splight.Alert
Explore with Pulumi AI
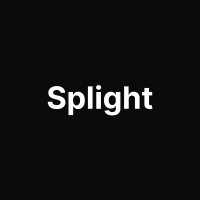
Example Usage
Create Alert Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new Alert(name: string, args: AlertArgs, opts?: CustomResourceOptions);
@overload
def Alert(resource_name: str,
args: AlertArgs,
opts: Optional[ResourceOptions] = None)
@overload
def Alert(resource_name: str,
opts: Optional[ResourceOptions] = None,
operator: Optional[str] = None,
alert_items: Optional[Sequence[AlertAlertItemArgs]] = None,
type: Optional[str] = None,
time_window: Optional[int] = None,
thresholds: Optional[Sequence[AlertThresholdArgs]] = None,
aggregation: Optional[str] = None,
target_variable: Optional[str] = None,
severity: Optional[str] = None,
description: Optional[str] = None,
cron_minutes: Optional[int] = None,
name: Optional[str] = None,
rate_unit: Optional[str] = None,
rate_value: Optional[int] = None,
related_assets: Optional[Sequence[AlertRelatedAssetArgs]] = None,
cron_year: Optional[int] = None,
tags: Optional[Sequence[AlertTagArgs]] = None,
cron_month: Optional[int] = None,
cron_hours: Optional[int] = None,
cron_dow: Optional[int] = None,
cron_dom: Optional[int] = None)
func NewAlert(ctx *Context, name string, args AlertArgs, opts ...ResourceOption) (*Alert, error)
public Alert(string name, AlertArgs args, CustomResourceOptions? opts = null)
type: splight:Alert
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args AlertArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args AlertArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args AlertArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args AlertArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args AlertArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var alertResource = new Splight.Alert("alertResource", new()
{
Operator = "string",
AlertItems = new[]
{
new Splight.Inputs.AlertAlertItemArgs
{
Expression = "string",
ExpressionPlain = "string",
QueryFilterAsset = new Splight.Inputs.AlertAlertItemQueryFilterAssetArgs
{
Id = "string",
Name = "string",
},
QueryFilterAttribute = new Splight.Inputs.AlertAlertItemQueryFilterAttributeArgs
{
Id = "string",
Name = "string",
},
QueryGroupFunction = "string",
QueryGroupUnit = "string",
QueryPlain = "string",
RefId = "string",
Type = "string",
Id = "string",
},
},
Type = "string",
TimeWindow = 0,
Thresholds = new[]
{
new Splight.Inputs.AlertThresholdArgs
{
Status = "string",
Value = 0,
StatusText = "string",
},
},
Aggregation = "string",
TargetVariable = "string",
Severity = "string",
Description = "string",
CronMinutes = 0,
Name = "string",
RateUnit = "string",
RateValue = 0,
RelatedAssets = new[]
{
new Splight.Inputs.AlertRelatedAssetArgs
{
Id = "string",
Name = "string",
},
},
CronYear = 0,
Tags = new[]
{
new Splight.Inputs.AlertTagArgs
{
Id = "string",
Name = "string",
},
},
CronMonth = 0,
CronHours = 0,
CronDow = 0,
CronDom = 0,
});
example, err := splight.NewAlert(ctx, "alertResource", &splight.AlertArgs{
Operator: pulumi.String("string"),
AlertItems: splight.AlertAlertItemArray{
&splight.AlertAlertItemArgs{
Expression: pulumi.String("string"),
ExpressionPlain: pulumi.String("string"),
QueryFilterAsset: &splight.AlertAlertItemQueryFilterAssetArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
QueryFilterAttribute: &splight.AlertAlertItemQueryFilterAttributeArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
QueryGroupFunction: pulumi.String("string"),
QueryGroupUnit: pulumi.String("string"),
QueryPlain: pulumi.String("string"),
RefId: pulumi.String("string"),
Type: pulumi.String("string"),
Id: pulumi.String("string"),
},
},
Type: pulumi.String("string"),
TimeWindow: pulumi.Int(0),
Thresholds: splight.AlertThresholdArray{
&splight.AlertThresholdArgs{
Status: pulumi.String("string"),
Value: pulumi.Float64(0),
StatusText: pulumi.String("string"),
},
},
Aggregation: pulumi.String("string"),
TargetVariable: pulumi.String("string"),
Severity: pulumi.String("string"),
Description: pulumi.String("string"),
CronMinutes: pulumi.Int(0),
Name: pulumi.String("string"),
RateUnit: pulumi.String("string"),
