splight.Function
Explore with Pulumi AI
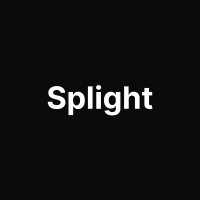
Example Usage
Create Function Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new Function(name: string, args: FunctionArgs, opts?: CustomResourceOptions);
@overload
def Function(resource_name: str,
args: FunctionArgs,
opts: Optional[ResourceOptions] = None)
@overload
def Function(resource_name: str,
opts: Optional[ResourceOptions] = None,
function_items: Optional[Sequence[FunctionFunctionItemArgs]] = None,
type: Optional[str] = None,
time_window: Optional[int] = None,
target_variable: Optional[str] = None,
target_attribute: Optional[FunctionTargetAttributeArgs] = None,
target_asset: Optional[FunctionTargetAssetArgs] = None,
description: Optional[str] = None,
name: Optional[str] = None,
cron_dom: Optional[int] = None,
rate_unit: Optional[str] = None,
rate_value: Optional[int] = None,
related_assets: Optional[Sequence[FunctionRelatedAssetArgs]] = None,
tags: Optional[Sequence[FunctionTagArgs]] = None,
cron_year: Optional[int] = None,
cron_month: Optional[int] = None,
cron_minutes: Optional[int] = None,
cron_hours: Optional[int] = None,
cron_dow: Optional[int] = None)
func NewFunction(ctx *Context, name string, args FunctionArgs, opts ...ResourceOption) (*Function, error)
public Function(string name, FunctionArgs args, CustomResourceOptions? opts = null)
public Function(String name, FunctionArgs args)
public Function(String name, FunctionArgs args, CustomResourceOptions options)
type: splight:Function
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args FunctionArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args FunctionArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args FunctionArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args FunctionArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args FunctionArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var functionResource = new Splight.Function("functionResource", new()
{
FunctionItems = new[]
{
new Splight.Inputs.FunctionFunctionItemArgs
{
Expression = "string",
ExpressionPlain = "string",
QueryFilterAsset = new Splight.Inputs.FunctionFunctionItemQueryFilterAssetArgs
{
Id = "string",
Name = "string",
},
QueryFilterAttribute = new Splight.Inputs.FunctionFunctionItemQueryFilterAttributeArgs
{
Id = "string",
Name = "string",
Type = "string",
},
QueryGroupFunction = "string",
QueryGroupUnit = "string",
QueryPlain = "string",
RefId = "string",
Type = "string",
Id = "string",
},
},
Type = "string",
TimeWindow = 0,
TargetVariable = "string",
TargetAttribute = new Splight.Inputs.FunctionTargetAttributeArgs
{
Id = "string",
Name = "string",
Type = "string",
},
TargetAsset = new Splight.Inputs.FunctionTargetAssetArgs
{
Id = "string",
Name = "string",
},
Description = "string",
Name = "string",
CronDom = 0,
RateUnit = "string",
RateValue = 0,
RelatedAssets = new[]
{
new Splight.Inputs.FunctionRelatedAssetArgs
{
Id = "string",
Name = "string",
},
},
Tags = new[]
{
new Splight.Inputs.FunctionTagArgs
{
Id = "string",
Name = "string",
},
},
CronYear = 0,
CronMonth = 0,
CronMinutes = 0,
CronHours = 0,
CronDow = 0,
});
example, err := splight.NewFunction(ctx, "functionResource", &splight.FunctionArgs{
FunctionItems: splight.FunctionFunctionItemArray{
&splight.FunctionFunctionItemArgs{
Expression: pulumi.String("string"),
ExpressionPlain: pulumi.String("string"),
QueryFilterAsset: &splight.FunctionFunctionItemQueryFilterAssetArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
QueryFilterAttribute: &splight.FunctionFunctionItemQueryFilterAttributeArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
},
QueryGroupFunction: pulumi.String("string"),
QueryGroupUnit: pulumi.String("string"),
QueryPlain: pulumi.String("string"),
RefId: pulumi.String("string"),
Type: pulumi.String("string"),
Id: pulumi.String("string"),
},
},
Type: pulumi.String("string"),
TimeWindow: pulumi.Int(0),
TargetVariable: pulumi.String("string"),
TargetAttribute: &splight.FunctionTargetAttributeArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
},
TargetAsset: &splight.FunctionTargetAssetArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
