splight.Action
Explore with Pulumi AI
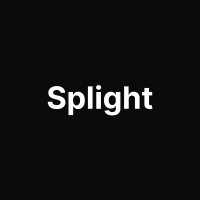
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as splight from "@splightplatform/pulumi-splight";
const myAsset = new splight.Asset("myAsset", {
description: "My Asset Description",
geometry: JSON.stringify({
type: "GeometryCollection",
geometries: [{
type: "Point",
coordinates: [
0,
0,
],
}],
}),
});
const myAttribute = new splight.AssetAttribute("myAttribute", {
type: "Number",
unit: "meters",
asset: myAsset.id,
});
const myAction = new splight.Action("myAction", {
asset: {
id: myAsset.id,
name: myAsset.name,
},
setpoints: [{
value: JSON.stringify(1),
attribute: {
id: myAttribute.id,
name: myAttribute.name,
},
}],
});
import pulumi
import json
import pulumi_splight as splight
my_asset = splight.Asset("myAsset",
description="My Asset Description",
geometry=json.dumps({
"type": "GeometryCollection",
"geometries": [{
"type": "Point",
"coordinates": [
0,
0,
],
}],
}))
my_attribute = splight.AssetAttribute("myAttribute",
type="Number",
unit="meters",
asset=my_asset.id)
my_action = splight.Action("myAction",
asset={
"id": my_asset.id,
"name": my_asset.name,
},
setpoints=[{
"value": json.dumps(1),
"attribute": {
"id": my_attribute.id,
"name": my_attribute.name,
},
}])
package main
import (
"encoding/json"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
"github.com/splightplatform/pulumi-splight/sdk/go/splight"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
tmpJSON0, err := json.Marshal(map[string]interface{}{
"type": "GeometryCollection",
"geometries": []map[string]interface{}{
map[string]interface{}{
"type": "Point",
"coordinates": []float64{
0,
0,
},
},
},
})
if err != nil {
return err
}
json0 := string(tmpJSON0)
myAsset, err := splight.NewAsset(ctx, "myAsset", &splight.AssetArgs{
Description: pulumi.String("My Asset Description"),
Geometry: pulumi.String(json0),
})
if err != nil {
return err
}
myAttribute, err := splight.NewAssetAttribute(ctx, "myAttribute", &splight.AssetAttributeArgs{
Type: pulumi.String("Number"),
Unit: pulumi.String("meters"),
Asset: myAsset.ID(),
})
if err != nil {
return err
}
tmpJSON1, err := json.Marshal(1)
if err != nil {
return err
}
json1 := string(tmpJSON1)
_, err = splight.NewAction(ctx, "myAction", &splight.ActionArgs{
Asset: &splight.ActionAssetArgs{
Id: myAsset.ID(),
Name: myAsset.Name,
},
Setpoints: splight.ActionSetpointArray{
&splight.ActionSetpointArgs{
Value: pulumi.String(json1),
Attribute: &splight.ActionSetpointAttributeArgs{
Id: myAttribute.ID(),
Name: myAttribute.Name,
},
},
},
})
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using System.Text.Json;
using Pulumi;
using Splight = Splight.Splight;
return await Deployment.RunAsync(() =>
{
var myAsset = new Splight.Asset("myAsset", new()
{
Description = "My Asset Description",
Geometry = JsonSerializer.Serialize(new Dictionary<string, object?>
{
["type"] = "GeometryCollection",
["geometries"] = new[]
{
new Dictionary<string, object?>
{
["type"] = "Point",
["coordinates"] = new[]
{
0,
0,
},
},
},
}),
});
var myAttribute = new Splight.AssetAttribute("myAttribute", new()
{
Type = "Number",
Unit = "meters",
Asset = myAsset.Id,
});
var myAction = new Splight.Action("myAction", new()
{
Asset = new Splight.Inputs.ActionAssetArgs
{
Id = myAsset.Id,
Name = myAsset.Name,
},
Setpoints = new[]
{
new Splight.Inputs.ActionSetpointArgs
{
Value = JsonSerializer.Serialize(1),
Attribute = new Splight.Inputs.ActionSetpointAttributeArgs
{
Id = myAttribute.Id,
Name = myAttribute.Name,
},
},
},
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.splight.Asset;
import com.pulumi.splight.AssetArgs;
import com.pulumi.splight.AssetAttribute;
import com.pulumi.splight.AssetAttributeArgs;
import com.pulumi.splight.Action;
import com.pulumi.splight.ActionArgs;
import com.pulumi.splight.inputs.ActionAssetArgs;
import com.pulumi.splight.inputs.ActionSetpointArgs;
import com.pulumi.splight.inputs.ActionSetpointAttributeArgs;
import static com.pulumi.codegen.internal.Serialization.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var myAsset = new Asset("myAsset", AssetArgs.builder()
.description("My Asset Description")
.geometry(serializeJson(
jsonObject(
jsonProperty("type", "GeometryCollection"),
