Threefold Grid v0.7.4 published on Thursday, Sep 5, 2024 by Threefold
threefold.Kubernetes
Explore with Pulumi AI
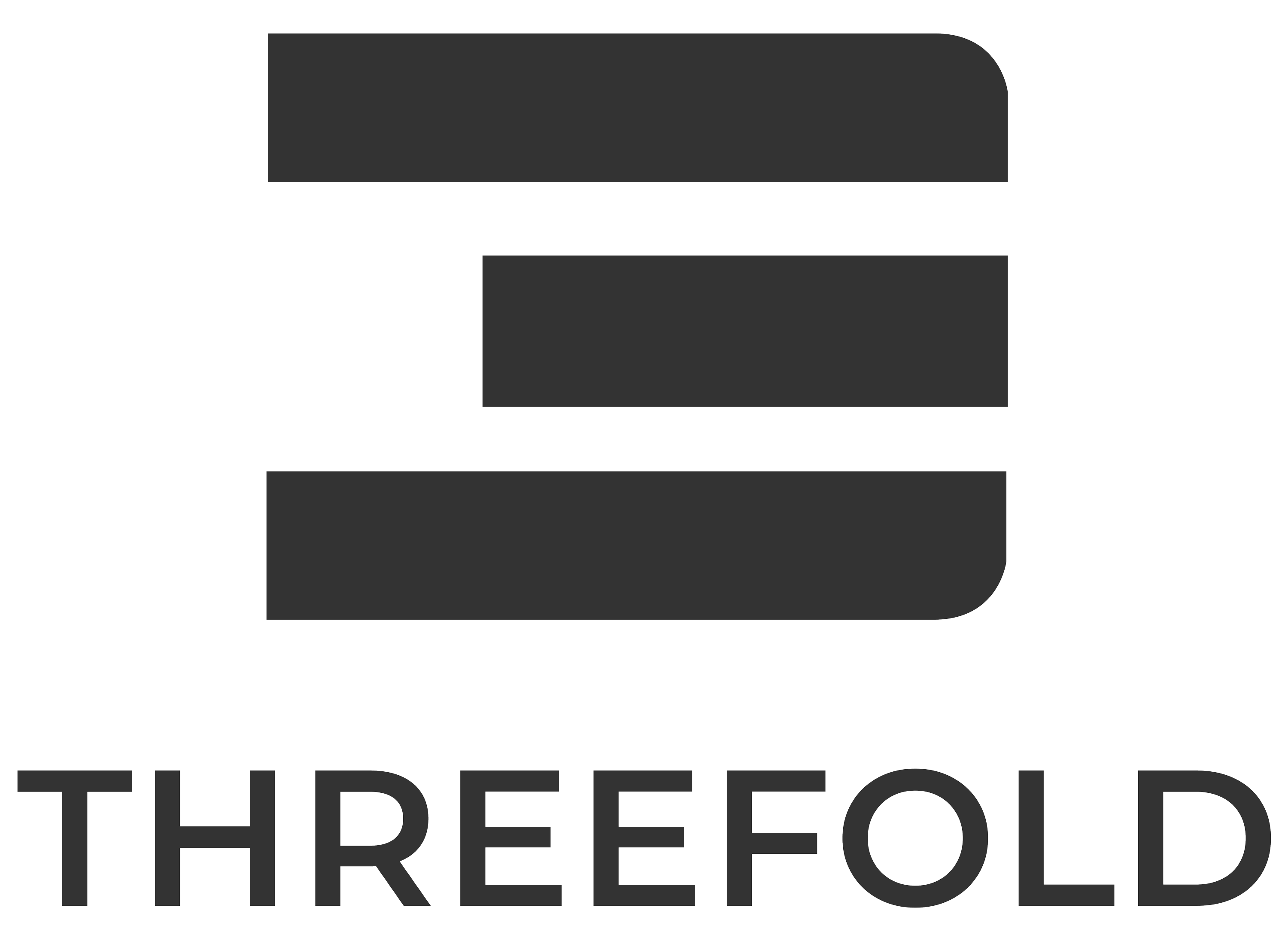
Threefold Grid v0.7.4 published on Thursday, Sep 5, 2024 by Threefold
Create Kubernetes Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new Kubernetes(name: string, args: KubernetesArgs, opts?: CustomResourceOptions);
@overload
def Kubernetes(resource_name: str,
args: KubernetesArgs,
opts: Optional[ResourceOptions] = None)
@overload
def Kubernetes(resource_name: str,
opts: Optional[ResourceOptions] = None,
master: Optional[K8sNodeInputArgs] = None,
network_name: Optional[str] = None,
token: Optional[str] = None,
workers: Optional[Sequence[K8sNodeInputArgs]] = None,
solution_type: Optional[str] = None,
ssh_key: Optional[str] = None)
func NewKubernetes(ctx *Context, name string, args KubernetesArgs, opts ...ResourceOption) (*Kubernetes, error)
public Kubernetes(string name, KubernetesArgs args, CustomResourceOptions? opts = null)
public Kubernetes(String name, KubernetesArgs args)
public Kubernetes(String name, KubernetesArgs args, CustomResourceOptions options)
type: threefold:Kubernetes
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args KubernetesArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args KubernetesArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args KubernetesArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args KubernetesArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args KubernetesArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var kubernetesResource = new Threefold.Kubernetes("kubernetesResource", new()
{
Master = new Threefold.Inputs.K8sNodeInputArgs
{
Name = "string",
Disk_size = 0,
Node = "any",
Cpu = 0,
Memory = 0,
Network_name = "string",
Flist_checksum = "string",
Mycelium_ip_seed = "string",
Mycelium = false,
Flist = "string",
Planetary = false,
Public_ip = false,
Public_ip6 = false,
},
Network_name = "string",
Token = "string",
Workers = new[]
{
new Threefold.Inputs.K8sNodeInputArgs
{
Name = "string",
Disk_size = 0,
Node = "any",
Cpu = 0,
Memory = 0,
Network_name = "string",
Flist_checksum = "string",
Mycelium_ip_seed = "string",
Mycelium = false,
Flist = "string",
Planetary = false,
Public_ip = false,
Public_ip6 = false,
},
},
Solution_type = "string",
Ssh_key = "string",
});
example, err := threefold.NewKubernetes(ctx, "kubernetesResource", &threefold.KubernetesArgs{