Description: pulumi.String("string"),
Name: pulumi.String("string"),
CronDom: pulumi.Int(0),
RateUnit: pulumi.String("string"),
RateValue: pulumi.Int(0),
RelatedAssets: splight.FunctionRelatedAssetArray{
&splight.FunctionRelatedAssetArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
},
Tags: splight.FunctionTagArray{
&splight.FunctionTagArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
},
CronYear: pulumi.Int(0),
CronMonth: pulumi.Int(0),
CronMinutes: pulumi.Int(0),
CronHours: pulumi.Int(0),
CronDow: pulumi.Int(0),
})
var functionResource = new Function("functionResource", FunctionArgs.builder()
.functionItems(FunctionFunctionItemArgs.builder()
.expression("string")
.expressionPlain("string")
.queryFilterAsset(FunctionFunctionItemQueryFilterAssetArgs.builder()
.id("string")
.name("string")
.build())
.queryFilterAttribute(FunctionFunctionItemQueryFilterAttributeArgs.builder()
.id("string")
.name("string")
.type("string")
.build())
.queryGroupFunction("string")
.queryGroupUnit("string")
.queryPlain("string")
.refId("string")
.type("string")
.id("string")
.build())
.type("string")
.timeWindow(0)
.targetVariable("string")
.targetAttribute(FunctionTargetAttributeArgs.builder()
.id("string")
.name("string")
.type("string")
.build())
.targetAsset(FunctionTargetAssetArgs.builder()
.id("string")
.name("string")
.build())
.description("string")
.name("string")
.cronDom(0)
.rateUnit("string")
.rateValue(0)
.relatedAssets(FunctionRelatedAssetArgs.builder()
.id("string")
.name("string")
.build())
.tags(FunctionTagArgs.builder()
.id("string")
.name("string")
.build())
.cronYear(0)
.cronMonth(0)
.cronMinutes(0)
.cronHours(0)
.cronDow(0)
.build());
function_resource = splight.Function("functionResource",
function_items=[splight.FunctionFunctionItemArgs(
expression="string",
expression_plain="string",
query_filter_asset=splight.FunctionFunctionItemQueryFilterAssetArgs(
id="string",
name="string",
),
query_filter_attribute=splight.FunctionFunctionItemQueryFilterAttributeArgs(
id="string",
name="string",
type="string",
),
query_group_function="string",
query_group_unit="string",
query_plain="string",
ref_id="string",
type="string",
id="string",
)],
type="string",
time_window=0,
target_variable="string",
target_attribute=splight.FunctionTargetAttributeArgs(
id="string",
name="string",
type="string",
),
target_asset=splight.FunctionTargetAssetArgs(
id="string",
name="string",
),
description="string",
name="string",
cron_dom=0,
rate_unit="string",
rate_value=0,
related_assets=[splight.FunctionRelatedAssetArgs(
id="string",
name="string",
)],
tags=[splight.FunctionTagArgs(
id="string",
name="string",
)],
cron_year=0,
cron_month=0,
cron_minutes=0,
cron_hours=0,
cron_dow=0)
const functionResource = new splight.Function("functionResource", {
functionItems: [{
expression: "string",
expressionPlain: "string",
queryFilterAsset: {
id: "string",
name: "string",
},
queryFilterAttribute: {
id: "string",
name: "string",
type: "string",
},
queryGroupFunction: "string",
queryGroupUnit: "string",
queryPlain: "string",
refId: "string",
type: "string",
id: "string",
}],
type: "string",
timeWindow: 0,
targetVariable: "string",
targetAttribute: {
id: "string",
name: "string",
type: "string",
},
targetAsset: {
id: "string",
name: "string",
},
description: "string",
name: "string",
cronDom: 0,
rateUnit: "string",
rateValue: 0,
relatedAssets: [{
id: "string",
name: "string",
}],
tags: [{
id: "string",
name: "string",
}],
cronYear: 0,
cronMonth: 0,
cronMinutes: 0,
cronHours: 0,
cronDow: 0,
});
type: splight:Function
properties:
cronDom: 0
cronDow: 0
cronHours: 0
cronMinutes: 0
cronMonth: 0
cronYear: 0
description: string
functionItems:
- expression: string
expressionPlain: string
id: string
queryFilterAsset:
id: string
name: string
queryFilterAttribute:
id: string
name: string
type: string
queryGroupFunction: string
queryGroupUnit: string
queryPlain: string
refId: string
type: string
name: string
rateUnit: string
rateValue: 0
relatedAssets:
- id: string
name: string
tags:
- id: string
name: string
targetAsset:
id: string
name: string
targetAttribute:
id: string
name: string
type: string
targetVariable: string
timeWindow: 0