jsonProperty("geometries", jsonArray(jsonObject(
jsonProperty("type", "Point"),
jsonProperty("coordinates", jsonArray(
0,
0
))
)))
)))
.build());
var myAttribute = new AssetAttribute("myAttribute", AssetAttributeArgs.builder()
.type("Number")
.unit("meters")
.asset(myAsset.id())
.build());
var myAction = new Action("myAction", ActionArgs.builder()
.asset(ActionAssetArgs.builder()
.id(myAsset.id())
.name(myAsset.name())
.build())
.setpoints(ActionSetpointArgs.builder()
.value(serializeJson(
1))
.attribute(ActionSetpointAttributeArgs.builder()
.id(myAttribute.id())
.name(myAttribute.name())
.build())
.build())
.build());
}
}
resources:
myAsset:
type: splight:Asset
properties:
description: My Asset Description
geometry:
fn::toJSON:
type: GeometryCollection
geometries:
- type: Point
coordinates:
- 0
- 0
myAttribute:
type: splight:AssetAttribute
properties:
type: Number
unit: meters
asset: ${myAsset.id}
myAction:
type: splight:Action
properties:
asset:
id: ${myAsset.id}
name: ${myAsset.name}
setpoints:
- value:
fn::toJSON: 1
attribute:
id: ${myAttribute.id}
name: ${myAttribute.name}
Create Action Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new Action(name: string, args: ActionArgs, opts?: CustomResourceOptions);
@overload
def Action(resource_name: str,
args: ActionArgs,
opts: Optional[ResourceOptions] = None)
@overload
def Action(resource_name: str,
opts: Optional[ResourceOptions] = None,
asset: Optional[ActionAssetArgs] = None,
setpoints: Optional[Sequence[ActionSetpointArgs]] = None,
name: Optional[str] = None)
func NewAction(ctx *Context, name string, args ActionArgs, opts ...ResourceOption) (*Action, error)
public Action(string name, ActionArgs args, CustomResourceOptions? opts = null)
public Action(String name, ActionArgs args)
public Action(String name, ActionArgs args, CustomResourceOptions options)
type: splight:Action
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args ActionArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args ActionArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args ActionArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args ActionArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args ActionArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var actionResource = new Splight.Action("actionResource", new()
{
Asset = new Splight.Inputs.ActionAssetArgs
{
Id = "string",
Name = "string",
},
Setpoints = new[]
{
new Splight.Inputs.ActionSetpointArgs
{
Attribute = new Splight.Inputs.ActionSetpointAttributeArgs
{
Id = "string",
Name = "string",
},
Value = "string",
Id = "string",
Name = "string",
},
},
Name = "string",
});
example, err := splight.NewAction(ctx, "actionResource", &splight.ActionArgs{
Asset: &splight.ActionAssetArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
Setpoints: splight.ActionSetpointArray{
&splight.ActionSetpointArgs{
Attribute: &splight.ActionSetpointAttributeArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
Value: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
},
Name: pulumi.String("string"),
})
var actionResource = new Action("actionResource", ActionArgs.builder()
.asset(ActionAssetArgs.builder()
.id("string")
.name("string")
.build())
.setpoints(ActionSetpointArgs.builder()
.attribute(ActionSetpointAttributeArgs.builder()
.id("string")
.name("string")
.build())
.value("string")
.id("string")
.name("string")
.build())
.name("string")
.build());
action_resource = splight.Action("actionResource",
asset=splight.ActionAssetArgs(
id="string",
name="string",
),
setpoints=[splight.ActionSetpointArgs(
attribute=splight.ActionSetpointAttributeArgs(
id="string",
name="string",
),
value="string",
id="string",
name="string",
)],
name="string")
const actionResource = new splight.Action("actionResource", {
asset: {
id: "string",
name: "string",
},
setpoints: [{
attribute: {
id: "string",
name: "string",
},
value: "string",
id: "string",
name: "string",
}],
name: "string",
});
type: splight:Action
properties:
asset:
id: string
name: string
name: string
setpoints:
- attribute:
id: string
name: string
id: string
name: string
value: string
Action Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The Action resource accepts the following input properties:
- Asset
Splight.