Master: &threefold.K8sNodeInputArgs{
Name: pulumi.String("string"),
Disk_size: pulumi.Int(0),
Node: pulumi.Any("any"),
Cpu: pulumi.Int(0),
Memory: pulumi.Int(0),
Network_name: pulumi.String("string"),
Flist_checksum: pulumi.String("string"),
Mycelium_ip_seed: pulumi.String("string"),
Mycelium: pulumi.Bool(false),
Flist: pulumi.String("string"),
Planetary: pulumi.Bool(false),
Public_ip: pulumi.Bool(false),
Public_ip6: pulumi.Bool(false),
},
Network_name: pulumi.String("string"),
Token: pulumi.String("string"),
Workers: threefold.K8sNodeInputArray{
&threefold.K8sNodeInputArgs{
Name: pulumi.String("string"),
Disk_size: pulumi.Int(0),
Node: pulumi.Any("any"),
Cpu: pulumi.Int(0),
Memory: pulumi.Int(0),
Network_name: pulumi.String("string"),
Flist_checksum: pulumi.String("string"),
Mycelium_ip_seed: pulumi.String("string"),
Mycelium: pulumi.Bool(false),
Flist: pulumi.String("string"),
Planetary: pulumi.Bool(false),
Public_ip: pulumi.Bool(false),
Public_ip6: pulumi.Bool(false),
},
},
Solution_type: pulumi.String("string"),
Ssh_key: pulumi.String("string"),
})
var kubernetesResource = new Kubernetes("kubernetesResource", KubernetesArgs.builder()
.master(K8sNodeInputArgs.builder()
.name("string")
.disk_size(0)
.node("any")
.cpu(0)
.memory(0)
.network_name("string")
.flist_checksum("string")
.mycelium_ip_seed("string")
.mycelium(false)
.flist("string")
.planetary(false)
.public_ip(false)
.public_ip6(false)
.build())
.network_name("string")
.token("string")
.workers(K8sNodeInputArgs.builder()
.name("string")
.disk_size(0)
.node("any")
.cpu(0)
.memory(0)
.network_name("string")
.flist_checksum("string")
.mycelium_ip_seed("string")
.mycelium(false)
.flist("string")
.planetary(false)
.public_ip(false)
.public_ip6(false)
.build())
.solution_type("string")
.ssh_key("string")
.build());
kubernetes_resource = threefold.Kubernetes("kubernetesResource",
master=threefold.K8sNodeInputArgs(
name="string",
disk_size=0,
node="any",
cpu=0,
memory=0,
network_name="string",
flist_checksum="string",
mycelium_ip_seed="string",
mycelium=False,
flist="string",
planetary=False,
public_ip=False,
public_ip6=False,
),
network_name="string",
token="string",
workers=[threefold.K8sNodeInputArgs(
name="string",
disk_size=0,
node="any",
cpu=0,
memory=0,
network_name="string",
flist_checksum="string",
mycelium_ip_seed="string",
mycelium=False,
flist="string",
planetary=False,
public_ip=False,
public_ip6=False,
)],
solution_type="string",
