Proxmox Virtual Environment (Proxmox VE) v6.14.0 published on Monday, Sep 9, 2024 by Daniel Muehlbachler-Pietrzykowski
proxmoxve.VM.getVirtualMachines
Explore with Pulumi AI
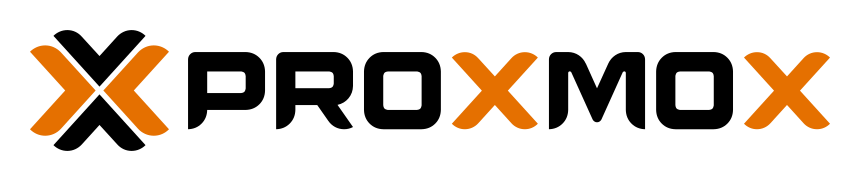
Proxmox Virtual Environment (Proxmox VE) v6.14.0 published on Monday, Sep 9, 2024 by Daniel Muehlbachler-Pietrzykowski
Retrieves information about all VMs in the Proxmox cluster.
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as proxmoxve from "@pulumi/proxmoxve";
const ubuntuVms = proxmoxve.VM.getVirtualMachines({
tags: ["ubuntu"],
});
const ubuntuTemplates = proxmoxve.VM.getVirtualMachines({
filters: [
{
name: "template",
values: ["true"],
},
{
name: "status",
values: ["stopped"],
},
{
name: "name",
regex: true,
values: ["^ubuntu-20.*$"],
},
{
name: "node_name",
regex: true,
values: [
"node_us_[1-3]",
"node_eu_[1-3]",
],
},
],
tags: [
"template",
"latest",
],
});
import pulumi
import pulumi_proxmoxve as proxmoxve
ubuntu_vms = proxmoxve.VM.get_virtual_machines(tags=["ubuntu"])
ubuntu_templates = proxmoxve.VM.get_virtual_machines(filters=[
{
"name": "template",
"values": ["true"],
},
{
"name": "status",
"values": ["stopped"],
},
{
"name": "name",
"regex": True,
"values": ["^ubuntu-20.*$"],
},
{
"name": "node_name",
"regex": True,
"values": [
"node_us_[1-3]",
"node_eu_[1-3]",
],
},
],
tags=[
"template",
"latest",
])
package main
import (
"github.com/muhlba91/pulumi-proxmoxve/sdk/v6/go/proxmoxve/VM"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := VM.GetVirtualMachines(ctx, &vm.GetVirtualMachinesArgs{
Tags: []string{
"ubuntu",
},
}, nil)
if err != nil {
return err
}
_, err = VM.GetVirtualMachines(ctx, &vm.GetVirtualMachinesArgs{
Filters: []vm.GetVirtualMachinesFilter{
{
Name: "template",
Values: []string{
"true",
},
},
{
Name: "status",
Values: []string{
"stopped",
},
},
{
Name: "name",
Regex: pulumi.BoolRef(true),
Values: []string{
"^ubuntu-20.*$",
},
},
{
Name: "node_name",
Regex: pulumi.BoolRef(true),
Values: []string{
"node_us_[1-3]",
"node_eu_[1-3]",
},
},
},
Tags: []string{
"template",
"latest",
},
}, nil)
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using ProxmoxVE = Pulumi.ProxmoxVE;
return await Deployment.RunAsync(() =>
{
var ubuntuVms = ProxmoxVE.VM.GetVirtualMachines.Invoke(new()
{
Tags = new[]
{
"ubuntu",
},
});
var ubuntuTemplates = ProxmoxVE.VM.GetVirtualMachines.Invoke(new()
{
Filters = new[]
{
new ProxmoxVE.VM.Inputs.GetVirtualMachinesFilterInputArgs
{
Name = "template",
Values = new[]
{
"true",
},
},
new ProxmoxVE.VM.Inputs.GetVirtualMachinesFilterInputArgs
{
Name = "status",
Values = new[]
{
"stopped",
},
},
new ProxmoxVE.VM.Inputs.GetVirtualMachinesFilterInputArgs
{
Name = "name",
Regex = true,
Values = new[]
{
"^ubuntu-20.*$",
},
},
new ProxmoxVE.VM.Inputs.GetVirtualMachinesFilterInputArgs
{
Name = "node_name",
Regex = true,
Values = new[]
{
"node_us_[1-3]",
"node_eu_[1-3]",
},
},
},
Tags = new[]
{
"template",
"latest",
},
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.proxmoxve.VM.VMFunctions;
import com.pulumi.proxmoxve.VM.inputs.GetVirtualMachinesArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
final var ubuntuVms = VMFunctions.getVirtualMachines(GetVirtualMachinesArgs.builder()
.tags("ubuntu")
.build());
final var ubuntuTemplates = VMFunctions.getVirtualMachines(GetVirtualMachinesArgs.builder()
.filters(
GetVirtualMachinesFilterArgs.builder()
.name("template")
.values(true)
.build(),
GetVirtualMachinesFilterArgs.builder()
.name("status")
.values("stopped")
.build(),
GetVirtualMachinesFilterArgs.builder()
.name("name")
.regex(true)
.values("^ubuntu-20.*$")
.build(),
GetVirtualMachinesFilterArgs.builder()
.name("node_name")
.regex(true)
.values(
"node_us_[1-3]",
"node_eu_[1-3]")
.build())
.tags(
"template",
"latest")
.build());
}
}
variables:
ubuntuVms:
fn::invoke:
Function: proxmoxve:VM:getVirtualMachines
Arguments:
tags:
- ubuntu
ubuntuTemplates:
fn::invoke:
Function: proxmoxve:VM:getVirtualMachines
Arguments:
filters:
- name: template
values:
- true
- name: status
values:
- stopped
- name: name
regex: true
values:
- ^ubuntu-20.*$
- name: node_name
regex: true
values:
- node_us_[1-3]
- node_eu_[1-3]
tags:
- template
- latest
Using getVirtualMachines
Two invocation forms are available. The direct form accepts plain arguments and either blocks until the result value is available, or returns a Promise-wrapped result. The output form accepts Input-wrapped arguments and returns an Output-wrapped result.
