splight.DashboardBarChart
Explore with Pulumi AI
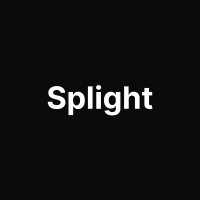
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as splight from "@splightplatform/pulumi-splight";
const assetTest = new splight.Asset("assetTest", {
description: "Created with Terraform",
geometry: JSON.stringify({
type: "GeometryCollection",
geometries: [],
}),
});
const attributeTest1 = new splight.AssetAttribute("attributeTest1", {
type: "Number",
unit: "meters",
asset: assetTest.id,
});
const attributeTest2 = new splight.AssetAttribute("attributeTest2", {
type: "Number",
unit: "seconds",
asset: assetTest.id,
});
const dashboardTest = new splight.Dashboard("dashboardTest", {relatedAssets: []});
const dashboardTabTest = new splight.DashboardTab("dashboardTabTest", {
order: 0,
dashboard: dashboardTest.id,
});
const dashboardChartTest = new splight.DashboardBarChart("dashboardChartTest", {
tab: dashboardTabTest.id,
timestampGte: "now - 7d",
timestampLte: "now",
description: "Chart description",
minHeight: 1,
minWidth: 4,
displayTimeRange: true,
labelsDisplay: true,
labelsAggregation: "last",
labelsPlacement: "bottom",
showBeyondData: true,
height: 10,
width: 20,
collection: "default",
yAxisUnit: "MW",
numberOfDecimals: 2,
orientation: "vertical",
chartItems: [
{
refId: "A",
type: "QUERY",
color: "red",
expressionPlain: "",
queryFilterAsset: {
id: assetTest.id,
name: assetTest.name,
},
queryFilterAttribute: {
id: attributeTest1.id,
name: attributeTest1.name,
},
queryPlain: pulumi.jsonStringify([
{
$match: {
asset: assetTest.id,
attribute: attributeTest1.id,
},
},
{
$addFields: {
timestamp: {
$dateTrunc: {
date: "$timestamp",
unit: "day",
binSize: 1,
},
},
},
},
{
$group: {
_id: "$timestamp",
value: {
$last: "$value",
},
timestamp: {
$last: "$timestamp",
},
},
},
]),
},
{
refId: "B",
color: "blue",
type: "QUERY",
expressionPlain: "",
queryFilterAsset: {
id: assetTest.id,
name: assetTest.name,
},
queryFilterAttribute: {
id: attributeTest2.id,
name: attributeTest2.name,
},
queryPlain: pulumi.jsonStringify([
{
$match: {
asset: assetTest.id,
attribute: attributeTest2.id,
},
},
{
$addFields: {
timestamp: {
$dateTrunc: {
date: "$timestamp",
unit: "hour",
binSize: 1,
},
},
},
},
{
$group: {
_id: "$timestamp",
value: {
$last: "$value",
},
timestamp: {
$last: "$timestamp",
},
},
},
]),
},
],
thresholds: [{
color: "#00edcf",
displayText: "T1Test",
value: 13.1,
}],
valueMappings: [{
displayText: "MODIFICADO",
matchValue: "123.3",
type: "exact_match",
order: 0,
}],
});
import pulumi
import json
import pulumi_splight as splight
asset_test = splight.Asset("assetTest",
description="Created with Terraform",
geometry=json.dumps({
"type": "GeometryCollection",
"geometries": [],
}))
attribute_test1 = splight.AssetAttribute("attributeTest1",
type="Number",
unit="meters",
asset=asset_test.id)
attribute_test2 = splight.AssetAttribute("attributeTest2",
type="Number",
unit="seconds",
asset=asset_test.id)
dashboard_test = splight.Dashboard("dashboardTest", related_assets=[])
dashboard_tab_test = splight.DashboardTab("dashboardTabTest",
order=0,
dashboard=dashboard_test.id)
dashboard_chart_test = splight.DashboardBarChart("dashboardChartTest",
tab=dashboard_tab_test.id,
timestamp_gte="now - 7d",
timestamp_lte="now",
description="Chart description",
min_height=1,
min_width=4,
display_time_range=True,
labels_display=True,
labels_aggregation="last",
labels_placement="bottom",
show_beyond_data=True,
height=10,
width=20,
collection="default",
y_axis_unit="MW",
number_of_decimals=2,
orientation="vertical",
chart_items=[
{
"ref_id": "A",
"type": "QUERY",
"color": "red",
"expression_plain": "",
"query_filter_asset": {
"id": asset_test.id,
"name": asset_test.name,
},
"query_filter_attribute": {
"id": attribute_test1.id,
"name": attribute_test1.name,
},
"query_plain": pulumi.Output.json_dumps([
{
"_match": {
"asset": asset_test.id,
"attribute": attribute_test1.id,
},
},
{
"$addFields": {
"timestamp": {
"$dateTrunc": {
"date": "$timestamp",
"unit": "day",
"binSize": 1,
},
},
},
},
{
"$group": {
"_id": "$timestamp",
"value": {
"$last": "$value",
},
"timestamp": {
"$last": "$timestamp",
},
},
},
]),
},
{
"ref_id": "B",
"color": "blue",
"type": "QUERY",
"expression_plain": "",
"query_filter_asset": {
"id": asset_test.id,
"name": asset_test.name,
},
"query_filter_attribute": {
"id": attribute_test2.id,
"name": attribute_test2.name,
},
"query_plain": pulumi.Output.json_dumps([
{
"_match": {
"asset": asset_test.id,
"attribute": attribute_test2.id,
},
},
{
"$addFields": {
"timestamp": {
"$dateTrunc": {
"date": "$timestamp",
"unit": "hour",
"binSize": 1,
},
},
},
},
{
"$group": {
"_id": "$timestamp",
"value": {
"$last": "$value",
},
"timestamp": {
"$last": "$timestamp",
},
},
},
]),
},
],
thresholds=[{
"color": "#00edcf",
"display_text": "T1Test",
"value": 13.1,
}],
value_mappings=[{
"display_text": "MODIFICADO",
"match_value": "123.3",
"type": "exact_match",
"order": 0,
}])
package main
import (
"encoding/json"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
"github.com/splightplatform/pulumi-splight/sdk/go/splight"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
tmpJSON0, err := json.Marshal(map[string]interface{}{
"type": "GeometryCollection",
"geometries": []interface{}{},
})
if err != nil {
return err
}
json0 := string(tmpJSON0)
assetTest, err := splight.NewAsset(ctx, "assetTest", &splight.AssetArgs{
Description: pulumi.String("Created with Terraform"),
Geometry: pulumi.String(json0),
})
if err != nil {
return err
}
attributeTest1, err := splight.NewAssetAttribute(ctx, "attributeTest1", &splight.AssetAttributeArgs{
Type: pulumi.String("Number"),
Unit: pulumi.String("meters"),
Asset: assetTest.ID(),
})
if err != nil {
return err
}
attributeTest2, err := splight.NewAssetAttribute(ctx, "attributeTest2", &splight.AssetAttributeArgs{
Type: pulumi.String("Number"),
Unit: pulumi.String("seconds"),
Asset: assetTest.ID(),
})
if err != nil {
return err
}
dashboardTest, err := splight.NewDashboard(ctx, "dashboardTest", &splight.DashboardArgs{
RelatedAssets: splight.DashboardRelatedAssetArray{},
})
if err != nil {
return err
}
dashboardTabTest, err := splight.NewDashboardTab(ctx, "dashboardTabTest", &splight.DashboardTabArgs{
Order: pulumi.Int(0),
Dashboard: dashboardTest.ID(),
})
if err != nil {
return err
}
_, err = splight.NewDashboardBarChart(ctx, "dashboardChartTest", &splight.DashboardBarChartArgs{
Tab: dashboardTabTest.ID(),
TimestampGte: pulumi.String("now - 7d"),
TimestampLte: pulumi.String("now"),
Description: pulumi.String("Chart description"),
