proxmoxve.VM.VirtualMachine
Explore with Pulumi AI
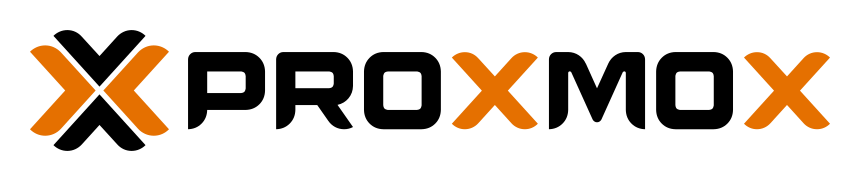
Manages a virtual machine.
This resource uses SSH access to the node. You might need to configure the
ssh
option in theprovider
section.
Create VirtualMachine Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new VirtualMachine(name: string, args: VirtualMachineArgs, opts?: CustomResourceOptions);
@overload
def VirtualMachine(resource_name: str,
args: VirtualMachineArgs,
opts: Optional[ResourceOptions] = None)
@overload
def VirtualMachine(resource_name: str,
opts: Optional[ResourceOptions] = None,
node_name: Optional[str] = None,
acpi: Optional[bool] = None,
agent: Optional[_vm.VirtualMachineAgentArgs] = None,
audio_device: Optional[_vm.VirtualMachineAudioDeviceArgs] = None,
bios: Optional[str] = None,
boot_orders: Optional[Sequence[str]] = None,
cdrom: Optional[_vm.VirtualMachineCdromArgs] = None,
clone: Optional[_vm.VirtualMachineCloneArgs] = None,
cpu: Optional[_vm.VirtualMachineCpuArgs] = None,
description: Optional[str] = None,
disks: Optional[Sequence[_vm.VirtualMachineDiskArgs]] = None,
efi_disk: Optional[_vm.VirtualMachineEfiDiskArgs] = None,
hook_script_file_id: Optional[str] = None,
hostpcis: Optional[Sequence[_vm.VirtualMachineHostpciArgs]] = None,
initialization: Optional[_vm.VirtualMachineInitializationArgs] = None,
keyboard_layout: Optional[str] = None,
kvm_arguments: Optional[str] = None,
mac_addresses: Optional[Sequence[str]] = None,
machine: Optional[str] = None,
memory: Optional[_vm.VirtualMachineMemoryArgs] = None,
migrate: Optional[bool] = None,
name: Optional[str] = None,
network_devices: Optional[Sequence[_vm.VirtualMachineNetworkDeviceArgs]] = None,
numas: Optional[Sequence[_vm.VirtualMachineNumaArgs]] = None,
on_boot: Optional[bool] = None,
operating_system: Optional[_vm.VirtualMachineOperatingSystemArgs] = None,
pool_id: Optional[str] = None,
protection: Optional[bool] = None,
reboot: Optional[bool] = None,
scsi_hardware: Optional[str] = None,
serial_devices: Optional[Sequence[_vm.VirtualMachineSerialDeviceArgs]] = None,
smbios: Optional[_vm.VirtualMachineSmbiosArgs] = None,
started: Optional[bool] = None,
startup: Optional[_vm.VirtualMachineStartupArgs] = None,
stop_on_destroy: Optional[bool] = None,
tablet_device: Optional[bool] = None,
tags: Optional[Sequence[str]] = None,
template: Optional[bool] = None,
timeout_clone: Optional[int] = None,
timeout_create: Optional[int] = None,
timeout_migrate: Optional[int] = None,
timeout_move_disk: Optional[int] = None,
timeout_reboot: Optional[int] = None,
timeout_shutdown_vm: Optional[int] = None,
timeout_start_vm: Optional[int] = None,
timeout_stop_vm: Optional[int] = None,
tpm_state: Optional[_vm.VirtualMachineTpmStateArgs] = None,
usbs: Optional[Sequence[_vm.VirtualMachineUsbArgs]] = None,
vga: Optional[_vm.VirtualMachineVgaArgs] = None,
vm_id: Optional[int] = None)
func NewVirtualMachine(ctx *Context, name string, args VirtualMachineArgs, opts ...ResourceOption) (*VirtualMachine, error)
public VirtualMachine(string name, VirtualMachineArgs args, CustomResourceOptions? opts = null)
public VirtualMachine(String name, VirtualMachineArgs args)
public VirtualMachine(String name, VirtualMachineArgs args, CustomResourceOptions options)
type: proxmoxve:VM:VirtualMachine
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args VirtualMachineArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args VirtualMachineArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args VirtualMachineArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args VirtualMachineArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args VirtualMachineArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var virtualMachineResource = new ProxmoxVE.VM.VirtualMachine("virtualMachineResource", new()
{
NodeName = "string",
Acpi = false,
Agent = new ProxmoxVE.VM.Inputs.VirtualMachineAgentArgs
{
Enabled = false,
Timeout = "string",
Trim = false,
Type = "string",
},
AudioDevice = new ProxmoxVE.VM.Inputs.VirtualMachineAudioDeviceArgs
{
Device = "string",
Driver = "string",
Enabled = false,
},
Bios = "string",
BootOrders = new[]
{
"string",
},
Cdrom = new ProxmoxVE.VM.Inputs.VirtualMachineCdromArgs
{
Enabled = false,
FileId = "string",
Interface = "string",
},
Clone = new ProxmoxVE.VM.Inputs.VirtualMachineCloneArgs
{
VmId = 0,
DatastoreId = "string",
Full = false,
NodeName = "string",
Retries = 0,
},
Cpu = new ProxmoxVE.VM.Inputs.VirtualMachineCpuArgs
{
Affinity = "string",
Architecture = "string",
Cores = 0,
Flags = new[]
{
"string",
},
Hotplugged = 0,
Limit = 0,
Numa = false,
Sockets = 0,
Type = "string",
Units = 0,
},
Description = "string",
Disks = new[]
{
new ProxmoxVE.VM.Inputs.VirtualMachineDiskArgs
{
Interface = "string",
FileId = "string",
Iothread = false,
DatastoreId = "string",
Discard = "string",
FileFormat = "string",
Aio = "string",
Backup = false,
Cache = "string",
PathInDatastore = "string",
Replicate = false,
Serial = "string",
Size = 0,
Speed = new ProxmoxVE.VM.Inputs.VirtualMachineDiskSpeedArgs
{
IopsRead = 0,
IopsReadBurstable = 0,
IopsWrite = 0,
IopsWriteBurstable = 0,
Read = 0,
ReadBurstable = 0,
Write = 0,
WriteBurstable = 0,
},
Ssd = false,
},
},
EfiDisk = new ProxmoxVE.VM.Inputs.VirtualMachineEfiDiskArgs
{
DatastoreId = "string",
FileFormat = "string",
PreEnrolledKeys = false,
Type = "string",
},
HookScriptFileId = "string",
Hostpcis = new[]
{
new ProxmoxVE.VM.Inputs.VirtualMachineHostpciArgs
{
Device = "string",
Id = "string",
Mapping = "string",
Mdev = "string",
Pcie = false,
RomFile = "string",
Rombar = false,
Xvga = false,
},
},
Initialization = new ProxmoxVE.VM.Inputs.VirtualMachineInitializationArgs
{
DatastoreId = "string",
Dns = new ProxmoxVE.VM.Inputs.VirtualMachineInitializationDnsArgs
{
Domain = "string",
Servers = new[]
{
"string",
},
},
Interface = "string",
IpConfigs = new[]
{
new ProxmoxVE.VM.Inputs.VirtualMachineInitializationIpConfigArgs
{
Ipv4 = new ProxmoxVE.VM.Inputs.VirtualMachineInitializationIpConfigIpv4Args
{
Address = "string",
Gateway = "string",
},
Ipv6 = new ProxmoxVE.VM.Inputs.VirtualMachineInitializationIpConfigIpv6Args
{
Address = "string",
Gateway = "string",
},
},
},
MetaDataFileId = "string",
NetworkDataFileId = "string",
Type = "string",
UserAccount = new ProxmoxVE.VM.Inputs.VirtualMachineInitializationUserAccountArgs
{
Keys = new[]
{
"string",
},
Password = "string",
Username = "string",
},
UserDataFileId = "string",
VendorDataFileId = "string",
},
KeyboardLayout = "string",
KvmArguments = "string",
MacAddresses = new[]
{
"string",
},
Machine = "string",
Memory = new ProxmoxVE.VM.Inputs.VirtualMachineMemoryArgs
{
Dedicated = 0,
Floating = 0,
Hugepages = "string",
KeepHugepages = false,
Shared = 0,
},
Migrate = false,
Name = "string",
NetworkDevices = new[]
{
new ProxmoxVE.VM.Inputs.VirtualMachineNetworkDeviceArgs
{
Bridge = "string",
Disconnected = false,
Enabled = false,
Firewall = false,
MacAddress = "string",
Model = "string",
Mtu = 0,
Queues = 0,
RateLimit = 0,
Trunks = "string",
VlanId = 0,
},
},
Numas = new[]
{
new ProxmoxVE.VM.Inputs.VirtualMachineNumaArgs
{
Cpus = "string",
Device = "string",
Memory = 0,
Hostnodes = "string",
Policy = "string",
},
},
OnBoot = false,
OperatingSystem = new ProxmoxVE.VM.Inputs.VirtualMachineOperatingSystemArgs
{
Type = "string",
},
PoolId = "string",
Protection = false,
Reboot = false,
ScsiHardware = "string",
SerialDevices = new[]
{
new ProxmoxVE.VM.Inputs.VirtualMachineSerialDeviceArgs
{
Device = "string",
},
},
Smbios = new ProxmoxVE.VM.Inputs.VirtualMachineSmbiosArgs
{
Family = "string",
Manufacturer = "string",
Product = "string",
Serial = "string",
Sku = "string",
Uuid = "string",
Version = "string",
},
Started = false,
Startup = new ProxmoxVE.VM.Inputs.VirtualMachineStartupArgs
{
DownDelay = 0,
Order = 0,
UpDelay = 0,
},
StopOnDestroy = false,
TabletDevice = false,
Tags = new[]
{
"string",
},
Template = false,
TimeoutClone = 0,
TimeoutCreate = 0,
TimeoutMigrate = 0,
TimeoutReboot = 0,
TimeoutShutdownVm = 0,
TimeoutStartVm = 0,
TimeoutStopVm = 0,
TpmState = new ProxmoxVE.VM.Inputs.VirtualMachineTpmStateArgs
{
DatastoreId = "string",
Version = "string",
},
Usbs = new[]
{
new ProxmoxVE.VM.Inputs.VirtualMachineUsbArgs
{
Host = "string",
Mapping = "string",
Usb3 = false,
},
},
Vga = new ProxmoxVE.VM.Inputs.VirtualMachineVgaArgs
{
Clipboard = "string",
Memory = 0,
Type = "string",
},
VmId = 0,
});
example, err := VM.NewVirtualMachine(ctx, "virtualMachineResource", &VM.VirtualMachineArgs{
NodeName: pulumi.String("string"),
Acpi: pulumi.Bool(false),
Agent: &vm.VirtualMachineAgentArgs{
Enabled: pulumi.Bool(false),
Timeout: pulumi.String("string"),
Trim: pulumi.Bool(false),
Type: pulumi.String("string"),
},
AudioDevice: &vm.VirtualMachineAudioDeviceArgs{
Device: pulumi.String("string"),
Driver: pulumi.String("string"),
Enabled: pulumi.Bool(false),
},
Bios: pulumi.String("string"),
BootOrders: pulumi.StringArray{
pulumi.String("string"),
},
Cdrom: &vm.VirtualMachineCdromArgs{
Enabled: pulumi.Bool(false),
FileId: pulumi.String("string"),
Interface: pulumi.String("string"),
},
Clone: &vm.VirtualMachineCloneArgs{
VmId: pulumi.Int(0),
DatastoreId: pulumi.String("string"),
Full: pulumi.Bool(false),
NodeName: pulumi.String("string"),
Retries: pulumi.Int(0),
},
Cpu: &vm.VirtualMachineCpuArgs{
Affinity: pulumi.String("string"),
Architecture: pulumi.String("string"),
Cores: pulumi.Int(0),
Flags: pulumi.StringArray{
pulumi.String("string"),
},
Hotplugged: pulumi.Int(0),
Limit: pulumi.Int(0),
Numa: pulumi.Bool(false),
Sockets: pulumi.Int(0),
Type: pulumi.String("string"),
Units: pulumi.Int(0),
},
Description: pulumi.String("string"),
Disks: vm.VirtualMachineDiskArray{
&vm.VirtualMachineDiskArgs{
Interface: pulumi.String("string"),
FileId: pulumi.String("string"),
Iothread: pulumi.Bool(false),
DatastoreId: pulumi.String("string"),
Discard: pulumi.String("string"),
FileFormat: pulumi.String("string"),
Aio: pulumi.String("string"),
Backup: pulumi.Bool(false),
Cache: pulumi.String("string"),
PathInDatastore: pulumi.String("string"),
Replicate: pulumi.Bool(false),
Serial: pulumi.String("string"),
Size: pulumi.Int(0),
Speed: &vm.VirtualMachineDiskSpeedArgs{
IopsRead: pulumi.Int(0),
IopsReadBurstable: pulumi.Int(0),
IopsWrite: pulumi.Int(0),
IopsWriteBurstable: pulumi.Int(0),
Read: pulumi.Int(0),
ReadBurstable: pulumi.Int(0),
Write: pulumi.Int(0),
WriteBurstable: pulumi.Int(0),
},
Ssd: pulumi.Bool(false),
},
},
EfiDisk: &vm.VirtualMachineEfiDiskArgs{
DatastoreId: pulumi.String("string"),
FileFormat: pulumi.String("string"),
PreEnrolledKeys: pulumi.Bool(false),
Type: pulumi.String("string"),
},
HookScriptFileId: pulumi.String("string"),
Hostpcis: vm.VirtualMachineHostpciArray{
&vm.VirtualMachineHostpciArgs{
Device: pulumi.String("string"),
Id: pulumi.String("string"),
Mapping: pulumi.String("string"),
Mdev: pulumi.String("string"),
Pcie: pulumi.Bool(false),
RomFile: pulumi.String("string"),
Rombar: pulumi.Bool(false),
Xvga: pulumi.Bool(false),
},
},
Initialization: &vm.VirtualMachineInitializationArgs{
DatastoreId: pulumi.String("string"),
Dns: &vm.VirtualMachineInitializationDnsArgs{
Domain: pulumi.String("string"),
Servers: pulumi.StringArray{
pulumi.String("string"),
},
},
Interface: pulumi.String("string"),
IpConfigs: vm.VirtualMachineInitializationIpConfigArray{
&vm.VirtualMachineInitializationIpConfigArgs{
Ipv4: &vm.VirtualMachineInitializationIpConfigIpv4Args{
Address: pulumi.String("string"),
Gateway: pulumi.String("string"),
},
Ipv6: &vm.VirtualMachineInitializationIpConfigIpv6Args{
Address: pulumi.String("string"),
Gateway: pulumi.String("string"),
},
},
},
MetaDataFileId: pulumi.String("string"),
NetworkDataFileId: pulumi.String("string"),
Type: pulumi.String("string"),
UserAccount: &vm.VirtualMachineInitializationUserAccountArgs{
Keys: pulumi.StringArray{
pulumi.String("string"),
},
Password: pulumi.String("string"),
Username: pulumi.String("string"),
},
UserDataFileId: pulumi.String("string"),
VendorDataFileId: pulumi.String("string"),
},
KeyboardLayout: pulumi.String("string"),
KvmArguments: pulumi.String("string"),
MacAddresses: pulumi.StringArray{
pulumi.String("string"),
},
Machine: pulumi.String("string"),
Memory: &vm.VirtualMachineMemoryArgs{
Dedicated: pulumi.Int(0),
Floating: pulumi.Int(0),
Hugepages: pulumi.String("string"),
KeepHugepages: pulumi.Bool(false),
Shared: pulumi.Int(0),
},
Migrate: pulumi.Bool(false),
Name: pulumi.String("string"),
NetworkDevices: vm.VirtualMachineNetworkDeviceArray{
&vm.VirtualMachineNetworkDeviceArgs{
Bridge: pulumi.String("string"),
Disconnected: pulumi.Bool(false),
Enabled: pulumi.Bool(false),
Firewall: pulumi.Bool(false),
MacAddress: pulumi.String("string"),
Model: pulumi.String("string"),
Mtu: pulumi.Int(0),
Queues: pulumi.Int(0),
RateLimit: pulumi.Float64(0),
Trunks: pulumi.String("string"),
VlanId: pulumi.Int(0),
},
},
Numas: vm.VirtualMachineNumaArray{
&vm.VirtualMachineNumaArgs{
Cpus: pulumi.String("string"),
Device: pulumi.String("string"),
Memory: pulumi.Int(0),
Hostnodes: pulumi.String("string"),
Policy: pulumi.String("string"),
},
},
OnBoot: pulumi.Bool(false),
OperatingSystem: &vm.VirtualMachineOperatingSystemArgs{
Type: pulumi.String("string"),
},
PoolId: pulumi.String("string"),
Protection: pulumi.Bool(false),
Reboot: pulumi.Bool(false),
ScsiHardware: pulumi.String("string"),
SerialDevices: vm.VirtualMachineSerialDeviceArray{
&vm.VirtualMachineSerialDeviceArgs{
Device: pulumi.String("string"),
},
},
Smbios: &vm.VirtualMachineSmbiosArgs{
Family: pulumi.String("string"),
Manufacturer: pulumi.String("string"),
Product: pulumi.String("string"),
Serial: pulumi.String("string"),
Sku: pulumi.String("string"),
Uuid: pulumi.String("string"),
Version: pulumi.String("string"),
},
Started: pulumi.Bool(false),
Startup: &vm.VirtualMachineStartupArgs{
DownDelay: pulumi.Int(0),
Order: pulumi.Int(0),
UpDelay: pulumi.Int(0),
},
StopOnDestroy: pulumi.Bool(false),
TabletDevice: pulumi.Bool(false),
Tags: pulumi.StringArray{
pulumi.String("string"),
},
Template: pulumi.Bool(false),
TimeoutClone: pulumi.Int(0),
TimeoutCreate: pulumi.Int(0),
TimeoutMigrate: pulumi.Int(0),
TimeoutReboot: pulumi.Int(0),
TimeoutShutdownVm: pulumi.Int(0),
TimeoutStartVm: pulumi.Int(0),
TimeoutStopVm: pulumi.Int(0),
TpmState: &vm.VirtualMachineTpmStateArgs{
DatastoreId: pulumi.String("string"),
Version: pulumi.String("string"),
},
Usbs: vm.VirtualMachineUsbArray{
&vm.VirtualMachineUsbArgs{
Host: pulumi.String("string"),
Mapping: pulumi.String("string"),
Usb3: pulumi.Bool(false),
},
},
Vga: &vm.VirtualMachineVgaArgs{
Clipboard: pulumi.String("string"),
Memory: pulumi.Int(0),
Type: pulumi.String("string"),
},
VmId: pulumi.Int(0),
})
var virtualMachineResource = new VirtualMachine("virtualMachineResource", VirtualMachineArgs.builder()
.nodeName("string")
.acpi(false)
.agent(VirtualMachineAgentArgs.builder()
.enabled(false)
.timeout("string")
.trim(false)
.type("string")
.build())
.audioDevice(VirtualMachineAudioDeviceArgs.builder()
.device("string")
.driver("string")
.enabled(false)
.build())
.bios("string")
.bootOrders("string")
.cdrom(VirtualMachineCdromArgs.builder()
.enabled(false)
.fileId("string")
.interface_("string")
.build())
.clone(VirtualMachineCloneArgs.builder()
.vmId(0)
.datastoreId("string")
.full(false)
.nodeName("string")
.retries(0)
.build())
.cpu(VirtualMachineCpuArgs.builder()
.affinity("string")
.architecture("string")
.cores(0)
.flags("string")
.hotplugged(0)
.limit(0)
.numa(false)
.sockets(0)
.type("string")
.units(0)
.build())
.description("string")
.disks(VirtualMachineDiskArgs.builder()
.interface_("string")
.fileId("string")
.iothread(false)
.datastoreId("string")
.discard("string")
.fileFormat("string")
.aio("string")
.backup(false)
.cache("string")
.pathInDatastore("string")
.replicate(false)
.serial("string")
.size(0)
.speed(VirtualMachineDiskSpeedArgs.builder()
.iopsRead(0)
.iopsReadBurstable(0)
.iopsWrite(0)
.iopsWriteBurstable(0)
.read(0)
.readBurstable(0)
.write(0)
.writeBurstable(0)
.build())
.ssd(false)
.build())
.efiDisk(VirtualMachineEfiDiskArgs.builder()
.datastoreId("string")
.fileFormat("string")
.preEnrolledKeys(false)
.type("string")
.build())
.hookScriptFileId("string")
.hostpcis(VirtualMachineHostpciArgs.builder()
.device("string")
.id("string")
.mapping("string")
.mdev("string")
.pcie(false)