type: string
Function Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The Function resource accepts the following input properties:
- Description string
- The description of the resource
- Function
Items List<Splight.Splight. Inputs. Function Function Item> - traces to be used to compute the results
- Target
Asset Splight.Splight. Inputs. Function Target Asset - Asset filter
- Target
Attribute Splight.Splight. Inputs. Function Target Attribute - Attribute filter
- Target
Variable string - variable to be considered to be ingested
- Time
Window int - window to fetch data from. Data out of that window will not be considered for evaluation
- Type string
- [cron|rate] type for the cron
- Cron
Dom int - schedule value for cron
- Cron
Dow int - schedule value for cron
- Cron
Hours int - schedule value for cron
- Cron
Minutes int - schedule value for cron
- Cron
Month int - schedule value for cron
- Cron
Year int - schedule value for cron
- Name string
- The name of the resource
- Rate
Unit string - [day|hour|minute] schedule unit
- Rate
Value int - schedule value
- List<Splight.
Splight. Inputs. Function Related Asset> - related assets of the resource
- List<Splight.
Splight. Inputs. Function Tag> - tags of the resource
- Description string
- The description of the resource
- Function
Items []FunctionFunction Item Args - traces to be used to compute the results
- Target
Asset FunctionTarget Asset Args - Asset filter
- Target
Attribute FunctionTarget Attribute Args - Attribute filter
- Target
Variable string - variable to be considered to be ingested
- Time
Window int - window to fetch data from. Data out of that window will not be considered for evaluation
- Type string
- [cron|rate] type for the cron
- Cron
Dom int - schedule value for cron
- Cron
Dow int - schedule value for cron
- Cron
Hours int - schedule value for cron
- Cron
Minutes int - schedule value for cron
- Cron
Month int - schedule value for cron
- Cron
Year int - schedule value for cron
- Name string
- The name of the resource
- Rate
Unit string - [day|hour|minute] schedule unit
- Rate
Value int - schedule value
- []Function
Related Asset Args - related assets of the resource
- []Function
Tag Args - tags of the resource
- description String
- The description of the resource
- function
Items List<FunctionFunction Item> - traces to be used to compute the results
- target
Asset FunctionTarget Asset - Asset filter
- target
Attribute FunctionTarget Attribute - Attribute filter
- target
Variable String - variable to be considered to be ingested
- time
Window Integer - window to fetch data from. Data out of that window will not be considered for evaluation
- type String
- [cron|rate] type for the cron
- cron
Dom Integer - schedule value for cron
- cron
Dow Integer - schedule value for cron
- cron
Hours Integer - schedule value for cron
- cron
Minutes Integer - schedule value for cron
- cron
Month Integer - schedule value for cron
- cron
Year Integer - schedule value for cron
- name String
- The name of the resource
- rate
Unit String - [day|hour|minute] schedule unit
- rate
Value Integer - schedule value
- List<Function
Related Asset> - related assets of the resource
- List<Function
Tag> - tags of the resource
- description string
- The description of the resource
- function
Items FunctionFunction Item[] - traces to be used to compute the results
- target
Asset FunctionTarget Asset - Asset filter
- target
Attribute FunctionTarget Attribute - Attribute filter
- target
Variable string - variable to be considered to be ingested
- time
Window number - window to fetch data from. Data out of that window will not be considered for evaluation
- type string
- [cron|rate] type for the cron
- cron
Dom number - schedule value for cron
- cron
Dow number - schedule value for cron
- cron
Hours number - schedule value for cron
- cron
Minutes number - schedule value for cron
- cron
Month number - schedule value for cron
- cron
Year number - schedule value for cron
- name string
- The name of the resource
- rate
Unit string - [day|hour|minute] schedule unit
- rate
Value number - schedule value
- Function
Related Asset[] - related assets of the resource