RateValue: pulumi.Int(0),
RelatedAssets: splight.AlertRelatedAssetArray{
&splight.AlertRelatedAssetArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
},
CronYear: pulumi.Int(0),
Tags: splight.AlertTagArray{
&splight.AlertTagArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
},
CronMonth: pulumi.Int(0),
CronHours: pulumi.Int(0),
CronDow: pulumi.Int(0),
CronDom: pulumi.Int(0),
})
var alertResource = new Alert("alertResource", AlertArgs.builder()
.operator("string")
.alertItems(AlertAlertItemArgs.builder()
.expression("string")
.expressionPlain("string")
.queryFilterAsset(AlertAlertItemQueryFilterAssetArgs.builder()
.id("string")
.name("string")
.build())
.queryFilterAttribute(AlertAlertItemQueryFilterAttributeArgs.builder()
.id("string")
.name("string")
.build())
.queryGroupFunction("string")
.queryGroupUnit("string")
.queryPlain("string")
.refId("string")
.type("string")
.id("string")
.build())
.type("string")
.timeWindow(0)
.thresholds(AlertThresholdArgs.builder()
.status("string")
.value(0)
.statusText("string")
.build())
.aggregation("string")
.targetVariable("string")
.severity("string")
.description("string")
.cronMinutes(0)
.name("string")
.rateUnit("string")
.rateValue(0)
.relatedAssets(AlertRelatedAssetArgs.builder()
.id("string")
.name("string")
.build())
.cronYear(0)
.tags(AlertTagArgs.builder()
.id("string")
.name("string")
.build())
.cronMonth(0)
.cronHours(0)
.cronDow(0)
.cronDom(0)
.build());
alert_resource = splight.Alert("alertResource",
operator="string",
alert_items=[splight.AlertAlertItemArgs(
expression="string",
expression_plain="string",
query_filter_asset=splight.AlertAlertItemQueryFilterAssetArgs(
id="string",
name="string",
),
query_filter_attribute=splight.AlertAlertItemQueryFilterAttributeArgs(
id="string",
name="string",
),
query_group_function="string",
query_group_unit="string",
query_plain="string",
ref_id="string",
type="string",
id="string",
)],
type="string",
time_window=0,
thresholds=[splight.AlertThresholdArgs(
status="string",
value=0,
status_text="string",
)],
aggregation="string",
target_variable="string",
severity="string",
description="string",
cron_minutes=0,
name="string",
rate_unit="string",
rate_value=0,
related_assets=[splight.AlertRelatedAssetArgs(
id="string",
name="string",
)],
cron_year=0,
tags=[splight.AlertTagArgs(
id="string",
name="string",
)],
cron_month=0,
cron_hours=0,
cron_dow=0,
cron_dom=0)
const alertResource = new splight.Alert("alertResource", {
operator: "string",
alertItems: [{
expression: "string",
expressionPlain: "string",
queryFilterAsset: {
id: "string",
name: "string",
},
queryFilterAttribute: {
id: "string",
name: "string",
},
queryGroupFunction: "string",
queryGroupUnit: "string",
queryPlain: "string",
refId: "string",
type: "string",
id: "string",
}],
type: "string",
timeWindow: 0,
thresholds: [{
status: "string",
value: 0,
statusText: "string",
}],
aggregation: "string",
targetVariable: "string",
severity: "string",
description: "string",
cronMinutes: 0,
name: "string",
rateUnit: "string",
rateValue: 0,
relatedAssets: [{
id: "string",
name: "string",
}],
cronYear: 0,
tags: [{
id: "string",
name: "string",
}],
cronMonth: 0,
cronHours: 0,
cronDow: 0,
cronDom: 0,
});
type: splight:Alert
properties:
aggregation: string
alertItems:
- expression: string
expressionPlain: string
id: string
queryFilterAsset:
id: string
name: string
queryFilterAttribute:
id: string
name: string
queryGroupFunction: string
queryGroupUnit: string
queryPlain: string
refId: string
type: string
cronDom: 0
cronDow: 0
cronHours: 0
cronMinutes: 0
cronMonth: 0
cronYear: 0
description: string
name: string
operator: string
rateUnit: string
rateValue: 0
relatedAssets:
- id: string
name: string
severity: string
tags:
- id: string
name: string
targetVariable: string
thresholds:
- status: string
statusText: string
value: 0
timeWindow: 0
type: string
Alert Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The Alert resource accepts the following input properties:
- Aggregation string
- aggregation to be applied to reads before comparisson
- Alert