MinHeight: pulumi.Int(1),
MinWidth: pulumi.Int(4),
DisplayTimeRange: pulumi.Bool(true),
LabelsDisplay: pulumi.Bool(true),
LabelsAggregation: pulumi.String("last"),
LabelsPlacement: pulumi.String("bottom"),
ShowBeyondData: pulumi.Bool(true),
Height: pulumi.Int(10),
Width: pulumi.Int(20),
Collection: pulumi.String("default"),
YAxisUnit: pulumi.String("MW"),
NumberOfDecimals: pulumi.Int(2),
Orientation: pulumi.String("vertical"),
ChartItems: splight.DashboardBarChartChartItemArray{
&splight.DashboardBarChartChartItemArgs{
RefId: pulumi.String("A"),
Type: pulumi.String("QUERY"),
Color: pulumi.String("red"),
ExpressionPlain: pulumi.String(""),
QueryFilterAsset: &splight.DashboardBarChartChartItemQueryFilterAssetArgs{
Id: assetTest.ID(),
Name: assetTest.Name,
},
QueryFilterAttribute: &splight.DashboardBarChartChartItemQueryFilterAttributeArgs{
Id: attributeTest1.ID(),
Name: attributeTest1.Name,
},
QueryPlain: pulumi.All(assetTest.ID(), attributeTest1.ID()).ApplyT(func(_args []interface{}) (string, error) {
assetTestId := _args[0].(string)
attributeTest1Id := _args[1].(string)
var _zero string
tmpJSON1, err := json.Marshal([]interface{}{
map[string]interface{}{
"$match": map[string]interface{}{
"asset": assetTestId,
"attribute": attributeTest1Id,
},
},
map[string]interface{}{
"$addFields": map[string]interface{}{
"timestamp": map[string]interface{}{
"$dateTrunc": map[string]interface{}{
"date": "$timestamp",
"unit": "day",
"binSize": 1,
},
},
},
},
map[string]interface{}{
"$group": map[string]interface{}{
"_id": "$timestamp",
"value": map[string]interface{}{
"$last": "$value",
},
"timestamp": map[string]interface{}{
"$last": "$timestamp",
},
},
},
})
if err != nil {
return _zero, err
}
json1 := string(tmpJSON1)
return json1, nil
}).(pulumi.StringOutput),
},
&splight.DashboardBarChartChartItemArgs{
RefId: pulumi.String("B"),
Color: pulumi.String("blue"),
Type: pulumi.String("QUERY"),
ExpressionPlain: pulumi.String(""),
QueryFilterAsset: &splight.DashboardBarChartChartItemQueryFilterAssetArgs{
Id: assetTest.ID(),
Name: assetTest.Name,
},
QueryFilterAttribute: &splight.DashboardBarChartChartItemQueryFilterAttributeArgs{
Id: attributeTest2.ID(),
Name: attributeTest2.Name,
},
QueryPlain: pulumi.All(assetTest.ID(), attributeTest2.ID()).ApplyT(func(_args []interface{}) (string, error) {
assetTestId := _args[0].(string)
attributeTest2Id := _args[1].(string)
var _zero string
tmpJSON2, err := json.Marshal([]interface{}{
map[string]interface{}{
"$match": map[string]interface{}{
"asset": assetTestId,
"attribute": attributeTest2Id,
},
},
map[string]interface{}{
"$addFields": map[string]interface{}{
"timestamp": map[string]interface{}{
"$dateTrunc": map[string]interface{}{
"date": "$timestamp",
"unit": "hour",
"binSize": 1,
},
},
},
},
map[string]interface{}{
"$group": map[string]interface{}{
"_id": "$timestamp",
"value": map[string]interface{}{
"$last": "$value",
},
"timestamp": map[string]interface{}{
"$last": "$timestamp",
},
},
},
})
if err != nil {
return _zero, err
}
json2 := string(tmpJSON2)
return json2, nil
}).(pulumi.StringOutput),
},
},
Thresholds: splight.DashboardBarChartThresholdArray{
&splight.DashboardBarChartThresholdArgs{
Color: pulumi.String("#00edcf"),
DisplayText: pulumi.String("T1Test"),
Value: pulumi.Float64(13.1),
},
},
ValueMappings: splight.DashboardBarChartValueMappingArray{
&splight.DashboardBarChartValueMappingArgs{
DisplayText: pulumi.String("MODIFICADO"),
MatchValue: pulumi.String("123.3"),
Type: pulumi.String("exact_match"),
Order: pulumi.Int(0),
},
},
})
if err != nil {
return err
}
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using System.Text.Json;
using Pulumi;
using Splight = Splight.Splight;
return await Deployment.RunAsync(() =>
{
var assetTest = new Splight.Asset("assetTest", new()
{
Description = "Created with Terraform",
Geometry = JsonSerializer.Serialize(new Dictionary<string, object?>
{
["type"] = "GeometryCollection",
["geometries"] = new[]
{
},
}),
});
var attributeTest1 = new Splight.AssetAttribute("attributeTest1", new()
{
Type = "Number",
Unit = "meters",
Asset = assetTest.Id,
});
var attributeTest2 = new Splight.AssetAttribute("attributeTest2", new()
{
Type = "Number",
Unit = "seconds",
Asset = assetTest.Id,
});
var dashboardTest = new Splight.Dashboard("dashboardTest", new()
{
RelatedAssets = new[] {},
});
var dashboardTabTest = new Splight.DashboardTab("dashboardTabTest", new()
{
Order = 0,
Dashboard = dashboardTest.Id,
});
var dashboardChartTest = new Splight.DashboardBarChart("dashboardChartTest", new()
{
Tab = dashboardTabTest.Id,
TimestampGte = "now - 7d",
TimestampLte = "now",
Description = "Chart description",
MinHeight = 1,
MinWidth = 4,
DisplayTimeRange = true,
LabelsDisplay = true,
LabelsAggregation = "last",
LabelsPlacement = "bottom",
ShowBeyondData = true,
Height = 10,
Width = 20,
Collection = "default",
YAxisUnit = "MW",
NumberOfDecimals = 2,
Orientation = "vertical",
ChartItems = new[]
{
new Splight.Inputs.DashboardBarChartChartItemArgs
{
RefId = "A",
Type = "QUERY",
Color = "red",
ExpressionPlain = "",
QueryFilterAsset = new Splight.Inputs.DashboardBarChartChartItemQueryFilterAssetArgs
{
Id = assetTest.Id,
Name = assetTest.Name,
},
QueryFilterAttribute = new Splight.Inputs.DashboardBarChartChartItemQueryFilterAttributeArgs
{
Id = attributeTest1.Id,
Name = attributeTest1.Name,
},
QueryPlain = Output.JsonSerialize(Output.Create(new[]
{
new Dictionary<string, object?>
{
["$match"] = new Dictionary<string, object?>
{
["asset"] = assetTest.Id,
["attribute"] = attributeTest1.Id,
},
},
new Dictionary<string, object?>
{
["$addFields"] = new Dictionary<string, object?>
{
["timestamp"] = new Dictionary<string, object?>
{
["$dateTrunc"] = new Dictionary<string, object?>
{
["date"] = "$timestamp",
["unit"] = "day",
["binSize"] = 1,
},
},
},
},
new Dictionary<string, object?>
{
["$group"] = new Dictionary<string, object?>
{
["_id"] = "$timestamp",
["value"] = new Dictionary<string, object?>
{
["$last"] = "$value",
},
["timestamp"] = new Dictionary<string, object?>
{
["$last"] = "$timestamp",
},
},
},
})),
},
new Splight.Inputs.DashboardBarChartChartItemArgs
{
RefId = "B",
Color = "blue",
Type = "QUERY",
ExpressionPlain = "",
QueryFilterAsset = new Splight.Inputs.DashboardBarChartChartItemQueryFilterAssetArgs
{
Id = assetTest.Id,
Name = assetTest.Name,
},
QueryFilterAttribute = new Splight.Inputs.DashboardBarChartChartItemQueryFilterAttributeArgs
{
Id = attributeTest2.Id,
Name = attributeTest2.Name,
},
QueryPlain = Output.JsonSerialize(Output.Create(new[]