ssh_key="string")
const kubernetesResource = new threefold.Kubernetes("kubernetesResource", {
master: {
name: "string",
disk_size: 0,
node: "any",
cpu: 0,
memory: 0,
network_name: "string",
flist_checksum: "string",
mycelium_ip_seed: "string",
mycelium: false,
flist: "string",
planetary: false,
public_ip: false,
public_ip6: false,
},
network_name: "string",
token: "string",
workers: [{
name: "string",
disk_size: 0,
node: "any",
cpu: 0,
memory: 0,
network_name: "string",
flist_checksum: "string",
mycelium_ip_seed: "string",
mycelium: false,
flist: "string",
planetary: false,
public_ip: false,
public_ip6: false,
}],
solution_type: "string",
ssh_key: "string",
});
type: threefold:Kubernetes
properties:
master:
cpu: 0
disk_size: 0
flist: string
flist_checksum: string
memory: 0
mycelium: false
mycelium_ip_seed: string
name: string
network_name: string
node: any
planetary: false
public_ip: false
public_ip6: false
network_name: string
solution_type: string
ssh_key: string
token: string
workers:
- cpu: 0
disk_size: 0
flist: string
flist_checksum: string
memory: 0
mycelium: false
mycelium_ip_seed: string
name: string
network_name: string
node: any
planetary: false
public_ip: false
public_ip6: false
Kubernetes Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
The Kubernetes resource accepts the following input properties:
- Master
K8s
Node Input - Network_
name string - Token string
- Workers
List<K8s
Node Input> - Solution_
type string - Ssh_
key string
- Master
K8s
Node Input Args - Network_
name string - Token string
- Workers
[]K8s
Node Input Args - Solution_
type string - Ssh_
key string
- master
K8s
Node Input - network_
name String - token String
- workers
List<K8s
Node Input> - solution_
type String - ssh_
key String
- master
K8s
Node Input - network_
name string - token string
- workers
K8s
Node Input[] - solution_
type string - ssh_
key string
- master Property Map
- network_
name String - token String
- workers List<Property Map>
- solution_
type String - ssh_
key String
Outputs
All input properties are implicitly available as output properties. Additionally, the Kubernetes resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Master_
computed K8sNode Computed - Node_
deployment_ Dictionary<string, int>id - Nodes_
ip_ Dictionary<string, string>range - Workers_
computed Dictionary<string, K8sNode Computed>
- Id string
- The provider-assigned unique ID for this managed resource.