.romFile("string")
.rombar(false)
.xvga(false)
.build())
.initialization(VirtualMachineInitializationArgs.builder()
.datastoreId("string")
.dns(VirtualMachineInitializationDnsArgs.builder()
.domain("string")
.servers("string")
.build())
.interface_("string")
.ipConfigs(VirtualMachineInitializationIpConfigArgs.builder()
.ipv4(VirtualMachineInitializationIpConfigIpv4Args.builder()
.address("string")
.gateway("string")
.build())
.ipv6(VirtualMachineInitializationIpConfigIpv6Args.builder()
.address("string")
.gateway("string")
.build())
.build())
.metaDataFileId("string")
.networkDataFileId("string")
.type("string")
.userAccount(VirtualMachineInitializationUserAccountArgs.builder()
.keys("string")
.password("string")
.username("string")
.build())
.userDataFileId("string")
.vendorDataFileId("string")
.build())
.keyboardLayout("string")
.kvmArguments("string")
.macAddresses("string")
.machine("string")
.memory(VirtualMachineMemoryArgs.builder()
.dedicated(0)
.floating(0)
.hugepages("string")
.keepHugepages(false)
.shared(0)
.build())
.migrate(false)
.name("string")
.networkDevices(VirtualMachineNetworkDeviceArgs.builder()
.bridge("string")
.disconnected(false)
.enabled(false)
.firewall(false)
.macAddress("string")
.model("string")
.mtu(0)
.queues(0)
.rateLimit(0)
.trunks("string")
.vlanId(0)
.build())
.numas(VirtualMachineNumaArgs.builder()
.cpus("string")
.device("string")
.memory(0)
.hostnodes("string")
.policy("string")
.build())
.onBoot(false)
.operatingSystem(VirtualMachineOperatingSystemArgs.builder()
.type("string")
.build())
.poolId("string")
.protection(false)
.reboot(false)
.scsiHardware("string")
.serialDevices(VirtualMachineSerialDeviceArgs.builder()
.device("string")
.build())
.smbios(VirtualMachineSmbiosArgs.builder()
.family("string")
.manufacturer("string")
.product("string")
.serial("string")
.sku("string")
.uuid("string")
.version("string")
.build())
.started(false)
.startup(VirtualMachineStartupArgs.builder()
.downDelay(0)
.order(0)
.upDelay(0)
.build())
.stopOnDestroy(false)
.tabletDevice(false)
.tags("string")
.template(false)
.timeoutClone(0)
.timeoutCreate(0)
.timeoutMigrate(0)
.timeoutReboot(0)
.timeoutShutdownVm(0)
.timeoutStartVm(0)
.timeoutStopVm(0)
.tpmState(VirtualMachineTpmStateArgs.builder()
.datastoreId("string")
.version("string")
.build())
.usbs(VirtualMachineUsbArgs.builder()
.host("string")
.mapping("string")
.usb3(false)
.build())
.vga(VirtualMachineVgaArgs.builder()
.clipboard("string")
.memory(0)
.type("string")
.build())
.vmId(0)
.build());
virtual_machine_resource = proxmoxve.vm.VirtualMachine("virtualMachineResource",
node_name="string",
acpi=False,
agent=proxmoxve.vm.VirtualMachineAgentArgs(
enabled=False,
timeout="string",
trim=False,
type="string",
),
audio_device=proxmoxve.vm.VirtualMachineAudioDeviceArgs(
device="string",
driver="string",
enabled=False,
),
bios="string",
boot_orders=["string"],
cdrom=proxmoxve.vm.VirtualMachineCdromArgs(
enabled=False,
file_id="string",
interface="string",
),
clone=proxmoxve.vm.VirtualMachineCloneArgs(
vm_id=0,
datastore_id="string",
full=False,
node_name="string",
retries=0,
),
cpu=proxmoxve.vm.VirtualMachineCpuArgs(
affinity="string",
architecture="string",
cores=0,
flags=["string"],
hotplugged=0,
limit=0,
numa=False,
sockets=0,
type="string",
units=0,
),
description="string",
disks=[proxmoxve.vm.VirtualMachineDiskArgs(
interface="string",
file_id="string",
iothread=False,
datastore_id="string",
discard="string",
file_format="string",
aio="string",
backup=False,
cache="string",
path_in_datastore="string",
replicate=False,
serial="string",
size=0,
speed=proxmoxve.vm.VirtualMachineDiskSpeedArgs(
iops_read=0,
iops_read_burstable=0,
iops_write=0,
iops_write_burstable=0,
read=0,
read_burstable=0,
write=0,
write_burstable=0,
),
ssd=False,
)],
efi_disk=proxmoxve.vm.VirtualMachineEfiDiskArgs(
datastore_id="string",
file_format="string",
pre_enrolled_keys=False,
type="string",
),
hook_script_file_id="string",
hostpcis=[proxmoxve.vm.VirtualMachineHostpciArgs(
device="string",
id="string",
mapping="string",
mdev="string",
pcie=False,
rom_file="string",
rombar=False,
xvga=False,
)],
initialization=proxmoxve.vm.VirtualMachineInitializationArgs(
datastore_id="string",
dns=proxmoxve.vm.VirtualMachineInitializationDnsArgs(
domain="string",
servers=["string"],
),
interface="string",
ip_configs=[proxmoxve.vm.VirtualMachineInitializationIpConfigArgs(
ipv4=proxmoxve.vm.VirtualMachineInitializationIpConfigIpv4Args(
address="string",
gateway="string",
),
ipv6=proxmoxve.vm.VirtualMachineInitializationIpConfigIpv6Args(
address="string",
gateway="string",
),
)],
meta_data_file_id="string",
network_data_file_id="string",
type="string",
user_account=proxmoxve.vm.VirtualMachineInitializationUserAccountArgs(
keys=["string"],
password="string",
username="string",
),
user_data_file_id="string",
vendor_data_file_id="string",
),
keyboard_layout="string",
kvm_arguments="string",
mac_addresses=["string"],
machine="string",
memory=proxmoxve.vm.VirtualMachineMemoryArgs(
dedicated=0,
floating=0,
hugepages="string",
keep_hugepages=False,
shared=0,
),
migrate=False,
name="string",
network_devices=[proxmoxve.vm.VirtualMachineNetworkDeviceArgs(
bridge="string",
disconnected=False,
enabled=False,
firewall=False,
mac_address="string",
model="string",
mtu=0,
queues=0,
rate_limit=0,
trunks="string",
vlan_id=0,
)],
numas=[proxmoxve.vm.VirtualMachineNumaArgs(
cpus="string",
device="string",
memory=0,
hostnodes="string",
policy="string",
)],
on_boot=False,
operating_system=proxmoxve.vm.VirtualMachineOperatingSystemArgs(
type="string",
),
pool_id="string",
protection=False,
reboot=False,
scsi_hardware="string",
serial_devices=[proxmoxve.vm.VirtualMachineSerialDeviceArgs(
device="string",
)],
smbios=proxmoxve.vm.VirtualMachineSmbiosArgs(
family="string",
manufacturer="string",
product="string",
serial="string",
sku="string",
uuid="string",
version="string",
),
started=False,
startup=proxmoxve.vm.VirtualMachineStartupArgs(
down_delay=0,
order=0,
up_delay=0,
),
stop_on_destroy=False,
tablet_device=False,
tags=["string"],
template=False,
timeout_clone=0,
timeout_create=0,
timeout_migrate=0,
timeout_reboot=0,
timeout_shutdown_vm=0,
timeout_start_vm=0,
timeout_stop_vm=0,
tpm_state=proxmoxve.vm.VirtualMachineTpmStateArgs(
datastore_id="string",
version="string",
),
usbs=[proxmoxve.vm.VirtualMachineUsbArgs(
host="string",
mapping="string",
usb3=False,
)],
vga=proxmoxve.vm.VirtualMachineVgaArgs(
clipboard="string",
memory=0,
type="string",
),
vm_id=0)
const virtualMachineResource = new proxmoxve.vm.VirtualMachine("virtualMachineResource", {
nodeName: "string",
acpi: false,
agent: {
enabled: false,
timeout: "string",
trim: false,
type: "string",
},
audioDevice: {
device: "string",
driver: "string",
enabled: false,
},
bios: "string",
bootOrders: ["string"],
cdrom: {
enabled: false,
fileId: "string",
"interface": "string",
},
clone: {
vmId: 0,
datastoreId: "string",
full: false,
nodeName: "string",
retries: 0,
},
cpu: {
affinity: "string",
architecture: "string",
cores: 0,
flags: ["string"],
hotplugged: 0,
limit: 0,
numa: false,
sockets: 0,
type: "string",
units: 0,
},
description: "string",
disks: [{
"interface": "string",
fileId: "string",
iothread: false,
datastoreId: "string",
discard: "string",
fileFormat: "string",
aio: "string",
backup: false,
cache: "string",
pathInDatastore: "string",
replicate: false,
serial: "string",
size: 0,
speed: {
iopsRead: 0,
iopsReadBurstable: 0,
iopsWrite: 0,
iopsWriteBurstable: 0,
read: 0,
readBurstable: 0,
write: 0,
writeBurstable: 0,
},
ssd: false,
}],
efiDisk: {
datastoreId: "string",
fileFormat: "string",
preEnrolledKeys: false,
type: "string",
},
hookScriptFileId: "string",
hostpcis: [{
device: "string",
id: "string",
mapping: "string",
mdev: "string",
pcie: false,
romFile: "string",
rombar: false,
xvga: false,
}],
initialization: {
datastoreId: "string",
dns: {
domain: "string",
servers: ["string"],
},
"interface": "string",
ipConfigs: [{
ipv4: {
address: "string",
gateway: "string",
},
ipv6: {
address: "string",
gateway: "string",
},
}],
metaDataFileId: "string",
networkDataFileId: "string",
type: "string",
userAccount: {
keys: ["string"],
password: "string",
username: "string",
},
userDataFileId: "string",
vendorDataFileId: "string",
},
keyboardLayout: "string",
kvmArguments: "string",
macAddresses: ["string"],
machine: "string",
memory: {
dedicated: 0,
floating: 0,
hugepages: "string",
keepHugepages: false,
shared: 0,
},
migrate: false,
name: "string",
networkDevices: [{
bridge: "string",
disconnected: false,
enabled: false,
firewall: false,
macAddress: "string",
model: "string",
mtu: 0,
queues: 0,
rateLimit: 0,
trunks: "string",
vlanId: 0,
}],
numas: [{
cpus: "string",
device: "string",
memory: 0,
hostnodes: "string",
policy: "string",
}],
onBoot: false,
operatingSystem: {
type: "string",
},
poolId: "string",
protection: false,
reboot: false,
scsiHardware: "string",
serialDevices: [{
device: "string",
}],
smbios: {
family: "string",
manufacturer: "string",
product: "string",
serial: "string",
sku: "string",
uuid: "string",
version: "string",
},
started: false,
startup: {
downDelay: 0,
order: 0,
upDelay: 0,
},
stopOnDestroy: false,
tabletDevice: false,
tags: ["string"],
template: false,
timeoutClone: 0,
timeoutCreate: 0,
timeoutMigrate: 0,
timeoutReboot: 0,
timeoutShutdownVm: 0,
timeoutStartVm: 0,
timeoutStopVm: 0,
tpmState: {
datastoreId: "string",
version: "string",
},
usbs: [{
host: "string",
mapping: "string",
usb3: false,
}],
vga: {
clipboard: "string",
memory: 0,
type: "string",
},
vmId: 0,
});
type: proxmoxve:VM:VirtualMachine
properties:
acpi: false
agent:
enabled: false
timeout: string
trim: false
type: string
audioDevice:
device: string
driver: string
enabled: false
bios: string
bootOrders:
- string
cdrom:
enabled: false
fileId: string
interface: string
clone:
datastoreId: string
full: false
nodeName: string
retries: 0
vmId: 0
cpu:
affinity: string
architecture: string
cores: 0
flags:
- string
hotplugged: 0
limit: 0
numa: false
sockets: 0
type: string
units: 0
description: string
disks:
- aio: string
backup: false
cache: string
datastoreId: string
discard: string
fileFormat: string
fileId: string
interface: string
iothread: false
pathInDatastore: string
replicate: false
serial: string
size: 0
speed:
iopsRead: 0
iopsReadBurstable: 0
iopsWrite: 0
iopsWriteBurstable: 0
read: 0
readBurstable: 0
write: 0
writeBurstable: 0
ssd: false
efiDisk:
datastoreId: string
fileFormat: string
preEnrolledKeys: false
type: string
hookScriptFileId: string
hostpcis:
- device: string
id: string
mapping: string
mdev: string
pcie: false
romFile: string
rombar: false
xvga: false
initialization:
datastoreId: string
dns:
domain: string
servers:
- string
interface: string
ipConfigs:
- ipv4:
address: string
gateway: string
ipv6:
address: string
gateway: string
metaDataFileId: string
networkDataFileId: string
type: string
userAccount:
keys:
- string
password: string
username: string
userDataFileId: string
vendorDataFileId: string
keyboardLayout: string
kvmArguments: string
macAddresses:
- string
machine: string
memory:
dedicated: 0
floating: 0
hugepages: string
keepHugepages: false
shared: 0
migrate: false
name: string
networkDevices:
- bridge: string
disconnected: false
enabled: false
firewall: false
macAddress: string
model: string
mtu: 0
queues: 0
rateLimit: 0
trunks: string
vlanId: 0
nodeName: string
numas:
- cpus: string
device: string
hostnodes: string
memory: 0
policy: string
onBoot: false
operatingSystem:
type: string
poolId: string
protection: false
reboot: false
scsiHardware: string
serialDevices:
- device: string
smbios:
family: string
manufacturer: string
product: string
serial: string
sku: string
uuid: string
version: string
started: false
startup:
downDelay: 0
order: 0
upDelay: 0
stopOnDestroy: false
tabletDevice: false
tags:
- string
template: false
timeoutClone: 0
timeoutCreate: 0
timeoutMigrate: 0
timeoutReboot: 0
timeoutShutdownVm: 0
timeoutStartVm: 0
timeoutStopVm: 0
tpmState:
datastoreId: string
version: string
usbs:
- host: string
mapping: string
usb3: false
vga:
clipboard: string
memory: 0
type: string
vmId: 0
VirtualMachine Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The VirtualMachine resource accepts the following input properties:
- Node
Name string - The name of the node to assign the virtual machine to.
- Acpi bool
- Whether to enable ACPI (defaults to
true
). - Agent
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Agent - The QEMU agent configuration.
- Audio
Device Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Audio Device - An audio device.
- Bios string
- The BIOS implementation (defaults to
seabios
). - Boot
Orders List<string> - Specify a list of devices to boot from in the order
they appear in the list (defaults to
[]
). - Cdrom
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Cdrom - The CDROM configuration.
- Clone
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Clone - The cloning configuration.
- Cpu
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Cpu - The CPU configuration.
- Description string
- The description.
- Disks
List<Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Disk> - A disk (multiple blocks supported).
- Efi
Disk Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Efi Disk - The efi disk device (required if
bios
is set toovmf
) - Hook
Script stringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - Hostpcis
List<Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Hostpci> - A host PCI device mapping (multiple blocks supported).
- Initialization
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Initialization - The cloud-init configuration.
- Keyboard
Layout string - The keyboard layout (defaults to
en-us
). - Kvm
Arguments string - Arbitrary arguments passed to kvm.
- Mac
Addresses List<string> - The MAC addresses published by the QEMU agent with fallback to the network device configuration, if the agent is disabled
- Machine string
- The VM machine type (defaults to
pc
). - Memory
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Memory - The memory configuration.
- Migrate bool
- Migrate the VM on node change instead of re-creating
it (defaults to
false
). - Name string
- The virtual machine name.
- Network
Devices List<Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Network Device> - A network device (multiple blocks supported).
- Numas
List<Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Numa> - The NUMA configuration.
- On
Boot bool - Specifies whether a VM will be started during system
boot. (defaults to
true
) - Operating
System Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Operating System - The Operating System configuration.
- Pool
Id string - The identifier for a pool to assign the virtual machine to.
- Protection bool
- Sets the protection flag of the VM. This will disable the remove VM and remove disk operations (defaults to
false
). - Reboot bool
- Reboot the VM after initial creation. (defaults to
false
) - Scsi
Hardware string - The SCSI hardware type (defaults to
virtio-scsi-pci
). - Serial
Devices List<Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Serial Device> - A serial device (multiple blocks supported).
- Smbios
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Smbios - The SMBIOS (type1) settings for the VM.
- Started bool
- Whether to start the virtual machine (defaults
to
true
). - Startup
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Startup - Defines startup and shutdown behavior of the VM.
- Stop
On boolDestroy - Whether to stop rather than shutdown on VM destroy (defaults to
false
) - Tablet
Device bool - Whether to enable the USB tablet device (defaults
to
true
). - List<string>
- A list of tags of the VM. This is only meta information (
defaults to
[]
). Note: Proxmox always sorts the VM tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - Template bool
- Whether to create a template (defaults to
false
). - Timeout
Clone int - Timeout for cloning a VM in seconds (defaults to 1800).
- Timeout
Create int - Timeout for creating a VM in seconds (defaults to 1800).
- Timeout
Migrate int - Timeout for migrating the VM (defaults to 1800).
- Timeout
Move intDisk - MoveDisk timeout
- Timeout
Reboot int - Timeout for rebooting a VM in seconds (defaults to 1800).