{
new Dictionary<string, object?>
{
["$match"] = new Dictionary<string, object?>
{
["asset"] = assetTest.Id,
["attribute"] = attributeTest2.Id,
},
},
new Dictionary<string, object?>
{
["$addFields"] = new Dictionary<string, object?>
{
["timestamp"] = new Dictionary<string, object?>
{
["$dateTrunc"] = new Dictionary<string, object?>
{
["date"] = "$timestamp",
["unit"] = "hour",
["binSize"] = 1,
},
},
},
},
new Dictionary<string, object?>
{
["$group"] = new Dictionary<string, object?>
{
["_id"] = "$timestamp",
["value"] = new Dictionary<string, object?>
{
["$last"] = "$value",
},
["timestamp"] = new Dictionary<string, object?>
{
["$last"] = "$timestamp",
},
},
},
})),
},
},
Thresholds = new[]
{
new Splight.Inputs.DashboardBarChartThresholdArgs
{
Color = "#00edcf",
DisplayText = "T1Test",
Value = 13.1,
},
},
ValueMappings = new[]
{
new Splight.Inputs.DashboardBarChartValueMappingArgs
{
DisplayText = "MODIFICADO",
MatchValue = "123.3",
Type = "exact_match",
Order = 0,
},
},
});
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.splight.Asset;
import com.pulumi.splight.AssetArgs;
import com.pulumi.splight.AssetAttribute;
import com.pulumi.splight.AssetAttributeArgs;
import com.pulumi.splight.Dashboard;
import com.pulumi.splight.DashboardArgs;
import com.pulumi.splight.DashboardTab;
import com.pulumi.splight.DashboardTabArgs;
import com.pulumi.splight.DashboardBarChart;
import com.pulumi.splight.DashboardBarChartArgs;
import com.pulumi.splight.inputs.DashboardBarChartChartItemArgs;
import com.pulumi.splight.inputs.DashboardBarChartChartItemQueryFilterAssetArgs;
import com.pulumi.splight.inputs.DashboardBarChartChartItemQueryFilterAttributeArgs;
import com.pulumi.splight.inputs.DashboardBarChartThresholdArgs;
import com.pulumi.splight.inputs.DashboardBarChartValueMappingArgs;
import static com.pulumi.codegen.internal.Serialization.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var assetTest = new Asset("assetTest", AssetArgs.builder()
.description("Created with Terraform")
.geometry(serializeJson(
jsonObject(
jsonProperty("type", "GeometryCollection"),
jsonProperty("geometries", jsonArray(
))
)))
.build());
var attributeTest1 = new AssetAttribute("attributeTest1", AssetAttributeArgs.builder()
.type("Number")
.unit("meters")
.asset(assetTest.id())
.build());
var attributeTest2 = new AssetAttribute("attributeTest2", AssetAttributeArgs.builder()
.type("Number")
.unit("seconds")
.asset(assetTest.id())
.build());
var dashboardTest = new Dashboard("dashboardTest", DashboardArgs.builder()
.relatedAssets()
.build());
var dashboardTabTest = new DashboardTab("dashboardTabTest", DashboardTabArgs.builder()
.order(0)
.dashboard(dashboardTest.id())
.build());
var dashboardChartTest = new DashboardBarChart("dashboardChartTest", DashboardBarChartArgs.builder()
.tab(dashboardTabTest.id())
.timestampGte("now - 7d")
.timestampLte("now")
.description("Chart description")
.minHeight(1)
.minWidth(4)
.displayTimeRange(true)
.labelsDisplay(true)
.labelsAggregation("last")
.labelsPlacement("bottom")
.showBeyondData(true)
.height(10)
.width(20)
.collection("default")
.yAxisUnit("MW")
.numberOfDecimals(2)
.orientation("vertical")
.chartItems(
DashboardBarChartChartItemArgs.builder()
.refId("A")
.type("QUERY")
.color("red")
.expressionPlain("")
.queryFilterAsset(DashboardBarChartChartItemQueryFilterAssetArgs.builder()
.id(assetTest.id())
.name(assetTest.name())
.build())
.queryFilterAttribute(DashboardBarChartChartItemQueryFilterAttributeArgs.builder()
.id(attributeTest1.id())
.name(attributeTest1.name())
.build())
.queryPlain(Output.tuple(assetTest.id(), attributeTest1.id()).applyValue(values -> {
var assetTestId = values.t1;
var attributeTest1Id = values.t2;
return serializeJson(
jsonArray(
jsonObject(
jsonProperty("$match", jsonObject(
jsonProperty("asset", assetTestId),
jsonProperty("attribute", attributeTest1Id)
))
),
jsonObject(
jsonProperty("$addFields", jsonObject(
jsonProperty("timestamp", jsonObject(
jsonProperty("$dateTrunc", jsonObject(
jsonProperty("date", "$timestamp"),
jsonProperty("unit", "day"),
jsonProperty("binSize", 1)
))
))
))
),
jsonObject(
jsonProperty("$group", jsonObject(
jsonProperty("_id", "$timestamp"),
jsonProperty("value", jsonObject(
jsonProperty("$last", "$value")
)),
jsonProperty("timestamp", jsonObject(
jsonProperty("$last", "$timestamp")
))
))
)
));
}))
.build(),
DashboardBarChartChartItemArgs.builder()
.refId("B")
.color("blue")
.type("QUERY")
.expressionPlain("")
.queryFilterAsset(DashboardBarChartChartItemQueryFilterAssetArgs.builder()
.id(assetTest.id())
.name(assetTest.name())
.build())
.queryFilterAttribute(DashboardBarChartChartItemQueryFilterAttributeArgs.builder()
.id(attributeTest2.id())
.name(attributeTest2.name())
.build())
.queryPlain(Output.tuple(assetTest.id(), attributeTest2.id()).applyValue(values -> {
var assetTestId = values.t1;
var attributeTest2Id = values.t2;
return serializeJson(
jsonArray(
jsonObject(
jsonProperty("$match", jsonObject(
jsonProperty("asset", assetTestId),
jsonProperty("attribute", attributeTest2Id)
))
),
jsonObject(
jsonProperty("$addFields", jsonObject(
jsonProperty("timestamp", jsonObject(
jsonProperty("$dateTrunc", jsonObject(
jsonProperty("date", "$timestamp"),
jsonProperty("unit", "hour"),
jsonProperty("binSize", 1)
))
))
))
),
jsonObject(
jsonProperty("$group", jsonObject(
jsonProperty("_id", "$timestamp"),
jsonProperty("value", jsonObject(
jsonProperty("$last", "$value")
)),
jsonProperty("timestamp", jsonObject(
jsonProperty("$last", "$timestamp")
))
))
)
));
}))
.build())
.thresholds(DashboardBarChartThresholdArgs.builder()
.color("#00edcf")
.displayText("T1Test")
.value(13.1)
.build())
.valueMappings(DashboardBarChartValueMappingArgs.builder()
.displayText("MODIFICADO")
.matchValue("123.3")
.type("exact_match")
.order(0)
.build())
.build());
}
}
resources:
assetTest:
type: splight:Asset
properties:
description: Created with Terraform
geometry:
fn::toJSON:
type: GeometryCollection
geometries: []
attributeTest1:
type: splight:AssetAttribute
properties:
type: Number
unit: meters
asset: ${assetTest.id}
attributeTest2:
type: splight:AssetAttribute
properties:
type: Number
unit: seconds
asset: ${assetTest.id}
dashboardTest:
type: splight:Dashboard
properties:
relatedAssets: []
dashboardTabTest:
type: splight:DashboardTab
properties:
order: 0
dashboard: ${dashboardTest.id}
dashboardChartTest:
type: splight:DashboardBarChart
properties:
tab: ${dashboardTabTest.id}
timestampGte: now - 7d
timestampLte: now