Items List<Splight.Splight. Inputs. Alert Alert Item> - traces to be used to compute the results
- Description string
- The description of the resource
- Operator string
- operator to be used to compare the read value with the threshold value
- Severity string
- [sev1,...,sev8] severity for the alert
- Target
Variable string - variable to be used to compare with thresholds
- Thresholds
List<Splight.
Splight. Inputs. Alert Threshold> - Time
Window int - window to fetch data from. Data out of that window will not be considered for evaluation
- Type string
- [cron|rate] type for the cron
- Cron
Dom int - schedule value for cron
- Cron
Dow int - schedule value for cron
- Cron
Hours int - schedule value for cron
- Cron
Minutes int - schedule value for cron
- Cron
Month int - schedule value for cron
- Cron
Year int - schedule value for cron
- Name string
- The name of the resource
- Rate
Unit string - [day|hour|minute] schedule unit
- Rate
Value int - schedule value
- List<Splight.
Splight. Inputs. Alert Related Asset> - related assets of the resource
- List<Splight.
Splight. Inputs. Alert Tag> - tags of the resource
- Aggregation string
- aggregation to be applied to reads before comparisson
- Alert
Items []AlertAlert Item Args - traces to be used to compute the results
- Description string
- The description of the resource
- Operator string
- operator to be used to compare the read value with the threshold value
- Severity string
- [sev1,...,sev8] severity for the alert
- Target
Variable string - variable to be used to compare with thresholds
- Thresholds
[]Alert
Threshold Args - Time
Window int - window to fetch data from. Data out of that window will not be considered for evaluation
- Type string
- [cron|rate] type for the cron
- Cron
Dom int - schedule value for cron
- Cron
Dow int - schedule value for cron
- Cron
Hours int - schedule value for cron
- Cron
Minutes int - schedule value for cron
- Cron
Month int - schedule value for cron
- Cron
Year int - schedule value for cron
- Name string
- The name of the resource
- Rate
Unit string - [day|hour|minute] schedule unit
- Rate
Value int - schedule value
- []Alert
Related Asset Args - related assets of the resource
- []Alert
Tag Args - tags of the resource
- aggregation String
- aggregation to be applied to reads before comparisson
- alert
Items List<AlertAlert Item> - traces to be used to compute the results
- description String
- The description of the resource
- operator String
- operator to be used to compare the read value with the threshold value
- severity String
- [sev1,...,sev8] severity for the alert
- target
Variable String - variable to be used to compare with thresholds
- thresholds
List<Alert
Threshold> - time
Window Integer - window to fetch data from. Data out of that window will not be considered for evaluation
- type String
- [cron|rate] type for the cron
- cron
Dom Integer - schedule value for cron
- cron
Dow Integer - schedule value for cron
- cron
Hours Integer - schedule value for cron
- cron
Minutes Integer - schedule value for cron
- cron
Month Integer - schedule value for cron
- cron
Year Integer - schedule value for cron
- name String
- The name of the resource
- rate
Unit String - [day|hour|minute] schedule unit
- rate
Value Integer - schedule value
- List<Alert
Related Asset> - related assets of the resource
- List<Alert
Tag> - tags of the resource
- aggregation string
- aggregation to be applied to reads before comparisson
- alert
Items AlertAlert Item[] - traces to be used to compute the results
- description string
- The description of the resource
- operator string
- operator to be used to compare the read value with the threshold value
- severity string
- [sev1,...,sev8] severity for the alert
- target
Variable string - variable to be used to compare with thresholds
- thresholds
Alert
Threshold[] - time
Window number - window to fetch data from. Data out of that window will not be considered for evaluation
- type string
- [cron|rate] type for the cron
- cron
Dom number - schedule value for cron
- cron
Dow number - schedule value for cron
- cron