- Master_
computed K8sNode Computed - Node_
deployment_ map[string]intid - Nodes_
ip_ map[string]stringrange - Workers_
computed map[string]K8sNode Computed
- id String
- The provider-assigned unique ID for this managed resource.
- master_
computed K8sNode Computed - node_
deployment_ Map<String,Integer>id - nodes_
ip_ Map<String,String>range - workers_
computed Map<String,K8sNode Computed>
- id string
- The provider-assigned unique ID for this managed resource.
- master_
computed K8sNode Computed - node_
deployment_ {[key: string]: number}id - nodes_
ip_ {[key: string]: string}range - workers_
computed {[key: string]: K8sNode Computed}
- id str
- The provider-assigned unique ID for this managed resource.
- master_
computed K8sNode Computed - node_
deployment_ Mapping[str, int]id - nodes_
ip_ Mapping[str, str]range - workers_
computed Mapping[str, K8sNode Computed]
- id String
- The provider-assigned unique ID for this managed resource.
- master_
computed Property Map - node_
deployment_ Map<Number>id - nodes_
ip_ Map<String>range - workers_
computed Map<Property Map>
Supporting Types
K8sNodeComputed, K8sNodeComputedArgs
- Computed_
ip string - Computed_
ip6 string - Console_
url string - Ip string
- Mycelium_
ip string - Mycelium_
ip_ stringseed - Planetary_
ip string
- Computed_
ip string - Computed_
ip6 string - Console_
url string - Ip string
- Mycelium_
ip string - Mycelium_
ip_ stringseed - Planetary_
ip string
- computed_
ip String - computed_
ip6 String - console_
url String - ip String
- mycelium_
ip String - mycelium_
ip_ Stringseed - planetary_
ip String
- computed_
ip string - computed_
ip6 string - console_
url string - ip string
- mycelium_
ip string - mycelium_
ip_ stringseed - planetary_
ip string
- computed_
ip str - computed_
ip6 str - console_
url str - ip str
- mycelium_
ip str - mycelium_