description: Chart description
minHeight: 1
minWidth: 4
displayTimeRange: true
labelsDisplay: true
labelsAggregation: last
labelsPlacement: bottom
showBeyondData: true
height: 10
width: 20
collection: default
yAxisUnit: MW
numberOfDecimals: 2
orientation: vertical
chartItems:
- refId: A
type: QUERY
color: red
expressionPlain:
queryFilterAsset:
id: ${assetTest.id}
name: ${assetTest.name}
queryFilterAttribute:
id: ${attributeTest1.id}
name: ${attributeTest1.name}
queryPlain:
fn::toJSON:
- $match:
asset: ${assetTest.id}
attribute: ${attributeTest1.id}
- $addFields:
timestamp:
$dateTrunc:
date: $timestamp
unit: day
binSize: 1
- $group:
_id: $timestamp
value:
$last: $value
timestamp:
$last: $timestamp
- refId: B
color: blue
type: QUERY
expressionPlain:
queryFilterAsset:
id: ${assetTest.id}
name: ${assetTest.name}
queryFilterAttribute:
id: ${attributeTest2.id}
name: ${attributeTest2.name}
queryPlain:
fn::toJSON:
- $match:
asset: ${assetTest.id}
attribute: ${attributeTest2.id}
- $addFields:
timestamp:
$dateTrunc:
date: $timestamp
unit: hour
binSize: 1
- $group:
_id: $timestamp
value:
$last: $value
timestamp:
$last: $timestamp
thresholds:
- color: '#00edcf'
displayText: T1Test
value: 13.1
valueMappings:
- displayText: MODIFICADO
matchValue: '123.3'
type: exact_match
order: 0
Create DashboardBarChart Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new DashboardBarChart(name: string, args: DashboardBarChartArgs, opts?: CustomResourceOptions);
@overload
def DashboardBarChart(resource_name: str,
args: DashboardBarChartArgs,
opts: Optional[ResourceOptions] = None)
@overload
def DashboardBarChart(resource_name: str,
opts: Optional[ResourceOptions] = None,
chart_items: Optional[Sequence[DashboardBarChartChartItemArgs]] = None,
timestamp_lte: Optional[str] = None,
timestamp_gte: Optional[str] = None,
tab: Optional[str] = None,
labels_display: Optional[bool] = None,
refresh_interval: Optional[str] = None,
height: Optional[int] = None,
labels_placement: Optional[str] = None,
min_height: Optional[int] = None,
min_width: Optional[int] = None,
name: Optional[str] = None,
number_of_decimals: Optional[int] = None,
orientation: Optional[str] = None,
position_x: Optional[int] = None,
position_y: Optional[int] = None,
labels_aggregation: Optional[str] = None,
relative_window_time: Optional[str] = None,
show_beyond_data: Optional[bool] = None,
display_time_range: Optional[bool] = None,
thresholds: Optional[Sequence[DashboardBarChartThresholdArgs]] = None,
description: Optional[str] = None,
collection: Optional[str] = None,
timezone: Optional[str] = None,
value_mappings: Optional[Sequence[DashboardBarChartValueMappingArgs]] = None,
width: Optional[int] = None,
y_axis_unit: Optional[str] = None)
func NewDashboardBarChart(ctx *Context, name string, args DashboardBarChartArgs, opts ...ResourceOption) (*DashboardBarChart, error)
public DashboardBarChart(string name, DashboardBarChartArgs args, CustomResourceOptions? opts = null)
public DashboardBarChart(String name, DashboardBarChartArgs args)
public DashboardBarChart(String name, DashboardBarChartArgs args, CustomResourceOptions options)
type: splight:DashboardBarChart
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args DashboardBarChartArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args DashboardBarChartArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args DashboardBarChartArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args DashboardBarChartArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args DashboardBarChartArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var dashboardBarChartResource = new Splight.DashboardBarChart("dashboardBarChartResource", new()
{
ChartItems = new[]
{
new Splight.Inputs.DashboardBarChartChartItemArgs
{
QueryPlain = "string",
ExpressionPlain = "string",
Type = "string",
Color = "string",
QueryFilterAsset = new Splight.Inputs.DashboardBarChartChartItemQueryFilterAssetArgs
{
Id = "string",
Name = "string",
},
QueryFilterAttribute = new Splight.Inputs.DashboardBarChartChartItemQueryFilterAttributeArgs
{
Id = "string",
Name = "string",
},
RefId = "string",
Label = "string",
QueryLimit = 0,
QueryGroupUnit = "string",
QuerySortDirection = 0,
QueryGroupFunction = "string",
Hidden = false,
},
},
TimestampLte = "string",
TimestampGte = "string",
Tab = "string",
LabelsDisplay = false,
RefreshInterval = "string",
Height = 0,
LabelsPlacement = "string",
MinHeight = 0,
MinWidth = 0,
Name = "string",
NumberOfDecimals = 0,
Orientation = "string",
PositionX = 0,
PositionY = 0,
LabelsAggregation = "string",
RelativeWindowTime = "string",
ShowBeyondData = false,
DisplayTimeRange = false,
Thresholds = new[]
{
new Splight.Inputs.DashboardBarChartThresholdArgs
{
Color = "string",
DisplayText = "string",
Value = 0,
},
},
Description = "string",
Collection = "string",
Timezone = "string",
ValueMappings = new[]
{
new Splight.Inputs.DashboardBarChartValueMappingArgs
{
DisplayText = "string",
MatchValue = "string",
Order = 0,
Type = "string",
},
},
Width = 0,
YAxisUnit = "string",
});
example, err := splight.NewDashboardBarChart(ctx, "dashboardBarChartResource", &splight.DashboardBarChartArgs{
ChartItems: splight.DashboardBarChartChartItemArray{
&splight.DashboardBarChartChartItemArgs{
QueryPlain: pulumi.String("string"),
ExpressionPlain: pulumi.String("string"),
Type: pulumi.String("string"),
Color: pulumi.String("string"),
QueryFilterAsset: &splight.DashboardBarChartChartItemQueryFilterAssetArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
QueryFilterAttribute: &splight.DashboardBarChartChartItemQueryFilterAttributeArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
RefId: pulumi.String("string"),
Label: pulumi.String("string"),
QueryLimit: pulumi.Int(0),
QueryGroupUnit: pulumi.String("string"),
QuerySortDirection: pulumi.Int(0),
QueryGroupFunction: pulumi.String("string"),
Hidden: pulumi.Bool(false),
},
},
TimestampLte: pulumi.String("string"),
TimestampGte: pulumi.String("string"),
Tab: pulumi.String("string"),
LabelsDisplay: pulumi.Bool(false),
RefreshInterval: pulumi.String("string"),
Height: pulumi.Int(0),
LabelsPlacement: pulumi.String("string"),
MinHeight: pulumi.Int(0),
MinWidth: pulumi.Int(0),
Name: pulumi.String("string"),
NumberOfDecimals: pulumi.Int(0),
Orientation: pulumi.String("string"),
PositionX: pulumi.Int(0),
PositionY: pulumi.Int(0),
LabelsAggregation: pulumi.String("string"),
RelativeWindowTime: pulumi.String("string"),
ShowBeyondData: pulumi.Bool(false),
DisplayTimeRange: pulumi.Bool(false),
