splight.Line
Explore with Pulumi AI
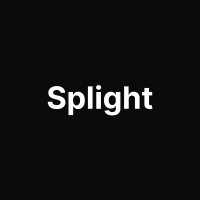
Example Usage
Create Line Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new Line(name: string, args: LineArgs, opts?: CustomResourceOptions);
@overload
def Line(resource_name: str,
args: LineArgs,
opts: Optional[ResourceOptions] = None)
@overload
def Line(resource_name: str,
opts: Optional[ResourceOptions] = None,
maximum_allowed_temperature_lte: Optional[LineMaximumAllowedTemperatureLteArgs] = None,
susceptance: Optional[LineSusceptanceArgs] = None,
capacitance: Optional[LineCapacitanceArgs] = None,
conductance: Optional[LineConductanceArgs] = None,
maximum_allowed_power: Optional[LineMaximumAllowedPowerArgs] = None,
diameter: Optional[LineDiameterArgs] = None,
emissivity: Optional[LineEmissivityArgs] = None,
maximum_allowed_temperature: Optional[LineMaximumAllowedTemperatureArgs] = None,
length: Optional[LineLengthArgs] = None,
maximum_allowed_current: Optional[LineMaximumAllowedCurrentArgs] = None,
temperature_coeff_resistance: Optional[LineTemperatureCoeffResistanceArgs] = None,
atmosphere: Optional[LineAtmosphereArgs] = None,
safety_margin_for_power: Optional[LineSafetyMarginForPowerArgs] = None,
maximum_allowed_temperature_ste: Optional[LineMaximumAllowedTemperatureSteArgs] = None,
absorptivity: Optional[LineAbsorptivityArgs] = None,
number_of_conductors: Optional[LineNumberOfConductorsArgs] = None,
reactance: Optional[LineReactanceArgs] = None,
reference_resistance: Optional[LineReferenceResistanceArgs] = None,
resistance: Optional[LineResistanceArgs] = None,
related_assets: Optional[Sequence[LineRelatedAssetArgs]] = None,
name: Optional[str] = None,
geometry: Optional[str] = None,
tags: Optional[Sequence[LineTagArgs]] = None,
description: Optional[str] = None)
func NewLine(ctx *Context, name string, args LineArgs, opts ...ResourceOption) (*Line, error)
public Line(string name, LineArgs args, CustomResourceOptions? opts = null)
type: splight:Line
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args LineArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args LineArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args LineArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args LineArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args LineArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var lineResource = new Splight.Line("lineResource", new()
{
MaximumAllowedTemperatureLte = new Splight.Inputs.LineMaximumAllowedTemperatureLteArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
Susceptance = new Splight.Inputs.LineSusceptanceArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
Capacitance = new Splight.Inputs.LineCapacitanceArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
Conductance = new Splight.Inputs.LineConductanceArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
MaximumAllowedPower = new Splight.Inputs.LineMaximumAllowedPowerArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
Diameter = new Splight.Inputs.LineDiameterArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
Emissivity = new Splight.Inputs.LineEmissivityArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
MaximumAllowedTemperature = new Splight.Inputs.LineMaximumAllowedTemperatureArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
Length = new Splight.Inputs.LineLengthArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
MaximumAllowedCurrent = new Splight.Inputs.LineMaximumAllowedCurrentArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
TemperatureCoeffResistance = new Splight.Inputs.LineTemperatureCoeffResistanceArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
Atmosphere = new Splight.Inputs.LineAtmosphereArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
SafetyMarginForPower = new Splight.Inputs.LineSafetyMarginForPowerArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
MaximumAllowedTemperatureSte = new Splight.Inputs.LineMaximumAllowedTemperatureSteArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
Absorptivity = new Splight.Inputs.LineAbsorptivityArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
NumberOfConductors = new Splight.Inputs.LineNumberOfConductorsArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
Reactance = new Splight.Inputs.LineReactanceArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
ReferenceResistance = new Splight.Inputs.LineReferenceResistanceArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
Resistance = new Splight.Inputs.LineResistanceArgs
{
Value = "string",
Asset = "string",
Id = "string",
Name = "string",
Type = "string",
Unit = "string",
},
RelatedAssets = new[]
{
new Splight.Inputs.LineRelatedAssetArgs
{
Id = "string",
Name = "string",
},
},
Name = "string",
Geometry = "string",
Tags = new[]
{
new Splight.Inputs.LineTagArgs
{
Id = "string",
Name = "string",
},
},
Description = "string",
});
example, err := splight.NewLine(ctx, "lineResource", &splight.LineArgs{
MaximumAllowedTemperatureLte: &splight.LineMaximumAllowedTemperatureLteArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
Susceptance: &splight.LineSusceptanceArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
Capacitance: &splight.LineCapacitanceArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
Conductance: &splight.LineConductanceArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
MaximumAllowedPower: &splight.LineMaximumAllowedPowerArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
Diameter: &splight.LineDiameterArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
Emissivity: &splight.LineEmissivityArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
MaximumAllowedTemperature: &splight.LineMaximumAllowedTemperatureArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
Length: &splight.LineLengthArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
MaximumAllowedCurrent: &splight.LineMaximumAllowedCurrentArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
TemperatureCoeffResistance: &splight.LineTemperatureCoeffResistanceArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
Atmosphere: &splight.LineAtmosphereArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
SafetyMarginForPower: &splight.LineSafetyMarginForPowerArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
MaximumAllowedTemperatureSte: &splight.LineMaximumAllowedTemperatureSteArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
Absorptivity: &splight.LineAbsorptivityArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
NumberOfConductors: &splight.LineNumberOfConductorsArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
Reactance: &splight.LineReactanceArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
ReferenceResistance: &splight.LineReferenceResistanceArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
Resistance: &splight.LineResistanceArgs{
Value: pulumi.String("string"),
Asset: pulumi.String("string"),
Id: pulumi.String("string"),
Name: pulumi.String("string"),
Type: pulumi.String("string"),
Unit: pulumi.String("string"),
},
RelatedAssets: splight.LineRelatedAssetArray{
&splight.LineRelatedAssetArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
},
Name: pulumi.String("string"),
Geometry: pulumi.String("string"),
Tags: splight.LineTagArray{
&splight.LineTagArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
},
Description: pulumi.String("string"),
})
var lineResource = new Line("lineResource", LineArgs.builder()