- Timeout
Shutdown intVm - Timeout for shutting down a VM in seconds ( defaults to 1800).
- Timeout
Start intVm - Timeout for starting a VM in seconds (defaults to 1800).
- Timeout
Stop intVm - Timeout for stopping a VM in seconds (defaults to 300).
- Tpm
State Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Tpm State - The TPM state device.
- Usbs
List<Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Usb> - A host USB device mapping (multiple blocks supported).
- Vga
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Vga - The VGA configuration.
- Vm
Id int - The VM identifier.
- Node
Name string - The name of the node to assign the virtual machine to.
- Acpi bool
- Whether to enable ACPI (defaults to
true
). - Agent
Virtual
Machine Agent Args - The QEMU agent configuration.
- Audio
Device VirtualMachine Audio Device Args - An audio device.
- Bios string
- The BIOS implementation (defaults to
seabios
). - Boot
Orders []string - Specify a list of devices to boot from in the order
they appear in the list (defaults to
[]
). - Cdrom
Virtual
Machine Cdrom Args - The CDROM configuration.
- Clone
Virtual
Machine Clone Args - The cloning configuration.
- Cpu
Virtual
Machine Cpu Args - The CPU configuration.
- Description string
- The description.
- Disks
[]Virtual
Machine Disk Args - A disk (multiple blocks supported).
- Efi
Disk VirtualMachine Efi Disk Args - The efi disk device (required if
bios
is set toovmf
) - Hook
Script stringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - Hostpcis
[]Virtual
Machine Hostpci Args - A host PCI device mapping (multiple blocks supported).
- Initialization
Virtual
Machine Initialization Args - The cloud-init configuration.
- Keyboard
Layout string - The keyboard layout (defaults to
en-us
). - Kvm
Arguments string - Arbitrary arguments passed to kvm.
- Mac
Addresses []string - The MAC addresses published by the QEMU agent with fallback to the network device configuration, if the agent is disabled
- Machine string
- The VM machine type (defaults to
pc
). - Memory
Virtual
Machine Memory Args - The memory configuration.
- Migrate bool
- Migrate the VM on node change instead of re-creating
it (defaults to
false
). - Name string
- The virtual machine name.
- Network
Devices []VirtualMachine Network Device Args - A network device (multiple blocks supported).
- Numas
[]Virtual
Machine Numa Args - The NUMA configuration.
- On
Boot bool - Specifies whether a VM will be started during system
boot. (defaults to
true
) - Operating
System VirtualMachine Operating System Args - The Operating System configuration.
- Pool
Id string - The identifier for a pool to assign the virtual machine to.
- Protection bool
- Sets the protection flag of the VM. This will disable the remove VM and remove disk operations (defaults to
false
). - Reboot bool
- Reboot the VM after initial creation. (defaults to
false
) - Scsi
Hardware string - The SCSI hardware type (defaults to
virtio-scsi-pci
). - Serial
Devices []VirtualMachine Serial Device Args - A serial device (multiple blocks supported).
- Smbios
Virtual
Machine Smbios Args - The SMBIOS (type1) settings for the VM.
- Started bool
- Whether to start the virtual machine (defaults
to
true
). - Startup
Virtual
Machine Startup Args - Defines startup and shutdown behavior of the VM.
- Stop
On boolDestroy - Whether to stop rather than shutdown on VM destroy (defaults to
false
) - Tablet
Device bool - Whether to enable the USB tablet device (defaults
to
true
). - []string
- A list of tags of the VM. This is only meta information (
defaults to
[]
). Note: Proxmox always sorts the VM tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - Template bool
- Whether to create a template (defaults to
false
). - Timeout
Clone int - Timeout for cloning a VM in seconds (defaults to 1800).
- Timeout
Create int - Timeout for creating a VM in seconds (defaults to 1800).
- Timeout
Migrate int - Timeout for migrating the VM (defaults to 1800).
- Timeout
Move intDisk - MoveDisk timeout
- Timeout
Reboot int - Timeout for rebooting a VM in seconds (defaults to 1800).
- Timeout
Shutdown intVm - Timeout for shutting down a VM in seconds ( defaults to 1800).
- Timeout
Start intVm - Timeout for starting a VM in seconds (defaults to 1800).
- Timeout
Stop intVm - Timeout for stopping a VM in seconds (defaults to 300).
- Tpm
State VirtualMachine Tpm State Args - The TPM state device.
- Usbs
[]Virtual
Machine Usb Args - A host USB device mapping (multiple blocks supported).
- Vga
Virtual
Machine Vga Args - The VGA configuration.
- Vm
Id int - The VM identifier.
- node
Name String - The name of the node to assign the virtual machine to.
- acpi Boolean
- Whether to enable ACPI (defaults to
true
). - agent
Virtual
Machine Agent - The QEMU agent configuration.
- audio
Device VirtualMachine Audio Device - An audio device.
- bios String
- The BIOS implementation (defaults to
seabios
). - boot
Orders List<String> - Specify a list of devices to boot from in the order
they appear in the list (defaults to
[]
). - cdrom
Virtual
Machine Cdrom - The CDROM configuration.
- clone_
Virtual
Machine Clone - The cloning configuration.
- cpu
Virtual
Machine Cpu - The CPU configuration.
- description String
- The description.
- disks
List<Virtual
Machine Disk> - A disk (multiple blocks supported).
- efi
Disk VirtualMachine Efi Disk - The efi disk device (required if
bios
is set toovmf
) - hook
Script StringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - hostpcis
List<Virtual
Machine Hostpci> - A host PCI device mapping (multiple blocks supported).
- initialization
Virtual
Machine Initialization - The cloud-init configuration.
- keyboard
Layout String - The keyboard layout (defaults to
en-us
). - kvm
Arguments String - Arbitrary arguments passed to kvm.
- mac
Addresses List<String> - The MAC addresses published by the QEMU agent with fallback to the network device configuration, if the agent is disabled
- machine String
- The VM machine type (defaults to
pc
). - memory
Virtual
Machine Memory - The memory configuration.
- migrate Boolean
- Migrate the VM on node change instead of re-creating
it (defaults to
false
). - name String
- The virtual machine name.
- network
Devices List<VirtualMachine Network Device> - A network device (multiple blocks supported).
- numas
List<Virtual
Machine Numa> - The NUMA configuration.
- on
Boot Boolean - Specifies whether a VM will be started during system
boot. (defaults to
true
) - operating
System VirtualMachine Operating System - The Operating System configuration.
- pool
Id String - The identifier for a pool to assign the virtual machine to.
- protection Boolean
- Sets the protection flag of the VM. This will disable the remove VM and remove disk operations (defaults to
false
). - reboot Boolean
- Reboot the VM after initial creation. (defaults to
false
) - scsi
Hardware String - The SCSI hardware type (defaults to
virtio-scsi-pci
). - serial
Devices List<VirtualMachine Serial Device> - A serial device (multiple blocks supported).
- smbios
Virtual
Machine Smbios - The SMBIOS (type1) settings for the VM.
- started Boolean
- Whether to start the virtual machine (defaults
to
true
). - startup
Virtual
Machine Startup - Defines startup and shutdown behavior of the VM.
- stop
On BooleanDestroy - Whether to stop rather than shutdown on VM destroy (defaults to
false
) - tablet
Device Boolean - Whether to enable the USB tablet device (defaults
to
true
). - List<String>
- A list of tags of the VM. This is only meta information (
defaults to
[]
). Note: Proxmox always sorts the VM tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template Boolean
- Whether to create a template (defaults to
false
). - timeout
Clone Integer - Timeout for cloning a VM in seconds (defaults to 1800).
- timeout
Create Integer - Timeout for creating a VM in seconds (defaults to 1800).
- timeout
Migrate Integer - Timeout for migrating the VM (defaults to 1800).
- timeout
Move IntegerDisk - MoveDisk timeout
- timeout
Reboot Integer - Timeout for rebooting a VM in seconds (defaults to 1800).
- timeout
Shutdown IntegerVm - Timeout for shutting down a VM in seconds ( defaults to 1800).
- timeout
Start IntegerVm - Timeout for starting a VM in seconds (defaults to 1800).
- timeout
Stop IntegerVm - Timeout for stopping a VM in seconds (defaults to 300).
- tpm
State VirtualMachine Tpm State - The TPM state device.
- usbs
List<Virtual
Machine Usb> - A host USB device mapping (multiple blocks supported).
- vga
Virtual
Machine Vga - The VGA configuration.
- vm
Id Integer - The VM identifier.
- node
Name string - The name of the node to assign the virtual machine to.
- acpi boolean
- Whether to enable ACPI (defaults to
true
). - agent
Virtual
Machine Agent - The QEMU agent configuration.
- audio
Device VirtualMachine Audio Device - An audio device.
- bios string
- The BIOS implementation (defaults to
seabios
). - boot
Orders string[] - Specify a list of devices to boot from in the order
they appear in the list (defaults to
[]
). - cdrom
Virtual
Machine Cdrom - The CDROM configuration.
- clone
Virtual
Machine Clone - The cloning configuration.
- cpu
Virtual
Machine Cpu - The CPU configuration.
- description string
- The description.
- disks
Virtual
Machine Disk[] - A disk (multiple blocks supported).
- efi
Disk VirtualMachine Efi Disk - The efi disk device (required if
bios
is set toovmf
) - hook
Script stringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - hostpcis
Virtual
Machine Hostpci[] - A host PCI device mapping (multiple blocks supported).
- initialization
Virtual
Machine Initialization - The cloud-init configuration.
- keyboard
Layout string - The keyboard layout (defaults to
en-us
). - kvm
Arguments string - Arbitrary arguments passed to kvm.
- mac
Addresses string[] - The MAC addresses published by the QEMU agent with fallback to the network device configuration, if the agent is disabled
- machine string
- The VM machine type (defaults to
pc
). - memory
Virtual
Machine Memory - The memory configuration.
- migrate boolean
- Migrate the VM on node change instead of re-creating
it (defaults to
false
). - name string
- The virtual machine name.
- network
Devices VirtualMachine Network Device[] - A network device (multiple blocks supported).
- numas
Virtual
Machine Numa[] - The NUMA configuration.
- on
Boot boolean - Specifies whether a VM will be started during system
boot. (defaults to
true
) - operating
System VirtualMachine Operating System - The Operating System configuration.
- pool
Id string - The identifier for a pool to assign the virtual machine to.
- protection boolean
- Sets the protection flag of the VM. This will disable the remove VM and remove disk operations (defaults to
false
). - reboot boolean
- Reboot the VM after initial creation. (defaults to
false
) - scsi
Hardware string - The SCSI hardware type (defaults to
virtio-scsi-pci
). - serial
Devices VirtualMachine Serial Device[] - A serial device (multiple blocks supported).
- smbios
Virtual
Machine Smbios - The SMBIOS (type1) settings for the VM.
- started boolean
- Whether to start the virtual machine (defaults
to
true
). - startup
Virtual
Machine Startup - Defines startup and shutdown behavior of the VM.
- stop
On booleanDestroy - Whether to stop rather than shutdown on VM destroy (defaults to
false
) - tablet
Device boolean - Whether to enable the USB tablet device (defaults
to
true
). - string[]
- A list of tags of the VM. This is only meta information (
defaults to
[]
). Note: Proxmox always sorts the VM tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template boolean
- Whether to create a template (defaults to
false
). - timeout
Clone number - Timeout for cloning a VM in seconds (defaults to 1800).
- timeout
Create number - Timeout for creating a VM in seconds (defaults to 1800).
- timeout
Migrate number - Timeout for migrating the VM (defaults to 1800).
- timeout
Move numberDisk - MoveDisk timeout
- timeout
Reboot number - Timeout for rebooting a VM in seconds (defaults to 1800).
- timeout
Shutdown numberVm - Timeout for shutting down a VM in seconds ( defaults to 1800).
- timeout
Start numberVm - Timeout for starting a VM in seconds (defaults to 1800).
- timeout
Stop numberVm - Timeout for stopping a VM in seconds (defaults to 300).
- tpm
State VirtualMachine Tpm State - The TPM state device.
- usbs
Virtual
Machine Usb[] - A host USB device mapping (multiple blocks supported).
- vga
Virtual
Machine Vga - The VGA configuration.
- vm
Id number - The VM identifier.
- node_
name str - The name of the node to assign the virtual machine to.
- acpi bool
- Whether to enable ACPI (defaults to
true
). - agent
vm.
Virtual Machine Agent Args - The QEMU agent configuration.
- audio_
device vm.Virtual Machine Audio Device Args - An audio device.
- bios str
- The BIOS implementation (defaults to
seabios
). - boot_
orders Sequence[str] - Specify a list of devices to boot from in the order
they appear in the list (defaults to
[]
). - cdrom
vm.
Virtual Machine Cdrom Args - The CDROM configuration.
- clone
vm.
Virtual Machine Clone Args - The cloning configuration.
- cpu
vm.
Virtual Machine Cpu Args - The CPU configuration.
- description str
- The description.
- disks
Sequence[vm.
Virtual Machine Disk Args] - A disk (multiple blocks supported).
- efi_
disk vm.Virtual Machine Efi Disk Args - The efi disk device (required if
bios
is set toovmf
) - hook_
script_ strfile_ id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - hostpcis
Sequence[vm.
Virtual Machine Hostpci Args] - A host PCI device mapping (multiple blocks supported).
- initialization
vm.
Virtual Machine Initialization Args - The cloud-init configuration.
- keyboard_
layout str - The keyboard layout (defaults to
en-us
). - kvm_
arguments str - Arbitrary arguments passed to kvm.
- mac_
addresses Sequence[str] - The MAC addresses published by the QEMU agent with fallback to the network device configuration, if the agent is disabled
- machine str
- The VM machine type (defaults to
pc
). - memory
vm.
Virtual Machine Memory Args - The memory configuration.
- migrate bool
- Migrate the VM on node change instead of re-creating
it (defaults to
false
). - name str
- The virtual machine name.
- network_
devices Sequence[vm.Virtual Machine Network Device Args] - A network device (multiple blocks supported).
- numas
Sequence[vm.
Virtual Machine Numa Args] - The NUMA configuration.
- on_
boot bool - Specifies whether a VM will be started during system
boot. (defaults to
true
) - operating_
system vm.Virtual Machine Operating System Args - The Operating System configuration.
- pool_
id str - The identifier for a pool to assign the virtual machine to.
- protection bool
- Sets the protection flag of the VM. This will disable the remove VM and remove disk operations (defaults to
false
). - reboot bool
- Reboot the VM after initial creation. (defaults to
false
) - scsi_
hardware str - The SCSI hardware type (defaults to
virtio-scsi-pci
). - serial_
devices Sequence[vm.Virtual Machine Serial Device Args] - A serial device (multiple blocks supported).
- smbios
vm.
Virtual Machine Smbios Args - The SMBIOS (type1) settings for the VM.
- started bool
- Whether to start the virtual machine (defaults
to
true
). - startup
vm.
Virtual Machine Startup Args - Defines startup and shutdown behavior of the VM.
- stop_
on_ booldestroy - Whether to stop rather than shutdown on VM destroy (defaults to
false
) - tablet_
device bool - Whether to enable the USB tablet device (defaults
to
true
). - Sequence[str]
- A list of tags of the VM. This is only meta information (
defaults to
[]
). Note: Proxmox always sorts the VM tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template bool
- Whether to create a template (defaults to
false
). - timeout_
clone int - Timeout for cloning a VM in seconds (defaults to 1800).
- timeout_
create int - Timeout for creating a VM in seconds (defaults to 1800).
- timeout_
migrate int - Timeout for migrating the VM (defaults to 1800).
- timeout_
move_ intdisk - MoveDisk timeout
- timeout_
reboot int - Timeout for rebooting a VM in seconds (defaults to 1800).
- timeout_
shutdown_ intvm - Timeout for shutting down a VM in seconds ( defaults to 1800).
- timeout_
start_ intvm - Timeout for starting a VM in seconds (defaults to 1800).
- timeout_
stop_ intvm - Timeout for stopping a VM in seconds (defaults to 300).
- tpm_
state vm.Virtual Machine Tpm State Args - The TPM state device.
- usbs
Sequence[vm.
Virtual Machine Usb Args] - A host USB device mapping (multiple blocks supported).
- vga
vm.
Virtual Machine Vga Args - The VGA configuration.
- vm_
id int - The VM identifier.
- node
Name String - The name of the node to assign the virtual machine to.
- acpi Boolean
- Whether to enable ACPI (defaults to
true
). - agent Property Map
- The QEMU agent configuration.
- audio
Device Property Map - An audio device.
- bios String
- The BIOS implementation (defaults to
seabios
). - boot
Orders List<String> - Specify a list of devices to boot from in the order
they appear in the list (defaults to
[]
). - cdrom Property Map
- The CDROM configuration.
- clone Property Map
- The cloning configuration.
- cpu Property Map
- The CPU configuration.
- description String
- The description.
- disks List<Property Map>
- A disk (multiple blocks supported).
- efi
Disk Property Map - The efi disk device (required if
bios
is set toovmf
) - hook
Script StringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - hostpcis List<Property Map>
- A host PCI device mapping (multiple blocks supported).
- initialization Property Map
- The cloud-init configuration.
- keyboard
Layout String - The keyboard layout (defaults to
en-us
). - kvm
Arguments String - Arbitrary arguments passed to kvm.
- mac
Addresses List<String> - The MAC addresses published by the QEMU agent with fallback to the network device configuration, if the agent is disabled
- machine String
- The VM machine type (defaults to
pc
). - memory Property Map
- The memory configuration.
- migrate Boolean
- Migrate the VM on node change instead of re-creating
it (defaults to
false
). - name String
- The virtual machine name.
- network
Devices List<Property Map> - A network device (multiple blocks supported).
- numas List<Property Map>
- The NUMA configuration.
- on
Boot Boolean - Specifies whether a VM will be started during system
boot. (defaults to
true
) - operating
System Property Map - The Operating System configuration.
- pool
Id String - The identifier for a pool to assign the virtual machine to.
- protection Boolean
- Sets the protection flag of the VM. This will disable the remove VM and remove disk operations (defaults to
false
). - reboot Boolean
- Reboot the VM after initial creation. (defaults to
false
) - scsi
Hardware String - The SCSI hardware type (defaults to
virtio-scsi-pci
). - serial
Devices List<Property Map> - A serial device (multiple blocks supported).
- smbios Property Map
- The SMBIOS (type1) settings for the VM.
- started Boolean
- Whether to start the virtual machine (defaults
to
true
). - startup Property Map
- Defines startup and shutdown behavior of the VM.
- stop
On BooleanDestroy - Whether to stop rather than shutdown on VM destroy (defaults to
false
) - tablet
Device Boolean - Whether to enable the USB tablet device (defaults
to
true
). - List<String>
- A list of tags of the VM. This is only meta information (
defaults to
[]
). Note: Proxmox always sorts the VM tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template Boolean
- Whether to create a template (defaults to
false
). - timeout
Clone Number - Timeout for cloning a VM in seconds (defaults to 1800).
- timeout
Create Number - Timeout for creating a VM in seconds (defaults to 1800).
- timeout
Migrate Number - Timeout for migrating the VM (defaults to 1800).