Thresholds: splight.DashboardBarChartThresholdArray{
&splight.DashboardBarChartThresholdArgs{
Color: pulumi.String("string"),
DisplayText: pulumi.String("string"),
Value: pulumi.Float64(0),
},
},
Description: pulumi.String("string"),
Collection: pulumi.String("string"),
Timezone: pulumi.String("string"),
ValueMappings: splight.DashboardBarChartValueMappingArray{
&splight.DashboardBarChartValueMappingArgs{
DisplayText: pulumi.String("string"),
MatchValue: pulumi.String("string"),
Order: pulumi.Int(0),
Type: pulumi.String("string"),
},
},
Width: pulumi.Int(0),
YAxisUnit: pulumi.String("string"),
})
var dashboardBarChartResource = new DashboardBarChart("dashboardBarChartResource", DashboardBarChartArgs.builder()
.chartItems(DashboardBarChartChartItemArgs.builder()
.queryPlain("string")
.expressionPlain("string")
.type("string")
.color("string")
.queryFilterAsset(DashboardBarChartChartItemQueryFilterAssetArgs.builder()
.id("string")
.name("string")
.build())
.queryFilterAttribute(DashboardBarChartChartItemQueryFilterAttributeArgs.builder()
.id("string")
.name("string")
.build())
.refId("string")
.label("string")
.queryLimit(0)
.queryGroupUnit("string")
.querySortDirection(0)
.queryGroupFunction("string")
.hidden(false)
.build())
.timestampLte("string")
.timestampGte("string")
.tab("string")
.labelsDisplay(false)
.refreshInterval("string")
.height(0)
.labelsPlacement("string")
.minHeight(0)
.minWidth(0)
.name("string")
.numberOfDecimals(0)
.orientation("string")
.positionX(0)
.positionY(0)
.labelsAggregation("string")
.relativeWindowTime("string")
.showBeyondData(false)
.displayTimeRange(false)
.thresholds(DashboardBarChartThresholdArgs.builder()
.color("string")
.displayText("string")
.value(0)
.build())
.description("string")
.collection("string")
.timezone("string")
.valueMappings(DashboardBarChartValueMappingArgs.builder()
.displayText("string")
.matchValue("string")
.order(0)
.type("string")
.build())
.width(0)
.yAxisUnit("string")
.build());
dashboard_bar_chart_resource = splight.DashboardBarChart("dashboardBarChartResource",
chart_items=[splight.DashboardBarChartChartItemArgs(
query_plain="string",
expression_plain="string",
type="string",
color="string",
query_filter_asset=splight.DashboardBarChartChartItemQueryFilterAssetArgs(
id="string",
name="string",
),
query_filter_attribute=splight.DashboardBarChartChartItemQueryFilterAttributeArgs(
id="string",
name="string",
),
ref_id="string",
label="string",
query_limit=0,
query_group_unit="string",
query_sort_direction=0,
query_group_function="string",
hidden=False,
)],
timestamp_lte="string",
timestamp_gte="string",
tab="string",
labels_display=False,
refresh_interval="string",
height=0,
labels_placement="string",
min_height=0,
min_width=0,
name="string",
number_of_decimals=0,
orientation="string",
position_x=0,
position_y=0,
labels_aggregation="string",
relative_window_time="string",
show_beyond_data=False,
display_time_range=False,
thresholds=[splight.DashboardBarChartThresholdArgs(
color="string",
display_text="string",
value=0,
)],
description="string",
collection="string",
timezone="string",
value_mappings=[splight.DashboardBarChartValueMappingArgs(
display_text="string",
match_value="string",
order=0,
type="string",
)],
width=0,
y_axis_unit="string")
const dashboardBarChartResource = new splight.DashboardBarChart("dashboardBarChartResource", {
chartItems: [{
queryPlain: "string",
expressionPlain: "string",
type: "string",
color: "string",
queryFilterAsset: {
id: "string",
name: "string",
},
queryFilterAttribute: {
id: "string",
name: "string",
},
refId: "string",
label: "string",
queryLimit: 0,
queryGroupUnit: "string",
querySortDirection: 0,
queryGroupFunction: "string",
hidden: false,
}],
timestampLte: "string",
timestampGte: "string",
tab: "string",
labelsDisplay: false,
refreshInterval: "string",
height: 0,
labelsPlacement: "string",
minHeight: 0,
minWidth: 0,
name: "string",
numberOfDecimals: 0,
orientation: "string",
positionX: 0,
positionY: 0,
labelsAggregation: "string",
relativeWindowTime: "string",
showBeyondData: false,
displayTimeRange: false,
thresholds: [{
color: "string",
displayText: "string",
value: 0,
}],
description: "string",
collection: "string",
timezone: "string",
valueMappings: [{
displayText: "string",
matchValue: "string",
order: 0,
type: "string",
}],
width: 0,
yAxisUnit: "string",
});
type: splight:DashboardBarChart
properties:
chartItems:
- color: string
expressionPlain: string
hidden: false
label: string
queryFilterAsset:
id: string
name: string
queryFilterAttribute:
id: string
name: string
queryGroupFunction: string
queryGroupUnit: string
queryLimit: 0
queryPlain: string
querySortDirection: 0
refId: string
type: string
collection: string
description: string
displayTimeRange: false
height: 0
labelsAggregation: string
labelsDisplay: false
labelsPlacement: string
minHeight: 0
minWidth: 0
name: string
numberOfDecimals: 0
orientation: string
positionX: 0
positionY: 0
refreshInterval: string
relativeWindowTime: string
showBeyondData: false
tab: string
thresholds:
- color: string
displayText: string
value: 0
timestampGte: string
timestampLte: string
timezone: string
valueMappings:
- displayText: string
matchValue: string
order: 0
type: string
width: 0
yAxisUnit: string
DashboardBarChart Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The DashboardBarChart resource accepts the following input properties:
- Chart
Items List<Splight.Splight. Inputs. Dashboard Bar Chart Chart Item> - chart traces to be included
- Tab string
- id for the tab where to place the chart
- Timestamp
Gte string - date in isoformat or shortcut string where to end reading
- Timestamp
Lte string - date in isoformat or shortcut string where to start reading
- Collection string
- Description string
- chart description
- Display
Time boolRange - whether to display the time range or not
- Height int
- chart height in px
- Labels
Aggregation string - [last|avg|...] aggregation
- Labels
Display bool - whether to display the labels or not
- Labels
Placement string - [right|bottom] placement
- Min
Height int - minimum chart height
- Min
Width int - minimum chart width
- Name string
- name of the chart
- Number
Of intDecimals - number of decimals
- Orientation string
- orientation
- Position
X int - chart x position
- Position
Y int - chart y position
- Refresh
Interval string - refresh interval
- Relative
Window stringTime - relative window time
- Show
Beyond boolData - whether to show data which is beyond timestamp_lte or not
- Thresholds
List<Splight.