.maximumAllowedTemperatureLte(LineMaximumAllowedTemperatureLteArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.susceptance(LineSusceptanceArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.capacitance(LineCapacitanceArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.conductance(LineConductanceArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.maximumAllowedPower(LineMaximumAllowedPowerArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.diameter(LineDiameterArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.emissivity(LineEmissivityArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.maximumAllowedTemperature(LineMaximumAllowedTemperatureArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.length(LineLengthArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.maximumAllowedCurrent(LineMaximumAllowedCurrentArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.temperatureCoeffResistance(LineTemperatureCoeffResistanceArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.atmosphere(LineAtmosphereArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.safetyMarginForPower(LineSafetyMarginForPowerArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.maximumAllowedTemperatureSte(LineMaximumAllowedTemperatureSteArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.absorptivity(LineAbsorptivityArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.numberOfConductors(LineNumberOfConductorsArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.reactance(LineReactanceArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.referenceResistance(LineReferenceResistanceArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.resistance(LineResistanceArgs.builder()
.value("string")
.asset("string")
.id("string")
.name("string")
.type("string")
.unit("string")
.build())
.relatedAssets(LineRelatedAssetArgs.builder()
.id("string")
.name("string")
.build())
.name("string")
.geometry("string")
.tags(LineTagArgs.builder()
.id("string")
.name("string")
.build())
.description("string")
.build());
line_resource = splight.Line("lineResource",
maximum_allowed_temperature_lte=splight.LineMaximumAllowedTemperatureLteArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
susceptance=splight.LineSusceptanceArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
capacitance=splight.LineCapacitanceArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
conductance=splight.LineConductanceArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
maximum_allowed_power=splight.LineMaximumAllowedPowerArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
diameter=splight.LineDiameterArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
emissivity=splight.LineEmissivityArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
maximum_allowed_temperature=splight.LineMaximumAllowedTemperatureArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
length=splight.LineLengthArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
maximum_allowed_current=splight.LineMaximumAllowedCurrentArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
temperature_coeff_resistance=splight.LineTemperatureCoeffResistanceArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
atmosphere=splight.LineAtmosphereArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
safety_margin_for_power=splight.LineSafetyMarginForPowerArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
maximum_allowed_temperature_ste=splight.LineMaximumAllowedTemperatureSteArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
absorptivity=splight.LineAbsorptivityArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
number_of_conductors=splight.LineNumberOfConductorsArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
reactance=splight.LineReactanceArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
reference_resistance=splight.LineReferenceResistanceArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
resistance=splight.LineResistanceArgs(
value="string",
asset="string",
id="string",
name="string",
type="string",
unit="string",
),
related_assets=[splight.LineRelatedAssetArgs(
id="string",
name="string",
)],
name="string",
geometry="string",
tags=[splight.LineTagArgs(
id="string",
name="string",
)],
description="string")
const lineResource = new splight.Line("lineResource", {
maximumAllowedTemperatureLte: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
susceptance: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
capacitance: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
conductance: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
maximumAllowedPower: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
diameter: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
emissivity: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
maximumAllowedTemperature: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
length: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
maximumAllowedCurrent: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
temperatureCoeffResistance: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
atmosphere: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
safetyMarginForPower: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
maximumAllowedTemperatureSte: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
absorptivity: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
numberOfConductors: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
reactance: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
referenceResistance: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
resistance: {
value: "string",
asset: "string",
id: "string",
name: "string",
type: "string",
unit: "string",
},
relatedAssets: [{
id: "string",
name: "string",
}],
name: "string",
geometry: "string",
tags: [{
id: "string",
name: "string",
}],
description: "string",
});
type: splight:Line
properties:
absorptivity:
asset: string
id: string
name: string
type: string
unit: string
value: string
atmosphere:
asset: string
id: string
name: string
type: string
unit: string
value: string
capacitance:
asset: string
id: string
name: string
type: string
unit: string
value: string
conductance:
asset: string
id: string
name: string
type: string
unit: string
value: string
description: string
diameter:
asset: string
id: string
name: string
type: string
unit: string
value: string
emissivity:
asset: string
id: string
name: string
type: string
unit: string
value: string
geometry: string
length:
asset: string
id: string
name: string
type: string
unit: string
value: string
maximumAllowedCurrent:
asset: string
id: string
name: string
type: string
unit: string
value: string
maximumAllowedPower:
asset: string
id: string
name: string
type: string
unit: string
value: string
maximumAllowedTemperature:
asset: string
id: string
name: string
type: string
unit: string
value: string
maximumAllowedTemperatureLte:
asset: string
id: string
name: string
type: string
unit: string
value: string
maximumAllowedTemperatureSte:
asset: string
id: string
name: string
type: string
unit: string
value: string
name: string
numberOfConductors:
asset: string
id: string
name: string
type: string
unit: string
value: string
reactance:
asset: string
id: string
name: string
type: string
unit: string
value: string
referenceResistance:
asset: string
id: string
name: string
type: string
unit: string
value: string
relatedAssets:
- id: string
name: string
resistance:
asset: string
id: string
name: string
type: string
unit: string
value: string
safetyMarginForPower:
asset: string
id: string
name: string
type: string
unit: string
value: string
susceptance:
asset: string
id: string
name: string
type: string
unit: string
value: string
tags:
- id: string
name: string
temperatureCoeffResistance:
asset: string
id: string
name: string
type: string
unit: string
value: string
Line Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The Line resource accepts the following input properties:
- Absorptivity
Splight.
Splight. Inputs. Line Absorptivity - attribute of the resource
- Atmosphere
Splight.
Splight. Inputs. Line Atmosphere - attribute of the resource
- Capacitance
Splight.
Splight. Inputs. Line Capacitance - attribute of the resource
- Conductance
Splight.