function getVirtualMachines(args: GetVirtualMachinesArgs, opts?: InvokeOptions): Promise<GetVirtualMachinesResult>
function getVirtualMachinesOutput(args: GetVirtualMachinesOutputArgs, opts?: InvokeOptions): Output<GetVirtualMachinesResult>
def get_virtual_machines(filters: Optional[Sequence[_vm.GetVirtualMachinesFilter]] = None,
node_name: Optional[str] = None,
tags: Optional[Sequence[str]] = None,
opts: Optional[InvokeOptions] = None) -> GetVirtualMachinesResult
def get_virtual_machines_output(filters: Optional[pulumi.Input[Sequence[pulumi.Input[_vm.GetVirtualMachinesFilterArgs]]]] = None,
node_name: Optional[pulumi.Input[str]] = None,
tags: Optional[pulumi.Input[Sequence[pulumi.Input[str]]]] = None,
opts: Optional[InvokeOptions] = None) -> Output[GetVirtualMachinesResult]
func GetVirtualMachines(ctx *Context, args *GetVirtualMachinesArgs, opts ...InvokeOption) (*GetVirtualMachinesResult, error)
func GetVirtualMachinesOutput(ctx *Context, args *GetVirtualMachinesOutputArgs, opts ...InvokeOption) GetVirtualMachinesResultOutput
> Note: This function is named GetVirtualMachines
in the Go SDK.
public static class GetVirtualMachines
{
public static Task<GetVirtualMachinesResult> InvokeAsync(GetVirtualMachinesArgs args, InvokeOptions? opts = null)
public static Output<GetVirtualMachinesResult> Invoke(GetVirtualMachinesInvokeArgs args, InvokeOptions? opts = null)
}
public static CompletableFuture<GetVirtualMachinesResult> getVirtualMachines(GetVirtualMachinesArgs args, InvokeOptions options)
// Output-based functions aren't available in Java yet
fn::invoke:
function: proxmoxve:VM/getVirtualMachines:getVirtualMachines
arguments:
# arguments dictionary
The following arguments are supported:
- Filters
List<Pulumi.
Proxmox VE. VM. Inputs. Get Virtual Machines Filter> - Filter blocks. The VM must satisfy all filter blocks to be included in the result.
- Node
Name string - The node name. All cluster nodes will be queried in case this is omitted
- List<string>
- A list of tags to filter the VMs. The VM must have all the tags to be included in the result.
- Filters
[]Get
Virtual Machines Filter - Filter blocks. The VM must satisfy all filter blocks to be included in the result.
- Node
Name string - The node name. All cluster nodes will be queried in case this is omitted
- []string
- A list of tags to filter the VMs. The VM must have all the tags to be included in the result.
- filters
List<Get
Virtual Machines Filter> - Filter blocks. The VM must satisfy all filter blocks to be included in the result.
- node
Name String - The node name. All cluster nodes will be queried in case this is omitted
- List<String>
- A list of tags to filter the VMs. The VM must have all the tags to be included in the result.
- filters
Get
Virtual Machines Filter[] - Filter blocks. The VM must satisfy all filter blocks to be included in the result.
- node
Name string - The node name. All cluster nodes will be queried in case this is omitted
- string[]
- A list of tags to filter the VMs. The VM must have all the tags to be included in the result.
- filters
Sequence[vm.
Get Virtual Machines Filter] - Filter blocks. The VM must satisfy all filter blocks to be included in the result.
- node_
name str - The node name. All cluster nodes will be queried in case this is omitted
- Sequence[str]
- A list of tags to filter the VMs. The VM must have all the tags to be included in the result.