Splight. Inputs. Dashboard Bar Chart Threshold> - optional static lines to be added to the chart as references
- Timezone string
- chart timezone
- Value
Mappings List<Splight.Splight. Inputs. Dashboard Bar Chart Value Mapping> - optional mappings to transform data with rules
- Width int
- chart width in cols (max 20)
- YAxis
Unit string - y axis units
- Chart
Items []DashboardBar Chart Chart Item Args - chart traces to be included
- Tab string
- id for the tab where to place the chart
- Timestamp
Gte string - date in isoformat or shortcut string where to end reading
- Timestamp
Lte string - date in isoformat or shortcut string where to start reading
- Collection string
- Description string
- chart description
- Display
Time boolRange - whether to display the time range or not
- Height int
- chart height in px
- Labels
Aggregation string - [last|avg|...] aggregation
- Labels
Display bool - whether to display the labels or not
- Labels
Placement string - [right|bottom] placement
- Min
Height int - minimum chart height
- Min
Width int - minimum chart width
- Name string
- name of the chart
- Number
Of intDecimals - number of decimals
- Orientation string
- orientation
- Position
X int - chart x position
- Position
Y int - chart y position
- Refresh
Interval string - refresh interval
- Relative
Window stringTime - relative window time
- Show
Beyond boolData - whether to show data which is beyond timestamp_lte or not
- Thresholds
[]Dashboard
Bar Chart Threshold Args - optional static lines to be added to the chart as references
- Timezone string
- chart timezone
- Value
Mappings []DashboardBar Chart Value Mapping Args - optional mappings to transform data with rules
- Width int
- chart width in cols (max 20)
- YAxis
Unit string - y axis units
- chart
Items List<DashboardBar Chart Chart Item> - chart traces to be included
- tab String
- id for the tab where to place the chart
- timestamp
Gte String - date in isoformat or shortcut string where to end reading
- timestamp
Lte String - date in isoformat or shortcut string where to start reading
- collection String
- description String
- chart description
- display
Time BooleanRange - whether to display the time range or not
- height Integer
- chart height in px
- labels
Aggregation String - [last|avg|...] aggregation
- labels
Display Boolean - whether to display the labels or not
- labels
Placement String - [right|bottom] placement
- min
Height Integer - minimum chart height
- min
Width Integer - minimum chart width
- name String
- name of the chart
- number
Of IntegerDecimals - number of decimals
- orientation String
- orientation
- position
X Integer - chart x position
- position
Y Integer - chart y position
- refresh
Interval String - refresh interval
- relative
Window StringTime - relative window time
- show
Beyond BooleanData - whether to show data which is beyond timestamp_lte or not
- thresholds
List<Dashboard
Bar Chart Threshold> - optional static lines to be added to the chart as references
- timezone String
- chart timezone
- value
Mappings List<DashboardBar Chart Value Mapping> - optional mappings to transform data with rules
- width Integer
- chart width in cols (max 20)
- y
Axis StringUnit - y axis units
- chart
Items DashboardBar Chart Chart Item[] - chart traces to be included
- tab string
- id for the tab where to place the chart
- timestamp
Gte string - date in isoformat or shortcut string where to end reading
- timestamp
Lte string - date in isoformat or shortcut string where to start reading
- collection string
- description string
- chart description
- display
Time booleanRange - whether to display the time range or not
- height number
- chart height in px
- labels
Aggregation string - [last|avg|...] aggregation
- labels
Display boolean - whether to display the labels or not
- labels
Placement string - [right|bottom] placement
- min
Height number - minimum chart height
- min
Width number - minimum chart width
- name string
- name of the chart
- number
Of numberDecimals - number of decimals
- orientation string
- orientation
- position
X number - chart x position
- position
Y number - chart y position
- refresh
Interval string - refresh interval
- relative
Window stringTime - relative window time
- show
Beyond booleanData - whether to show data which is beyond timestamp_lte or not
- thresholds
Dashboard
Bar Chart Threshold[] - optional static lines to be added to the chart as references
- timezone string
- chart timezone
- value
Mappings DashboardBar Chart Value Mapping[] - optional mappings to transform data with rules
- width number
- chart width in cols (max 20)
- y
Axis stringUnit - y axis units
- chart_
items Sequence[DashboardBar Chart Chart Item Args] - chart traces to be included
- tab str
- id for the tab where to place the chart
- timestamp_
gte str - date in isoformat or shortcut string where to end reading
- timestamp_
lte str - date in isoformat or shortcut string where to start reading
- collection str
- description str
- chart description
- display_
time_ boolrange - whether to display the time range or not
- height int
- chart height in px
- labels_
aggregation str - [last|avg|...] aggregation
- labels_
display bool - whether to display the labels or not
- labels_
placement str - [right|bottom] placement
- min_
height int - minimum chart height
- min_
width int - minimum chart width
- name str
- name of the chart
- number_
of_ intdecimals - number of decimals
- orientation str
- orientation
- position_
x int - chart x position
- position_
y int - chart y position
- refresh_
interval str - refresh interval
- relative_
window_ strtime - relative window time
- show_
beyond_ booldata - whether to show data which is beyond timestamp_lte or not
- thresholds
Sequence[Dashboard
Bar Chart Threshold Args] - optional static lines to be added to the chart as references
- timezone str
- chart timezone
- value_
mappings Sequence[DashboardBar Chart Value Mapping Args] - optional mappings to transform data with rules
- width int
- chart width in cols (max 20)
- y_
axis_ strunit - y axis units
- chart
Items List<Property Map> - chart traces to be included
- tab String
- id for the tab where to place the chart
- timestamp
Gte String - date in isoformat or shortcut string where to end reading
- timestamp
Lte String - date in isoformat or shortcut string where to start reading
- collection String
- description String
- chart description
- display
Time BooleanRange - whether to display the time range or not
- height Number
- chart height in px
- labels
Aggregation String - [last|avg|...] aggregation
- labels
Display Boolean - whether to display the labels or not
- labels
Placement String - [right|bottom] placement
- min
Height Number - minimum chart height
- min
Width Number - minimum chart width
- name String
- name of the chart
- number
Of NumberDecimals - number of decimals
- orientation String
- orientation
- position
X Number - chart x position
- position
Y Number - chart y position
- refresh
Interval String - refresh interval
- relative
Window StringTime - relative window time
- show
Beyond BooleanData - whether to show data which is beyond timestamp_lte or not
- thresholds List<Property Map>
- optional static lines to be added to the chart as references