ip_ strseed - planetary_
ip str
- computed_
ip String - computed_
ip6 String - console_
url String - ip String
- mycelium_
ip String - mycelium_
ip_ Stringseed - planetary_
ip String
K8sNodeInput, K8sNodeInputArgs
- Cpu int
- Disk_
size int - Memory int
- Name string
- Network_
name string - Node object
- Flist string
- Flist_
checksum string - Mycelium bool
- Mycelium_
ip_ stringseed - Planetary bool
- Public_
ip bool - Public_
ip6 bool
- Cpu int
- Disk_
size int - Memory int
- Name string
- Network_
name string - Node interface{}
- Flist string
- Flist_
checksum string - Mycelium bool
- Mycelium_
ip_ stringseed - Planetary bool
- Public_
ip bool - Public_
ip6 bool
- cpu Integer
- disk_
size Integer - memory Integer
- name String
- network_
name String - node Object
- flist String
- flist_
checksum String - mycelium Boolean
- mycelium_
ip_ Stringseed - planetary Boolean
- public_
ip Boolean - public_
ip6 Boolean
- cpu number
- disk_
size number - memory number
- name string
- network_
name string - node any
- flist string
- flist_
checksum string - mycelium boolean
- mycelium_
ip_ stringseed - planetary boolean
- public_
ip boolean - public_
ip6 boolean
- cpu int
- disk_
size int - memory int
- name str
- network_
name str - node Any
- flist str
- flist_
checksum str - mycelium bool
- mycelium_
ip_ strseed - planetary bool
- public_
ip bool - public_
ip6 bool
- cpu Number
- disk_
size Number - memory Number
- name String
- network_
name String - node Any
- flist String
- flist_
checksum String - mycelium Boolean
- mycelium_
ip_ Stringseed - planetary Boolean
- public_
ip Boolean - public_
ip6 Boolean
Package Details
- Repository
- threefold threefoldtech/pulumi-threefold
- License
- Apache-2.0
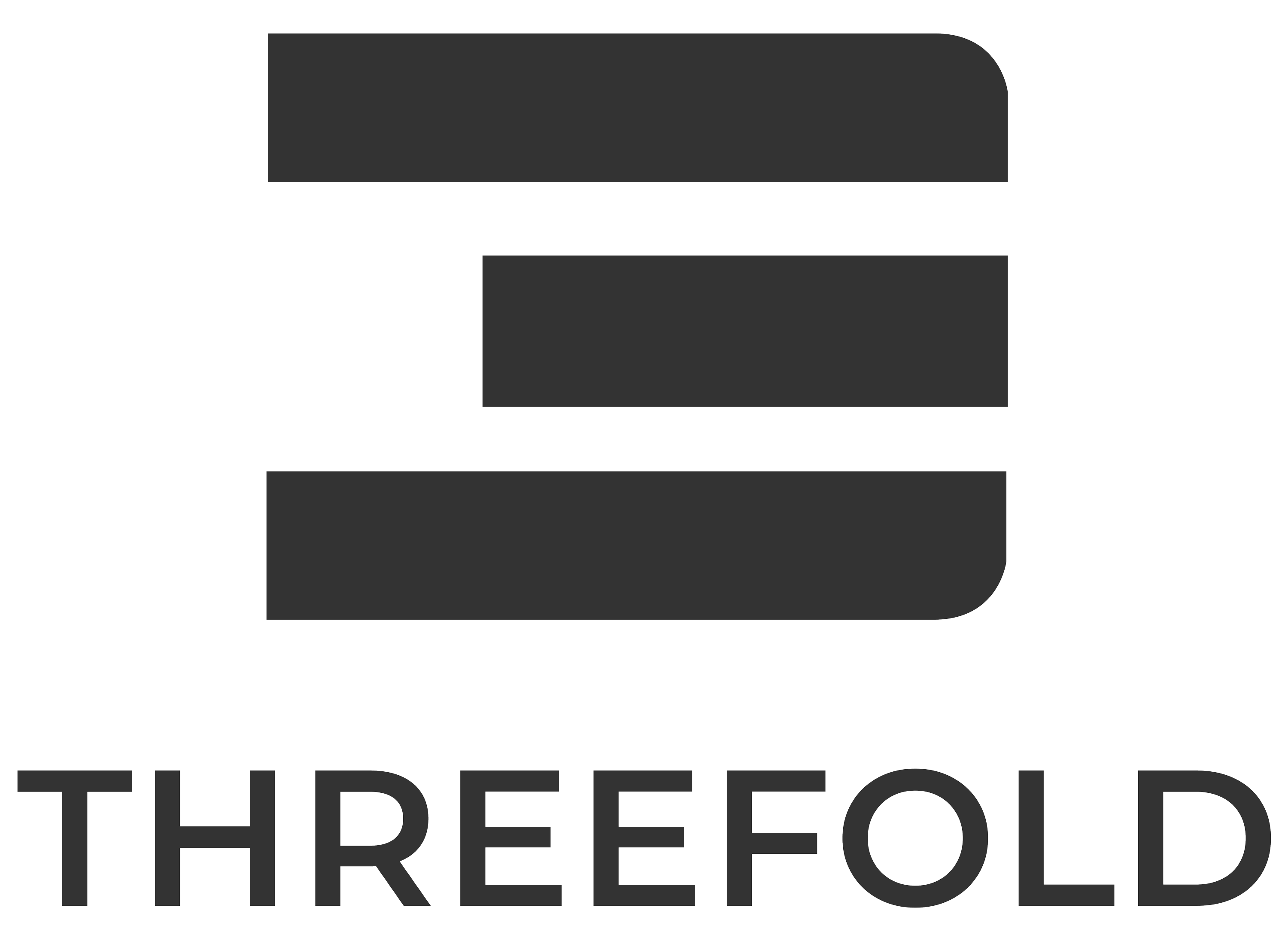
Threefold Grid v0.7.4 published on Thursday, Sep 5, 2024 by Threefold