. "Supported values:
1.0|1.1|1.2|1.3
(defaults to1.3
).
- path String
- A path to a local file or a URL.
- changed Boolean
- Whether the source file has changed since the last run
- checksum String
- The SHA256 checksum of the source file.
- file
Name String - The file name to use instead of the source file
name. Useful when the source file does not have a valid file extension,
for example when the source file is a URL referencing a
.qcow2
image. - insecure Boolean
- Whether to skip the TLS verification step for
HTTPS sources (defaults to
false
). - min
Tls String - The minimum required TLS version for HTTPS
sources. "Supported values:
1.0|1.1|1.2|1.3
(defaults to1.3
).
FileSourceRaw, FileSourceRawArgs
Import
ant Notes
The Proxmox VE API endpoint for file uploads does not support chunked transfer encoding, which means that we must first store the source file as a temporary file locally before uploading it.
You must ensure that you have at least Size-in-MB * 2 + 1
MB of storage space
available (twice the size plus overhead because a multipart payload needs to be
created as another temporary file).
By default, if the specified file already exists, the resource will
unconditionally replace it and take ownership of the resource. On destruction,
the file will be deleted as if it did not exist before. If you want to prevent
the resource from replacing the file, set overwrite
to false
.
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
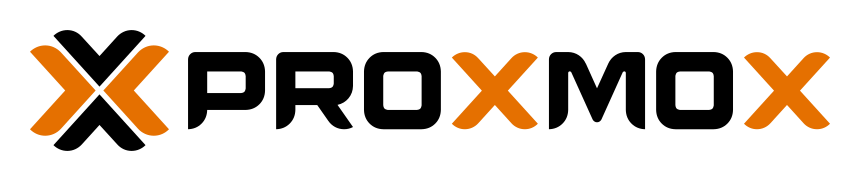