Splight. Inputs. Line Conductance - attribute of the resource
- Diameter
Splight.
Splight. Inputs. Line Diameter - attribute of the resource
- Emissivity
Splight.
Splight. Inputs. Line Emissivity - attribute of the resource
- Length
Splight.
Splight. Inputs. Line Length - attribute of the resource
- Maximum
Allowed Splight.Current Splight. Inputs. Line Maximum Allowed Current - attribute of the resource
- Maximum
Allowed Splight.Power Splight. Inputs. Line Maximum Allowed Power - attribute of the resource
- Maximum
Allowed Splight.Temperature Splight. Inputs. Line Maximum Allowed Temperature - attribute of the resource
- Maximum
Allowed Splight.Temperature Lte Splight. Inputs. Line Maximum Allowed Temperature Lte - attribute of the resource
- Maximum
Allowed Splight.Temperature Ste Splight. Inputs. Line Maximum Allowed Temperature Ste - attribute of the resource
- Number
Of Splight.Conductors Splight. Inputs. Line Number Of Conductors - attribute of the resource
- Reactance
Splight.
Splight. Inputs. Line Reactance - attribute of the resource
- Reference
Resistance Splight.Splight. Inputs. Line Reference Resistance - attribute of the resource
- Resistance
Splight.
Splight. Inputs. Line Resistance - attribute of the resource
- Safety
Margin Splight.For Power Splight. Inputs. Line Safety Margin For Power - attribute of the resource
- Susceptance
Splight.
Splight. Inputs. Line Susceptance - attribute of the resource
- Temperature
Coeff Splight.Resistance Splight. Inputs. Line Temperature Coeff Resistance - attribute of the resource
- Description string
- description of the resource
- Geometry string
- geo position and shape of the resource
- Name string
- name of the resource
- List<Splight.
Splight. Inputs. Line Related Asset> - related assets of the resource
- List<Splight.
Splight. Inputs. Line Tag> - tags of the resource
- Absorptivity
Line
Absorptivity Args - attribute of the resource
- Atmosphere
Line
Atmosphere Args - attribute of the resource
- Capacitance
Line
Capacitance Args - attribute of the resource
- Conductance
Line
Conductance Args - attribute of the resource
- Diameter
Line
Diameter Args - attribute of the resource
- Emissivity
Line
Emissivity Args - attribute of the resource
- Length
Line
Length Args - attribute of the resource
- Maximum
Allowed LineCurrent Maximum Allowed Current Args - attribute of the resource
- Maximum
Allowed LinePower Maximum Allowed Power Args - attribute of the resource
- Maximum
Allowed LineTemperature Maximum Allowed Temperature Args - attribute of the resource
- Maximum
Allowed LineTemperature Lte Maximum Allowed Temperature Lte Args - attribute of the resource
- Maximum
Allowed LineTemperature Ste Maximum Allowed Temperature Ste Args - attribute of the resource
- Number
Of LineConductors Number Of Conductors Args - attribute of the resource
- Reactance
Line
Reactance Args - attribute of the resource
- Reference
Resistance LineReference Resistance Args - attribute of the resource
- Resistance
Line
Resistance Args - attribute of the resource
- Safety
Margin LineFor Power Safety Margin For Power Args - attribute of the resource
- Susceptance
Line
Susceptance Args - attribute of the resource
- Temperature
Coeff LineResistance Temperature Coeff Resistance Args - attribute of the resource
- Description string
- description of the resource
- Geometry string
- geo position and shape of the resource
- Name string
- name of the resource
- []Line
Related Asset Args - related assets of the resource
- []Line
Tag Args - tags of the resource
- absorptivity
Line
Absorptivity - attribute of the resource
- atmosphere
Line
Atmosphere - attribute of the resource
- capacitance
Line
Capacitance - attribute of the resource
- conductance
Line
Conductance - attribute of the resource
- diameter
Line
Diameter - attribute of the resource
- emissivity
Line
Emissivity - attribute of the resource
- length
Line
Length - attribute of the resource
- maximum
Allowed LineCurrent Maximum Allowed Current - attribute of the resource
- maximum
Allowed LinePower Maximum Allowed Power - attribute of the resource
- maximum
Allowed LineTemperature Maximum Allowed Temperature - attribute of the resource
- maximum
Allowed LineTemperature Lte Maximum Allowed Temperature Lte - attribute of the resource
- maximum
Allowed LineTemperature Ste Maximum Allowed Temperature Ste - attribute of the resource
- number
Of LineConductors Number Of Conductors - attribute of the resource
- reactance
Line
Reactance - attribute of the resource
- reference
Resistance LineReference Resistance - attribute of the resource
- resistance
Line
Resistance - attribute of the resource
- safety
Margin LineFor Power Safety Margin For Power - attribute of the resource
- susceptance
Line
Susceptance - attribute of the resource
- temperature
Coeff LineResistance Temperature Coeff Resistance - attribute of the resource
- description String
- description of the resource
- geometry String
- geo position and shape of the resource
- name String
- name of the resource
- List<Line
Related Asset> - related assets of the resource
- List<Line
Tag> - tags of the resource
- absorptivity
Line
Absorptivity - attribute of the resource
- atmosphere
Line
Atmosphere - attribute of the resource
- capacitance
Line
Capacitance - attribute of the resource
- conductance
Line
Conductance - attribute of the resource
- diameter
Line
Diameter - attribute of the resource
- emissivity
Line
Emissivity - attribute of the resource
- length
Line
Length - attribute of the resource
- maximum
Allowed LineCurrent Maximum Allowed Current - attribute of the resource
- maximum
Allowed LinePower Maximum Allowed Power - attribute of the resource
- maximum
Allowed LineTemperature Maximum Allowed Temperature - attribute of the resource
- maximum
Allowed LineTemperature Lte Maximum Allowed Temperature Lte - attribute of the resource
- maximum
Allowed LineTemperature Ste Maximum Allowed Temperature Ste - attribute of the resource
- number
Of LineConductors Number Of Conductors - attribute of the resource
- reactance
Line
Reactance - attribute of the resource
- reference
Resistance LineReference Resistance - attribute of the resource
- resistance
Line
Resistance - attribute of the resource
- safety
Margin LineFor Power Safety Margin For Power - attribute of the resource
- susceptance
Line
Susceptance - attribute of the resource