- Function
Tag[] - tags of the resource
- description str
- The description of the resource
- function_
items Sequence[FunctionFunction Item Args] - traces to be used to compute the results
- target_
asset FunctionTarget Asset Args - Asset filter
- target_
attribute FunctionTarget Attribute Args - Attribute filter
- target_
variable str - variable to be considered to be ingested
- time_
window int - window to fetch data from. Data out of that window will not be considered for evaluation
- type str
- [cron|rate] type for the cron
- cron_
dom int - schedule value for cron
- cron_
dow int - schedule value for cron
- cron_
hours int - schedule value for cron
- cron_
minutes int - schedule value for cron
- cron_
month int - schedule value for cron
- cron_
year int - schedule value for cron
- name str
- The name of the resource
- rate_
unit str - [day|hour|minute] schedule unit
- rate_
value int - schedule value
- Sequence[Function
Related Asset Args] - related assets of the resource
- Sequence[Function
Tag Args] - tags of the resource
- description String
- The description of the resource
- function
Items List<Property Map> - traces to be used to compute the results
- target
Asset Property Map - Asset filter
- target
Attribute Property Map - Attribute filter
- target
Variable String - variable to be considered to be ingested
- time
Window Number - window to fetch data from. Data out of that window will not be considered for evaluation
- type String
- [cron|rate] type for the cron
- cron
Dom Number - schedule value for cron
- cron
Dow Number - schedule value for cron
- cron
Hours Number - schedule value for cron
- cron
Minutes Number - schedule value for cron
- cron
Month Number - schedule value for cron
- cron
Year Number - schedule value for cron
- name String
- The name of the resource
- rate
Unit String - [day|hour|minute] schedule unit
- rate
Value Number - schedule value
- List<Property Map>
- related assets of the resource
- List<Property Map>
- tags of the resource
Outputs
All input properties are implicitly available as output properties. Additionally, the Function resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing Function Resource
Get an existing Function resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: FunctionState, opts?: CustomResourceOptions): Function
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
cron_dom: Optional[int] = None,
cron_dow: Optional[int] = None,
cron_hours: Optional[int] = None,
cron_minutes: Optional[int] = None,
cron_month: Optional[int] = None,
cron_year: Optional[int] = None,
description: Optional[str] = None,
function_items: Optional[Sequence[FunctionFunctionItemArgs]] = None,
name: Optional[str] = None,
rate_unit: Optional[str] = None,
rate_value: Optional[int] = None,
related_assets: Optional[Sequence[FunctionRelatedAssetArgs]] = None,
tags: Optional[Sequence[FunctionTagArgs]] = None,
target_asset: Optional[FunctionTargetAssetArgs] = None,
target_attribute: Optional[FunctionTargetAttributeArgs] = None,
target_variable: Optional[str] = None,
time_window: Optional[int] = None,
type: Optional[str] = None) -> Function
func GetFunction(ctx *Context, name string, id IDInput, state *FunctionState, opts ...ResourceOption) (*Function, error)
public static Function Get(string name, Input<string> id, FunctionState? state, CustomResourceOptions? opts = null)
public static Function get(String name, Output<String> id, FunctionState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Cron
Dom int - schedule value for cron
- Cron
Dow int - schedule value for cron
- Cron
Hours int - schedule value for cron
- Cron
Minutes int - schedule value for cron
- Cron
Month int - schedule value for cron
- Cron
Year int - schedule value for cron
- Description string
- The description of the resource
- Function
Items List<Splight.Splight. Inputs. Function Function Item> - traces to be used to compute the results
- Name string
- The name of the resource
- Rate
Unit string - [day|hour|minute] schedule unit
- Rate
Value int - schedule value
- List<Splight.
Splight. Inputs. Function Related Asset> - related assets of the resource
- List<Splight.