- timeout
Move NumberDisk - MoveDisk timeout
- timeout
Reboot Number - Timeout for rebooting a VM in seconds (defaults to 1800).
- timeout
Shutdown NumberVm - Timeout for shutting down a VM in seconds ( defaults to 1800).
- timeout
Start NumberVm - Timeout for starting a VM in seconds (defaults to 1800).
- timeout
Stop NumberVm - Timeout for stopping a VM in seconds (defaults to 300).
- tpm
State Property Map - The TPM state device.
- usbs List<Property Map>
- A host USB device mapping (multiple blocks supported).
- vga Property Map
- The VGA configuration.
- vm
Id Number - The VM identifier.
Outputs
All input properties are implicitly available as output properties. Additionally, the VirtualMachine resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Ipv4Addresses
List<Immutable
Array<string>> - The IPv4 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - Ipv6Addresses
List<Immutable
Array<string>> - The IPv6 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - Network
Interface List<string>Names - The network interface names published by the QEMU
agent (empty list when
agent.enabled
isfalse
)
- Id string
- The provider-assigned unique ID for this managed resource.
- Ipv4Addresses [][]string
- The IPv4 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - Ipv6Addresses [][]string
- The IPv6 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - Network
Interface []stringNames - The network interface names published by the QEMU
agent (empty list when
agent.enabled
isfalse
)
- id String
- The provider-assigned unique ID for this managed resource.
- ipv4Addresses List<List<String>>
- The IPv4 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - ipv6Addresses List<List<String>>
- The IPv6 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - network
Interface List<String>Names - The network interface names published by the QEMU
agent (empty list when
agent.enabled
isfalse
)
- id string
- The provider-assigned unique ID for this managed resource.
- ipv4Addresses string[][]
- The IPv4 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - ipv6Addresses string[][]
- The IPv6 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - network
Interface string[]Names - The network interface names published by the QEMU
agent (empty list when
agent.enabled
isfalse
)
- id str
- The provider-assigned unique ID for this managed resource.
- ipv4_
addresses Sequence[Sequence[str]] - The IPv4 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - ipv6_
addresses Sequence[Sequence[str]] - The IPv6 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - network_
interface_ Sequence[str]names - The network interface names published by the QEMU
agent (empty list when
agent.enabled
isfalse
)
- id String
- The provider-assigned unique ID for this managed resource.
- ipv4Addresses List<List<String>>
- The IPv4 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - ipv6Addresses List<List<String>>
- The IPv6 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - network
Interface List<String>Names - The network interface names published by the QEMU
agent (empty list when
agent.enabled
isfalse
)
Look up Existing VirtualMachine Resource
Get an existing VirtualMachine resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: VirtualMachineState, opts?: CustomResourceOptions): VirtualMachine
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
acpi: Optional[bool] = None,
agent: Optional[_vm.VirtualMachineAgentArgs] = None,
audio_device: Optional[_vm.VirtualMachineAudioDeviceArgs] = None,
bios: Optional[str] = None,
boot_orders: Optional[Sequence[str]] = None,
cdrom: Optional[_vm.VirtualMachineCdromArgs] = None,
clone: Optional[_vm.VirtualMachineCloneArgs] = None,
cpu: Optional[_vm.VirtualMachineCpuArgs] = None,
description: Optional[str] = None,
disks: Optional[Sequence[_vm.VirtualMachineDiskArgs]] = None,
efi_disk: Optional[_vm.VirtualMachineEfiDiskArgs] = None,
hook_script_file_id: Optional[str] = None,
hostpcis: Optional[Sequence[_vm.VirtualMachineHostpciArgs]] = None,
initialization: Optional[_vm.VirtualMachineInitializationArgs] = None,
ipv4_addresses: Optional[Sequence[Sequence[str]]] = None,
ipv6_addresses: Optional[Sequence[Sequence[str]]] = None,
keyboard_layout: Optional[str] = None,
kvm_arguments: Optional[str] = None,
mac_addresses: Optional[Sequence[str]] = None,
machine: Optional[str] = None,
memory: Optional[_vm.VirtualMachineMemoryArgs] = None,
migrate: Optional[bool] = None,
name: Optional[str] = None,
network_devices: Optional[Sequence[_vm.VirtualMachineNetworkDeviceArgs]] = None,
network_interface_names: Optional[Sequence[str]] = None,
node_name: Optional[str] = None,
numas: Optional[Sequence[_vm.VirtualMachineNumaArgs]] = None,
on_boot: Optional[bool] = None,
operating_system: Optional[_vm.VirtualMachineOperatingSystemArgs] = None,
pool_id: Optional[str] = None,
protection: Optional[bool] = None,
reboot: Optional[bool] = None,
scsi_hardware: Optional[str] = None,
serial_devices: Optional[Sequence[_vm.VirtualMachineSerialDeviceArgs]] = None,
smbios: Optional[_vm.VirtualMachineSmbiosArgs] = None,
started: Optional[bool] = None,
startup: Optional[_vm.VirtualMachineStartupArgs] = None,
stop_on_destroy: Optional[bool] = None,
tablet_device: Optional[bool] = None,
tags: Optional[Sequence[str]] = None,
template: Optional[bool] = None,
timeout_clone: Optional[int] = None,
timeout_create: Optional[int] = None,
timeout_migrate: Optional[int] = None,
timeout_move_disk: Optional[int] = None,
timeout_reboot: Optional[int] = None,
timeout_shutdown_vm: Optional[int] = None,
timeout_start_vm: Optional[int] = None,
timeout_stop_vm: Optional[int] = None,
tpm_state: Optional[_vm.VirtualMachineTpmStateArgs] = None,
usbs: Optional[Sequence[_vm.VirtualMachineUsbArgs]] = None,
vga: Optional[_vm.VirtualMachineVgaArgs] = None,
vm_id: Optional[int] = None) -> VirtualMachine
func GetVirtualMachine(ctx *Context, name string, id IDInput, state *VirtualMachineState, opts ...ResourceOption) (*VirtualMachine, error)
public static VirtualMachine Get(string name, Input<string> id, VirtualMachineState? state, CustomResourceOptions? opts = null)
public static VirtualMachine get(String name, Output<String> id, VirtualMachineState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Acpi bool
- Whether to enable ACPI (defaults to
true
). - Agent
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Agent - The QEMU agent configuration.
- Audio
Device Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Audio Device - An audio device.
- Bios string
- The BIOS implementation (defaults to
seabios
). - Boot
Orders List<string> - Specify a list of devices to boot from in the order
they appear in the list (defaults to
[]
). - Cdrom
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Cdrom - The CDROM configuration.
- Clone
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Clone - The cloning configuration.
- Cpu
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Cpu - The CPU configuration.
- Description string
- The description.
- Disks
List<Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Disk> - A disk (multiple blocks supported).
- Efi
Disk Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Efi Disk - The efi disk device (required if
bios
is set toovmf
) - Hook
Script stringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - Hostpcis
List<Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Hostpci> - A host PCI device mapping (multiple blocks supported).
- Initialization
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Initialization - The cloud-init configuration.
- Ipv4Addresses
List<Immutable
Array<string>> - The IPv4 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - Ipv6Addresses
List<Immutable
Array<string>> - The IPv6 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - Keyboard
Layout string - The keyboard layout (defaults to
en-us
). - Kvm
Arguments string - Arbitrary arguments passed to kvm.
- Mac
Addresses List<string> - The MAC addresses published by the QEMU agent with fallback to the network device configuration, if the agent is disabled
- Machine string
- The VM machine type (defaults to
pc
). - Memory
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Memory - The memory configuration.
- Migrate bool
- Migrate the VM on node change instead of re-creating
it (defaults to
false
). - Name string
- The virtual machine name.
- Network
Devices List<Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Network Device> - A network device (multiple blocks supported).
- Network
Interface List<string>Names - The network interface names published by the QEMU
agent (empty list when
agent.enabled
isfalse
) - Node
Name string - The name of the node to assign the virtual machine to.
- Numas
List<Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Numa> - The NUMA configuration.
- On
Boot bool - Specifies whether a VM will be started during system
boot. (defaults to
true
) - Operating
System Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Operating System - The Operating System configuration.
- Pool
Id string - The identifier for a pool to assign the virtual machine to.
- Protection bool
- Sets the protection flag of the VM. This will disable the remove VM and remove disk operations (defaults to
false
). - Reboot bool
- Reboot the VM after initial creation. (defaults to
false
) - Scsi
Hardware string - The SCSI hardware type (defaults to
virtio-scsi-pci
). - Serial
Devices List<Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Serial Device> - A serial device (multiple blocks supported).
- Smbios
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Smbios - The SMBIOS (type1) settings for the VM.
- Started bool
- Whether to start the virtual machine (defaults
to
true
). - Startup
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Startup - Defines startup and shutdown behavior of the VM.
- Stop
On boolDestroy - Whether to stop rather than shutdown on VM destroy (defaults to
false
) - Tablet
Device bool - Whether to enable the USB tablet device (defaults
to
true
). - List<string>
- A list of tags of the VM. This is only meta information (
defaults to
[]
). Note: Proxmox always sorts the VM tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - Template bool
- Whether to create a template (defaults to
false
). - Timeout
Clone int - Timeout for cloning a VM in seconds (defaults to 1800).
- Timeout
Create int - Timeout for creating a VM in seconds (defaults to 1800).
- Timeout
Migrate int - Timeout for migrating the VM (defaults to 1800).
- Timeout
Move intDisk - MoveDisk timeout
- Timeout
Reboot int - Timeout for rebooting a VM in seconds (defaults to 1800).
- Timeout
Shutdown intVm - Timeout for shutting down a VM in seconds ( defaults to 1800).
- Timeout
Start intVm - Timeout for starting a VM in seconds (defaults to 1800).
- Timeout
Stop intVm - Timeout for stopping a VM in seconds (defaults to 300).
- Tpm
State Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Tpm State - The TPM state device.
- Usbs
List<Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Usb> - A host USB device mapping (multiple blocks supported).
- Vga
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Vga - The VGA configuration.
- Vm
Id int - The VM identifier.
- Acpi bool
- Whether to enable ACPI (defaults to
true
). - Agent
Virtual
Machine Agent Args - The QEMU agent configuration.
- Audio
Device VirtualMachine Audio Device Args - An audio device.
- Bios string
- The BIOS implementation (defaults to
seabios
). - Boot
Orders []string - Specify a list of devices to boot from in the order
they appear in the list (defaults to
[]
). - Cdrom
Virtual
Machine Cdrom Args - The CDROM configuration.
- Clone
Virtual
Machine Clone Args - The cloning configuration.
- Cpu
Virtual
Machine Cpu Args - The CPU configuration.
- Description string
- The description.
- Disks
[]Virtual
Machine Disk Args - A disk (multiple blocks supported).
- Efi
Disk VirtualMachine Efi Disk Args - The efi disk device (required if
bios
is set toovmf
) - Hook
Script stringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - Hostpcis
[]Virtual
Machine Hostpci Args - A host PCI device mapping (multiple blocks supported).
- Initialization
Virtual
Machine Initialization Args - The cloud-init configuration.
- Ipv4Addresses [][]string
- The IPv4 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - Ipv6Addresses [][]string
- The IPv6 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - Keyboard
Layout string - The keyboard layout (defaults to
en-us
). - Kvm
Arguments string - Arbitrary arguments passed to kvm.
- Mac
Addresses []string - The MAC addresses published by the QEMU agent with fallback to the network device configuration, if the agent is disabled
- Machine string
- The VM machine type (defaults to
pc
). - Memory
Virtual
Machine Memory Args - The memory configuration.
- Migrate bool
- Migrate the VM on node change instead of re-creating
it (defaults to
false
). - Name string
- The virtual machine name.
- Network
Devices []VirtualMachine Network Device Args - A network device (multiple blocks supported).
- Network
Interface []stringNames - The network interface names published by the QEMU
agent (empty list when
agent.enabled
isfalse
) - Node
Name string - The name of the node to assign the virtual machine to.
- Numas
[]Virtual
Machine Numa Args - The NUMA configuration.
- On
Boot bool - Specifies whether a VM will be started during system
boot. (defaults to
true
) - Operating
System VirtualMachine Operating System Args - The Operating System configuration.
- Pool
Id string - The identifier for a pool to assign the virtual machine to.
- Protection bool
- Sets the protection flag of the VM. This will disable the remove VM and remove disk operations (defaults to
false
). - Reboot bool
- Reboot the VM after initial creation. (defaults to
false
) - Scsi
Hardware string - The SCSI hardware type (defaults to
virtio-scsi-pci
). - Serial
Devices []VirtualMachine Serial Device Args - A serial device (multiple blocks supported).
- Smbios
Virtual
Machine Smbios Args - The SMBIOS (type1) settings for the VM.
- Started bool
- Whether to start the virtual machine (defaults
to
true
). - Startup
Virtual
Machine Startup Args - Defines startup and shutdown behavior of the VM.
- Stop
On boolDestroy - Whether to stop rather than shutdown on VM destroy (defaults to
false
) - Tablet
Device bool - Whether to enable the USB tablet device (defaults
to
true
). - []string
- A list of tags of the VM. This is only meta information (
defaults to
[]
). Note: Proxmox always sorts the VM tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - Template bool
- Whether to create a template (defaults to
false
). - Timeout
Clone int - Timeout for cloning a VM in seconds (defaults to 1800).
- Timeout
Create int - Timeout for creating a VM in seconds (defaults to 1800).
- Timeout
Migrate int - Timeout for migrating the VM (defaults to 1800).
- Timeout
Move intDisk - MoveDisk timeout
- Timeout
Reboot int - Timeout for rebooting a VM in seconds (defaults to 1800).
- Timeout
Shutdown intVm - Timeout for shutting down a VM in seconds ( defaults to 1800).
- Timeout
Start intVm - Timeout for starting a VM in seconds (defaults to 1800).
- Timeout
Stop intVm - Timeout for stopping a VM in seconds (defaults to 300).
- Tpm
State VirtualMachine Tpm State Args - The TPM state device.
- Usbs
[]Virtual
Machine Usb Args - A host USB device mapping (multiple blocks supported).
- Vga
Virtual
Machine Vga Args - The VGA configuration.
- Vm
Id int - The VM identifier.
- acpi Boolean
- Whether to enable ACPI (defaults to
true
). - agent
Virtual
Machine Agent - The QEMU agent configuration.
- audio
Device VirtualMachine Audio Device - An audio device.
- bios String
- The BIOS implementation (defaults to
seabios
). - boot
Orders List<String> - Specify a list of devices to boot from in the order
they appear in the list (defaults to
[]
). - cdrom
Virtual
Machine Cdrom - The CDROM configuration.
- clone_
Virtual
Machine Clone - The cloning configuration.
- cpu
Virtual
Machine Cpu - The CPU configuration.
- description String
- The description.
- disks
List<Virtual
Machine Disk> - A disk (multiple blocks supported).
- efi
Disk VirtualMachine Efi Disk - The efi disk device (required if
bios
is set toovmf
) - hook
Script StringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - hostpcis
List<Virtual
Machine Hostpci> - A host PCI device mapping (multiple blocks supported).
- initialization
Virtual
Machine Initialization - The cloud-init configuration.
- ipv4Addresses List<List<String>>
- The IPv4 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - ipv6Addresses List<List<String>>
- The IPv6 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - keyboard
Layout String - The keyboard layout (defaults to
en-us
). - kvm
Arguments String - Arbitrary arguments passed to kvm.
- mac
Addresses List<String> - The MAC addresses published by the QEMU agent with fallback to the network device configuration, if the agent is disabled
- machine String
- The VM machine type (defaults to
pc
). - memory
Virtual
Machine Memory - The memory configuration.
- migrate Boolean
- Migrate the VM on node change instead of re-creating
it (defaults to
false
). - name String
- The virtual machine name.
- network
Devices List<VirtualMachine Network Device> - A network device (multiple blocks supported).
- network
Interface List<String>Names - The network interface names published by the QEMU
agent (empty list when
agent.enabled
isfalse
) - node
Name String - The name of the node to assign the virtual machine to.
- numas
List<Virtual
Machine Numa> - The NUMA configuration.
- on
Boot Boolean - Specifies whether a VM will be started during system
boot. (defaults to
true
) - operating
System VirtualMachine Operating System - The Operating System configuration.
- pool
Id String - The identifier for a pool to assign the virtual machine to.
- protection Boolean
- Sets the protection flag of the VM. This will disable the remove VM and remove disk operations (defaults to
false
). - reboot Boolean
- Reboot the VM after initial creation. (defaults to
false
) - scsi
Hardware String - The SCSI hardware type (defaults to
virtio-scsi-pci
). - serial
Devices List<VirtualMachine Serial Device> - A serial device (multiple blocks supported).
- smbios
Virtual
Machine Smbios - The SMBIOS (type1) settings for the VM.
- started Boolean
- Whether to start the virtual machine (defaults
to
true
). - startup
Virtual
Machine Startup - Defines startup and shutdown behavior of the VM.
- stop
On BooleanDestroy - Whether to stop rather than shutdown on VM destroy (defaults to
false
) - tablet
Device Boolean - Whether to enable the USB tablet device (defaults
to
true
). - List<String>
- A list of tags of the VM. This is only meta information (
defaults to
[]
). Note: Proxmox always sorts the VM tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template Boolean
- Whether to create a template (defaults to
false
). - timeout
Clone Integer - Timeout for cloning a VM in seconds (defaults to 1800).
- timeout
Create Integer - Timeout for creating a VM in seconds (defaults to 1800).
- timeout
Migrate Integer - Timeout for migrating the VM (defaults to 1800).
- timeout
Move IntegerDisk - MoveDisk timeout
- timeout
Reboot Integer - Timeout for rebooting a VM in seconds (defaults to 1800).
- timeout
Shutdown IntegerVm - Timeout for shutting down a VM in seconds ( defaults to 1800).
- timeout
Start IntegerVm - Timeout for starting a VM in seconds (defaults to 1800).
- timeout
Stop IntegerVm - Timeout for stopping a VM in seconds (defaults to 300).
- tpm
State VirtualMachine Tpm State - The TPM state device.
- usbs
List<Virtual
Machine Usb> - A host USB device mapping (multiple blocks supported).
- vga
Virtual
Machine Vga - The VGA configuration.
- vm
Id Integer - The VM identifier.
- acpi boolean
- Whether to enable ACPI (defaults to
true
). - agent
Virtual
Machine Agent - The QEMU agent configuration.
- audio
Device VirtualMachine Audio Device - An audio device.
- bios string
- The BIOS implementation (defaults to
seabios
). - boot
Orders string[] - Specify a list of devices to boot from in the order
they appear in the list (defaults to
[]
). - cdrom
Virtual
Machine Cdrom - The CDROM configuration.
- clone
Virtual
Machine Clone - The cloning configuration.
- cpu
Virtual
Machine Cpu - The CPU configuration.
- description string
- The description.
- disks
Virtual
Machine Disk[] - A disk (multiple blocks supported).
- efi
Disk VirtualMachine Efi Disk - The efi disk device (required if
bios
is set toovmf
) - hook
Script stringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - hostpcis
Virtual
Machine Hostpci[] - A host PCI device mapping (multiple blocks supported).
- initialization
Virtual
Machine Initialization - The cloud-init configuration.