- temperature
Coeff LineResistance Temperature Coeff Resistance - attribute of the resource
- description string
- description of the resource
- geometry string
- geo position and shape of the resource
- name string
- name of the resource
- Line
Related Asset[] - related assets of the resource
- Line
Tag[] - tags of the resource
- absorptivity
Line
Absorptivity Args - attribute of the resource
- atmosphere
Line
Atmosphere Args - attribute of the resource
- capacitance
Line
Capacitance Args - attribute of the resource
- conductance
Line
Conductance Args - attribute of the resource
- diameter
Line
Diameter Args - attribute of the resource
- emissivity
Line
Emissivity Args - attribute of the resource
- length
Line
Length Args - attribute of the resource
- maximum_
allowed_ Linecurrent Maximum Allowed Current Args - attribute of the resource
- maximum_
allowed_ Linepower Maximum Allowed Power Args - attribute of the resource
- maximum_
allowed_ Linetemperature Maximum Allowed Temperature Args - attribute of the resource
- maximum_
allowed_ Linetemperature_ lte Maximum Allowed Temperature Lte Args - attribute of the resource
- maximum_
allowed_ Linetemperature_ ste Maximum Allowed Temperature Ste Args - attribute of the resource
- number_
of_ Lineconductors Number Of Conductors Args - attribute of the resource
- reactance
Line
Reactance Args - attribute of the resource
- reference_
resistance LineReference Resistance Args - attribute of the resource
- resistance
Line
Resistance Args - attribute of the resource
- safety_
margin_ Linefor_ power Safety Margin For Power Args - attribute of the resource
- susceptance
Line
Susceptance Args - attribute of the resource
- temperature_
coeff_ Lineresistance Temperature Coeff Resistance Args - attribute of the resource
- description str
- description of the resource
- geometry str
- geo position and shape of the resource
- name str
- name of the resource
- Sequence[Line
Related Asset Args] - related assets of the resource
- Sequence[Line
Tag Args] - tags of the resource
- absorptivity Property Map
- attribute of the resource
- atmosphere Property Map
- attribute of the resource
- capacitance Property Map
- attribute of the resource
- conductance Property Map
- attribute of the resource
- diameter Property Map
- attribute of the resource
- emissivity Property Map
- attribute of the resource
- length Property Map
- attribute of the resource
- maximum
Allowed Property MapCurrent - attribute of the resource
- maximum
Allowed Property MapPower - attribute of the resource
- maximum
Allowed Property MapTemperature - attribute of the resource
- maximum
Allowed Property MapTemperature Lte - attribute of the resource
- maximum
Allowed Property MapTemperature Ste - attribute of the resource
- number
Of Property MapConductors - attribute of the resource
- reactance Property Map
- attribute of the resource
- reference
Resistance Property Map - attribute of the resource
- resistance Property Map
- attribute of the resource
- safety
Margin Property MapFor Power - attribute of the resource
- susceptance Property Map
- attribute of the resource
- temperature
Coeff Property MapResistance - attribute of the resource
- description String
- description of the resource
- geometry String
- geo position and shape of the resource
- name String
- name of the resource
- List<Property Map>
- related assets of the resource
- List<Property Map>
- tags of the resource
Outputs
All input properties are implicitly available as output properties. Additionally, the Line resource produces the following output properties:
- Active
Power List<Splight.Ends Splight. Outputs. Line Active Power End> - attribute of the resource
- Active
Powers List<Splight.Splight. Outputs. Line Active Power> - attribute of the resource
- Ampacities
List<Splight.
Splight. Outputs. Line Ampacity> - attribute of the resource
- Current
Rs List<Splight.Splight. Outputs. Line Current R> - attribute of the resource
- Current
S List<Splight.Splight. Outputs. Line Current> - attribute of the resource
- Current
Ts List<Splight.Splight. Outputs. Line Current T> - attribute of the resource
- Currents
List<Splight.
Splight. Outputs. Line Current> - attribute of the resource
- Energies
List<Splight.
Splight. Outputs. Line Energy> - attribute of the resource
- Id string
- The provider-assigned unique ID for this managed resource.
- Kinds
List<Splight.
Splight. Outputs. Line Kind> - kind of the resource
- Max
Temperatures List<Splight.Splight. Outputs. Line Max Temperature> - attribute of the resource
- Reactive
Powers List<Splight.Splight. Outputs. Line Reactive Power> - attribute of the resource
- Voltage
Rs List<Splight.Splight. Outputs. Line Voltage R> - attribute of the resource
- Voltage
Sts List<Splight.Splight. Outputs. Line Voltage St> - attribute of the resource
- Voltage
Trs List<Splight.Splight. Outputs. Line Voltage Tr> - attribute of the resource
- Active
Power []LineEnds Active Power End - attribute of the resource
- Active
Powers []LineActive Power - attribute of the resource
- Ampacities
[]Line
Ampacity - attribute of the resource
- Current
Rs []LineCurrent R - attribute of the resource
- Current
S []LineCurrent - attribute of the resource
- Current
Ts []LineCurrent T - attribute of the resource
- Currents
[]Line
Current - attribute of the resource
- Energies
[]Line
Energy - attribute of the resource
- Id string
- The provider-assigned unique ID for this managed resource.
- Kinds
[]Line
Kind - kind of the resource
- Max
Temperatures []LineMax Temperature - attribute of the resource
- Reactive
Powers []LineReactive Power - attribute of the resource
- Voltage
Rs []LineVoltage R - attribute of the resource
- Voltage
Sts []LineVoltage St - attribute of the resource
- Voltage
Trs []LineVoltage Tr - attribute of the resource
- active
Power List<LineEnds Active Power End> - attribute of the resource
- active
Powers List<LineActive Power> - attribute of the resource
- ampacities
List<Line
Ampacity> - attribute of the resource
- current
Rs List<LineCurrent R> - attribute of the resource
- current
S List<LineCurrent> - attribute of the resource
- current
Ts List<LineCurrent T> - attribute of the resource
- currents
List<Line
Current> - attribute of the resource
- energies
List<Line
Energy> - attribute of the resource
- id String
- The provider-assigned unique ID for this managed resource.