- filters List<Property Map>
- Filter blocks. The VM must satisfy all filter blocks to be included in the result.
- node
Name String - The node name. All cluster nodes will be queried in case this is omitted
- List<String>
- A list of tags to filter the VMs. The VM must have all the tags to be included in the result.
getVirtualMachines Result
The following output properties are available:
- Id string
- The provider-assigned unique ID for this managed resource.
- Vms
List<Pulumi.
Proxmox VE. VM. Outputs. Get Virtual Machines Vm> - The VMs list.
- Filters
List<Pulumi.
Proxmox VE. VM. Outputs. Get Virtual Machines Filter> - Node
Name string - The node name.
- List<string>
- A list of tags of the VM.
- Id string
- The provider-assigned unique ID for this managed resource.
- Vms
[]Get
Virtual Machines Vm - The VMs list.
- Filters
[]Get
Virtual Machines Filter - Node
Name string - The node name.
- []string
- A list of tags of the VM.
- id String
- The provider-assigned unique ID for this managed resource.
- vms
List<Get
Virtual Machines Vm> - The VMs list.
- filters
List<Get
Virtual Machines Filter> - node
Name String - The node name.
- List<String>
- A list of tags of the VM.
- id string
- The provider-assigned unique ID for this managed resource.
- vms
Get
Virtual Machines Vm[] - The VMs list.
- filters
Get
Virtual Machines Filter[] - node
Name string - The node name.
- string[]
- A list of tags of the VM.
- id str
- The provider-assigned unique ID for this managed resource.
- vms
Sequence[vm.
Get Virtual Machines Vm] - The VMs list.
- filters
Sequence[vm.
Get Virtual Machines Filter] - node_
name str - The node name.
- Sequence[str]
- A list of tags of the VM.
- id String
- The provider-assigned unique ID for this managed resource.
- vms List<Property Map>
- The VMs list.
- filters List<Property Map>
- node
Name String - The node name.
- List<String>
- A list of tags of the VM.
Supporting Types
GetVirtualMachinesFilter
GetVirtualMachinesVm
- Name string
- The virtual machine name.
- Node
Name string - The node name. All cluster nodes will be queried in case this is omitted
- List<string>
- A list of tags to filter the VMs. The VM must have all the tags to be included in the result.
- Vm
Id int - The VM identifier.
- Status string
- Status of the VM
- Template bool
- Is VM a template (true) or a regular VM (false)
- Name string
- The virtual machine name.
- Node
Name string - The node name. All cluster nodes will be queried in case this is omitted
- []string
- A list of tags to filter the VMs. The VM must have all the tags to be included in the result.
- Vm
Id int - The VM identifier.
- Status string
- Status of the VM
- Template bool
- Is VM a template (true) or a regular VM (false)
- name String
- The virtual machine name.
- node
Name String - The node name. All cluster nodes will be queried in case this is omitted
- List<String>
- A list of tags to filter the VMs. The VM must have all the tags to be included in the result.
- vm
Id Integer - The VM identifier.
- status String
- Status of the VM
- template Boolean
- Is VM a template (true) or a regular VM (false)
- name string
- The virtual machine name.
- node
Name string - The node name. All cluster nodes will be queried in case this is omitted
- string[]
- A list of tags to filter the VMs. The VM must have all the tags to be included in the result.
- vm
Id number - The VM identifier.
- status string
- Status of the VM
- template boolean
- Is VM a template (true) or a regular VM (false)
- name str
- The virtual machine name.
- node_
name str - The node name. All cluster nodes will be queried in case this is omitted
- Sequence[str]
- A list of tags to filter the VMs. The VM must have all the tags to be included in the result.
- vm_
id int - The VM identifier.
- status str
- Status of the VM
- template bool
- Is VM a template (true) or a regular VM (false)
- name String
- The virtual machine name.
- node
Name String - The node name. All cluster nodes will be queried in case this is omitted
- List<String>
- A list of tags to filter the VMs. The VM must have all the tags to be included in the result.
- vm
Id Number - The VM identifier.
- status String
- Status of the VM
- template Boolean
- Is VM a template (true) or a regular VM (false)
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
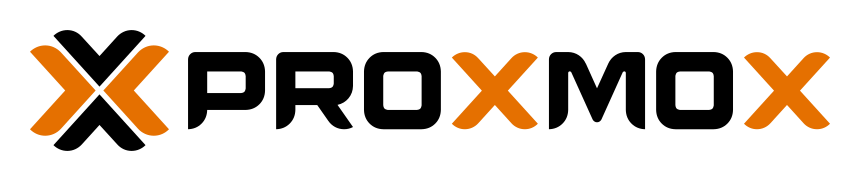
Proxmox Virtual Environment (Proxmox VE) v6.14.0 published on Monday, Sep 9, 2024 by Daniel Muehlbachler-Pietrzykowski