Splight. Inputs. Action Asset - target asset of the setpoint
- Setpoints
List<Splight.
Splight. Inputs. Action Setpoint> - action setpoints
- Name string
- the name of the action to be created
- Asset
Action
Asset Args - target asset of the setpoint
- Setpoints
[]Action
Setpoint Args - action setpoints
- Name string
- the name of the action to be created
- asset
Action
Asset - target asset of the setpoint
- setpoints
List<Action
Setpoint> - action setpoints
- name String
- the name of the action to be created
- asset
Action
Asset - target asset of the setpoint
- setpoints
Action
Setpoint[] - action setpoints
- name string
- the name of the action to be created
- asset
Action
Asset Args - target asset of the setpoint
- setpoints
Sequence[Action
Setpoint Args] - action setpoints
- name str
- the name of the action to be created
- asset Property Map
- target asset of the setpoint
- setpoints List<Property Map>
- action setpoints
- name String
- the name of the action to be created
Outputs
All input properties are implicitly available as output properties. Additionally, the Action resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing Action Resource
Get an existing Action resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: ActionState, opts?: CustomResourceOptions): Action
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
asset: Optional[ActionAssetArgs] = None,
name: Optional[str] = None,
setpoints: Optional[Sequence[ActionSetpointArgs]] = None) -> Action
func GetAction(ctx *Context, name string, id IDInput, state *ActionState, opts ...ResourceOption) (*Action, error)
public static Action Get(string name, Input<string> id, ActionState? state, CustomResourceOptions? opts = null)
public static Action get(String name, Output<String> id, ActionState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Asset
Splight.
Splight. Inputs. Action Asset - target asset of the setpoint
- Name string
- the name of the action to be created
- Setpoints
List<Splight.
Splight. Inputs. Action Setpoint> - action setpoints
- Asset
Action
Asset Args - target asset of the setpoint
- Name string
- the name of the action to be created
- Setpoints
[]Action
Setpoint Args - action setpoints
- asset
Action
Asset - target asset of the setpoint
- name String
- the name of the action to be created
- setpoints
List<Action
Setpoint> - action setpoints
- asset
Action
Asset - target asset of the setpoint
- name string
- the name of the action to be created
- setpoints
Action
Setpoint[] - action setpoints
- asset
Action
Asset Args - target asset of the setpoint
- name str
- the name of the action to be created
- setpoints
Sequence[Action
Setpoint Args] - action setpoints
- asset Property Map
- target asset of the setpoint
- name String
- the name of the action to be created
- setpoints List<Property Map>
- action setpoints
Supporting Types
ActionAsset, ActionAssetArgs
ActionSetpoint, ActionSetpointArgs
- Attribute
Splight.
Splight. Inputs. Action Setpoint Attribute - the target attribute of the setpoint which should also be an attribute of the specified asset
- Value string
- JSON encoded scalar value
- Id string
- setpoint Id
- Name string
- setpoint name
- Attribute
Action
Setpoint Attribute - the target attribute of the setpoint which should also be an attribute of the specified asset
- Value string
- JSON encoded scalar value
- Id string
- setpoint Id
- Name string
- setpoint name
- attribute
Action
Setpoint Attribute - the target attribute of the setpoint which should also be an attribute of the specified asset
- value String
- JSON encoded scalar value
- id String
- setpoint Id
- name String
- setpoint name
- attribute
Action
Setpoint Attribute - the target attribute of the setpoint which should also be an attribute of the specified asset
- value string
- JSON encoded scalar value
- id string
- setpoint Id
- name string
- setpoint name
- attribute
Action
Setpoint Attribute - the target attribute of the setpoint which should also be an attribute of the specified asset
- value str
- JSON encoded scalar value
- id str
- setpoint Id
- name str
- setpoint name
- attribute Property Map
- the target attribute of the setpoint which should also be an attribute of the specified asset
- value String
- JSON encoded scalar value
- id String
- setpoint Id
- name String
- setpoint name
ActionSetpointAttribute, ActionSetpointAttributeArgs
Import
$ pulumi import splight:index/action:Action [options] splight_action.<name> <action_id>
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- splight splightplatform/pulumi-splight
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
splight
Terraform Provider.
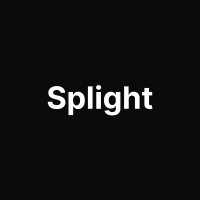