- kinds
List<Line
Kind> - kind of the resource
- max
Temperatures List<LineMax Temperature> - attribute of the resource
- reactive
Powers List<LineReactive Power> - attribute of the resource
- voltage
Rs List<LineVoltage R> - attribute of the resource
- voltage
Sts List<LineVoltage St> - attribute of the resource
- voltage
Trs List<LineVoltage Tr> - attribute of the resource
- active
Power LineEnds Active Power End[] - attribute of the resource
- active
Powers LineActive Power[] - attribute of the resource
- ampacities
Line
Ampacity[] - attribute of the resource
- current
Rs LineCurrent R[] - attribute of the resource
- current
S LineCurrent[] - attribute of the resource
- current
Ts LineCurrent T[] - attribute of the resource
- currents
Line
Current[] - attribute of the resource
- energies
Line
Energy[] - attribute of the resource
- id string
- The provider-assigned unique ID for this managed resource.
- kinds
Line
Kind[] - kind of the resource
- max
Temperatures LineMax Temperature[] - attribute of the resource
- reactive
Powers LineReactive Power[] - attribute of the resource
- voltage
Rs LineVoltage R[] - attribute of the resource
- voltage
Sts LineVoltage St[] - attribute of the resource
- voltage
Trs LineVoltage Tr[] - attribute of the resource
- active_
power_ Sequence[Lineends Active Power End] - attribute of the resource
- active_
powers Sequence[LineActive Power] - attribute of the resource
- ampacities
Sequence[Line
Ampacity] - attribute of the resource
- current_
rs Sequence[LineCurrent R] - attribute of the resource
- current_
s Sequence[LineCurrent] - attribute of the resource
- current_
ts Sequence[LineCurrent T] - attribute of the resource
- currents
Sequence[Line
Current] - attribute of the resource
- energies
Sequence[Line
Energy] - attribute of the resource
- id str
- The provider-assigned unique ID for this managed resource.
- kinds
Sequence[Line
Kind] - kind of the resource
- max_
temperatures Sequence[LineMax Temperature] - attribute of the resource
- reactive_
powers Sequence[LineReactive Power] - attribute of the resource
- voltage_
rs Sequence[LineVoltage R] - attribute of the resource
- voltage_
sts Sequence[LineVoltage St] - attribute of the resource
- voltage_
trs Sequence[LineVoltage Tr] - attribute of the resource
- active
Power List<Property Map>Ends - attribute of the resource
- active
Powers List<Property Map> - attribute of the resource
- ampacities List<Property Map>
- attribute of the resource
- current
Rs List<Property Map> - attribute of the resource
- current
S List<Property Map> - attribute of the resource
- current
Ts List<Property Map> - attribute of the resource
- currents List<Property Map>
- attribute of the resource
- energies List<Property Map>
- attribute of the resource
- id String
- The provider-assigned unique ID for this managed resource.
- kinds List<Property Map>
- kind of the resource
- max
Temperatures List<Property Map> - attribute of the resource
- reactive
Powers List<Property Map> - attribute of the resource
- voltage
Rs List<Property Map> - attribute of the resource
- voltage
Sts List<Property Map> - attribute of the resource
- voltage
Trs List<Property Map> - attribute of the resource
Look up Existing Line Resource
Get an existing Line resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: LineState, opts?: CustomResourceOptions): Line
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
absorptivity: Optional[LineAbsorptivityArgs] = None,
active_power_ends: Optional[Sequence[LineActivePowerEndArgs]] = None,
active_powers: Optional[Sequence[LineActivePowerArgs]] = None,
ampacities: Optional[Sequence[LineAmpacityArgs]] = None,
atmosphere: Optional[LineAtmosphereArgs] = None,
capacitance: Optional[LineCapacitanceArgs] = None,
conductance: Optional[LineConductanceArgs] = None,
current_rs: Optional[Sequence[LineCurrentRArgs]] = None,
current_s: Optional[Sequence[LineCurrentArgs]] = None,
current_ts: Optional[Sequence[LineCurrentTArgs]] = None,
currents: Optional[Sequence[LineCurrentArgs]] = None,
description: Optional[str] = None,
diameter: Optional[LineDiameterArgs] = None,
emissivity: Optional[LineEmissivityArgs] = None,
energies: Optional[Sequence[LineEnergyArgs]] = None,
geometry: Optional[str] = None,
kinds: Optional[Sequence[LineKindArgs]] = None,
length: Optional[LineLengthArgs] = None,
max_temperatures: Optional[Sequence[LineMaxTemperatureArgs]] = None,
maximum_allowed_current: Optional[LineMaximumAllowedCurrentArgs] = None,
maximum_allowed_power: Optional[LineMaximumAllowedPowerArgs] = None,
maximum_allowed_temperature: Optional[LineMaximumAllowedTemperatureArgs] = None,
maximum_allowed_temperature_lte: Optional[LineMaximumAllowedTemperatureLteArgs] = None,
maximum_allowed_temperature_ste: Optional[LineMaximumAllowedTemperatureSteArgs] = None,
name: Optional[str] = None,
number_of_conductors: Optional[LineNumberOfConductorsArgs] = None,
reactance: Optional[LineReactanceArgs] = None,
reactive_powers: Optional[Sequence[LineReactivePowerArgs]] = None,
reference_resistance: Optional[LineReferenceResistanceArgs] = None,
related_assets: Optional[Sequence[LineRelatedAssetArgs]] = None,
resistance: Optional[LineResistanceArgs] = None,
safety_margin_for_power: Optional[LineSafetyMarginForPowerArgs] = None,
susceptance: Optional[LineSusceptanceArgs] = None,
tags: Optional[Sequence[LineTagArgs]] = None,
temperature_coeff_resistance: Optional[LineTemperatureCoeffResistanceArgs] = None,
voltage_rs: Optional[Sequence[LineVoltageRArgs]] = None,
voltage_sts: Optional[Sequence[LineVoltageStArgs]] = None,
voltage_trs: Optional[Sequence[LineVoltageTrArgs]] = None) -> Line
func GetLine(ctx *Context, name string, id IDInput, state *LineState, opts ...ResourceOption) (*Line, error)
public static Line Get(string name, Input<string> id, LineState? state, CustomResourceOptions? opts = null)
public static Line get(String name, Output<String> id, LineState state, CustomResourceOptions options)
Resource lookup is not supported in YAML
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Absorptivity
Splight.