- timezone String
- chart timezone
- value
Mappings List<Property Map> - optional mappings to transform data with rules
- width Number
- chart width in cols (max 20)
- y
Axis StringUnit - y axis units
Outputs
All input properties are implicitly available as output properties. Additionally, the DashboardBarChart resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing DashboardBarChart Resource
Get an existing DashboardBarChart resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: DashboardBarChartState, opts?: CustomResourceOptions): DashboardBarChart
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
chart_items: Optional[Sequence[DashboardBarChartChartItemArgs]] = None,
collection: Optional[str] = None,
description: Optional[str] = None,
display_time_range: Optional[bool] = None,
height: Optional[int] = None,
labels_aggregation: Optional[str] = None,
labels_display: Optional[bool] = None,
labels_placement: Optional[str] = None,
min_height: Optional[int] = None,
min_width: Optional[int] = None,
name: Optional[str] = None,
number_of_decimals: Optional[int] = None,
orientation: Optional[str] = None,
position_x: Optional[int] = None,
position_y: Optional[int] = None,
refresh_interval: Optional[str] = None,
relative_window_time: Optional[str] = None,
show_beyond_data: Optional[bool] = None,
tab: Optional[str] = None,
thresholds: Optional[Sequence[DashboardBarChartThresholdArgs]] = None,
timestamp_gte: Optional[str] = None,
timestamp_lte: Optional[str] = None,
timezone: Optional[str] = None,
value_mappings: Optional[Sequence[DashboardBarChartValueMappingArgs]] = None,
width: Optional[int] = None,
y_axis_unit: Optional[str] = None) -> DashboardBarChart
func GetDashboardBarChart(ctx *Context, name string, id IDInput, state *DashboardBarChartState, opts ...ResourceOption) (*DashboardBarChart, error)
public static DashboardBarChart Get(string name, Input<string> id, DashboardBarChartState? state, CustomResourceOptions? opts = null)
public static DashboardBarChart get(String name, Output<String> id, DashboardBarChartState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Chart
Items List<Splight.Splight. Inputs. Dashboard Bar Chart Chart Item> - chart traces to be included
- Collection string
- Description string
- chart description
- Display
Time boolRange - whether to display the time range or not
- Height int
- chart height in px
- Labels
Aggregation string - [last|avg|...] aggregation
- Labels
Display bool - whether to display the labels or not
- Labels
Placement string - [right|bottom] placement
- Min
Height int - minimum chart height
- Min
Width int - minimum chart width
- Name string
- name of the chart
- Number
Of intDecimals - number of decimals
- Orientation string
- orientation
- Position
X int - chart x position
- Position
Y int - chart y position
- Refresh
Interval string - refresh interval
- Relative
Window stringTime - relative window time
- Show
Beyond boolData - whether to show data which is beyond timestamp_lte or not
- Tab string
- id for the tab where to place the chart
- Thresholds
List<Splight.
Splight. Inputs. Dashboard Bar Chart Threshold> - optional static lines to be added to the chart as references
- Timestamp
Gte string - date in isoformat or shortcut string where to end reading
- Timestamp
Lte string - date in isoformat or shortcut string where to start reading
- Timezone string
- chart timezone
- Value
Mappings List<Splight.Splight. Inputs. Dashboard Bar Chart Value Mapping> - optional mappings to transform data with rules
- Width int
- chart width in cols (max 20)
- YAxis
Unit string - y axis units
- Chart
Items []DashboardBar Chart Chart Item Args - chart traces to be included
- Collection string
- Description string
- chart description
- Display
Time boolRange - whether to display the time range or not
- Height int
- chart height in px
- Labels
Aggregation string - [last|avg|...] aggregation
- Labels
Display bool - whether to display the labels or not
- Labels
Placement string - [right|bottom] placement
- Min
Height int - minimum chart height
- Min
Width int - minimum chart width
- Name string
- name of the chart
- Number
Of intDecimals - number of decimals
- Orientation string
- orientation
- Position
X int - chart x position
- Position
Y int - chart y position
- Refresh
Interval string - refresh interval
- Relative
Window stringTime - relative window time
- Show
Beyond boolData - whether to show data which is beyond timestamp_lte or not
- Tab string
- id for the tab where to place the chart
- Thresholds
[]Dashboard
Bar Chart Threshold Args - optional static lines to be added to the chart as references
- Timestamp
Gte string - date in isoformat or shortcut string where to end reading
- Timestamp
Lte string - date in isoformat or shortcut string where to start reading
- Timezone string
- chart timezone
- Value
Mappings []DashboardBar Chart Value Mapping Args - optional mappings to transform data with rules
- Width int
- chart width in cols (max 20)
- YAxis
Unit string - y axis units
- chart
Items List<DashboardBar Chart Chart Item> - chart traces to be included
- collection String
- description String
- chart description
- display
Time BooleanRange - whether to display the time range or not
- height Integer
- chart height in px
- labels
Aggregation String - [last|avg|...] aggregation
- labels
Display Boolean - whether to display the labels or not
- labels
Placement String - [right|bottom] placement
- min
Height Integer - minimum chart height
- min
Width Integer - minimum chart width
- name String
- name of the chart
- number
Of IntegerDecimals - number of decimals
- orientation String
- orientation
- position
X Integer - chart x position
- position
Y Integer - chart y position
- refresh
Interval String - refresh interval
- relative
Window StringTime - relative window time
- show
Beyond BooleanData - whether to show data which is beyond timestamp_lte or not
- tab String
- id for the tab where to place the chart
- thresholds
List<Dashboard
Bar Chart Threshold> - optional static lines to be added to the chart as references
- timestamp
Gte String - date in isoformat or shortcut string where to end reading
- timestamp
Lte String - date in isoformat or shortcut string where to start reading
- timezone String
- chart timezone
- value
Mappings List<DashboardBar Chart Value Mapping> - optional mappings to transform data with rules
- width Integer
- chart width in cols (max 20)
- y
Axis StringUnit - y axis units
- chart
Items DashboardBar Chart Chart Item[] - chart traces to be included
- collection string
- description string
- chart description
- display
Time booleanRange - whether to display the time range or not
- height number
- chart height in px
- labels
Aggregation string - [last|avg|...] aggregation
- labels
Display boolean - whether to display the labels or not
- labels
Placement string - [right|bottom] placement
- min
Height number - minimum chart height
- min
Width number - minimum chart width
- name string
- name of the chart
- number
Of numberDecimals - number of decimals
- orientation string
- orientation
- position
X number - chart x position
- position
Y number - chart y position
- refresh
Interval string - refresh interval
- relative
Window stringTime - relative window time
- show