Hours number - schedule value for cron
- cron
Minutes number - schedule value for cron
- cron
Month number - schedule value for cron
- cron
Year number - schedule value for cron
- name string
- The name of the resource
- rate
Unit string - [day|hour|minute] schedule unit
- rate
Value number - schedule value
- Alert
Related Asset[] - related assets of the resource
- Alert
Tag[] - tags of the resource
- aggregation str
- aggregation to be applied to reads before comparisson
- alert_
items Sequence[AlertAlert Item Args] - traces to be used to compute the results
- description str
- The description of the resource
- operator str
- operator to be used to compare the read value with the threshold value
- severity str
- [sev1,...,sev8] severity for the alert
- target_
variable str - variable to be used to compare with thresholds
- thresholds
Sequence[Alert
Threshold Args] - time_
window int - window to fetch data from. Data out of that window will not be considered for evaluation
- type str
- [cron|rate] type for the cron
- cron_
dom int - schedule value for cron
- cron_
dow int - schedule value for cron
- cron_
hours int - schedule value for cron
- cron_
minutes int - schedule value for cron
- cron_
month int - schedule value for cron
- cron_
year int - schedule value for cron
- name str
- The name of the resource
- rate_
unit str - [day|hour|minute] schedule unit
- rate_
value int - schedule value
- Sequence[Alert
Related Asset Args] - related assets of the resource
- Sequence[Alert
Tag Args] - tags of the resource
- aggregation String
- aggregation to be applied to reads before comparisson
- alert
Items List<Property Map> - traces to be used to compute the results
- description String
- The description of the resource
- operator String
- operator to be used to compare the read value with the threshold value
- severity String
- [sev1,...,sev8] severity for the alert
- target
Variable String - variable to be used to compare with thresholds
- thresholds List<Property Map>
- time
Window Number - window to fetch data from. Data out of that window will not be considered for evaluation
- type String
- [cron|rate] type for the cron
- cron
Dom Number - schedule value for cron
- cron
Dow Number - schedule value for cron
- cron
Hours Number - schedule value for cron
- cron
Minutes Number - schedule value for cron
- cron
Month Number - schedule value for cron
- cron
Year Number - schedule value for cron
- name String
- The name of the resource
- rate
Unit String - [day|hour|minute] schedule unit
- rate
Value Number - schedule value
- List<Property Map>
- related assets of the resource
- List<Property Map>
- tags of the resource
Outputs
All input properties are implicitly available as output properties. Additionally, the Alert resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing Alert Resource
Get an existing Alert resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: AlertState, opts?: CustomResourceOptions): Alert
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
aggregation: Optional[str] = None,
alert_items: Optional[Sequence[AlertAlertItemArgs]] = None,
cron_dom: Optional[int] = None,
cron_dow: Optional[int] = None,
cron_hours: Optional[int] = None,
cron_minutes: Optional[int] = None,
cron_month: Optional[int] = None,
cron_year: Optional[int] = None,
description: Optional[str] = None,
name: Optional[str] = None,
operator: Optional[str] = None,
rate_unit: Optional[str] = None,
rate_value: Optional[int] = None,
related_assets: Optional[Sequence[AlertRelatedAssetArgs]] = None,
severity: Optional[str] = None,
tags: Optional[Sequence[AlertTagArgs]] = None,
target_variable: Optional[str] = None,
thresholds: Optional[Sequence[AlertThresholdArgs]] = None,
time_window: Optional[int] = None,
type: Optional[str] = None) -> Alert
func GetAlert(ctx *Context, name string, id IDInput, state *AlertState, opts ...ResourceOption) (*Alert, error)
public static Alert Get(string name, Input<string> id, AlertState? state, CustomResourceOptions? opts = null)
public static Alert get(String name, Output<String> id, AlertState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Aggregation string