Splight. Inputs. Line Absorptivity - attribute of the resource
- Active
Power List<Splight.Ends Splight. Inputs. Line Active Power End> - attribute of the resource
- Active
Powers List<Splight.Splight. Inputs. Line Active Power> - attribute of the resource
- Ampacities
List<Splight.
Splight. Inputs. Line Ampacity> - attribute of the resource
- Atmosphere
Splight.
Splight. Inputs. Line Atmosphere - attribute of the resource
- Capacitance
Splight.
Splight. Inputs. Line Capacitance - attribute of the resource
- Conductance
Splight.
Splight. Inputs. Line Conductance - attribute of the resource
- Current
Rs List<Splight.Splight. Inputs. Line Current R> - attribute of the resource
- Current
S List<Splight.Splight. Inputs. Line Current> - attribute of the resource
- Current
Ts List<Splight.Splight. Inputs. Line Current T> - attribute of the resource
- Currents
List<Splight.
Splight. Inputs. Line Current> - attribute of the resource
- Description string
- description of the resource
- Diameter
Splight.
Splight. Inputs. Line Diameter - attribute of the resource
- Emissivity
Splight.
Splight. Inputs. Line Emissivity - attribute of the resource
- Energies
List<Splight.
Splight. Inputs. Line Energy> - attribute of the resource
- Geometry string
- geo position and shape of the resource
- Kinds
List<Splight.
Splight. Inputs. Line Kind> - kind of the resource
- Length
Splight.
Splight. Inputs. Line Length - attribute of the resource
- Max
Temperatures List<Splight.Splight. Inputs. Line Max Temperature> - attribute of the resource
- Maximum
Allowed Splight.Current Splight. Inputs. Line Maximum Allowed Current - attribute of the resource
- Maximum
Allowed Splight.Power Splight. Inputs. Line Maximum Allowed Power - attribute of the resource
- Maximum
Allowed Splight.Temperature Splight. Inputs. Line Maximum Allowed Temperature - attribute of the resource
- Maximum
Allowed Splight.Temperature Lte Splight. Inputs. Line Maximum Allowed Temperature Lte - attribute of the resource
- Maximum
Allowed Splight.Temperature Ste Splight. Inputs. Line Maximum Allowed Temperature Ste - attribute of the resource
- Name string
- name of the resource
- Number
Of Splight.Conductors Splight. Inputs. Line Number Of Conductors - attribute of the resource
- Reactance
Splight.
Splight. Inputs. Line Reactance - attribute of the resource
- Reactive
Powers List<Splight.Splight. Inputs. Line Reactive Power> - attribute of the resource
- Reference
Resistance Splight.Splight. Inputs. Line Reference Resistance - attribute of the resource
- List<Splight.
Splight. Inputs. Line Related Asset> - related assets of the resource
- Resistance
Splight.
Splight. Inputs. Line Resistance - attribute of the resource
- Safety
Margin Splight.For Power Splight. Inputs. Line Safety Margin For Power - attribute of the resource
- Susceptance
Splight.
Splight. Inputs. Line Susceptance - attribute of the resource
- List<Splight.
Splight. Inputs. Line Tag> - tags of the resource
- Temperature
Coeff Splight.Resistance Splight. Inputs. Line Temperature Coeff Resistance - attribute of the resource
- Voltage
Rs List<Splight.Splight. Inputs. Line Voltage R> - attribute of the resource
- Voltage
Sts List<Splight.Splight. Inputs. Line Voltage St> - attribute of the resource
- Voltage
Trs List<Splight.Splight. Inputs. Line Voltage Tr> - attribute of the resource
- Absorptivity
Line
Absorptivity Args - attribute of the resource
- Active
Power []LineEnds Active Power End Args - attribute of the resource
- Active
Powers []LineActive Power Args - attribute of the resource
- Ampacities
[]Line
Ampacity Args - attribute of the resource
- Atmosphere
Line
Atmosphere Args - attribute of the resource
- Capacitance
Line
Capacitance Args - attribute of the resource
- Conductance
Line
Conductance Args - attribute of the resource
- Current
Rs []LineCurrent RArgs - attribute of the resource
- Current
S []LineCurrent Args - attribute of the resource
- Current
Ts []LineCurrent TArgs - attribute of the resource
- Currents
[]Line
Current Args - attribute of the resource
- Description string
- description of the resource
- Diameter
Line
Diameter Args - attribute of the resource
- Emissivity
Line
Emissivity Args - attribute of the resource
- Energies
[]Line
Energy Args - attribute of the resource
- Geometry string
- geo position and shape of the resource
- Kinds
[]Line
Kind Args - kind of the resource
- Length
Line
Length Args - attribute of the resource
- Max
Temperatures []LineMax Temperature Args - attribute of the resource
- Maximum
Allowed LineCurrent Maximum Allowed Current Args - attribute of the resource
- Maximum
Allowed LinePower Maximum Allowed Power Args - attribute of the resource
- Maximum
Allowed LineTemperature Maximum Allowed Temperature Args - attribute of the resource
- Maximum
Allowed LineTemperature Lte Maximum Allowed Temperature Lte Args - attribute of the resource
- Maximum
Allowed LineTemperature Ste Maximum Allowed Temperature Ste Args - attribute of the resource
- Name string
- name of the resource
- Number
Of LineConductors Number Of Conductors Args - attribute of the resource
- Reactance
Line
Reactance Args - attribute of the resource
- Reactive
Powers []LineReactive Power Args - attribute of the resource
- Reference
Resistance LineReference Resistance Args - attribute of the resource
- []Line
Related Asset Args - related assets of the resource
- Resistance
Line
Resistance Args - attribute of the resource
- Safety
Margin LineFor Power Safety Margin For Power Args - attribute of the resource
- Susceptance
Line
Susceptance Args - attribute of the resource
- []Line
Tag Args - tags of the resource
- Temperature
Coeff LineResistance Temperature Coeff Resistance Args - attribute of the resource
- Voltage
Rs []LineVoltage RArgs - attribute of the resource
- Voltage
Sts []LineVoltage St Args - attribute of the resource