- ipv4Addresses string[][]
- The IPv4 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - ipv6Addresses string[][]
- The IPv6 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - keyboard
Layout string - The keyboard layout (defaults to
en-us
). - kvm
Arguments string - Arbitrary arguments passed to kvm.
- mac
Addresses string[] - The MAC addresses published by the QEMU agent with fallback to the network device configuration, if the agent is disabled
- machine string
- The VM machine type (defaults to
pc
). - memory
Virtual
Machine Memory - The memory configuration.
- migrate boolean
- Migrate the VM on node change instead of re-creating
it (defaults to
false
). - name string
- The virtual machine name.
- network
Devices VirtualMachine Network Device[] - A network device (multiple blocks supported).
- network
Interface string[]Names - The network interface names published by the QEMU
agent (empty list when
agent.enabled
isfalse
) - node
Name string - The name of the node to assign the virtual machine to.
- numas
Virtual
Machine Numa[] - The NUMA configuration.
- on
Boot boolean - Specifies whether a VM will be started during system
boot. (defaults to
true
) - operating
System VirtualMachine Operating System - The Operating System configuration.
- pool
Id string - The identifier for a pool to assign the virtual machine to.
- protection boolean
- Sets the protection flag of the VM. This will disable the remove VM and remove disk operations (defaults to
false
). - reboot boolean
- Reboot the VM after initial creation. (defaults to
false
) - scsi
Hardware string - The SCSI hardware type (defaults to
virtio-scsi-pci
). - serial
Devices VirtualMachine Serial Device[] - A serial device (multiple blocks supported).
- smbios
Virtual
Machine Smbios - The SMBIOS (type1) settings for the VM.
- started boolean
- Whether to start the virtual machine (defaults
to
true
). - startup
Virtual
Machine Startup - Defines startup and shutdown behavior of the VM.
- stop
On booleanDestroy - Whether to stop rather than shutdown on VM destroy (defaults to
false
) - tablet
Device boolean - Whether to enable the USB tablet device (defaults
to
true
). - string[]
- A list of tags of the VM. This is only meta information (
defaults to
[]
). Note: Proxmox always sorts the VM tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template boolean
- Whether to create a template (defaults to
false
). - timeout
Clone number - Timeout for cloning a VM in seconds (defaults to 1800).
- timeout
Create number - Timeout for creating a VM in seconds (defaults to 1800).
- timeout
Migrate number - Timeout for migrating the VM (defaults to 1800).
- timeout
Move numberDisk - MoveDisk timeout
- timeout
Reboot number - Timeout for rebooting a VM in seconds (defaults to 1800).
- timeout
Shutdown numberVm - Timeout for shutting down a VM in seconds ( defaults to 1800).
- timeout
Start numberVm - Timeout for starting a VM in seconds (defaults to 1800).
- timeout
Stop numberVm - Timeout for stopping a VM in seconds (defaults to 300).
- tpm
State VirtualMachine Tpm State - The TPM state device.
- usbs
Virtual
Machine Usb[] - A host USB device mapping (multiple blocks supported).
- vga
Virtual
Machine Vga - The VGA configuration.
- vm
Id number - The VM identifier.
- acpi bool
- Whether to enable ACPI (defaults to
true
). - agent
vm.
Virtual Machine Agent Args - The QEMU agent configuration.
- audio_
device vm.Virtual Machine Audio Device Args - An audio device.
- bios str
- The BIOS implementation (defaults to
seabios
). - boot_
orders Sequence[str] - Specify a list of devices to boot from in the order
they appear in the list (defaults to
[]
). - cdrom
vm.
Virtual Machine Cdrom Args - The CDROM configuration.
- clone
vm.
Virtual Machine Clone Args - The cloning configuration.
- cpu
vm.
Virtual Machine Cpu Args - The CPU configuration.
- description str
- The description.
- disks
Sequence[vm.
Virtual Machine Disk Args] - A disk (multiple blocks supported).
- efi_
disk vm.Virtual Machine Efi Disk Args - The efi disk device (required if
bios
is set toovmf
) - hook_
script_ strfile_ id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - hostpcis
Sequence[vm.
Virtual Machine Hostpci Args] - A host PCI device mapping (multiple blocks supported).
- initialization
vm.
Virtual Machine Initialization Args - The cloud-init configuration.
- ipv4_
addresses Sequence[Sequence[str]] - The IPv4 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - ipv6_
addresses Sequence[Sequence[str]] - The IPv6 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - keyboard_
layout str - The keyboard layout (defaults to
en-us
). - kvm_
arguments str - Arbitrary arguments passed to kvm.
- mac_
addresses Sequence[str] - The MAC addresses published by the QEMU agent with fallback to the network device configuration, if the agent is disabled
- machine str
- The VM machine type (defaults to
pc
). - memory
vm.
Virtual Machine Memory Args - The memory configuration.
- migrate bool
- Migrate the VM on node change instead of re-creating
it (defaults to
false
). - name str
- The virtual machine name.
- network_
devices Sequence[vm.Virtual Machine Network Device Args] - A network device (multiple blocks supported).
- network_
interface_ Sequence[str]names - The network interface names published by the QEMU
agent (empty list when
agent.enabled
isfalse
) - node_
name str - The name of the node to assign the virtual machine to.
- numas
Sequence[vm.
Virtual Machine Numa Args] - The NUMA configuration.
- on_
boot bool - Specifies whether a VM will be started during system
boot. (defaults to
true
) - operating_
system vm.Virtual Machine Operating System Args - The Operating System configuration.
- pool_
id str - The identifier for a pool to assign the virtual machine to.
- protection bool
- Sets the protection flag of the VM. This will disable the remove VM and remove disk operations (defaults to
false
). - reboot bool
- Reboot the VM after initial creation. (defaults to
false
) - scsi_
hardware str - The SCSI hardware type (defaults to
virtio-scsi-pci
). - serial_
devices Sequence[vm.Virtual Machine Serial Device Args] - A serial device (multiple blocks supported).
- smbios
vm.
Virtual Machine Smbios Args - The SMBIOS (type1) settings for the VM.
- started bool
- Whether to start the virtual machine (defaults
to
true
). - startup
vm.
Virtual Machine Startup Args - Defines startup and shutdown behavior of the VM.
- stop_
on_ booldestroy - Whether to stop rather than shutdown on VM destroy (defaults to
false
) - tablet_
device bool - Whether to enable the USB tablet device (defaults
to
true
). - Sequence[str]
- A list of tags of the VM. This is only meta information (
defaults to
[]
). Note: Proxmox always sorts the VM tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template bool
- Whether to create a template (defaults to
false
). - timeout_
clone int - Timeout for cloning a VM in seconds (defaults to 1800).
- timeout_
create int - Timeout for creating a VM in seconds (defaults to 1800).
- timeout_
migrate int - Timeout for migrating the VM (defaults to 1800).
- timeout_
move_ intdisk - MoveDisk timeout
- timeout_
reboot int - Timeout for rebooting a VM in seconds (defaults to 1800).
- timeout_
shutdown_ intvm - Timeout for shutting down a VM in seconds ( defaults to 1800).
- timeout_
start_ intvm - Timeout for starting a VM in seconds (defaults to 1800).
- timeout_
stop_ intvm - Timeout for stopping a VM in seconds (defaults to 300).
- tpm_
state vm.Virtual Machine Tpm State Args - The TPM state device.
- usbs
Sequence[vm.
Virtual Machine Usb Args] - A host USB device mapping (multiple blocks supported).
- vga
vm.
Virtual Machine Vga Args - The VGA configuration.
- vm_
id int - The VM identifier.
- acpi Boolean
- Whether to enable ACPI (defaults to
true
). - agent Property Map
- The QEMU agent configuration.
- audio
Device Property Map - An audio device.
- bios String
- The BIOS implementation (defaults to
seabios
). - boot
Orders List<String> - Specify a list of devices to boot from in the order
they appear in the list (defaults to
[]
). - cdrom Property Map
- The CDROM configuration.
- clone Property Map
- The cloning configuration.
- cpu Property Map
- The CPU configuration.
- description String
- The description.
- disks List<Property Map>
- A disk (multiple blocks supported).
- efi
Disk Property Map - The efi disk device (required if
bios
is set toovmf
) - hook
Script StringFile Id - The identifier for a file containing a hook script (needs to be executable, e.g. by using the
proxmox_virtual_environment_file.file_mode
attribute). - hostpcis List<Property Map>
- A host PCI device mapping (multiple blocks supported).
- initialization Property Map
- The cloud-init configuration.
- ipv4Addresses List<List<String>>
- The IPv4 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - ipv6Addresses List<List<String>>
- The IPv6 addresses per network interface published by the
QEMU agent (empty list when
agent.enabled
isfalse
) - keyboard
Layout String - The keyboard layout (defaults to
en-us
). - kvm
Arguments String - Arbitrary arguments passed to kvm.
- mac
Addresses List<String> - The MAC addresses published by the QEMU agent with fallback to the network device configuration, if the agent is disabled
- machine String
- The VM machine type (defaults to
pc
). - memory Property Map
- The memory configuration.
- migrate Boolean
- Migrate the VM on node change instead of re-creating
it (defaults to
false
). - name String
- The virtual machine name.
- network
Devices List<Property Map> - A network device (multiple blocks supported).
- network
Interface List<String>Names - The network interface names published by the QEMU
agent (empty list when
agent.enabled
isfalse
) - node
Name String - The name of the node to assign the virtual machine to.
- numas List<Property Map>
- The NUMA configuration.
- on
Boot Boolean - Specifies whether a VM will be started during system
boot. (defaults to
true
) - operating
System Property Map - The Operating System configuration.
- pool
Id String - The identifier for a pool to assign the virtual machine to.
- protection Boolean
- Sets the protection flag of the VM. This will disable the remove VM and remove disk operations (defaults to
false
). - reboot Boolean
- Reboot the VM after initial creation. (defaults to
false
) - scsi
Hardware String - The SCSI hardware type (defaults to
virtio-scsi-pci
). - serial
Devices List<Property Map> - A serial device (multiple blocks supported).
- smbios Property Map
- The SMBIOS (type1) settings for the VM.
- started Boolean
- Whether to start the virtual machine (defaults
to
true
). - startup Property Map
- Defines startup and shutdown behavior of the VM.
- stop
On BooleanDestroy - Whether to stop rather than shutdown on VM destroy (defaults to
false
) - tablet
Device Boolean - Whether to enable the USB tablet device (defaults
to
true
). - List<String>
- A list of tags of the VM. This is only meta information (
defaults to
[]
). Note: Proxmox always sorts the VM tags. If the list in template is not sorted, then Proxmox will always report a difference on the resource. You may use theignore_changes
lifecycle meta-argument to ignore changes to this attribute. - template Boolean
- Whether to create a template (defaults to
false
). - timeout
Clone Number - Timeout for cloning a VM in seconds (defaults to 1800).
- timeout
Create Number - Timeout for creating a VM in seconds (defaults to 1800).
- timeout
Migrate Number - Timeout for migrating the VM (defaults to 1800).
- timeout
Move NumberDisk - MoveDisk timeout
- timeout
Reboot Number - Timeout for rebooting a VM in seconds (defaults to 1800).
- timeout
Shutdown NumberVm - Timeout for shutting down a VM in seconds ( defaults to 1800).
- timeout
Start NumberVm - Timeout for starting a VM in seconds (defaults to 1800).
- timeout
Stop NumberVm - Timeout for stopping a VM in seconds (defaults to 300).
- tpm
State Property Map - The TPM state device.
- usbs List<Property Map>
- A host USB device mapping (multiple blocks supported).
- vga Property Map
- The VGA configuration.
- vm
Id Number - The VM identifier.
Supporting Types
VirtualMachineAgent, VirtualMachineAgentArgs
- Enabled bool
- Whether to enable the QEMU agent (defaults
to
false
). - Timeout string
- The maximum amount of time to wait for data from
the QEMU agent to become available ( defaults to
15m
). - Trim bool
- Whether to enable the FSTRIM feature in the QEMU agent
(defaults to
false
). - Type string
- The QEMU agent interface type (defaults to
virtio
).
- Enabled bool
- Whether to enable the QEMU agent (defaults
to
false
). - Timeout string
- The maximum amount of time to wait for data from
the QEMU agent to become available ( defaults to
15m
). - Trim bool
- Whether to enable the FSTRIM feature in the QEMU agent
(defaults to
false
). - Type string
- The QEMU agent interface type (defaults to
virtio
).
- enabled Boolean
- Whether to enable the QEMU agent (defaults
to
false
). - timeout String
- The maximum amount of time to wait for data from
the QEMU agent to become available ( defaults to
15m
). - trim Boolean
- Whether to enable the FSTRIM feature in the QEMU agent
(defaults to
false
). - type String
- The QEMU agent interface type (defaults to
virtio
).
- enabled boolean
- Whether to enable the QEMU agent (defaults
to
false
). - timeout string
- The maximum amount of time to wait for data from
the QEMU agent to become available ( defaults to
15m
). - trim boolean
- Whether to enable the FSTRIM feature in the QEMU agent
(defaults to
false
). - type string
- The QEMU agent interface type (defaults to
virtio
).
- enabled bool
- Whether to enable the QEMU agent (defaults
to
false
). - timeout str
- The maximum amount of time to wait for data from
the QEMU agent to become available ( defaults to
15m
). - trim bool
- Whether to enable the FSTRIM feature in the QEMU agent
(defaults to
false
). - type str
- The QEMU agent interface type (defaults to
virtio
).
- enabled Boolean
- Whether to enable the QEMU agent (defaults
to
false
). - timeout String
- The maximum amount of time to wait for data from
the QEMU agent to become available ( defaults to
15m
). - trim Boolean
- Whether to enable the FSTRIM feature in the QEMU agent
(defaults to
false
). - type String
- The QEMU agent interface type (defaults to
virtio
).
VirtualMachineAudioDevice, VirtualMachineAudioDeviceArgs
VirtualMachineCdrom, VirtualMachineCdromArgs
- Enabled bool
- Whether to enable the CDROM drive (defaults
to
false
). - File
Id string - A file ID for an ISO file (defaults to
cdrom
as in the physical drive). Usenone
to leave the CDROM drive empty. - Interface string
- A hardware interface to connect CDROM drive to,
must be
ideN
(defaults toide3
). Note thatq35
machine type only supportside0
andide2
.
- Enabled bool
- Whether to enable the CDROM drive (defaults
to
false
). - File
Id string - A file ID for an ISO file (defaults to
cdrom
as in the physical drive). Usenone
to leave the CDROM drive empty. - Interface string
- A hardware interface to connect CDROM drive to,
must be
ideN
(defaults toide3
). Note thatq35
machine type only supportside0
andide2
.
- enabled Boolean
- Whether to enable the CDROM drive (defaults
to
false
). - file
Id String - A file ID for an ISO file (defaults to
cdrom
as in the physical drive). Usenone
to leave the CDROM drive empty. - interface_ String
- A hardware interface to connect CDROM drive to,
must be
ideN
(defaults toide3
). Note thatq35
machine type only supportside0
andide2
.
- enabled boolean
- Whether to enable the CDROM drive (defaults
to
false
). - file
Id string - A file ID for an ISO file (defaults to
cdrom
as in the physical drive). Usenone
to leave the CDROM drive empty. - interface string
- A hardware interface to connect CDROM drive to,
must be
ideN
(defaults toide3
). Note thatq35
machine type only supportside0
andide2
.
- enabled bool
- Whether to enable the CDROM drive (defaults
to
false
). - file_
id str - A file ID for an ISO file (defaults to
cdrom
as in the physical drive). Usenone
to leave the CDROM drive empty. - interface str
- A hardware interface to connect CDROM drive to,
must be
ideN
(defaults toide3
). Note thatq35
machine type only supportside0
andide2
.
- enabled Boolean
- Whether to enable the CDROM drive (defaults
to
false
). - file
Id String - A file ID for an ISO file (defaults to
cdrom
as in the physical drive). Usenone
to leave the CDROM drive empty. - interface String
- A hardware interface to connect CDROM drive to,
must be
ideN
(defaults toide3
). Note thatq35
machine type only supportside0
andide2
.
VirtualMachineClone, VirtualMachineCloneArgs
- Vm
Id int - The identifier for the source VM.
- Datastore
Id string - The identifier for the target datastore.
- Full bool
- Full or linked clone (defaults to
true
). - Node
Name string - The name of the source node (leave blank, if
equal to the
node_name
argument). - Retries int
- Number of retries in Proxmox for clone vm. Sometimes Proxmox errors with timeout when creating multiple clones at once.
- Vm
Id int - The identifier for the source VM.
- Datastore
Id string - The identifier for the target datastore.
- Full bool
- Full or linked clone (defaults to
true
). - Node
Name string - The name of the source node (leave blank, if
equal to the
node_name
argument). - Retries int
- Number of retries in Proxmox for clone vm. Sometimes Proxmox errors with timeout when creating multiple clones at once.
- vm
Id Integer - The identifier for the source VM.
- datastore
Id String - The identifier for the target datastore.
- full Boolean
- Full or linked clone (defaults to
true
). - node
Name String - The name of the source node (leave blank, if
equal to the
node_name
argument). - retries Integer
- Number of retries in Proxmox for clone vm. Sometimes Proxmox errors with timeout when creating multiple clones at once.
- vm
Id number - The identifier for the source VM.
- datastore
Id string - The identifier for the target datastore.
- full boolean
- Full or linked clone (defaults to
true
). - node
Name string - The name of the source node (leave blank, if
equal to the
node_name
argument). - retries number
- Number of retries in Proxmox for clone vm. Sometimes Proxmox errors with timeout when creating multiple clones at once.
- vm_
id int - The identifier for the source VM.
- datastore_
id str - The identifier for the target datastore.
- full bool
- Full or linked clone (defaults to
true
). - node_
name str - The name of the source node (leave blank, if
equal to the
node_name
argument). - retries int
- Number of retries in Proxmox for clone vm. Sometimes Proxmox errors with timeout when creating multiple clones at once.
- vm
Id Number - The identifier for the source VM.
- datastore
Id String - The identifier for the target datastore.
- full Boolean
- Full or linked clone (defaults to
true
). - node
Name String - The name of the source node (leave blank, if
equal to the
node_name
argument). - retries Number
- Number of retries in Proxmox for clone vm. Sometimes Proxmox errors with timeout when creating multiple clones at once.
VirtualMachineCpu, VirtualMachineCpuArgs
- Affinity string
- The CPU cores that are used to run the VM’s vCPU. The
value is a list of CPU IDs, separated by commas. The CPU IDs are zero-based.
For example,
0,1,2,3
(which also can be shortened to0-3
) means that the VM’s vCPUs are run on the first four CPU cores. Settingaffinity
is only allowed forroot@pam
authenticated user. - Architecture string
- The CPU architecture (defaults to
x86_64
). - Cores int
- The number of CPU cores (defaults to
1
). - Flags List<string>
- The CPU flags.