Beyond booleanData - whether to show data which is beyond timestamp_lte or not
- tab string
- id for the tab where to place the chart
- thresholds
Dashboard
Bar Chart Threshold[] - optional static lines to be added to the chart as references
- timestamp
Gte string - date in isoformat or shortcut string where to end reading
- timestamp
Lte string - date in isoformat or shortcut string where to start reading
- timezone string
- chart timezone
- value
Mappings DashboardBar Chart Value Mapping[] - optional mappings to transform data with rules
- width number
- chart width in cols (max 20)
- y
Axis stringUnit - y axis units
- chart_
items Sequence[DashboardBar Chart Chart Item Args] - chart traces to be included
- collection str
- description str
- chart description
- display_
time_ boolrange - whether to display the time range or not
- height int
- chart height in px
- labels_
aggregation str - [last|avg|...] aggregation
- labels_
display bool - whether to display the labels or not
- labels_
placement str - [right|bottom] placement
- min_
height int - minimum chart height
- min_
width int - minimum chart width
- name str
- name of the chart
- number_
of_ intdecimals - number of decimals
- orientation str
- orientation
- position_
x int - chart x position
- position_
y int - chart y position
- refresh_
interval str - refresh interval
- relative_
window_ strtime - relative window time
- show_
beyond_ booldata - whether to show data which is beyond timestamp_lte or not
- tab str
- id for the tab where to place the chart
- thresholds
Sequence[Dashboard
Bar Chart Threshold Args] - optional static lines to be added to the chart as references
- timestamp_
gte str - date in isoformat or shortcut string where to end reading
- timestamp_
lte str - date in isoformat or shortcut string where to start reading
- timezone str
- chart timezone
- value_
mappings Sequence[DashboardBar Chart Value Mapping Args] - optional mappings to transform data with rules
- width int
- chart width in cols (max 20)
- y_
axis_ strunit - y axis units
- chart
Items List<Property Map> - chart traces to be included
- collection String
- description String
- chart description
- display
Time BooleanRange - whether to display the time range or not
- height Number
- chart height in px
- labels
Aggregation String - [last|avg|...] aggregation
- labels
Display Boolean - whether to display the labels or not
- labels
Placement String - [right|bottom] placement
- min
Height Number - minimum chart height
- min
Width Number - minimum chart width
- name String
- name of the chart
- number
Of NumberDecimals - number of decimals
- orientation String
- orientation
- position
X Number - chart x position
- position
Y Number - chart y position
- refresh
Interval String - refresh interval
- relative
Window StringTime - relative window time
- show
Beyond BooleanData - whether to show data which is beyond timestamp_lte or not
- tab String
- id for the tab where to place the chart
- thresholds List<Property Map>
- optional static lines to be added to the chart as references
- timestamp
Gte String - date in isoformat or shortcut string where to end reading
- timestamp
Lte String - date in isoformat or shortcut string where to start reading
- timezone String
- chart timezone
- value
Mappings List<Property Map> - optional mappings to transform data with rules
- width Number
- chart width in cols (max 20)
- y
Axis StringUnit - y axis units
Supporting Types
DashboardBarChartChartItem, DashboardBarChartChartItemArgs
- Color string
- Expression
Plain string - Query
Filter Splight.Asset Splight. Inputs. Dashboard Bar Chart Chart Item Query Filter Asset - Asset filter
- Query
Filter Splight.Attribute Splight. Inputs. Dashboard Bar Chart Chart Item Query Filter Attribute - Attribute filter
- Query
Plain string - Ref
Id string - Type string
- bool
- Label string
- Query
Group stringFunction - Query
Group stringUnit - Query
Limit int - Query
Sort intDirection
- Color string
- Expression
Plain string - Query
Filter DashboardAsset Bar Chart Chart Item Query Filter Asset - Asset filter
- Query
Filter DashboardAttribute Bar Chart Chart Item Query Filter Attribute - Attribute filter
- Query
Plain string - Ref
Id string - Type string
- bool
- Label string
- Query
Group stringFunction - Query
Group stringUnit - Query
Limit int - Query
Sort intDirection
- color String
- expression
Plain String - query
Filter DashboardAsset Bar Chart Chart Item Query Filter Asset - Asset filter
- query
Filter DashboardAttribute Bar Chart Chart Item Query Filter Attribute - Attribute filter
- query
Plain String - ref
Id String - type String
- Boolean
- label String
- query
Group StringFunction - query
Group StringUnit - query
Limit Integer - query
Sort IntegerDirection
- color string
- expression
Plain string - query
Filter DashboardAsset Bar Chart Chart Item Query Filter Asset - Asset filter
- query
Filter DashboardAttribute Bar Chart Chart Item Query Filter Attribute - Attribute filter
- query
Plain string - ref
Id string - type string
- boolean
- label string
- query
Group stringFunction - query
Group stringUnit - query
Limit number - query
Sort numberDirection
- color str
- expression_
plain str - query_
filter_ Dashboardasset Bar Chart Chart Item Query Filter Asset - Asset filter
- query_
filter_ Dashboardattribute Bar Chart Chart Item Query Filter Attribute - Attribute filter
- query_
plain str - ref_
id str - type str
- bool
- label str
- query_
group_ strfunction - query_
group_ strunit - query_
limit int - query_
sort_ intdirection
- color String
- expression
Plain String - query
Filter Property MapAsset - Asset filter
- query
Filter Property MapAttribute - Attribute filter
- query
Plain String - ref
Id String - type String
- Boolean
- label String
- query
Group StringFunction - query
Group StringUnit - query
Limit Number - query
Sort NumberDirection
DashboardBarChartChartItemQueryFilterAsset, DashboardBarChartChartItemQueryFilterAssetArgs
DashboardBarChartChartItemQueryFilterAttribute, DashboardBarChartChartItemQueryFilterAttributeArgs
DashboardBarChartThreshold, DashboardBarChartThresholdArgs
- Color string
- Display
Text string - Value double
- Color string
- Display
Text string - Value float64
- color String
- display
Text String - value Double
- color string
- display
Text string - value number
- color str
- display_
text str - value float
- color String
- display
Text String - value Number
DashboardBarChartValueMapping, DashboardBarChartValueMappingArgs
- Display
Text string - Match
Value string - Order int
- Type string
- Display
Text string - Match
Value string - Order int
- Type string
- display
Text String - match
Value String - order Integer
- type String
- display
Text string - match
Value string - order number
- type string
- display_
text str - match_
value str - order int
- type str
- display
Text String - match
Value String - order Number
- type String
Import
$ pulumi import splight:index/dashboardBarChart:DashboardBarChart [options] splight_dashboard_bar_chart.<name> <dashboard_chart_id>
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- splight splightplatform/pulumi-splight
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
splight
Terraform Provider.
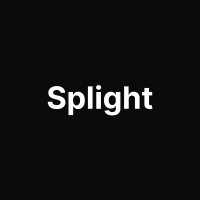