- Voltage
Trs []LineVoltage Tr Args - attribute of the resource
- absorptivity
Line
Absorptivity - attribute of the resource
- active
Power List<LineEnds Active Power End> - attribute of the resource
- active
Powers List<LineActive Power> - attribute of the resource
- ampacities
List<Line
Ampacity> - attribute of the resource
- atmosphere
Line
Atmosphere - attribute of the resource
- capacitance
Line
Capacitance - attribute of the resource
- conductance
Line
Conductance - attribute of the resource
- current
Rs List<LineCurrent R> - attribute of the resource
- current
S List<LineCurrent> - attribute of the resource
- current
Ts List<LineCurrent T> - attribute of the resource
- currents
List<Line
Current> - attribute of the resource
- description String
- description of the resource
- diameter
Line
Diameter - attribute of the resource
- emissivity
Line
Emissivity - attribute of the resource
- energies
List<Line
Energy> - attribute of the resource
- geometry String
- geo position and shape of the resource
- kinds
List<Line
Kind> - kind of the resource
- length
Line
Length - attribute of the resource
- max
Temperatures List<LineMax Temperature> - attribute of the resource
- maximum
Allowed LineCurrent Maximum Allowed Current - attribute of the resource
- maximum
Allowed LinePower Maximum Allowed Power - attribute of the resource
- maximum
Allowed LineTemperature Maximum Allowed Temperature - attribute of the resource
- maximum
Allowed LineTemperature Lte Maximum Allowed Temperature Lte - attribute of the resource
- maximum
Allowed LineTemperature Ste Maximum Allowed Temperature Ste - attribute of the resource
- name String
- name of the resource
- number
Of LineConductors Number Of Conductors - attribute of the resource
- reactance
Line
Reactance - attribute of the resource
- reactive
Powers List<LineReactive Power> - attribute of the resource
- reference
Resistance LineReference Resistance - attribute of the resource
- List<Line
Related Asset> - related assets of the resource
- resistance
Line
Resistance - attribute of the resource
- safety
Margin LineFor Power Safety Margin For Power - attribute of the resource
- susceptance
Line
Susceptance - attribute of the resource
- List<Line
Tag> - tags of the resource
- temperature
Coeff LineResistance Temperature Coeff Resistance - attribute of the resource
- voltage
Rs List<LineVoltage R> - attribute of the resource
- voltage
Sts List<LineVoltage St> - attribute of the resource
- voltage
Trs List<LineVoltage Tr> - attribute of the resource
- absorptivity
Line
Absorptivity - attribute of the resource
- active
Power LineEnds Active Power End[] - attribute of the resource
- active
Powers LineActive Power[] - attribute of the resource
- ampacities
Line
Ampacity[] - attribute of the resource
- atmosphere
Line
Atmosphere - attribute of the resource
- capacitance
Line
Capacitance - attribute of the resource
- conductance
Line
Conductance - attribute of the resource
- current
Rs LineCurrent R[] - attribute of the resource
- current
S LineCurrent[] - attribute of the resource
- current
Ts LineCurrent T[] - attribute of the resource
- currents
Line
Current[] - attribute of the resource
- description string
- description of the resource
- diameter
Line
Diameter - attribute of the resource
- emissivity
Line
Emissivity - attribute of the resource
- energies
Line
Energy[] - attribute of the resource
- geometry string
- geo position and shape of the resource
- kinds
Line
Kind[] - kind of the resource
- length
Line
Length - attribute of the resource
- max
Temperatures LineMax Temperature[] - attribute of the resource
- maximum
Allowed LineCurrent Maximum Allowed Current - attribute of the resource
- maximum
Allowed LinePower Maximum Allowed Power - attribute of the resource
- maximum
Allowed LineTemperature Maximum Allowed Temperature - attribute of the resource
- maximum
Allowed LineTemperature Lte Maximum Allowed Temperature Lte - attribute of the resource
- maximum
Allowed LineTemperature Ste Maximum Allowed Temperature Ste - attribute of the resource
- name string
- name of the resource
- number
Of LineConductors Number Of Conductors - attribute of the resource
- reactance
Line
Reactance - attribute of the resource
- reactive
Powers LineReactive Power[] - attribute of the resource
- reference
Resistance LineReference Resistance - attribute of the resource
- Line
Related Asset[] - related assets of the resource
- resistance
Line
Resistance - attribute of the resource
- safety
Margin LineFor Power Safety Margin For Power - attribute of the resource
- susceptance
Line
Susceptance - attribute of the resource
- Line
Tag[] - tags of the resource
- temperature
Coeff LineResistance Temperature Coeff Resistance - attribute of the resource
- voltage
Rs LineVoltage R[] - attribute of the resource
- voltage
Sts LineVoltage St[] - attribute of the resource
- voltage
Trs LineVoltage Tr[] - attribute of the resource
- absorptivity
Line
Absorptivity Args - attribute of the resource
- active_
power_ Sequence[Lineends Active Power End Args] - attribute of the resource
- active_
powers Sequence[LineActive Power Args] - attribute of the resource
- ampacities
Sequence[Line
Ampacity Args] - attribute of the resource
- atmosphere
Line
Atmosphere Args - attribute of the resource
- capacitance
Line
Capacitance Args - attribute of the resource
- conductance
Line
Conductance Args - attribute of the resource
- current_
rs Sequence[LineCurrent RArgs] - attribute of the resource
- current_
s Sequence[LineCurrent Args] - attribute of the resource
- current_
ts Sequence[LineCurrent TArgs] - attribute of the resource
- currents
Sequence[Line
Current Args] - attribute of the resource
- description str
- description of the resource
- diameter
Line
Diameter Args - attribute of the resource
- emissivity
Line