- aggregation to be applied to reads before comparisson
- Alert
Items List<Splight.Splight. Inputs. Alert Alert Item> - traces to be used to compute the results
- Cron
Dom int - schedule value for cron
- Cron
Dow int - schedule value for cron
- Cron
Hours int - schedule value for cron
- Cron
Minutes int - schedule value for cron
- Cron
Month int - schedule value for cron
- Cron
Year int - schedule value for cron
- Description string
- The description of the resource
- Name string
- The name of the resource
- Operator string
- operator to be used to compare the read value with the threshold value
- Rate
Unit string - [day|hour|minute] schedule unit
- Rate
Value int - schedule value
- List<Splight.
Splight. Inputs. Alert Related Asset> - related assets of the resource
- Severity string
- [sev1,...,sev8] severity for the alert
- List<Splight.
Splight. Inputs. Alert Tag> - tags of the resource
- Target
Variable string - variable to be used to compare with thresholds
- Thresholds
List<Splight.
Splight. Inputs. Alert Threshold> - Time
Window int - window to fetch data from. Data out of that window will not be considered for evaluation
- Type string
- [cron|rate] type for the cron
- Aggregation string
- aggregation to be applied to reads before comparisson
- Alert
Items []AlertAlert Item Args - traces to be used to compute the results
- Cron
Dom int - schedule value for cron
- Cron
Dow int - schedule value for cron
- Cron
Hours int - schedule value for cron
- Cron
Minutes int - schedule value for cron
- Cron
Month int - schedule value for cron
- Cron
Year int - schedule value for cron
- Description string
- The description of the resource
- Name string
- The name of the resource
- Operator string
- operator to be used to compare the read value with the threshold value
- Rate
Unit string - [day|hour|minute] schedule unit
- Rate
Value int - schedule value
- []Alert
Related Asset Args - related assets of the resource
- Severity string
- [sev1,...,sev8] severity for the alert
- []Alert
Tag Args - tags of the resource
- Target
Variable string - variable to be used to compare with thresholds
- Thresholds
[]Alert
Threshold Args - Time
Window int - window to fetch data from. Data out of that window will not be considered for evaluation
- Type string
- [cron|rate] type for the cron
- aggregation String
- aggregation to be applied to reads before comparisson
- alert
Items List<AlertAlert Item> - traces to be used to compute the results
- cron
Dom Integer - schedule value for cron
- cron
Dow Integer - schedule value for cron
- cron
Hours Integer - schedule value for cron
- cron
Minutes Integer - schedule value for cron
- cron
Month Integer - schedule value for cron
- cron
Year Integer - schedule value for cron
- description String
- The description of the resource
- name String
- The name of the resource
- operator String
- operator to be used to compare the read value with the threshold value
- rate
Unit String - [day|hour|minute] schedule unit
- rate
Value Integer - schedule value
- List<Alert
Related Asset> - related assets of the resource
- severity String
- [sev1,...,sev8] severity for the alert
- List<Alert
Tag> - tags of the resource
- target
Variable String - variable to be used to compare with thresholds
- thresholds
List<Alert
Threshold> - time
Window Integer - window to fetch data from. Data out of that window will not be considered for evaluation
- type String
- [cron|rate] type for the cron
- aggregation string
- aggregation to be applied to reads before comparisson
- alert
Items AlertAlert Item[] - traces to be used to compute the results
- cron
Dom number - schedule value for cron
- cron
Dow number - schedule value for cron
- cron
Hours number - schedule value for cron
- cron
Minutes number - schedule value for cron
- cron
Month number - schedule value for cron
- cron
Year number - schedule value for cron
- description string
- The description of the resource
- name string
- The name of the resource
- operator string
- operator to be used to compare the read value with the threshold value