+aes
/-aes
- Activate AES instruction set for HW acceleration.+amd-no-ssb
/-amd-no-ssb
- Notifies guest OS that host is not vulnerable for Spectre on AMD CPUs.+amd-ssbd
/-amd-ssbd
- Improves Spectre mitigation performance with AMD CPUs, best used with "virt-ssbd".+hv-evmcs
/-hv-evmcs
- Improve performance for nested virtualization (only supported on Intel CPUs).+hv-tlbflush
/-hv-tlbflush
- Improve performance in overcommitted Windows guests (may lead to guest BSOD on old CPUs).+ibpb
/-ibpb
- Allows improved Spectre mitigation on AMD CPUs.+md-clear
/-md-clear
- Required to let the guest OS know if MDS is mitigated correctly.+pcid
/-pcid
- Meltdown fix cost reduction on Westmere, Sandy- and Ivy Bridge Intel CPUs.+pdpe1gb
/-pdpe1gb
- Allows guest OS to use 1 GB size pages, if host HW supports it.+spec-ctrl
/-spec-ctrl
- Allows improved Spectre mitigation with Intel CPUs.+ssbd
/-ssbd
- Protection for "Speculative Store Bypass" for Intel models.+virt-ssbd
/-virt-ssbd
- Basis for "Speculative Store Bypass" protection for AMD models.
- Hotplugged int
- The number of hotplugged vCPUs (defaults
to
0
). - Limit int
- Limit of CPU usage,
0...128
. (defaults to0
-- no limit). - Numa bool
- Enable/disable NUMA. (default to
false
) - Sockets int
- The number of CPU sockets (defaults to
1
). - Type string
- The emulated CPU type, it's recommended to
use
x86-64-v2-AES
(defaults toqemu64
). - Units int
- The CPU units (defaults to
1024
).
- Affinity string
- The CPU cores that are used to run the VM’s vCPU. The
value is a list of CPU IDs, separated by commas. The CPU IDs are zero-based.
For example,
0,1,2,3
(which also can be shortened to0-3
) means that the VM’s vCPUs are run on the first four CPU cores. Settingaffinity
is only allowed forroot@pam
authenticated user. - Architecture string
- The CPU architecture (defaults to
x86_64
). - Cores int
- The number of CPU cores (defaults to
1
). - Flags []string
- The CPU flags.
+aes
/-aes
- Activate AES instruction set for HW acceleration.+amd-no-ssb
/-amd-no-ssb
- Notifies guest OS that host is not vulnerable for Spectre on AMD CPUs.+amd-ssbd
/-amd-ssbd
- Improves Spectre mitigation performance with AMD CPUs, best used with "virt-ssbd".+hv-evmcs
/-hv-evmcs
- Improve performance for nested virtualization (only supported on Intel CPUs).+hv-tlbflush
/-hv-tlbflush
- Improve performance in overcommitted Windows guests (may lead to guest BSOD on old CPUs).+ibpb
/-ibpb
- Allows improved Spectre mitigation on AMD CPUs.+md-clear
/-md-clear
- Required to let the guest OS know if MDS is mitigated correctly.+pcid
/-pcid
- Meltdown fix cost reduction on Westmere, Sandy- and Ivy Bridge Intel CPUs.+pdpe1gb
/-pdpe1gb
- Allows guest OS to use 1 GB size pages, if host HW supports it.+spec-ctrl
/-spec-ctrl
- Allows improved Spectre mitigation with Intel CPUs.+ssbd
/-ssbd
- Protection for "Speculative Store Bypass" for Intel models.+virt-ssbd
/-virt-ssbd
- Basis for "Speculative Store Bypass" protection for AMD models.
- Hotplugged int
- The number of hotplugged vCPUs (defaults
to
0
). - Limit int
- Limit of CPU usage,
0...128
. (defaults to0
-- no limit). - Numa bool
- Enable/disable NUMA. (default to
false
) - Sockets int
- The number of CPU sockets (defaults to
1
). - Type string
- The emulated CPU type, it's recommended to
use
x86-64-v2-AES
(defaults toqemu64
). - Units int
- The CPU units (defaults to
1024
).
- affinity String
- The CPU cores that are used to run the VM’s vCPU. The
value is a list of CPU IDs, separated by commas. The CPU IDs are zero-based.
For example,
0,1,2,3
(which also can be shortened to0-3
) means that the VM’s vCPUs are run on the first four CPU cores. Settingaffinity
is only allowed forroot@pam
authenticated user. - architecture String
- The CPU architecture (defaults to
x86_64
). - cores Integer
- The number of CPU cores (defaults to
1
). - flags List<String>
- The CPU flags.
+aes
/-aes
- Activate AES instruction set for HW acceleration.+amd-no-ssb
/-amd-no-ssb
- Notifies guest OS that host is not vulnerable for Spectre on AMD CPUs.+amd-ssbd
/-amd-ssbd
- Improves Spectre mitigation performance with AMD CPUs, best used with "virt-ssbd".+hv-evmcs
/-hv-evmcs
- Improve performance for nested virtualization (only supported on Intel CPUs).+hv-tlbflush
/-hv-tlbflush
- Improve performance in overcommitted Windows guests (may lead to guest BSOD on old CPUs).+ibpb
/-ibpb
- Allows improved Spectre mitigation on AMD CPUs.+md-clear
/-md-clear
- Required to let the guest OS know if MDS is mitigated correctly.+pcid
/-pcid
- Meltdown fix cost reduction on Westmere, Sandy- and Ivy Bridge Intel CPUs.+pdpe1gb
/-pdpe1gb
- Allows guest OS to use 1 GB size pages, if host HW supports it.+spec-ctrl
/-spec-ctrl
- Allows improved Spectre mitigation with Intel CPUs.+ssbd
/-ssbd
- Protection for "Speculative Store Bypass" for Intel models.+virt-ssbd
/-virt-ssbd
- Basis for "Speculative Store Bypass" protection for AMD models.
- hotplugged Integer
- The number of hotplugged vCPUs (defaults
to
0
). - limit Integer
- Limit of CPU usage,
0...128
. (defaults to0
-- no limit). - numa Boolean
- Enable/disable NUMA. (default to
false
) - sockets Integer
- The number of CPU sockets (defaults to
1
). - type String
- The emulated CPU type, it's recommended to
use
x86-64-v2-AES
(defaults toqemu64
). - units Integer
- The CPU units (defaults to
1024
).
- affinity string
- The CPU cores that are used to run the VM’s vCPU. The
value is a list of CPU IDs, separated by commas. The CPU IDs are zero-based.
For example,
0,1,2,3
(which also can be shortened to0-3
) means that the VM’s vCPUs are run on the first four CPU cores. Settingaffinity
is only allowed forroot@pam
authenticated user. - architecture string
- The CPU architecture (defaults to
x86_64
). - cores number
- The number of CPU cores (defaults to
1
). - flags string[]
- The CPU flags.
+aes
/-aes
- Activate AES instruction set for HW acceleration.+amd-no-ssb
/-amd-no-ssb
- Notifies guest OS that host is not vulnerable for Spectre on AMD CPUs.+amd-ssbd
/-amd-ssbd
- Improves Spectre mitigation performance with AMD CPUs, best used with "virt-ssbd".+hv-evmcs
/-hv-evmcs
- Improve performance for nested virtualization (only supported on Intel CPUs).+hv-tlbflush
/-hv-tlbflush
- Improve performance in overcommitted Windows guests (may lead to guest BSOD on old CPUs).+ibpb
/-ibpb
- Allows improved Spectre mitigation on AMD CPUs.+md-clear
/-md-clear
- Required to let the guest OS know if MDS is mitigated correctly.+pcid
/-pcid
- Meltdown fix cost reduction on Westmere, Sandy- and Ivy Bridge Intel CPUs.+pdpe1gb
/-pdpe1gb
- Allows guest OS to use 1 GB size pages, if host HW supports it.+spec-ctrl
/-spec-ctrl
- Allows improved Spectre mitigation with Intel CPUs.+ssbd
/-ssbd
- Protection for "Speculative Store Bypass" for Intel models.+virt-ssbd
/-virt-ssbd
- Basis for "Speculative Store Bypass" protection for AMD models.
- hotplugged number
- The number of hotplugged vCPUs (defaults
to
0
). - limit number
- Limit of CPU usage,
0...128
. (defaults to0
-- no limit). - numa boolean
- Enable/disable NUMA. (default to
false
) - sockets number
- The number of CPU sockets (defaults to
1
). - type string
- The emulated CPU type, it's recommended to
use
x86-64-v2-AES
(defaults toqemu64
). - units number
- The CPU units (defaults to
1024
).
- affinity str
- The CPU cores that are used to run the VM’s vCPU. The
value is a list of CPU IDs, separated by commas. The CPU IDs are zero-based.
For example,
0,1,2,3
(which also can be shortened to0-3
) means that the VM’s vCPUs are run on the first four CPU cores. Settingaffinity
is only allowed forroot@pam
authenticated user. - architecture str
- The CPU architecture (defaults to
x86_64
). - cores int
- The number of CPU cores (defaults to
1
). - flags Sequence[str]
- The CPU flags.
+aes
/-aes
- Activate AES instruction set for HW acceleration.+amd-no-ssb
/-amd-no-ssb
- Notifies guest OS that host is not vulnerable for Spectre on AMD CPUs.+amd-ssbd
/-amd-ssbd
- Improves Spectre mitigation performance with AMD CPUs, best used with "virt-ssbd".+hv-evmcs
/-hv-evmcs
- Improve performance for nested virtualization (only supported on Intel CPUs).+hv-tlbflush
/-hv-tlbflush
- Improve performance in overcommitted Windows guests (may lead to guest BSOD on old CPUs).+ibpb
/-ibpb
- Allows improved Spectre mitigation on AMD CPUs.+md-clear
/-md-clear
- Required to let the guest OS know if MDS is mitigated correctly.+pcid
/-pcid
- Meltdown fix cost reduction on Westmere, Sandy- and Ivy Bridge Intel CPUs.+pdpe1gb
/-pdpe1gb
- Allows guest OS to use 1 GB size pages, if host HW supports it.+spec-ctrl
/-spec-ctrl
- Allows improved Spectre mitigation with Intel CPUs.+ssbd
/-ssbd
- Protection for "Speculative Store Bypass" for Intel models.+virt-ssbd
/-virt-ssbd
- Basis for "Speculative Store Bypass" protection for AMD models.
- hotplugged int
- The number of hotplugged vCPUs (defaults
to
0
). - limit int
- Limit of CPU usage,
0...128
. (defaults to0
-- no limit). - numa bool
- Enable/disable NUMA. (default to
false
) - sockets int
- The number of CPU sockets (defaults to
1
). - type str
- The emulated CPU type, it's recommended to
use
x86-64-v2-AES
(defaults toqemu64
). - units int
- The CPU units (defaults to
1024
).
- affinity String
- The CPU cores that are used to run the VM’s vCPU. The
value is a list of CPU IDs, separated by commas. The CPU IDs are zero-based.
For example,
0,1,2,3
(which also can be shortened to0-3
) means that the VM’s vCPUs are run on the first four CPU cores. Settingaffinity
is only allowed forroot@pam
authenticated user. - architecture String
- The CPU architecture (defaults to
x86_64
). - cores Number
- The number of CPU cores (defaults to
1
). - flags List<String>
- The CPU flags.
+aes
/-aes
- Activate AES instruction set for HW acceleration.+amd-no-ssb
/-amd-no-ssb
- Notifies guest OS that host is not vulnerable for Spectre on AMD CPUs.+amd-ssbd
/-amd-ssbd
- Improves Spectre mitigation performance with AMD CPUs, best used with "virt-ssbd".+hv-evmcs
/-hv-evmcs
- Improve performance for nested virtualization (only supported on Intel CPUs).+hv-tlbflush
/-hv-tlbflush
- Improve performance in overcommitted Windows guests (may lead to guest BSOD on old CPUs).+ibpb
/-ibpb
- Allows improved Spectre mitigation on AMD CPUs.+md-clear
/-md-clear
- Required to let the guest OS know if MDS is mitigated correctly.+pcid
/-pcid
- Meltdown fix cost reduction on Westmere, Sandy- and Ivy Bridge Intel CPUs.+pdpe1gb
/-pdpe1gb
- Allows guest OS to use 1 GB size pages, if host HW supports it.+spec-ctrl
/-spec-ctrl
- Allows improved Spectre mitigation with Intel CPUs.+ssbd
/-ssbd
- Protection for "Speculative Store Bypass" for Intel models.+virt-ssbd
/-virt-ssbd
- Basis for "Speculative Store Bypass" protection for AMD models.
- hotplugged Number
- The number of hotplugged vCPUs (defaults
to
0
). - limit Number
- Limit of CPU usage,
0...128
. (defaults to0
-- no limit). - numa Boolean
- Enable/disable NUMA. (default to
false
) - sockets Number
- The number of CPU sockets (defaults to
1
). - type String
- The emulated CPU type, it's recommended to
use
x86-64-v2-AES
(defaults toqemu64
). - units Number
- The CPU units (defaults to
1024
).
VirtualMachineDisk, VirtualMachineDiskArgs
- Interface string
- The disk interface for Proxmox, currently
scsi
,sata
andvirtio
interfaces are supported. Append the disk index at the end, for example,virtio0
for the first virtio disk,virtio1
for the second, etc. - Aio string
- The disk AIO mode (defaults to
io_uring
). - Backup bool
- Whether the drive should be included when making backups (defaults to
true
). - Cache string
- The cache type (defaults to
none
). - Datastore
Id string - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - Discard string
- Whether to pass discard/trim requests to the
underlying storage. Supported values are
on
/ignore
(defaults toignore
). - File
Format string - The file format (defaults to
qcow2
). - File
Id string - The file ID for a disk image. The ID format is
<datastore_id>:<content_type>/<file_name>
, for examplelocal:iso/centos8.img
. Can be also taken fromproxmoxve.Download.File
resource. - Iothread bool
- Whether to use iothreads for this disk (defaults
to
false
). - Path
In stringDatastore - The in-datastore path to the disk image.
***Experimental.***Use to attach another VM's disks,
or (as root only) host's filesystem paths (
datastore_id
empty string). See "Example: Attached disks". - Replicate bool
- Whether the drive should be considered for replication jobs (defaults to
true
). - Serial string
- The serial number of the disk, up to 20 bytes long.
- Size int
- The disk size in gigabytes (defaults to
8
). - Speed
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Disk Speed - The speed limits.
- Ssd bool
- Whether to use an SSD emulation option for this disk (
defaults to
false
). Note that SSD emulation is not supported on VirtIO Block drives.
- Interface string
- The disk interface for Proxmox, currently
scsi
,sata
andvirtio
interfaces are supported. Append the disk index at the end, for example,virtio0
for the first virtio disk,virtio1
for the second, etc. - Aio string
- The disk AIO mode (defaults to
io_uring
). - Backup bool
- Whether the drive should be included when making backups (defaults to
true
). - Cache string
- The cache type (defaults to
none
). - Datastore
Id string - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - Discard string
- Whether to pass discard/trim requests to the
underlying storage. Supported values are
on
/ignore
(defaults toignore
). - File
Format string - The file format (defaults to
qcow2
). - File
Id string - The file ID for a disk image. The ID format is
<datastore_id>:<content_type>/<file_name>
, for examplelocal:iso/centos8.img
. Can be also taken fromproxmoxve.Download.File
resource. - Iothread bool
- Whether to use iothreads for this disk (defaults
to
false
). - Path
In stringDatastore - The in-datastore path to the disk image.
***Experimental.***Use to attach another VM's disks,
or (as root only) host's filesystem paths (
datastore_id
empty string). See "Example: Attached disks". - Replicate bool
- Whether the drive should be considered for replication jobs (defaults to
true
). - Serial string
- The serial number of the disk, up to 20 bytes long.
- Size int
- The disk size in gigabytes (defaults to
8
). - Speed
Virtual
Machine Disk Speed - The speed limits.
- Ssd bool
- Whether to use an SSD emulation option for this disk (
defaults to
false
). Note that SSD emulation is not supported on VirtIO Block drives.
- interface_ String
- The disk interface for Proxmox, currently
scsi
,sata
andvirtio
interfaces are supported. Append the disk index at the end, for example,virtio0
for the first virtio disk,virtio1
for the second, etc. - aio String
- The disk AIO mode (defaults to
io_uring
). - backup Boolean
- Whether the drive should be included when making backups (defaults to
true
). - cache String
- The cache type (defaults to
none
). - datastore
Id String - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - discard String
- Whether to pass discard/trim requests to the
underlying storage. Supported values are
on
/ignore
(defaults toignore
). - file
Format String - The file format (defaults to
qcow2
). - file
Id String - The file ID for a disk image. The ID format is
<datastore_id>:<content_type>/<file_name>
, for examplelocal:iso/centos8.img
. Can be also taken fromproxmoxve.Download.File
resource. - iothread Boolean
- Whether to use iothreads for this disk (defaults
to
false
). - path
In StringDatastore - The in-datastore path to the disk image.
***Experimental.***Use to attach another VM's disks,
or (as root only) host's filesystem paths (
datastore_id
empty string). See "Example: Attached disks". - replicate Boolean
- Whether the drive should be considered for replication jobs (defaults to
true
). - serial String
- The serial number of the disk, up to 20 bytes long.
- size Integer
- The disk size in gigabytes (defaults to
8
). - speed
Virtual
Machine Disk Speed - The speed limits.
- ssd Boolean
- Whether to use an SSD emulation option for this disk (
defaults to
false
). Note that SSD emulation is not supported on VirtIO Block drives.
- interface string
- The disk interface for Proxmox, currently
scsi
,sata
andvirtio
interfaces are supported. Append the disk index at the end, for example,virtio0
for the first virtio disk,virtio1
for the second, etc. - aio string
- The disk AIO mode (defaults to
io_uring
). - backup boolean
- Whether the drive should be included when making backups (defaults to
true
). - cache string
- The cache type (defaults to
none
). - datastore
Id string - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - discard string
- Whether to pass discard/trim requests to the
underlying storage. Supported values are
on
/ignore
(defaults toignore
). - file
Format string - The file format (defaults to
qcow2
). - file
Id string - The file ID for a disk image. The ID format is
<datastore_id>:<content_type>/<file_name>
, for examplelocal:iso/centos8.img
. Can be also taken fromproxmoxve.Download.File
resource. - iothread boolean
- Whether to use iothreads for this disk (defaults
to
false
). - path
In stringDatastore - The in-datastore path to the disk image.
***Experimental.***Use to attach another VM's disks,
or (as root only) host's filesystem paths (
datastore_id
empty string). See "Example: Attached disks". - replicate boolean
- Whether the drive should be considered for replication jobs (defaults to
true
). - serial string
- The serial number of the disk, up to 20 bytes long.
- size number
- The disk size in gigabytes (defaults to
8
). - speed
Virtual
Machine Disk Speed - The speed limits.
- ssd boolean
- Whether to use an SSD emulation option for this disk (
defaults to
false
). Note that SSD emulation is not supported on VirtIO Block drives.