Emissivity Args - attribute of the resource
- energies
Sequence[Line
Energy Args] - attribute of the resource
- geometry str
- geo position and shape of the resource
- kinds
Sequence[Line
Kind Args] - kind of the resource
- length
Line
Length Args - attribute of the resource
- max_
temperatures Sequence[LineMax Temperature Args] - attribute of the resource
- maximum_
allowed_ Linecurrent Maximum Allowed Current Args - attribute of the resource
- maximum_
allowed_ Linepower Maximum Allowed Power Args - attribute of the resource
- maximum_
allowed_ Linetemperature Maximum Allowed Temperature Args - attribute of the resource
- maximum_
allowed_ Linetemperature_ lte Maximum Allowed Temperature Lte Args - attribute of the resource
- maximum_
allowed_ Linetemperature_ ste Maximum Allowed Temperature Ste Args - attribute of the resource
- name str
- name of the resource
- number_
of_ Lineconductors Number Of Conductors Args - attribute of the resource
- reactance
Line
Reactance Args - attribute of the resource
- reactive_
powers Sequence[LineReactive Power Args] - attribute of the resource
- reference_
resistance LineReference Resistance Args - attribute of the resource
- Sequence[Line
Related Asset Args] - related assets of the resource
- resistance
Line
Resistance Args - attribute of the resource
- safety_
margin_ Linefor_ power Safety Margin For Power Args - attribute of the resource
- susceptance
Line
Susceptance Args - attribute of the resource
- Sequence[Line
Tag Args] - tags of the resource
- temperature_
coeff_ Lineresistance Temperature Coeff Resistance Args - attribute of the resource
- voltage_
rs Sequence[LineVoltage RArgs] - attribute of the resource
- voltage_
sts Sequence[LineVoltage St Args] - attribute of the resource
- voltage_
trs Sequence[LineVoltage Tr Args] - attribute of the resource
- absorptivity Property Map
- attribute of the resource
- active
Power List<Property Map>Ends - attribute of the resource
- active
Powers List<Property Map> - attribute of the resource
- ampacities List<Property Map>
- attribute of the resource
- atmosphere Property Map
- attribute of the resource
- capacitance Property Map
- attribute of the resource
- conductance Property Map
- attribute of the resource
- current
Rs List<Property Map> - attribute of the resource
- current
S List<Property Map> - attribute of the resource
- current
Ts List<Property Map> - attribute of the resource
- currents List<Property Map>
- attribute of the resource
- description String
- description of the resource
- diameter Property Map
- attribute of the resource
- emissivity Property Map
- attribute of the resource
- energies List<Property Map>
- attribute of the resource
- geometry String
- geo position and shape of the resource
- kinds List<Property Map>
- kind of the resource
- length Property Map
- attribute of the resource
- max
Temperatures List<Property Map> - attribute of the resource
- maximum
Allowed Property MapCurrent - attribute of the resource
- maximum
Allowed Property MapPower - attribute of the resource
- maximum
Allowed Property MapTemperature - attribute of the resource
- maximum
Allowed Property MapTemperature Lte - attribute of the resource
- maximum
Allowed Property MapTemperature Ste - attribute of the resource
- name String
- name of the resource
- number
Of Property MapConductors - attribute of the resource
- reactance Property Map
- attribute of the resource
- reactive
Powers List<Property Map> - attribute of the resource
- reference
Resistance Property Map - attribute of the resource
- List<Property Map>
- related assets of the resource
- resistance Property Map
- attribute of the resource
- safety
Margin Property MapFor Power - attribute of the resource
- susceptance Property Map
- attribute of the resource
- List<Property Map>
- tags of the resource
- temperature
Coeff Property MapResistance - attribute of the resource
- voltage
Rs List<Property Map> - attribute of the resource
- voltage
Sts List<Property Map> - attribute of the resource
- voltage
Trs List<Property Map> - attribute of the resource
Supporting Types
LineAbsorptivity, LineAbsorptivityArgs
LineActivePower, LineActivePowerArgs
LineActivePowerEnd, LineActivePowerEndArgs
LineAmpacity, LineAmpacityArgs
LineAtmosphere, LineAtmosphereArgs
LineCapacitance, LineCapacitanceArgs
LineConductance, LineConductanceArgs
LineCurrent, LineCurrentArgs
LineCurrentR, LineCurrentRArgs
LineCurrentT, LineCurrentTArgs
LineDiameter, LineDiameterArgs
LineEmissivity, LineEmissivityArgs
LineEnergy, LineEnergyArgs
LineKind, LineKindArgs
LineLength, LineLengthArgs
LineMaxTemperature, LineMaxTemperatureArgs
LineMaximumAllowedCurrent, LineMaximumAllowedCurrentArgs
LineMaximumAllowedPower, LineMaximumAllowedPowerArgs
LineMaximumAllowedTemperature, LineMaximumAllowedTemperatureArgs
LineMaximumAllowedTemperatureLte, LineMaximumAllowedTemperatureLteArgs
LineMaximumAllowedTemperatureSte, LineMaximumAllowedTemperatureSteArgs
LineNumberOfConductors, LineNumberOfConductorsArgs
LineReactance, LineReactanceArgs
LineReactivePower, LineReactivePowerArgs
LineReferenceResistance, LineReferenceResistanceArgs
LineRelatedAsset, LineRelatedAssetArgs
LineResistance, LineResistanceArgs
LineSafetyMarginForPower, LineSafetyMarginForPowerArgs
LineSusceptance, LineSusceptanceArgs
LineTag, LineTagArgs
LineTemperatureCoeffResistance, LineTemperatureCoeffResistanceArgs
LineVoltageR, LineVoltageRArgs
LineVoltageSt, LineVoltageStArgs
LineVoltageTr, LineVoltageTrArgs
Import
$ pulumi import splight:index/line:Line [options] splight_line.<name> <line_id>
To learn more about importing existing cloud resources, see Importing resources.
Package Details
- Repository
- splight splightplatform/pulumi-splight
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
splight
Terraform Provider.
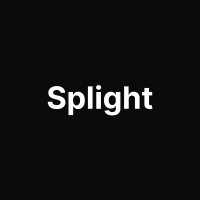