- rate
Unit string - [day|hour|minute] schedule unit
- rate
Value number - schedule value
- Alert
Related Asset[] - related assets of the resource
- severity string
- [sev1,...,sev8] severity for the alert
- Alert
Tag[] - tags of the resource
- target
Variable string - variable to be used to compare with thresholds
- thresholds
Alert
Threshold[] - time
Window number - window to fetch data from. Data out of that window will not be considered for evaluation
- type string
- [cron|rate] type for the cron
- aggregation str
- aggregation to be applied to reads before comparisson
- alert_
items Sequence[AlertAlert Item Args] - traces to be used to compute the results
- cron_
dom int - schedule value for cron
- cron_
dow int - schedule value for cron
- cron_
hours int - schedule value for cron
- cron_
minutes int - schedule value for cron
- cron_
month int - schedule value for cron
- cron_
year int - schedule value for cron
- description str
- The description of the resource
- name str
- The name of the resource
- operator str
- operator to be used to compare the read value with the threshold value
- rate_
unit str - [day|hour|minute] schedule unit
- rate_
value int - schedule value
- Sequence[Alert
Related Asset Args] - related assets of the resource
- severity str
- [sev1,...,sev8] severity for the alert
- Sequence[Alert
Tag Args] - tags of the resource
- target_
variable str - variable to be used to compare with thresholds
- thresholds
Sequence[Alert
Threshold Args] - time_
window int - window to fetch data from. Data out of that window will not be considered for evaluation
- type str
- [cron|rate] type for the cron
- aggregation String
- aggregation to be applied to reads before comparisson
- alert
Items List<Property Map> - traces to be used to compute the results
- cron
Dom Number - schedule value for cron
- cron
Dow Number - schedule value for cron
- cron
Hours Number - schedule value for cron
- cron
Minutes Number - schedule value for cron
- cron
Month Number - schedule value for cron
- cron
Year Number - schedule value for cron
- description String
- The description of the resource
- name String
- The name of the resource
- operator String
- operator to be used to compare the read value with the threshold value
- rate
Unit String - [day|hour|minute] schedule unit
- rate
Value Number - schedule value
- List<Property Map>
- related assets of the resource
- severity String
- [sev1,...,sev8] severity for the alert
- List<Property Map>
- tags of the resource
- target
Variable String - variable to be used to compare with thresholds
- thresholds List<Property Map>
- time
Window Number - window to fetch data from. Data out of that window will not be considered for evaluation
- type String
- [cron|rate] type for the cron
Supporting Types
AlertAlertItem, AlertAlertItemArgs
- Expression string
- how the expression is shown (i.e 'A * 2')
- Expression
Plain string - actual mongo query containing the expression
- Query
Filter Splight.Asset Splight. Inputs. Alert Alert Item Query Filter Asset - Asset filter
- Query
Filter Splight.Attribute Splight. Inputs. Alert Alert Item Query Filter Attribute - Attribute filter
- Query
Group stringFunction - function used to aggregate data
- Query
Group stringUnit - time window to apply the aggregation
- Query
Plain string - actual mongo query
- Ref
Id string - identifier of the variable (i.e 'A')
- Type string
- either QUERY or EXPRESSION
- Id string
- Id of the function item
- Expression string
- how the expression is shown (i.e 'A * 2')
- Expression
Plain string - actual mongo query containing the expression
- Query
Filter AlertAsset Alert Item Query Filter Asset - Asset filter
- Query
Filter AlertAttribute Alert Item Query Filter Attribute - Attribute filter
- Query
Group stringFunction - function used to aggregate data
- Query
Group stringUnit - time window to apply the aggregation
- Query
Plain string - actual mongo query
- Ref
Id string - identifier of the variable (i.e 'A')
- Type string
- either QUERY or EXPRESSION
- Id string
- Id of the function item
