Proxmox Virtual Environment (Proxmox VE) v6.14.0 published on Monday, Sep 9, 2024 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.Storage.getDatastores
Explore with Pulumi AI
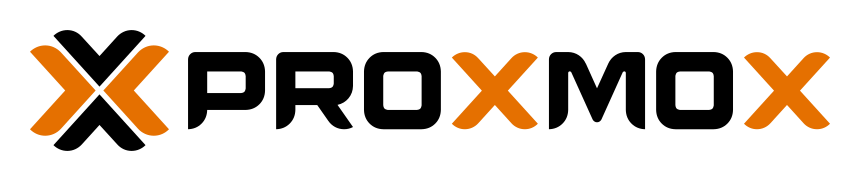
Proxmox Virtual Environment (Proxmox VE) v6.14.0 published on Monday, Sep 9, 2024 by Daniel Muehlbachler-Pietrzykowski
Retrieves information about all the datastores available to a specific node.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@pulumi/proxmoxve";
const firstNode = proxmoxve.Storage.getDatastores({
nodeName: "first-node",
});
import pulumi
import pulumi_proxmoxve as proxmoxve
first_node = proxmoxve.Storage.get_datastores(node_name="first-node")
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/Storage"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := Storage.GetDatastores(ctx, &storage.GetDatastoresArgs{
NodeName: "first-node",
}, nil)
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var firstNode = ProxmoxVE.Storage.GetDatastores.Invoke(new()
{
NodeName = "first-node",
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.Storage.StorageFunctions;
import com.pulumi.proxmoxve.Storage.inputs.GetDatastoresArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var firstNode = StorageFunctions.getDatastores(GetDatastoresArgs.builder()
.nodeName("first-node")
.build());
}
}
variables:
firstNode:
fn::invoke:
Function: proxmoxve:Storage:getDatastores
Arguments:
nodeName: first-node
Using getDatastores
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getDatastores(args: GetDatastoresArgs, opts?: InvokeOptions): Promise<GetDatastoresResult>
function getDatastoresOutput(args: GetDatastoresOutputArgs, opts?: InvokeOptions): Output<GetDatastoresResult>
def get_datastores(node_name: Optional[str] = None,
opts: Optional[InvokeOptions] = None) -> GetDatastoresResult
def get_datastores_output(node_name: Optional[pulumi.Input[str]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetDatastoresResult]
func GetDatastores(ctx *Context, args *GetDatastoresArgs, opts ...InvokeOption) (*GetDatastoresResult, error)
func GetDatastoresOutput(ctx *Context, args *GetDatastoresOutputArgs, opts ...InvokeOption) GetDatastoresResultOutput
> Note: This function is named GetDatastores
in the Go SDK.
public static class GetDatastores
{
public static Task<GetDatastoresResult> InvokeAsync(GetDatastoresArgs args, InvokeOptions? opts = null)
public static Output<GetDatastoresResult> Invoke(GetDatastoresInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetDatastoresResult> getDatastores(GetDatastoresArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: proxmoxve:Storage/getDatastores:getDatastores
arguments:
# arguments dictionary
The following arguments are supported:
- Node
Name string - A node name.
- Node
Name string - A node name.
- node
Name String - A node name.
- node
Name string - A node name.
- node_
name str - A node name.
- node
Name String - A node name.
getDatastores Result
The following output properties are available:
- Actives List<bool>
- Whether the datastore is active.
- Content
Types List<ImmutableArray<string>> - The allowed content types.
- Datastore
Ids List<string> - The datastore identifiers.
- Enableds List<bool>
- Whether the datastore is enabled.
- Id string
- The provider-assigned unique ID for this managed resource.
- Node
Name string - List<bool>
- Whether the datastore is shared.
- Space
Availables List<int> - The available space in bytes.
- Space
Totals List<int> - The total space in bytes.
- Space
Useds List<int> - The used space in bytes.
- Types List<string>
- The storage types.
- Actives []bool
- Whether the datastore is active.
- Content
Types [][]string - The allowed content types.
- Datastore
Ids []string - The datastore identifiers.
- Enableds []bool
- Whether the datastore is enabled.
- Id string
- The provider-assigned unique ID for this managed resource.
- Node
Name string - []bool
- Whether the datastore is shared.
- Space
Availables []int - The available space in bytes.
- Space
Totals []int - The total space in bytes.
- Space
Useds []int - The used space in bytes.
- Types []string
- The storage types.
- actives List<Boolean>
- Whether the datastore is active.
- content
Types List<List<String>> - The allowed content types.
- datastore
Ids List<String> - The datastore identifiers.
- enableds List<Boolean>
- Whether the datastore is enabled.
- id String
- The provider-assigned unique ID for this managed resource.
- node
Name String - List<Boolean>
- Whether the datastore is shared.
- space
Availables List<Integer> - The available space in bytes.
- space
Totals List<Integer> - The total space in bytes.
- space
Useds List<Integer> - The used space in bytes.
- types List<String>
- The storage types.
- actives boolean[]
- Whether the datastore is active.
- content
Types string[][] - The allowed content types.
- datastore
Ids string[] - The datastore identifiers.
- enableds boolean[]
- Whether the datastore is enabled.
- id string
- The provider-assigned unique ID for this managed resource.
- node
Name string - boolean[]
- Whether the datastore is shared.
- space
Availables number[] - The available space in bytes.
- space
Totals number[] - The total space in bytes.
- space
Useds number[] - The used space in bytes.
- types string[]
- The storage types.
- actives Sequence[bool]
- Whether the datastore is active.
- content_
types Sequence[Sequence[str]] - The allowed content types.
- datastore_
ids Sequence[str] - The datastore identifiers.
- enableds Sequence[bool]
- Whether the datastore is enabled.
- id str
- The provider-assigned unique ID for this managed resource.
- node_
name str - Sequence[bool]
- Whether the datastore is shared.
- space_
availables Sequence[int] - The available space in bytes.
- space_
totals Sequence[int] - The total space in bytes.
- space_
useds Sequence[int] - The used space in bytes.
- types Sequence[str]
- The storage types.
- actives List<Boolean>
- Whether the datastore is active.
- content
Types List<List<String>> - The allowed content types.
- datastore
Ids List<String> - The datastore identifiers.
- enableds List<Boolean>
- Whether the datastore is enabled.
- id String
- The provider-assigned unique ID for this managed resource.
- node
Name String - List<Boolean>
- Whether the datastore is shared.
- space
Availables List<Number> - The available space in bytes.
- space
Totals List<Number> - The total space in bytes.
- space
Useds List<Number> - The used space in bytes.
- types List<String>
- The storage types.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
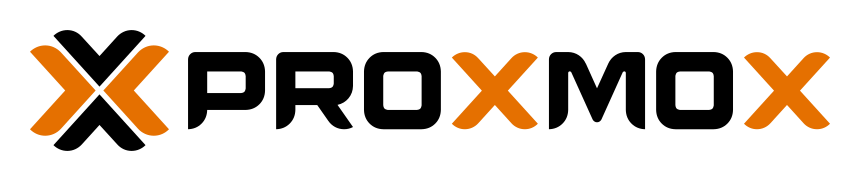
Proxmox Virtual Environment (Proxmox VE) v6.14.0 published on Monday, Sep 9, 2024 by Daniel Muehlbachler-Pietrzykowski