- interface str
- The disk interface for Proxmox, currently
scsi
,sata
andvirtio
interfaces are supported. Append the disk index at the end, for example,virtio0
for the first virtio disk,virtio1
for the second, etc. - aio str
- The disk AIO mode (defaults to
io_uring
). - backup bool
- Whether the drive should be included when making backups (defaults to
true
). - cache str
- The cache type (defaults to
none
). - datastore_
id str - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - discard str
- Whether to pass discard/trim requests to the
underlying storage. Supported values are
on
/ignore
(defaults toignore
). - file_
format str - The file format (defaults to
qcow2
). - file_
id str - The file ID for a disk image. The ID format is
<datastore_id>:<content_type>/<file_name>
, for examplelocal:iso/centos8.img
. Can be also taken fromproxmoxve.Download.File
resource. - iothread bool
- Whether to use iothreads for this disk (defaults
to
false
). - path_
in_ strdatastore - The in-datastore path to the disk image.
***Experimental.***Use to attach another VM's disks,
or (as root only) host's filesystem paths (
datastore_id
empty string). See "Example: Attached disks". - replicate bool
- Whether the drive should be considered for replication jobs (defaults to
true
). - serial str
- The serial number of the disk, up to 20 bytes long.
- size int
- The disk size in gigabytes (defaults to
8
). - speed
vm.
Virtual Machine Disk Speed - The speed limits.
- ssd bool
- Whether to use an SSD emulation option for this disk (
defaults to
false
). Note that SSD emulation is not supported on VirtIO Block drives.
- interface String
- The disk interface for Proxmox, currently
scsi
,sata
andvirtio
interfaces are supported. Append the disk index at the end, for example,virtio0
for the first virtio disk,virtio1
for the second, etc. - aio String
- The disk AIO mode (defaults to
io_uring
). - backup Boolean
- Whether the drive should be included when making backups (defaults to
true
). - cache String
- The cache type (defaults to
none
). - datastore
Id String - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - discard String
- Whether to pass discard/trim requests to the
underlying storage. Supported values are
on
/ignore
(defaults toignore
). - file
Format String - The file format (defaults to
qcow2
). - file
Id String - The file ID for a disk image. The ID format is
<datastore_id>:<content_type>/<file_name>
, for examplelocal:iso/centos8.img
. Can be also taken fromproxmoxve.Download.File
resource. - iothread Boolean
- Whether to use iothreads for this disk (defaults
to
false
). - path
In StringDatastore - The in-datastore path to the disk image.
***Experimental.***Use to attach another VM's disks,
or (as root only) host's filesystem paths (
datastore_id
empty string). See "Example: Attached disks". - replicate Boolean
- Whether the drive should be considered for replication jobs (defaults to
true
). - serial String
- The serial number of the disk, up to 20 bytes long.
- size Number
- The disk size in gigabytes (defaults to
8
). - speed Property Map
- The speed limits.
- ssd Boolean
- Whether to use an SSD emulation option for this disk (
defaults to
false
). Note that SSD emulation is not supported on VirtIO Block drives.
VirtualMachineDiskSpeed, VirtualMachineDiskSpeedArgs
- Iops
Read int - The maximum read I/O in operations per second.
- Iops
Read intBurstable - The maximum unthrottled read I/O pool in operations per second.
- Iops
Write int - The maximum write I/O in operations per second.
- Iops
Write intBurstable - The maximum unthrottled write I/O pool in operations per second.
- Read int
- The maximum read speed in megabytes per second.
- Read
Burstable int - The maximum burstable read speed in megabytes per second.
- Write int
- The maximum write speed in megabytes per second.
- Write
Burstable int - The maximum burstable write speed in megabytes per second.
- Iops
Read int - The maximum read I/O in operations per second.
- Iops
Read intBurstable - The maximum unthrottled read I/O pool in operations per second.
- Iops
Write int - The maximum write I/O in operations per second.
- Iops
Write intBurstable - The maximum unthrottled write I/O pool in operations per second.
- Read int
- The maximum read speed in megabytes per second.
- Read
Burstable int - The maximum burstable read speed in megabytes per second.
- Write int
- The maximum write speed in megabytes per second.
- Write
Burstable int - The maximum burstable write speed in megabytes per second.
- iops
Read Integer - The maximum read I/O in operations per second.
- iops
Read IntegerBurstable - The maximum unthrottled read I/O pool in operations per second.
- iops
Write Integer - The maximum write I/O in operations per second.
- iops
Write IntegerBurstable - The maximum unthrottled write I/O pool in operations per second.
- read Integer
- The maximum read speed in megabytes per second.
- read
Burstable Integer - The maximum burstable read speed in megabytes per second.
- write Integer
- The maximum write speed in megabytes per second.
- write
Burstable Integer - The maximum burstable write speed in megabytes per second.
- iops
Read number - The maximum read I/O in operations per second.
- iops
Read numberBurstable - The maximum unthrottled read I/O pool in operations per second.
- iops
Write number - The maximum write I/O in operations per second.
- iops
Write numberBurstable - The maximum unthrottled write I/O pool in operations per second.
- read number
- The maximum read speed in megabytes per second.
- read
Burstable number - The maximum burstable read speed in megabytes per second.
- write number
- The maximum write speed in megabytes per second.
- write
Burstable number - The maximum burstable write speed in megabytes per second.
- iops_
read int - The maximum read I/O in operations per second.
- iops_
read_ intburstable - The maximum unthrottled read I/O pool in operations per second.
- iops_
write int - The maximum write I/O in operations per second.
- iops_
write_ intburstable - The maximum unthrottled write I/O pool in operations per second.
- read int
- The maximum read speed in megabytes per second.
- read_
burstable int - The maximum burstable read speed in megabytes per second.
- write int
- The maximum write speed in megabytes per second.
- write_
burstable int - The maximum burstable write speed in megabytes per second.
- iops
Read Number - The maximum read I/O in operations per second.
- iops
Read NumberBurstable - The maximum unthrottled read I/O pool in operations per second.
- iops
Write Number - The maximum write I/O in operations per second.
- iops
Write NumberBurstable - The maximum unthrottled write I/O pool in operations per second.
- read Number
- The maximum read speed in megabytes per second.
- read
Burstable Number - The maximum burstable read speed in megabytes per second.
- write Number
- The maximum write speed in megabytes per second.
- write
Burstable Number - The maximum burstable write speed in megabytes per second.
VirtualMachineEfiDisk, VirtualMachineEfiDiskArgs
- Datastore
Id string - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - File
Format string - The file format (defaults to
raw
). - Pre
Enrolled boolKeys - Use am EFI vars template with
distribution-specific and Microsoft Standard keys enrolled, if used with
EFI type=
4m
. Ignored for VMs with cpu.architecture=aarch64
(defaults tofalse
). - Type string
- Size and type of the OVMF EFI disk.
4m
is newer and recommended, and required for Secure Boot. For backwards compatibility use2m
. Ignored for VMs with cpu.architecture=aarch64
(defaults to2m
).
- Datastore
Id string - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - File
Format string - The file format (defaults to
raw
). - Pre
Enrolled boolKeys - Use am EFI vars template with
distribution-specific and Microsoft Standard keys enrolled, if used with
EFI type=
4m
. Ignored for VMs with cpu.architecture=aarch64
(defaults tofalse
). - Type string
- Size and type of the OVMF EFI disk.
4m
is newer and recommended, and required for Secure Boot. For backwards compatibility use2m
. Ignored for VMs with cpu.architecture=aarch64
(defaults to2m
).
- datastore
Id String - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - file
Format String - The file format (defaults to
raw
). - pre
Enrolled BooleanKeys - Use am EFI vars template with
distribution-specific and Microsoft Standard keys enrolled, if used with
EFI type=
4m
. Ignored for VMs with cpu.architecture=aarch64
(defaults tofalse
). - type String
- Size and type of the OVMF EFI disk.
4m
is newer and recommended, and required for Secure Boot. For backwards compatibility use2m
. Ignored for VMs with cpu.architecture=aarch64
(defaults to2m
).
- datastore
Id string - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - file
Format string - The file format (defaults to
raw
). - pre
Enrolled booleanKeys - Use am EFI vars template with
distribution-specific and Microsoft Standard keys enrolled, if used with
EFI type=
4m
. Ignored for VMs with cpu.architecture=aarch64
(defaults tofalse
). - type string
- Size and type of the OVMF EFI disk.
4m
is newer and recommended, and required for Secure Boot. For backwards compatibility use2m
. Ignored for VMs with cpu.architecture=aarch64
(defaults to2m
).
- datastore_
id str - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - file_
format str - The file format (defaults to
raw
). - pre_
enrolled_ boolkeys - Use am EFI vars template with
distribution-specific and Microsoft Standard keys enrolled, if used with
EFI type=
4m
. Ignored for VMs with cpu.architecture=aarch64
(defaults tofalse
). - type str
- Size and type of the OVMF EFI disk.
4m
is newer and recommended, and required for Secure Boot. For backwards compatibility use2m
. Ignored for VMs with cpu.architecture=aarch64
(defaults to2m
).
- datastore
Id String - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - file
Format String - The file format (defaults to
raw
). - pre
Enrolled BooleanKeys - Use am EFI vars template with
distribution-specific and Microsoft Standard keys enrolled, if used with
EFI type=
4m
. Ignored for VMs with cpu.architecture=aarch64
(defaults tofalse
). - type String
- Size and type of the OVMF EFI disk.
4m
is newer and recommended, and required for Secure Boot. For backwards compatibility use2m
. Ignored for VMs with cpu.architecture=aarch64
(defaults to2m
).
VirtualMachineHostpci, VirtualMachineHostpciArgs
- Device string
- The PCI device name for Proxmox, in form
of
hostpciX
whereX
is a sequential number from 0 to 3. - Id string
- The PCI device ID. This parameter is not compatible
with
api_token
and requires the rootusername
andpassword
configured in the proxmox provider. Use either this ormapping
. - Mapping string
- The resource mapping name of the device, for
example gpu. Use either this or
id
. - Mdev string
- The mediated device ID to use.
- Pcie bool
- Tells Proxmox to use a PCIe or PCI port. Some guests/device combination require PCIe rather than PCI. PCIe is only available for q35 machine types.
- Rom
File string - A path to a ROM file for the device to use. This
is a relative path under
/usr/share/kvm/
. - Rombar bool
- Makes the firmware ROM visible for the VM (defaults
to
true
). - Xvga bool
- Marks the PCI(e) device as the primary GPU of the VM.
With this enabled the
vga
configuration argument will be ignored.
- Device string
- The PCI device name for Proxmox, in form
of
hostpciX
whereX
is a sequential number from 0 to 3. - Id string
- The PCI device ID. This parameter is not compatible
with
api_token
and requires the rootusername
andpassword
configured in the proxmox provider. Use either this ormapping
. - Mapping string
- The resource mapping name of the device, for
example gpu. Use either this or
id
. - Mdev string
- The mediated device ID to use.
- Pcie bool
- Tells Proxmox to use a PCIe or PCI port. Some guests/device combination require PCIe rather than PCI. PCIe is only available for q35 machine types.
- Rom
File string - A path to a ROM file for the device to use. This
is a relative path under
/usr/share/kvm/
. - Rombar bool
- Makes the firmware ROM visible for the VM (defaults
to
true
). - Xvga bool
- Marks the PCI(e) device as the primary GPU of the VM.
With this enabled the
vga
configuration argument will be ignored.
- device String
- The PCI device name for Proxmox, in form
of
hostpciX
whereX
is a sequential number from 0 to 3. - id String
- The PCI device ID. This parameter is not compatible
with
api_token
and requires the rootusername
andpassword
configured in the proxmox provider. Use either this ormapping
. - mapping String
- The resource mapping name of the device, for
example gpu. Use either this or
id
. - mdev String
- The mediated device ID to use.
- pcie Boolean
- Tells Proxmox to use a PCIe or PCI port. Some guests/device combination require PCIe rather than PCI. PCIe is only available for q35 machine types.
- rom
File String - A path to a ROM file for the device to use. This
is a relative path under
/usr/share/kvm/
. - rombar Boolean
- Makes the firmware ROM visible for the VM (defaults
to
true
). - xvga Boolean
- Marks the PCI(e) device as the primary GPU of the VM.
With this enabled the
vga
configuration argument will be ignored.
- device string
- The PCI device name for Proxmox, in form
of
hostpciX
whereX
is a sequential number from 0 to 3. - id string
- The PCI device ID. This parameter is not compatible
with
api_token
and requires the rootusername
andpassword
configured in the proxmox provider. Use either this ormapping
. - mapping string
- The resource mapping name of the device, for
example gpu. Use either this or
id
. - mdev string
- The mediated device ID to use.
- pcie boolean
- Tells Proxmox to use a PCIe or PCI port. Some guests/device combination require PCIe rather than PCI. PCIe is only available for q35 machine types.
- rom
File string - A path to a ROM file for the device to use. This
is a relative path under
/usr/share/kvm/
. - rombar boolean
- Makes the firmware ROM visible for the VM (defaults
to
true
). - xvga boolean
- Marks the PCI(e) device as the primary GPU of the VM.
With this enabled the
vga
configuration argument will be ignored.
- device str
- The PCI device name for Proxmox, in form
of
hostpciX
whereX
is a sequential number from 0 to 3. - id str
- The PCI device ID. This parameter is not compatible
with
api_token
and requires the rootusername
andpassword
configured in the proxmox provider. Use either this ormapping
. - mapping str
- The resource mapping name of the device, for
example gpu. Use either this or
id
. - mdev str
- The mediated device ID to use.
- pcie bool
- Tells Proxmox to use a PCIe or PCI port. Some guests/device combination require PCIe rather than PCI. PCIe is only available for q35 machine types.
- rom_
file str - A path to a ROM file for the device to use. This
is a relative path under
/usr/share/kvm/
. - rombar bool
- Makes the firmware ROM visible for the VM (defaults
to
true
). - xvga bool
- Marks the PCI(e) device as the primary GPU of the VM.
With this enabled the
vga
configuration argument will be ignored.
- device String
- The PCI device name for Proxmox, in form
of
hostpciX
whereX
is a sequential number from 0 to 3. - id String
- The PCI device ID. This parameter is not compatible
with
api_token
and requires the rootusername
andpassword
configured in the proxmox provider. Use either this ormapping
. - mapping String
- The resource mapping name of the device, for
example gpu. Use either this or
id
. - mdev String
- The mediated device ID to use.
- pcie Boolean
- Tells Proxmox to use a PCIe or PCI port. Some guests/device combination require PCIe rather than PCI. PCIe is only available for q35 machine types.
- rom
File String - A path to a ROM file for the device to use. This
is a relative path under
/usr/share/kvm/
. - rombar Boolean
- Makes the firmware ROM visible for the VM (defaults
to
true
). - xvga Boolean
- Marks the PCI(e) device as the primary GPU of the VM.
With this enabled the
vga
configuration argument will be ignored.
VirtualMachineInitialization, VirtualMachineInitializationArgs
- Datastore
Id string - The identifier for the datastore to create the
cloud-init disk in (defaults to
local-lvm
). - Dns
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Initialization Dns - The DNS configuration.
- Interface string
- The hardware interface to connect the cloud-init
image to. Must be one of
ide0..3
,sata0..5
,scsi0..30
. Will be detected if the setting is missing but a cloud-init image is present, otherwise defaults toide2
. - Ip
Configs List<Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Initialization Ip Config> - The IP configuration (one block per network device).
- Meta
Data stringFile Id - The identifier for a file containing all meta data passed to the VM via cloud-init.
- Network
Data stringFile Id - The identifier for a file containing
network configuration data passed to the VM via cloud-init (conflicts
with
ip_config
). - Type string
- The cloud-init configuration format
- Upgrade bool
- Whether to do an automatic package upgrade after the first boot
- User
Account Pulumi.Proxmox VE. VM. Inputs. Virtual Machine Initialization User Account - The user account configuration (conflicts
with
user_data_file_id
). - User
Data stringFile Id - The identifier for a file containing
custom user data (conflicts with
user_account
). - Vendor
Data stringFile Id - The identifier for a file containing all vendor data passed to the VM via cloud-init.
- Datastore
Id string - The identifier for the datastore to create the
cloud-init disk in (defaults to
local-lvm
). - Dns
Virtual
Machine Initialization Dns - The DNS configuration.
- Interface string
- The hardware interface to connect the cloud-init
image to. Must be one of
ide0..3
,sata0..5
,scsi0..30
. Will be detected if the setting is missing but a cloud-init image is present, otherwise defaults toide2
. - Ip
Configs []VirtualMachine Initialization Ip Config - The IP configuration (one block per network device).
- Meta
Data stringFile Id - The identifier for a file containing all meta data passed to the VM via cloud-init.
- Network
Data stringFile Id - The identifier for a file containing
network configuration data passed to the VM via cloud-init (conflicts
with
ip_config
). - Type string
- The cloud-init configuration format
- Upgrade bool
- Whether to do an automatic package upgrade after the first boot
- User
Account VirtualMachine Initialization User Account - The user account configuration (conflicts
with
user_data_file_id
). - User
Data stringFile Id - The identifier for a file containing
custom user data (conflicts with
user_account
). - Vendor
Data stringFile Id - The identifier for a file containing all vendor data passed to the VM via cloud-init.
- datastore
Id String - The identifier for the datastore to create the
cloud-init disk in (defaults to
local-lvm
). - dns
Virtual
Machine Initialization Dns - The DNS configuration.
- interface_ String
- The hardware interface to connect the cloud-init
image to. Must be one of
ide0..3
,sata0..5
,scsi0..30
. Will be detected if the setting is missing but a cloud-init image is present, otherwise defaults toide2
. - ip
Configs List<VirtualMachine Initialization Ip Config> - The IP configuration (one block per network device).
- meta
Data StringFile Id - The identifier for a file containing all meta data passed to the VM via cloud-init.
- network
Data StringFile Id - The identifier for a file containing
network configuration data passed to the VM via cloud-init (conflicts
with
ip_config
). - type String
- The cloud-init configuration format
- upgrade Boolean
- Whether to do an automatic package upgrade after the first boot
- user
Account VirtualMachine Initialization User Account - The user account configuration (conflicts
with
user_data_file_id
). - user
Data StringFile Id - The identifier for a file containing
custom user data (conflicts with
user_account
). - vendor
Data StringFile Id - The identifier for a file containing all vendor data passed to the VM via cloud-init.
- datastore
Id string - The identifier for the datastore to create the
cloud-init disk in (defaults to
local-lvm
). - dns
Virtual
Machine Initialization Dns - The DNS configuration.
- interface string
- The hardware interface to connect the cloud-init
image to. Must be one of
ide0..3
,sata0..5
,scsi0..30
. Will be detected if the setting is missing but a cloud-init image is present, otherwise defaults toide2
. - ip
Configs VirtualMachine Initialization Ip Config[] - The IP configuration (one block per network device).
- meta
Data stringFile Id - The identifier for a file containing all meta data passed to the VM via cloud-init.
- network
Data stringFile Id - The identifier for a file containing
network configuration data passed to the VM via cloud-init (conflicts
with
ip_config
). - type string
- The cloud-init configuration format
- upgrade boolean
- Whether to do an automatic package upgrade after the first boot
- user
Account VirtualMachine Initialization User Account - The user account configuration (conflicts
with
user_data_file_id
). - user
Data stringFile Id - The identifier for a file containing
custom user data (conflicts with
user_account
). - vendor
Data stringFile Id - The identifier for a file containing all vendor data passed to the VM via cloud-init.
- datastore_
id str - The identifier for the datastore to create the
cloud-init disk in (defaults to
local-lvm
). - dns
vm.