- expression String
- how the expression is shown (i.e 'A * 2')
- expression
Plain String - actual mongo query containing the expression
- query
Filter AlertAsset Alert Item Query Filter Asset - Asset filter
- query
Filter AlertAttribute Alert Item Query Filter Attribute - Attribute filter
- query
Group StringFunction - function used to aggregate data
- query
Group StringUnit - time window to apply the aggregation
- query
Plain String - actual mongo query
- ref
Id String - identifier of the variable (i.e 'A')
- type String
- either QUERY or EXPRESSION
- id String
- Id of the function item
- expression string
- how the expression is shown (i.e 'A * 2')
- expression
Plain string - actual mongo query containing the expression
- query
Filter AlertAsset Alert Item Query Filter Asset - Asset filter
- query
Filter AlertAttribute Alert Item Query Filter Attribute - Attribute filter
- query
Group stringFunction - function used to aggregate data
- query
Group stringUnit - time window to apply the aggregation
- query
Plain string - actual mongo query
- ref
Id string - identifier of the variable (i.e 'A')
- type string
- either QUERY or EXPRESSION
- id string
- Id of the function item
- expression str
- how the expression is shown (i.e 'A * 2')
- expression_
plain str - actual mongo query containing the expression
- query_
filter_ Alertasset Alert Item Query Filter Asset - Asset filter
- query_
filter_ Alertattribute Alert Item Query Filter Attribute - Attribute filter
- query_
group_ strfunction - function used to aggregate data
- query_
group_ strunit - time window to apply the aggregation
- query_
plain str - actual mongo query
- ref_
id str - identifier of the variable (i.e 'A')
- type str
- either QUERY or EXPRESSION
- id str
- Id of the function item
- expression String
- how the expression is shown (i.e 'A * 2')
- expression
Plain String - actual mongo query containing the expression
- query
Filter Property MapAsset - Asset filter
- query
Filter Property MapAttribute - Attribute filter
- query
Group StringFunction - function used to aggregate data
- query
Group StringUnit - time window to apply the aggregation
- query
Plain String - actual mongo query
- ref
Id String - identifier of the variable (i.e 'A')
- type String
- either QUERY or EXPRESSION
- id String
- Id of the function item
AlertAlertItemQueryFilterAsset, AlertAlertItemQueryFilterAssetArgs
AlertAlertItemQueryFilterAttribute, AlertAlertItemQueryFilterAttributeArgs
AlertRelatedAsset, AlertRelatedAssetArgs
AlertTag, AlertTagArgs
AlertThreshold, AlertThresholdArgs
- Status string
- [alert|warning|no_alert] status value for the threshold
- Value double
- value to be considered to compare
- Status
Text string - optional custom value to be displayed in the platform.
- Status string
- [alert|warning|no_alert] status value for the threshold
- Value float64
- value to be considered to compare
- Status
Text string - optional custom value to be displayed in the platform.
- status String
- [alert|warning|no_alert] status value for the threshold
- value Double
- value to be considered to compare
- status
Text String - optional custom value to be displayed in the platform.
- status string
- [alert|warning|no_alert] status value for the threshold
- value number
- value to be considered to compare
- status
Text string - optional custom value to be displayed in the platform.
- status str
- [alert|warning|no_alert] status value for the threshold
- value float
- value to be considered to compare
- status_
text str - optional custom value to be displayed in the platform.
- status String
- [alert|warning|no_alert] status value for the threshold
- value Number
- value to be considered to compare
- status
Text String - optional custom value to be displayed in the platform.
Import
$ pulumi import splight:index/alert:Alert [options] splight_alert.<name> <alert_id>
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- splight splightplatform/pulumi-splight
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
splight
Terraform Provider.
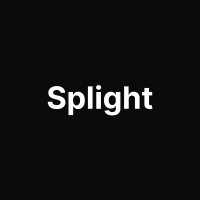