Splight. Inputs. Function Tag> - tags of the resource
- Target
Asset Splight.Splight. Inputs. Function Target Asset - Asset filter
- Target
Attribute Splight.Splight. Inputs. Function Target Attribute - Attribute filter
- Target
Variable string - variable to be considered to be ingested
- Time
Window int - window to fetch data from. Data out of that window will not be considered for evaluation
- Type string
- [cron|rate] type for the cron
- Cron
Dom int - schedule value for cron
- Cron
Dow int - schedule value for cron
- Cron
Hours int - schedule value for cron
- Cron
Minutes int - schedule value for cron
- Cron
Month int - schedule value for cron
- Cron
Year int - schedule value for cron
- Description string
- The description of the resource
- Function
Items []FunctionFunction Item Args - traces to be used to compute the results
- Name string
- The name of the resource
- Rate
Unit string - [day|hour|minute] schedule unit
- Rate
Value int - schedule value
- []Function
Related Asset Args - related assets of the resource
- []Function
Tag Args - tags of the resource
- Target
Asset FunctionTarget Asset Args - Asset filter
- Target
Attribute FunctionTarget Attribute Args - Attribute filter
- Target
Variable string - variable to be considered to be ingested
- Time
Window int - window to fetch data from. Data out of that window will not be considered for evaluation
- Type string
- [cron|rate] type for the cron
- cron
Dom Integer - schedule value for cron
- cron
Dow Integer - schedule value for cron
- cron
Hours Integer - schedule value for cron
- cron
Minutes Integer - schedule value for cron
- cron
Month Integer - schedule value for cron
- cron
Year Integer - schedule value for cron
- description String
- The description of the resource
- function
Items List<FunctionFunction Item> - traces to be used to compute the results
- name String
- The name of the resource
- rate
Unit String - [day|hour|minute] schedule unit
- rate
Value Integer - schedule value
- List<Function
Related Asset> - related assets of the resource
- List<Function
Tag> - tags of the resource
- target
Asset FunctionTarget Asset - Asset filter
- target
Attribute FunctionTarget Attribute - Attribute filter
- target
Variable String - variable to be considered to be ingested
- time
Window Integer - window to fetch data from. Data out of that window will not be considered for evaluation
- type String
- [cron|rate] type for the cron
- cron
Dom number - schedule value for cron
- cron
Dow number - schedule value for cron
- cron
Hours number - schedule value for cron
- cron
Minutes number - schedule value for cron
- cron
Month number - schedule value for cron
- cron
Year number - schedule value for cron
- description string
- The description of the resource
- function
Items FunctionFunction Item[] - traces to be used to compute the results
- name string
- The name of the resource
- rate
Unit string - [day|hour|minute] schedule unit
- rate
Value number - schedule value
- Function
Related Asset[] - related assets of the resource
- Function
Tag[] - tags of the resource
- target
Asset FunctionTarget Asset - Asset filter
- target
Attribute FunctionTarget Attribute - Attribute filter
- target
Variable string - variable to be considered to be ingested
- time
Window number - window to fetch data from. Data out of that window will not be considered for evaluation
- type string
- [cron|rate] type for the cron
- cron_
dom int - schedule value for cron
- cron_
dow int - schedule value for cron
- cron_
hours int - schedule value for cron
- cron_
minutes int - schedule value for cron
- cron_
month int - schedule value for cron
- cron_
year int - schedule value for cron
- description str
- The description of the resource
- function_
items Sequence[FunctionFunction Item Args] - traces to be used to compute the results
- name str
- The name of the resource
- rate_
unit str - [day|hour|minute] schedule unit
- rate_
value int - schedule value
- Sequence[Function
Related Asset Args] - related assets of the resource
- Sequence[Function
Tag Args] - tags of the resource
- target_
asset FunctionTarget Asset Args - Asset filter
- target_
attribute FunctionTarget Attribute Args - Attribute filter
- target_
variable str - variable to be considered to be ingested
- time_
window int - window to fetch data from. Data out of that window will not be considered for evaluation
- type str
- [cron|rate] type for the cron
- cron
Dom Number - schedule value for cron
- cron
Dow Number - schedule value for cron
- cron
Hours Number - schedule value for cron
- cron
Minutes Number - schedule value for cron
- cron
Month Number - schedule value for cron
- cron
Year Number - schedule value for cron
- description String
- The description of the resource
- function
Items List<Property Map> - traces to be used to compute the results
- name String
- The name of the resource