Virtual Machine Initialization Dns - The DNS configuration.
- interface str
- The hardware interface to connect the cloud-init
image to. Must be one of
ide0..3
,sata0..5
,scsi0..30
. Will be detected if the setting is missing but a cloud-init image is present, otherwise defaults toide2
. - ip_
configs Sequence[vm.Virtual Machine Initialization Ip Config] - The IP configuration (one block per network device).
- meta_
data_ strfile_ id - The identifier for a file containing all meta data passed to the VM via cloud-init.
- network_
data_ strfile_ id - The identifier for a file containing
network configuration data passed to the VM via cloud-init (conflicts
with
ip_config
). - type str
- The cloud-init configuration format
- upgrade bool
- Whether to do an automatic package upgrade after the first boot
- user_
account vm.Virtual Machine Initialization User Account - The user account configuration (conflicts
with
user_data_file_id
). - user_
data_ strfile_ id - The identifier for a file containing
custom user data (conflicts with
user_account
). - vendor_
data_ strfile_ id - The identifier for a file containing all vendor data passed to the VM via cloud-init.
- datastore
Id String - The identifier for the datastore to create the
cloud-init disk in (defaults to
local-lvm
). - dns Property Map
- The DNS configuration.
- interface String
- The hardware interface to connect the cloud-init
image to. Must be one of
ide0..3
,sata0..5
,scsi0..30
. Will be detected if the setting is missing but a cloud-init image is present, otherwise defaults toide2
. - ip
Configs List<Property Map> - The IP configuration (one block per network device).
- meta
Data StringFile Id - The identifier for a file containing all meta data passed to the VM via cloud-init.
- network
Data StringFile Id - The identifier for a file containing
network configuration data passed to the VM via cloud-init (conflicts
with
ip_config
). - type String
- The cloud-init configuration format
- upgrade Boolean
- Whether to do an automatic package upgrade after the first boot
- user
Account Property Map - The user account configuration (conflicts
with
user_data_file_id
). - user
Data StringFile Id - The identifier for a file containing
custom user data (conflicts with
user_account
). - vendor
Data StringFile Id - The identifier for a file containing all vendor data passed to the VM via cloud-init.
VirtualMachineInitializationDns, VirtualMachineInitializationDnsArgs
VirtualMachineInitializationIpConfig, VirtualMachineInitializationIpConfigArgs
- Ipv4
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Initialization Ip Config Ipv4 - The IPv4 configuration.
- Ipv6
Pulumi.
Proxmox VE. VM. Inputs. Virtual Machine Initialization Ip Config Ipv6 - The IPv6 configuration.
- Ipv4
Virtual
Machine Initialization Ip Config Ipv4 - The IPv4 configuration.
- Ipv6
Virtual
Machine Initialization Ip Config Ipv6 - The IPv6 configuration.
- ipv4
Virtual
Machine Initialization Ip Config Ipv4 - The IPv4 configuration.
- ipv6
Virtual
Machine Initialization Ip Config Ipv6 - The IPv6 configuration.
- ipv4
Virtual
Machine Initialization Ip Config Ipv4 - The IPv4 configuration.
- ipv6
Virtual
Machine Initialization Ip Config Ipv6 - The IPv6 configuration.
- ipv4
vm.
Virtual Machine Initialization Ip Config Ipv4 - The IPv4 configuration.
- ipv6
vm.
Virtual Machine Initialization Ip Config Ipv6 - The IPv6 configuration.
- ipv4 Property Map
- The IPv4 configuration.
- ipv6 Property Map
- The IPv6 configuration.
VirtualMachineInitializationIpConfigIpv4, VirtualMachineInitializationIpConfigIpv4Args
VirtualMachineInitializationIpConfigIpv6, VirtualMachineInitializationIpConfigIpv6Args
VirtualMachineInitializationUserAccount, VirtualMachineInitializationUserAccountArgs
VirtualMachineMemory, VirtualMachineMemoryArgs
- Dedicated int
- The dedicated memory in megabytes (defaults
to
512
). - Floating int
- The floating memory in megabytes (defaults
to
0
). - Hugepages string
- Enable/disable hugepages memory (defaults to disable).
- Keep
Hugepages bool Keep hugepages memory after the VM is stopped (defaults to
false
).Settings
hugepages
andkeep_hugepages
are only allowed forroot@pam
authenticated user. And requiredcpu.numa
to be enabled.- int
- The shared memory in megabytes (defaults to
0
).
- Dedicated int
- The dedicated memory in megabytes (defaults
to
512
). - Floating int
- The floating memory in megabytes (defaults
to
0
). - Hugepages string
- Enable/disable hugepages memory (defaults to disable).
- Keep
Hugepages bool Keep hugepages memory after the VM is stopped (defaults to
false
).Settings
hugepages
andkeep_hugepages
are only allowed forroot@pam
authenticated user. And requiredcpu.numa
to be enabled.- int
- The shared memory in megabytes (defaults to
0
).
- dedicated Integer
- The dedicated memory in megabytes (defaults
to
512
). - floating Integer
- The floating memory in megabytes (defaults
to
0
). - hugepages String
- Enable/disable hugepages memory (defaults to disable).
- keep
Hugepages Boolean Keep hugepages memory after the VM is stopped (defaults to
false
).Settings
hugepages
andkeep_hugepages
are only allowed forroot@pam
authenticated user. And requiredcpu.numa
to be enabled.- Integer
- The shared memory in megabytes (defaults to
0
).
- dedicated number
- The dedicated memory in megabytes (defaults
to
512
). - floating number
- The floating memory in megabytes (defaults
to
0
). - hugepages string
- Enable/disable hugepages memory (defaults to disable).
- keep
Hugepages boolean Keep hugepages memory after the VM is stopped (defaults to
false
).Settings
hugepages
andkeep_hugepages
are only allowed forroot@pam
authenticated user. And requiredcpu.numa
to be enabled.- number
- The shared memory in megabytes (defaults to
0
).
- dedicated int
- The dedicated memory in megabytes (defaults
to
512
). - floating int
- The floating memory in megabytes (defaults
to
0
). - hugepages str
- Enable/disable hugepages memory (defaults to disable).
- keep_
hugepages bool Keep hugepages memory after the VM is stopped (defaults to
false
).Settings
hugepages
andkeep_hugepages
are only allowed forroot@pam
authenticated user. And requiredcpu.numa
to be enabled.- int
- The shared memory in megabytes (defaults to
0
).
- dedicated Number
- The dedicated memory in megabytes (defaults
to
512
). - floating Number
- The floating memory in megabytes (defaults
to
0
). - hugepages String
- Enable/disable hugepages memory (defaults to disable).
- keep
Hugepages Boolean Keep hugepages memory after the VM is stopped (defaults to
false
).Settings
hugepages
andkeep_hugepages
are only allowed forroot@pam
authenticated user. And requiredcpu.numa
to be enabled.- Number
- The shared memory in megabytes (defaults to
0
).
VirtualMachineNetworkDevice, VirtualMachineNetworkDeviceArgs
- Bridge string
- The name of the network bridge (defaults to
vmbr0
). - Disconnected bool
- Whether to disconnect the network device from the network (defaults to
false
). - Enabled bool
- Whether to enable the network device (defaults to
true
). - Firewall bool
- Whether this interface's firewall rules should be used (defaults to
false
). - Mac
Address string - The MAC address.
- Model string
- The network device model (defaults to
virtio
). - Mtu int
- Force MTU, for VirtIO only. Set to 1 to use the bridge MTU. Cannot be larger than the bridge MTU.
- Queues int
- The number of queues for VirtIO (1..64).
- Rate
Limit double - The rate limit in megabytes per second.
- Trunks string
- String containing a
;
separated list of VLAN trunks ("10;20;30"). Note that the VLAN-aware feature need to be enabled on the PVE Linux Bridge to use trunks. - Vlan
Id int - The VLAN identifier.
- Bridge string
- The name of the network bridge (defaults to
vmbr0
). - Disconnected bool
- Whether to disconnect the network device from the network (defaults to
false
). - Enabled bool
- Whether to enable the network device (defaults to
true
). - Firewall bool
- Whether this interface's firewall rules should be used (defaults to
false
). - Mac
Address string - The MAC address.
- Model string
- The network device model (defaults to
virtio
). - Mtu int
- Force MTU, for VirtIO only. Set to 1 to use the bridge MTU. Cannot be larger than the bridge MTU.
- Queues int
- The number of queues for VirtIO (1..64).
- Rate
Limit float64 - The rate limit in megabytes per second.
- Trunks string
- String containing a
;
separated list of VLAN trunks ("10;20;30"). Note that the VLAN-aware feature need to be enabled on the PVE Linux Bridge to use trunks. - Vlan
Id int - The VLAN identifier.
- bridge String
- The name of the network bridge (defaults to
vmbr0
). - disconnected Boolean
- Whether to disconnect the network device from the network (defaults to
false
). - enabled Boolean
- Whether to enable the network device (defaults to
true
). - firewall Boolean
- Whether this interface's firewall rules should be used (defaults to
false
). - mac
Address String - The MAC address.
- model String
- The network device model (defaults to
virtio
). - mtu Integer
- Force MTU, for VirtIO only. Set to 1 to use the bridge MTU. Cannot be larger than the bridge MTU.
- queues Integer
- The number of queues for VirtIO (1..64).
- rate
Limit Double - The rate limit in megabytes per second.
- trunks String
- String containing a
;
separated list of VLAN trunks ("10;20;30"). Note that the VLAN-aware feature need to be enabled on the PVE Linux Bridge to use trunks. - vlan
Id Integer - The VLAN identifier.
- bridge string
- The name of the network bridge (defaults to
vmbr0
). - disconnected boolean
- Whether to disconnect the network device from the network (defaults to
false
). - enabled boolean
- Whether to enable the network device (defaults to
true
). - firewall boolean
- Whether this interface's firewall rules should be used (defaults to
false
). - mac
Address string - The MAC address.
- model string
- The network device model (defaults to
virtio
). - mtu number
- Force MTU, for VirtIO only. Set to 1 to use the bridge MTU. Cannot be larger than the bridge MTU.
- queues number
- The number of queues for VirtIO (1..64).
- rate
Limit number - The rate limit in megabytes per second.
- trunks string
- String containing a
;
separated list of VLAN trunks ("10;20;30"). Note that the VLAN-aware feature need to be enabled on the PVE Linux Bridge to use trunks. - vlan
Id number - The VLAN identifier.
- bridge str
- The name of the network bridge (defaults to
vmbr0
). - disconnected bool
- Whether to disconnect the network device from the network (defaults to
false
). - enabled bool
- Whether to enable the network device (defaults to
true
). - firewall bool
- Whether this interface's firewall rules should be used (defaults to
false
). - mac_
address str - The MAC address.
- model str
- The network device model (defaults to
virtio
). - mtu int
- Force MTU, for VirtIO only. Set to 1 to use the bridge MTU. Cannot be larger than the bridge MTU.
- queues int
- The number of queues for VirtIO (1..64).
- rate_
limit float - The rate limit in megabytes per second.
- trunks str
- String containing a
;
separated list of VLAN trunks ("10;20;30"). Note that the VLAN-aware feature need to be enabled on the PVE Linux Bridge to use trunks. - vlan_
id int - The VLAN identifier.
- bridge String
- The name of the network bridge (defaults to
vmbr0
). - disconnected Boolean
- Whether to disconnect the network device from the network (defaults to
false
). - enabled Boolean
- Whether to enable the network device (defaults to
true
). - firewall Boolean
- Whether this interface's firewall rules should be used (defaults to
false
). - mac
Address String - The MAC address.
- model String
- The network device model (defaults to
virtio
). - mtu Number
- Force MTU, for VirtIO only. Set to 1 to use the bridge MTU. Cannot be larger than the bridge MTU.
- queues Number
- The number of queues for VirtIO (1..64).
- rate
Limit Number - The rate limit in megabytes per second.
- trunks String
- String containing a
;
separated list of VLAN trunks ("10;20;30"). Note that the VLAN-aware feature need to be enabled on the PVE Linux Bridge to use trunks. - vlan
Id Number - The VLAN identifier.
VirtualMachineNuma, VirtualMachineNumaArgs
- Cpus string
- The CPU cores to assign to the NUMA node (format is
0-7;16-31
). - Device string
- The NUMA device name for Proxmox, in form
of
numaX
whereX
is a sequential number from 0 to 7. - Memory int
- The memory in megabytes to assign to the NUMA node.
- Hostnodes string
- The NUMA host nodes.
- Policy string
- The NUMA policy (defaults to
preferred
).
- Cpus string
- The CPU cores to assign to the NUMA node (format is
0-7;16-31
). - Device string
- The NUMA device name for Proxmox, in form
of
numaX
whereX
is a sequential number from 0 to 7. - Memory int
- The memory in megabytes to assign to the NUMA node.
- Hostnodes string
- The NUMA host nodes.
- Policy string
- The NUMA policy (defaults to
preferred
).
- cpus String
- The CPU cores to assign to the NUMA node (format is
0-7;16-31
). - device String
- The NUMA device name for Proxmox, in form
of
numaX
whereX
is a sequential number from 0 to 7. - memory Integer
- The memory in megabytes to assign to the NUMA node.
- hostnodes String
- The NUMA host nodes.
- policy String
- The NUMA policy (defaults to
preferred
).
- cpus string
- The CPU cores to assign to the NUMA node (format is
0-7;16-31
). - device string
- The NUMA device name for Proxmox, in form
of
numaX
whereX
is a sequential number from 0 to 7. - memory number
- The memory in megabytes to assign to the NUMA node.
- hostnodes string
- The NUMA host nodes.
- policy string
- The NUMA policy (defaults to
preferred
).
- cpus str
- The CPU cores to assign to the NUMA node (format is
0-7;16-31
). - device str
- The NUMA device name for Proxmox, in form
of
numaX
whereX
is a sequential number from 0 to 7. - memory int
- The memory in megabytes to assign to the NUMA node.
- hostnodes str
- The NUMA host nodes.
- policy str
- The NUMA policy (defaults to
preferred
).
- cpus String
- The CPU cores to assign to the NUMA node (format is
0-7;16-31
). - device String
- The NUMA device name for Proxmox, in form
of
numaX
whereX
is a sequential number from 0 to 7. - memory Number
- The memory in megabytes to assign to the NUMA node.
- hostnodes String
- The NUMA host nodes.
- policy String
- The NUMA policy (defaults to
preferred
).
VirtualMachineOperatingSystem, VirtualMachineOperatingSystemArgs
- Type string
- The type (defaults to
other
).
- Type string
- The type (defaults to
other
).
- type String
- The type (defaults to
other
).
- type string
- The type (defaults to
other
).
- type str
- The type (defaults to
other
).
- type String
- The type (defaults to
other
).
VirtualMachineSerialDevice, VirtualMachineSerialDeviceArgs
- Device string
- The device (defaults to
socket
)./dev/*
- A host serial device.
- Device string
- The device (defaults to
socket
)./dev/*
- A host serial device.
- device String
- The device (defaults to
socket
)./dev/*
- A host serial device.
- device string
- The device (defaults to
socket
)./dev/*
- A host serial device.
- device str
- The device (defaults to
socket
)./dev/*
- A host serial device.
- device String
- The device (defaults to
socket
)./dev/*
- A host serial device.
VirtualMachineSmbios, VirtualMachineSmbiosArgs
VirtualMachineStartup, VirtualMachineStartupArgs
- down_
delay int - A non-negative number defining the delay in seconds before the next VM is shut down.
- order int
- A non-negative number defining the general startup order.
- up_
delay int - A non-negative number defining the delay in seconds before the next VM is started.
VirtualMachineTpmState, VirtualMachineTpmStateArgs
- Datastore
Id string - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - Version string
- TPM state device version. Can be
v1.2
orv2.0
. (defaults tov2.0
).
- Datastore
Id string - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - Version string
- TPM state device version. Can be
v1.2
orv2.0
. (defaults tov2.0
).
- datastore
Id String - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - version String
- TPM state device version. Can be
v1.2
orv2.0
. (defaults tov2.0
).
- datastore
Id string - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - version string
- TPM state device version. Can be
v1.2
orv2.0
. (defaults tov2.0
).
- datastore_
id str - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - version str
- TPM state device version. Can be
v1.2
orv2.0
. (defaults tov2.0
).
- datastore
Id String - The identifier for the datastore to create
the disk in (defaults to
local-lvm
). - version String
- TPM state device version. Can be
v1.2
orv2.0
. (defaults tov2.0
).
VirtualMachineUsb, VirtualMachineUsbArgs
VirtualMachineVga, VirtualMachineVgaArgs
- Clipboard string
- Enable VNC clipboard by setting to
vnc
. See the Proxmox documentation section 10.2.8 for more information. - Enabled bool
- Whether to enable the VGA device
- Memory int
- The VGA memory in megabytes (defaults to
16
). - Type string
- The VGA type (defaults to
std
).
- Clipboard string
- Enable VNC clipboard by setting to
vnc
. See the Proxmox documentation section 10.2.8 for more information. - Enabled bool
- Whether to enable the VGA device
- Memory int
- The VGA memory in megabytes (defaults to
16
). - Type string
- The VGA type (defaults to
std
).
- clipboard String
- Enable VNC clipboard by setting to
vnc
. See the Proxmox documentation section 10.2.8 for more information. - enabled Boolean
- Whether to enable the VGA device
- memory Integer
- The VGA memory in megabytes (defaults to
16
). - type String
- The VGA type (defaults to
std
).
- clipboard string
- Enable VNC clipboard by setting to
vnc
. See the Proxmox documentation section 10.2.8 for more information. - enabled boolean
- Whether to enable the VGA device
- memory number
- The VGA memory in megabytes (defaults to
16
). - type string
- The VGA type (defaults to
std
).
- clipboard str
- Enable VNC clipboard by setting to
vnc
. See the Proxmox documentation section 10.2.8 for more information. - enabled bool
- Whether to enable the VGA device
- memory int
- The VGA memory in megabytes (defaults to
16
). - type str
- The VGA type (defaults to
std
).
- clipboard String
- Enable VNC clipboard by setting to
vnc
. See the Proxmox documentation section 10.2.8 for more information. - enabled Boolean
- Whether to enable the VGA device
- memory Number
- The VGA memory in megabytes (defaults to
16
). - type String
- The VGA type (defaults to
std
).
Import
Instances can be imported using the node_name
and the vm_id
, e.g.,
bash
$ pulumi import proxmoxve:VM/virtualMachine:VirtualMachine ubuntu_vm first-node/4321
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- proxmoxve muhlba91/pulumi-proxmoxve
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
proxmox
Terraform Provider.
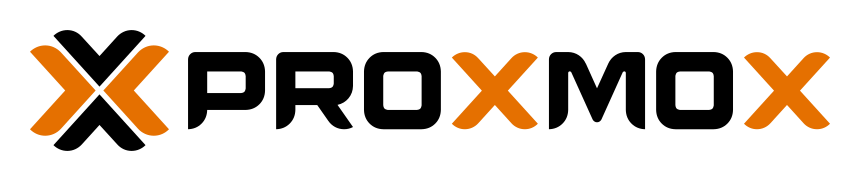