- rate
Unit String - [day|hour|minute] schedule unit
- rate
Value Number - schedule value
- List<Property Map>
- related assets of the resource
- List<Property Map>
- tags of the resource
- target
Asset Property Map - Asset filter
- target
Attribute Property Map - Attribute filter
- target
Variable String - variable to be considered to be ingested
- time
Window Number - window to fetch data from. Data out of that window will not be considered for evaluation
- type String
- [cron|rate] type for the cron
Supporting Types
FunctionFunctionItem, FunctionFunctionItemArgs
- Expression string
- how the expression is shown (i.e 'A * 2')
- Expression
Plain string - actual mongo query containing the expression
- Query
Filter Splight.Asset Splight. Inputs. Function Function Item Query Filter Asset - Asset filter
- Query
Filter Splight.Attribute Splight. Inputs. Function Function Item Query Filter Attribute - Attribute filter
- Query
Group stringFunction - function used to aggregate data
- Query
Group stringUnit - time window to apply the aggregation
- Query
Plain string - actual mongo query
- Ref
Id string - identifier of the variable (i.e 'A')
- Type string
- either QUERY or EXPRESSION
- Id string
- Id of the function item
- Expression string
- how the expression is shown (i.e 'A * 2')
- Expression
Plain string - actual mongo query containing the expression
- Query
Filter FunctionAsset Function Item Query Filter Asset - Asset filter
- Query
Filter FunctionAttribute Function Item Query Filter Attribute - Attribute filter
- Query
Group stringFunction - function used to aggregate data
- Query
Group stringUnit - time window to apply the aggregation
- Query
Plain string - actual mongo query
- Ref
Id string - identifier of the variable (i.e 'A')
- Type string
- either QUERY or EXPRESSION
- Id string
- Id of the function item
- expression String
- how the expression is shown (i.e 'A * 2')
- expression
Plain String - actual mongo query containing the expression
- query
Filter FunctionAsset Function Item Query Filter Asset - Asset filter
- query
Filter FunctionAttribute Function Item Query Filter Attribute - Attribute filter
- query
Group StringFunction - function used to aggregate data
- query
Group StringUnit - time window to apply the aggregation
- query
Plain String - actual mongo query
- ref
Id String - identifier of the variable (i.e 'A')
- type String
- either QUERY or EXPRESSION
- id String
- Id of the function item
- expression string
- how the expression is shown (i.e 'A * 2')
- expression
Plain string - actual mongo query containing the expression
- query
Filter FunctionAsset Function Item Query Filter Asset - Asset filter
- query
Filter FunctionAttribute Function Item Query Filter Attribute - Attribute filter
- query
Group stringFunction - function used to aggregate data
- query
Group stringUnit - time window to apply the aggregation
- query
Plain string - actual mongo query
- ref
Id string - identifier of the variable (i.e 'A')
- type string
- either QUERY or EXPRESSION
- id string
- Id of the function item
- expression str
- how the expression is shown (i.e 'A * 2')
- expression_
plain str - actual mongo query containing the expression
- query_
filter_ Functionasset Function Item Query Filter Asset - Asset filter
- query_
filter_ Functionattribute Function Item Query Filter Attribute - Attribute filter
- query_
group_ strfunction - function used to aggregate data
- query_
group_ strunit - time window to apply the aggregation
- query_
plain str - actual mongo query
- ref_
id str - identifier of the variable (i.e 'A')
- type str
- either QUERY or EXPRESSION
- id str
- Id of the function item
- expression String
- how the expression is shown (i.e 'A * 2')
- expression
Plain String - actual mongo query containing the expression
- query
Filter Property MapAsset - Asset filter
- query
Filter Property MapAttribute - Attribute filter
- query
Group StringFunction - function used to aggregate data
- query
Group StringUnit - time window to apply the aggregation
- query
Plain String - actual mongo query
- ref
Id String - identifier of the variable (i.e 'A')
- type String
- either QUERY or EXPRESSION
- id String
- Id of the function item
FunctionFunctionItemQueryFilterAsset, FunctionFunctionItemQueryFilterAssetArgs
FunctionFunctionItemQueryFilterAttribute, FunctionFunctionItemQueryFilterAttributeArgs
FunctionRelatedAsset, FunctionRelatedAssetArgs
FunctionTag, FunctionTagArgs
FunctionTargetAsset, FunctionTargetAssetArgs
FunctionTargetAttribute, FunctionTargetAttributeArgs
Import
$ pulumi import splight:index/function:Function [options] splight_function.<name> <function_id>
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- splight splightplatform/pulumi-splight
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
splight
Terraform Provider.
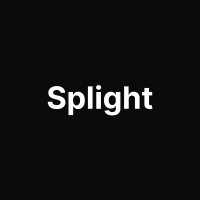